ADO.NET provides different types of support for data entry
into a table. For example, you can use the classic INSERT INTO expression
from the SQL. You can also create a stored procedure that internally creates a
new record. Besides the INSERT expression or a stored procedure, to
perform data entry, you can use a DataSet object.
The DataSet class is equipped with a Tables
property, which is a collection of the tables that are part of the data set. The
Tables property is an object of type DataTableCollection. As its
name indicates, the DataTableCollection itself is a list of tables and
each table can be located using an index, either by the numeric order of the
table or its name. The DataTableCollection class supports this through an
Item property which is based on the DataTable class. This
relationship makes it possible to access the contents of a table. Fundamentally,
a table is made of columns and records and each record is also called a row.
The records of a table are stored in a member of the DataTable
called Rows. The Rows property is an object of type DataRowCollection.
An individual record is an object of type DataRow. To represent each
record, the DataRowCollection is equipped with an Item property which is
of type DataRow. To perform data entry, you can assign a value to the
name of each column of the desired table of your database. Each column is
accessible through the Item property of the DataRow class. One of
the overloaded versions of this property takes a String value as its
index.
After assigning the right value to each DataRow::Item
that corresponds to the desired column of a table, you can call the Update()
method of the data adapter you were using. This means that, previously,
you should have initialized a data adapter using OLEDB.
Practical Learning: Performing Data Entry With DataRow
|
|
- Start Microsoft Access and create a Blank Database named CarInventory1
- In the Tables section of the Database window, double-click Create Table In
Design View
- Complete it as follows:
Field Name |
Data Type |
Length |
Properties |
CarID |
AutoNumber |
|
Primary Key |
TagNumber |
Text |
20 |
Indexed: Yes(No Duplicate) |
Make |
Text |
40 |
|
Model |
Text |
40 |
|
CarYear |
Text |
10 |
|
Category |
Text |
40 |
Row Source Type: Value List
Row Source: "Economy";"Compact";"Standard";"Full Size";"Mini Van";"SUV";"Truck";"Van" |
HasK7Player |
Yes/No |
|
|
HasCDPlayer |
Yes/No |
|
|
HasDVDPlayer |
Yes/No |
|
|
CarPicture |
Text |
240 |
|
IsAvailable |
Yes/No |
|
|
- Save the table as Cars and close it
- Close Microsoft Access
- Start Microsoft Visual C++ .NET or Microsoft Visual Studio .NET and create
a Windows Forms Application named CarInventory3
- Design the form as follows:
 |
Control |
Text |
Name |
Other Properties |
Label |
Text # |
|
|
TextBox |
|
txtTagNumber |
|
Label |
Make: |
|
|
TextBox |
|
txtMake |
|
Label |
Model: |
|
|
TextBox |
|
txtModel |
|
Label |
Year: |
|
|
TextBox |
|
txtYear |
|
Label |
Category: |
|
|
ComboBox |
|
cboCategory |
DropDownStyle: DropDownList |
Items: |
Economy
Compact
Standard
Full Size
Mini Van
SUV
Truck
Van |
CheckBox |
Cassete Player |
chkK7Player |
CheckAlign: MiddleRight |
CheckBox |
DVD Player |
chkDVDPlayer |
CheckAlign: MiddleRight |
CheckBox |
CD Player |
chkCDPlayer |
CheckAlign: MiddleRight |
CheckBox |
Available |
chkAvailable |
CheckAlign: MiddleRight |
PictureBox |
|
pctCar |
SizeMode: CenterImage |
Button |
Picture |
btnPicture |
|
TextBox |
|
txtPicture |
|
Button |
Add Car |
btnAddCar |
|
Button |
Close |
btnClose |
DialogResult: OK |
Form |
|
|
