 |
Operators and Operands |
|
Fundamental Visual Basic Operators
Introduction
An operation is an action performed on one or more
values either to modify the value held by one or both of the variables, or
to produce a new value by combining existing values. Therefore, an operation
is performed using at least one symbol and at least one value. The symbol
used in an operation is called an operator. A value involved in an operation
is called an operand.
A unary operator is an operator that performs its
operation on only one operand. An operator is referred to as binary if it
operates on two operands.
The Line Continuation Operator: _
If you plan to write a long piece of code, to make it
easier to read, you may need to divide it in various lines. You can do it
as you would in any text editor. Here is an example:
<%
Dim Message
Message =
"Welcome to our website."
%>
You can use the line continuation operator represented by a white space followed by an
underscore and an empty space. Here is an example:
<%
Dim Message
Message = _
"Welcome to our website."
%>
The Colon Operator :
To make various statements easier to read, you usually write each on its own line. The Visual Basic language allows you
to write as many statements as possible on the same line. To do this, the
statements must be separated by colons. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Kolo Bank - Customer Record</title>
</head>
<body>
<h2>Kolo Bank - Customer Record</h2>
<%
Dim CustomerName
Dim AccountNumber
CustomerName = "James Reinder" : AccountNumber = "928-3947-025"
Response.Write("Customer: ") : Response.Write(CustomerName) : Response.Write(" - ") : Response.Write(AccountNumber)
%>
</body>
</html>
This would produce:
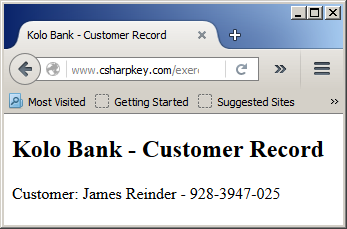
String Concatenation: &
The & operator is used to add two strings or
expressions. This is considered as concatenating them. The formula to follow is:
value1 & value2
To display a concatenated expression, use the
assignment operator on the field. To assign a concatenated expression to a
variable, use the assignment operator the same way:
<%
Dim FirstName
Dim LastName
Dim FullName
FirstName = "Francis "
LastName = "Pottelson"
FullName = FirstName & LastName
%>
To concatenate more than two expressions, you can use
as many & operators between any two strings or expressions as necessary.
After concatenating the expressions or values, you can assign the result
to another variable or expression using the assignment operator.
Carriage Return-Line Feed
If you are displaying a string but judge it too long,
you can segment it in appropriate sections as you see fit. To do this, use vbCrLf.
Using a Tab
To get the effect of pressing the Tab key on the computer keyboard, you can use the vbTab operator of the Visual Basic language.
The Addition Operation
Introduction
The positive operator is used as a unary operator to
indicate that a number is positive. The addition operation, represented by
the + symbol, is used to add numeric values.
Incrementing a Variable
To increment a value, add 1 to it. After adding 1, the
value or the variable is (permanently) modified and the variable would
hold the new value. This is illustrated in the following example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Incrementing a Variable</title>
</head>
<body>
<h2>Incrementing a Variable</h2>
<%
Dim Value = 12
Response.Write("Techniques of incrementing a value")
Response.Write("<br>Value = ")
Response.Write(Value)
Value = Value + 1
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This would produce:
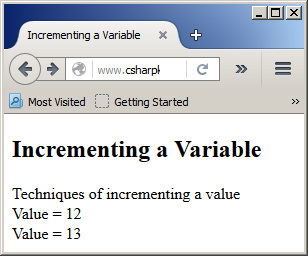
Compound Addition
As you may be aware aready, you can add a constant
value to a variable. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Compound Addition</title>
</head>
<body>
<h2>Compound Addition</h2>
<%
Dim Value = 12.75
Dim NewValue As Double
Response.Write("Techniques of incrementing and decrementing a value")
Response.Write("<br>Value = ")
Response.Write(value)
NewValue = Value + 2.42
Response.Write("<br>Value = ")
Response.Write(NewValue)
%>
</body>
</html>
This would produce:

