 |
Introduction to Procedures and Functions |
|
Introduction to Procedures
Overview
A procedure is a section of instructions that solve
a relatively small problem. There are two categories of procedures you will use in your programs:
those that have already been created and are available to you, and those
you will create yourself.
Creating a Procedure
To create a procedure, start by typing the Sub
keyword followed by a name (like everything else, a procedure must have a
name). At the end of the procedure, you must type End Sub.
Therefore, the primary formula of a procedure is:
Sub procedure-name()
End Sub
A procedure is created in a script. Therefore, its code can be included in a script element. This would be done as follows:
<script runat="server">
Sub procedure-name()
End Sub
</script>
The name of a procedure follows the rules of
variables in the Visual Basic language. The section between the Subprocedure-name() and the End Sub
lines is referred to as the body of the procedure. The body of the procedure is used to define what, and
how, the assignment should be carried. Here is an example:
<script runat="server">
Sub Describe()
Response.Write("Helium is a chemical element found, or used, in solids, in liquids, and in gases.")
End Sub
</script>
Iff you need to use a
variable inside the procude, you can declare it and use it.
Calling a Sub Procedure
Once you have a procedure, whether you created it or
it is part of the Visual Basic language, you can use it. Using a procedure
is also referred to as calling it. To call a simple procedure, type its name followed by parentheses in the section
where you want to use it. To call a procedure in the body section of a webpage, you can do it side the <% and %> delimiters. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub Describe()
Response.Write("Helium is a chemical element found, or used, in solids, in liquids, and in gases.")
End Sub
</script>
<title>Chemistry - Helium</title>
</head>
<body>
<%
Describe()
%>
</body>
</html>
This would produce:
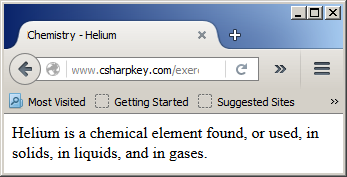
Besides using the name of a
procedure to call it, you can also precede it with the Call
keyword. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub Display()
Response.Write("Welcome to our website.")
End Sub
</script>
<title>Exercises</title>
</head>
<body>
<%
Call Display()
%>
</body>
</html>
Introduction to Functions
Introduction
Like a procedure, a function is a sub-program used to
perform an assignment. The main difference between a procedure and a function is
that, after carrying its assignment, a function gives back a result. We also say
that a function "returns a value".
Creating a Function
To create a function, you use the Function
keyword followed by a name and parentheses. Unlike a procedure, because a
function returns a value, you must specify the type of value the function
will produce. To give this information, on the right side of the closing
parenthesis, you can type the As keyword, followed by a data type.
To indicate where a function stops, type End Function. Based on this,
the minimum formula to create a function is:
AccessModifier(s) Function function-name() As data-type
End Function
As seen for procedures, a function can be created in a
script section. Other than that, a function can start as follows:
<script runat="server">
Function GetFullName() As String
End Function
</script>
As done with variables, you can also use a type
character as the return type of a function and omit the As
DataType expression. The type character is typed on the right side of
the function name and before the opening parenthesis. An example would be
GetFullname$(). As with the variables, you must use the appropriate type
character for the function:
Character |
The function must return |
$ |
A String type |
% |
A Byte, Short, Int16, or In32 |
& |
An Int64 or a Long |
! |
A Single type |
# |
A Double |
@ |
A Long integer |
Here is an example:
<script runat="server">
Function GetFullName$()
End Function
</script>
As mentioned already, the section between the
Function function-name() and the End Function lines is the body of the
function. It is used to describe what the function does. As done on a sub
procedure, one of the actions you can perform in a function is to declare
a (local) variable and use it as you see fit. Here is an example:
<script runat="server">
Function CalculateNetPay() As Double
Dim HourlySalary = 48.26
Dim TimeWorked = 36.50
Dim Multiplication As Double
Multiplication = HourlySalary * TimeWorked
End Function
</script>
Returning a Value From a Function
After performing an assignment in a function, to
indicate the value it returns, before
the End Function line, you can type the name of the function,
followed by the = sign, followed by the value the function returns. Here
is an example in which a function returns a name:
<script runat="server">
Function CalculateNetPay() As Double
Dim HourlySalary = 48.26
Dim TimeWorked = 36.50
Dim Multiplication As Double
Multiplication = HourlySalary * TimeWorked
CalculateNetPay = Multiplication
End Function
</script>
As an alternative, in place of the name of the function and =, type the Return keyword followed by the value or the expression that the function
returns. Here is an example:
<script runat="server">
Function CalculateNetPay() As Double
Dim HourlySalary = 48.26
Dim TimeWorked = 36.50
Dim Multiplication As Double
Multiplication = HourlySalary * TimeWorked
Return Multiplication
End Function
</script>
As you can see from the above example, you can use a local variable that would carry the returned value. As an alternative, you can return an expression from the function. This can be done as follows:
<script runat="server">
Function CalculateNetPay() As Double
Dim HourlySalary = 48.26
Dim TimeWorked = 36.50
Dim Multiplication As Double
Multiplication = HourlySalary * TimeWorked
' This
CalculateNetPay = HourlySalary * TimeWorked
' or this
' Return HourlySalary * TimeWorked
End Function
</script>
 |
New Convention:
From now on, in our lessons, to refer to a procedure or
function, we will use the name of a procedure followed by
parentheses. For example, we may write "the Convert() procedure"
or "the Convert() function".
