|
A conditional statement is an expression that produces a
true or false result. To create the
expression, you use a Boolean operator and a logical keyword.
|
Practical
Learning: Introducing Conditional Expressions
|
|
- Start Microsoft Visual Studio or Microsoft Visual Web Developer
- Start creating a web site
- Set the language to Visual C# et set the site name to timesheet1
- Click OK
- In the Solution Explorer, right-click Default.aspx and click Rename
- Type index.aspx and press Enter
- Click the Source button and change the file as follows:
<%@ Page Language="C#"
AutoEventWireup="true"
CodeFile="index.aspx.cs"
Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Time Sheet</title>
</head>
<body>
<form id="frmTimeSheet" runat="server">
<div>
<table border="0" width="600">
<tr>
<td width="80"></td>
<td align="center">Monday</td>
<td align="center">Tuesday</td>
<td align="center">Wednesday</td>
<td align="center">Thursday</td>
<td align="center">Friday</td>
<td align="center">Saturday</td>
<td align="center">Sunday</td>
</tr>
<tr>
<td>Week 1:</td>
<td align="center">
<asp:TextBox ID="txtWeek1Monday"
runat="server"
Columns="5">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Tuesday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Wednesday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Thursday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Friday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Saturday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek1Sunday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
</tr>
<tr>
<td>Week 2:</td>
<td align="center">
<asp:TextBox ID="txtWeek2Monday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Tuesday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Wednesday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Thursday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Friday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Saturday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
<td align="center">
<asp:TextBox ID="txtWeek2Sunday"
Columns="5" runat="server">0.00</asp:TextBox>
</td>
</tr>
</table>
<table width="600">
<tr>
<td align="center">
<asp:Button ID="btnEvaluate" Width="200"
Text="Evaluate Time Sheet" runat="server" />
</td>
</tr>
</table>
<table border="0" width="300">
<tr>
<td width="33%"></td>
<td align="center">Regular Time</td>
<td align="center">Overtime</td>
</tr>
<tr>
<td align="center">Week 1</td>
<td width="33%" align="center">
<asp:TextBox ID="txtWeek1RegularTime"
Columns="10" runat="server">0.00</asp:TextBox>
</td>
<td width="34%" align="center">
<asp:TextBox ID="txtWeek1Overtime"
Columns="10" runat="server">0.00</asp:TextBox>
</td>
</tr>
<tr>
<td width="33%" align="center">Week 2</td>
<td width="33%" align="center">
<asp:TextBox ID="txtWeek2RegularTime"
Columns="10" runat="server">0.00</asp:TextBox>
</td>
<td width="34%" align="center">
<asp:TextBox ID="txtWeek2Overtime"
Columns="10" runat="server">0.00</asp:TextBox>
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
|
- Save the file and click the Design button

Consider the following code:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
private void IndexHouseSelected(object sender, EventArgs e)
{
lblSelection.Text = TypesOfHouses.Text;
}
</script>
<title>Altair Real Estate</title>
</head>
<body>
<h3>Altair Real Estate</h3>
<form id="frmRealEstate" method="post" runat="server">
<table>
<tr>
<td>What type of property do you want to purchase?</td>
<td>
<asp:DropDownList id="TypesOfHouses"
AutoPostBack="True"
OnSelectedIndexChanged="IndexHouseSelected" runat="server">
<asp:ListItem>Condominium</asp:ListItem>
<asp:ListItem>Townhouse</asp:ListItem>
<asp:ListItem>Single Family</asp:ListItem>
<asp:ListItem>Unknown</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Label id="lblSelection" runat="server"></asp:Label></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of using it:
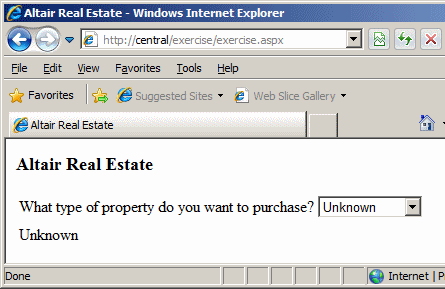