To add a value to a variable and change the value that
the variable is holding, you can combine the assignment "=" and the
addition "+" operators to produce a new operator as +=. Here is an
example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Compound Addition</title>
</head>
<body>
<h2>Compound Addition</h2>
<%
Dim Value = 12.75
Dim NewValue As Double
Response.Write("Techniques of incrementing and decrementing a value")
Response.Write("<br>Value = ")
Response.Write(Value)
Value += 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This code would produce the same result as above.
The Subtraction Operations
Introduction
The negative operator must be used to indicate that a
number is negative. Examples are -12, -4.48, or -32706. The subtraction
operation is used to take out or subtract a value from another value.
Decrementing a Variable
Decrementing a variable consists of subtracting 1 from
it. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Decrementing a Variable</title>
</head>
<body>
<h2>Decrementing a Variable</h2>
<%
Dim Value = 12
Response.Write("Techniques of decrementing a value")
Response.Write("<br>Value = ")
Response.Write(Value)
Value = Value - 1
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This would produce:
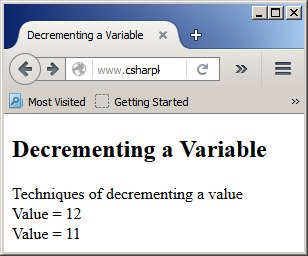
Compound Subtraction
To decrement a value from a variable, use the -=
operator. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Value = 12.75
Response.Write("Techniques of incrementing and decrementing a value")
Response.Write("<br>Value = ")
Response.Write(Value)
Value -= 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This would produce:
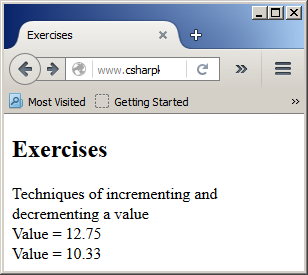
The Multiplication Operations
Introduction
The multiplication allows adding one value to itself a
certain number of times, set by a second value. Like the addition, the
multiplication is associative: a * b * c = c * b * a.
Exponentiation ^
Exponentiation is the ability to raise a number to the
power of another number. This operation is performed using the ^ operator
(Shift + 6). It uses the following formula:
yx
In Microsoft Visual Basic, this formula is written as:
y^x
and means the same thing. Either or both y and x can be
values, variables, or expressions, but they must carry valid values that can
be evaluated.
Compound Multiplication
As seen with the addition, you can multiply a constant
value by a variable. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Value = 12.75
Response.Write("Value = ")
Response.Write(Value)
Value = Value * 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This would produce:
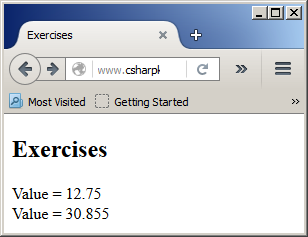
To make this operation easy, the C# language supports
the compound multiplication assignment operator represented as *=. To use
it, apply the *= operator to the variable and assign the desired value. Here
is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Value = 12.75
Response.Write("Value = ")
Response.Write(Value)
Value *= 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
The Division Operation
Introduction
The division operation consists of cutting a number in
pieces or fractions. When performing the division, be aware of its many
rules. Never divide by zero (0). Make sure that you know the relationship(s)
between the numbers involved in the operation.
Integer Division \
The Visual Basic language supports two types of divisions. If you want the result of the operation to be a
natural number, called an integer, use the backlash operator "\" as the
divisor. The formula to follow is:
Value1 \ Value2
This operation can be performed on two types of valid
numbers, with or without decimal parts. After the operation, the result
would be a natural number. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Numerator = 12.75, Denominator = 3.59
Dim Division = Numerator \ Denominator
Response.Write(CStr(Numerator) & " \ " & CStr(Denominator) & " = " & CStr(Division))
%>
</body>
</html>
This would produce:
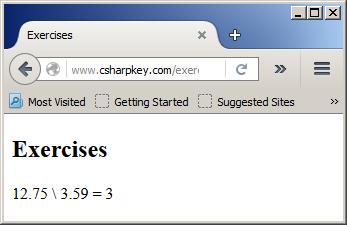
Decimal Division /
The second type of division results in a decimal number.
It is performed with the forward slash "/". Its formula is:
Value1 / Value2
After the operation is performed, the result is a
decimal number. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Numerator = 12.75, Denominator = 3.59
Dim Division = Numerator / Denominator
Response.Write(CStr(Numerator) & " / " & CStr(Denominator) & " = " & CStr(Division))
%>
</body>
</html>
This would produce:

Compound Division
As you can add, subtract, or multiply a value to a
variable and assign the result to the variable itself, you can also divide a
variable by a constant value. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Compound Division</title>
</head>
<body>
<h2>Compound Division</h2>
<%
Dim Value = 12.75
Response.Write("Value = ")
Response.Write(Value)
Value = Value / 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
This would produce:
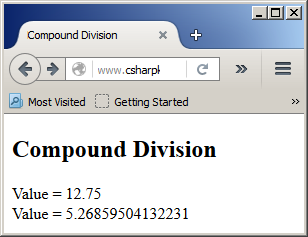
A shortcut of this operation uses the /= operator. Here is an example of using it:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Compound Division</title>
</head>
<body>
<h2>Compound Division</h2>
<%
Dim Value = 12.75
Response.Write("Value = ")
Response.Write(Value)
Value /= 2.42
Response.Write("<br>Value = ")
Response.Write(Value)
%>
</body>
</html>
The Remainder
The remainder operation is usedt to get the value remaining after a division renders a natural result. The remainder operation is performed using the Mod operator. Its formula is:
value1 Mod value2
The result of the operation can be used as you see fit or you can display it in a control or be involved in another operation or expression.
As seen with the other arithmetic operators, you can find the remainder of a variable and assign the result to the variable itself. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercises</title>
</head>
<body>
<h2>Exercises</h2>
<%
Dim Players = 18
' When the game starts, how many players will wait?.
Response.Write("Out of ")
Response.Write(Players)
Response.Write(" players, ")
Players = Players Mod 11
Response.Write(Players)
Response.Write(" players will have to wait when the game starts.")
%>
</body>
</html>
This would produce:

|