|
Calling a Function
In order to use a function, you must call it. Like a sub procedure, to call
a function, you can simply type its name in the desired section of your code. Since the primary purpose of a function is to return a
value, to better take advantage of such a value, you can assign the name
of the function to a variable in the section where you are calling the
function. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Function CalculateNetPay() As Double
Dim HourlySalary = 48.26
Dim TimeWorked = 36.50
Dim Multiplication As Double
Multiplication = HourlySalary * TimeWorked
CalculateNetPay = Multiplication
End Function
</script>
<title>Exercises</title>
</head>
<body>
<%
Dim Result As Double
Result = CalculateNetPay()
Response.Write("Net Pay: ")
Response.Write(Result)
%>
</body>
</html>
This would produce:
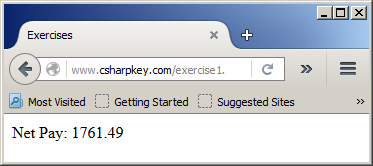
The Scope and Lifetime of a Variable
Local Variables
The scope of a variable determines the areas of code
where the variable is available. A variable declared inside of a procedure or function is referred to as a local variable. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Sub PresentRectangle()
' A local variable
Dim Height = 27.19
Response.Write("Geometry: Rectangle")
Response.Write("<br>Height: " & CStr(Height))
End Sub
</script>
<title>Exercises</title>
</head>
<body>
<%
PresentRectangle()
%>
</body>
</html>
This would produce:
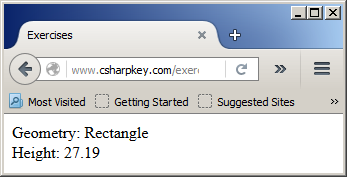
Such as variable cannot be accessed by another function or another procedure created in the webpage.
Global Variables
A variable that is declared outside of any procedure or function is
referred to as global. To declare a global variable, use the same formula as
we have done so far. You can type Dim, followed by the name of the variable, the As
keyword, its type and an optional initialization. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
' A global variable
Dim Width = 48.16
Sub PresentRectangle()
' A local variable
Dim Height = 27.19
Response.Write("Geometry: Rectangle")
Response.Write("<br>Width: " & Width)
Response.Write("<br>Height: " & Height)
End Sub
</script>
<title>Exercises</title>
</head>
<body>
<%
PresentRectangle()
%>
</body>
</html>
This would produce:

You can initialize the global variable when or after declaring it.
Access Modifiers
Friendly Members
A global variable declared in the code of a webpage is referred to as a
friend. To declare a friendly variable, instead of Dim,
use the Friend keyword. Here is an example:
<script runat="server">
Friend Length = 117.09
Sub Present()
End Sub
</script>
You can also declare many friendly variables with one Friend keyword. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Friend AreaOfStudy = "Social Science", Major = "Psychology", Degree = "Bachelor of Art (B.A.)"
Sub Display()
Response.Write("Area of Study: " & AreaOfStudy)
Response.Write("<br>Major: " & Major)
Response.Write("<br>Degree: " & Degree)
End Sub
</script>
<title>Exercises</title>
</head>
<body>
<%
Display()
%>
</body>
</html>
This would produce:
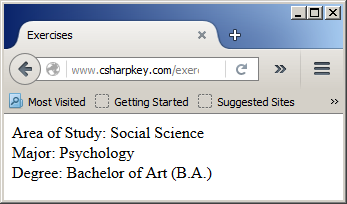
After declaring a friendly variable, if your website has many files with code, the variable can be accessed from other files.
Private Members
You can declare a variable that can be accessed only within the file that contains it. Such a variable is referred to as private.
To declare a private variable, in place of Dim or Friend,
use the Private keyword. Here is an example:
<script runat="server">
Private Side = 34.85
Sub Present()
End Sub
</script>
Public Members
A variable can be made publicly available. To declare such a variable, use the Public keyword. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<script runat="server">
Friend FullName As String
Private DateHired As String
Public HourlySalary As Double
Sub Display()
FullName = "Abigail Ammos"
DateHired = "4-8-2016"
HourlySalary = 36.75
Dim Information As String
Information = "Full Name: " & FullName &
"<br>Date Hired: " & DateHired &
"<br>Hourly Salary: " & HourlySalary
Response.Write(Information)
End Sub
</script>
<title>Exercises</title>
</head>
<body>
<%
Display()
%>
</body>
</html>
This would produce:
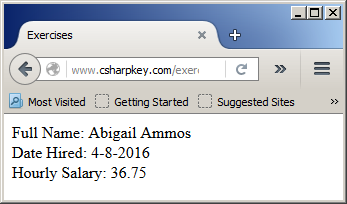
Access Modifiers Applied to Procedures and Functions
Like a variable, a procedure or a function can use access modifiers.
A procedure or function can be made private, friendly, or
public, using the Private, the Friend, or the
Public keywords respectively:
- Private: A procedure or function marked as private can be called only
by members of the same code section
- Friend: A friendly procedure or function can be called by members of
the code section and other files of the same project
- Public: A public procedure can be called from its code
section and other code sections
|