AcceptButton: btnAddCar
MaximizeBox: False
StartPosition: CenterScreen |
|
- In the Windows Forms section of the Toolbox, click the OpenFileDialog
button and click the form
- Set its Filter as Picture Files (*.bmp;*.gif;*.jpeg;*jpg)|*.bmp;*.gif;*.jpeg;*jpg
- Set its Title to Select Car Picture
- On the form, double-click the Picture button and implement its event as
follows:
private void btnPicture_Click(object sender, System.EventArgs e)
{
if( openFileDialog1.ShowDialog() == DialogResult.OK )
{
this.txtPicture.Text = openFileDialog1.FileName;
this.pctCar.Image = Bitmap.FromFile(openFileDialog1.FileName);
}
}
|
- Return to the form
- From the Data section of the Toolbox, click OleDbDataAdapter and click the
form
- In the first page of the Data Adapter Configuration Wizard, click Next
- In the second page of the wizard, click New Connection
- In the Data Link Properties, click the Provider property page
- In the Provider tab, click Microsoft Jet 4.0 OLE DB Provider

- Click Next
- In the Connection page, click the ellipsis button on the right side of 1
- Locate and select the CarInventory1 database you created above and click
Open
- Click Test Connection and click OK twice
- In the second page of the wizard, click Next
- In the third page, accept the first radio button (Use SQL Statement) and
click Next
- In the fourth page, click Query Builder
- In the Add Table dialog box, as Cars is selected, click Add and click
Close
- In the list of fields, click the check box on the left of the * column
- Click OK
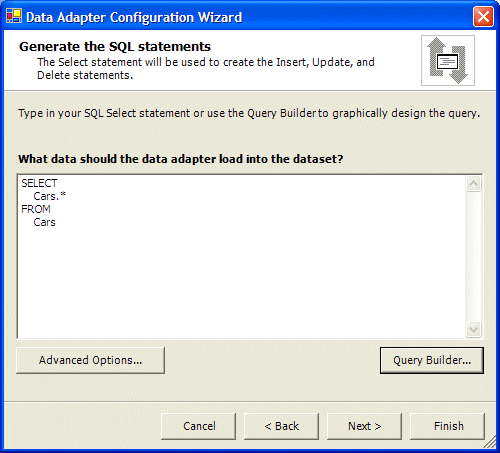
- Click Next and click Finish. For this exercise, if a message box comes up,
click Don't Include Password
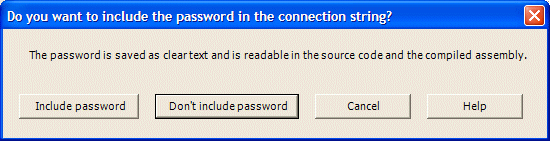
- On the main menu, click Data -> Generate Dataset...
- While the New radio button is selected, change the name of the dataset to dsCarInventory
and click OK
- Double-click an empty area of the form and change the Load event as
follows:
private void Form1_Load(object sender, System.EventArgs e)
{
this.oleDbDataAdapter1.Fill(this.dsCarInventory1);
}
|
- Return to the form and double-click the Add Car followed by the Close
buttons
- Implement the events as follows:
private void btnAddCar_Click(object sender, System.EventArgs e)
{
DataTable tblCars = new DataTable();
tblCars = this.dsCarInventory1.Tables["Cars"];
DataRow rowNewCar;
rowNewCar = tblCars.NewRow();
rowNewCar["TagNumber"] = this.txtTagNumber.Text;
rowNewCar["Make"] = this.txtMake.Text;
rowNewCar["Model"] = this.txtModel.Text;
rowNewCar["CarYear"] = this.txtYear.Text;
rowNewCar["Category"] = this.cboCategory.Text;
rowNewCar["HasK7Player"] = this.chkK7Player.Checked.ToString();
rowNewCar["HasCDPlayer"] = this.chkCDPlayer.Checked.ToString();
rowNewCar["HasDVDPlayer"] = this.chkDVDPlayer.Checked.ToString();
rowNewCar["CarPicture"] = this.txtPicture.Text;
rowNewCar["IsAvailable"] = this.chkAvailable.Checked.ToString();
tblCars.Rows.Add(rowNewCar);
this.oleDbDataAdapter1.Update(this.dsCarInventory1);
this.txtTagNumber.Text = "";
this.txtMake.Text = "";
this.txtModel.Text = "";
this.txtYear.Text = "";
this.cboCategory.SelectedIndex = -1;
this.chkK7Player.Checked = false;
this.chkCDPlayer.Checked = false;
this.chkDVDPlayer.Checked = false;
this.txtPicture.Text = "";
this.chkAvailable.Checked = false;
this.pctCar.Image = null;
this.txtTagNumber.Focus();
}
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Execute the application and create a record (you can first download
a few pictures of cars):

- Click Add Car to submit the record
- Click Close to close the form
|
|