To check if an expression is true and use its Boolean
result, you can use the if operator. Its formula is:
if(Condition) Statement;
The Condition can be a type of Boolean operation. That is, it can have the following formula:
Operand1 BooleanOperator Operand2
If the Condition produces a true result, then the Statement
is executed. If the statement to execute is short,
you can write it on the same line with the condition that is being checked. Here
is an example:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
private void IndexHouseSelected(object sender, EventArgs e)
{
int choice = TypesOfHouses.SelectedIndex;
string description = "Unknow means the property cannot be clearly described";
if( choice == 3 ) lblSelection.Text = description;
}
</script>
<title>Altair Real Estate</title>
</head>
<body>
<h3>Altair Real Estate</h3>
<form id="frmRealEstate" method="post" runat="server">
<table>
<tr>
<td>What type of property do you want to purchase?</td>
<td>
<asp:DropDownList id="TypesOfHouses"
AutoPostBack="True"
OnSelectedIndexChanged="IndexHouseSelected" runat="server">
<asp:ListItem>Condominium</asp:ListItem>
<asp:ListItem>Townhouse</asp:ListItem>
<asp:ListItem>Single Family</asp:ListItem>
<asp:ListItem>Unknown</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
<tr>
<td colspan="2">
<asp:Label id="lblSelection" runat="server"></asp:Label></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of running the program:
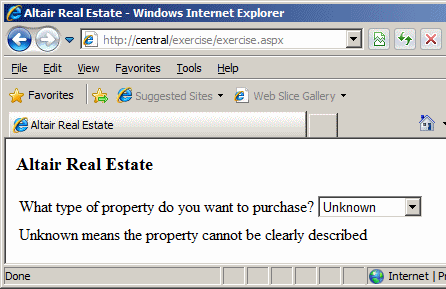
If the Statement is too long, you can write it on a
different line than the if condition. Here is an example:
<script runat="server">
private void IndexHouseSelected(object sender, EventArgs e)
{
if( TypesOfHouses.SelectedIndex == 3 )
lblSelection.Text = "This property is not easily defined";
}
</script>
You can also write the Statement on its own line even
if the statement is short enough to fit on the same line with the Condition.
Although the (simple) if statement is used to check
one condition, it can lead to executing multiple dependent statements. If that
is the case, enclose the group of statements between an opening curly bracket
“{“ and a closing curly bracket “}”. Here is an example:
<script runat="server">
private void IndexHouseSelected(object sender, EventArgs e)
{
string description = "";
if( TypesOfHouses.SelectedIndex == 3 )
{
description = "A property is marked as \"Unknown\" ";
description = description + "if is neither a condo, ";
description = description + "a townhouse (or town home), ";
description = description + "nor a single family.";
}
lblSelection.Text = description;
}
</script>
This would produce:
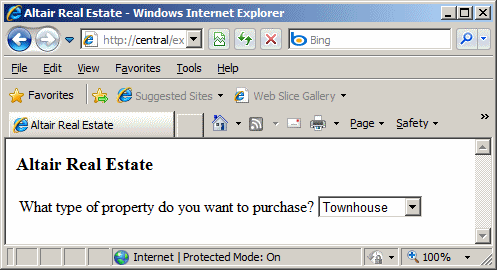
Here is another example of using it:
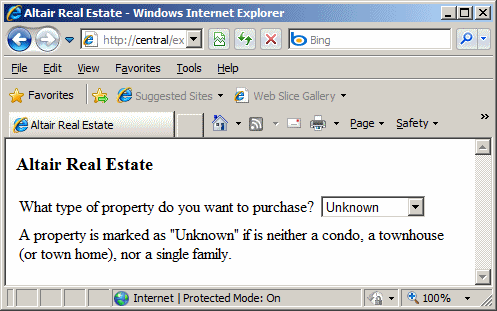
If you omit the brackets, only the statement that
immediately follows the condition would be executed.
Just as you can write one if condition, you can write
more than one. Here are examples:
<%@ Page Language="C#" %>
<html>
<head>
<script runat="server">
private void HouseSelected(object sender, EventArgs e)
{
string description = "";
if( TypesOfHouses.SelectedIndex == 0 )
description = "A condominium is a sectional home " +
"built as part of building. The building " +
"would be made of one or more levels and " +
"the condominiums are spread in different " +
"parts. The building could be a simple " +
"classic one or luxurious.";
if( TypesOfHouses.SelectedIndex == 1 )
description = "A townhouse, also called a town house, " +
"or a townhome, or a town home is a house that " +
"is attached to at least one other.";
if( TypesOfHouses.SelectedIndex == 2 )
description = "A single family is a house that stands " +
"by itself. The house touches the floor and has " +
"a roof. The house is not attached to any other " +
"house. A single family can have one or more stories.";
txtDescription.Text = description;
}
</script>
<title>Altair Real Estate</title>
</head>
<body>
<h3>Altair Real Estate</h3>
<form id="frmRealEstate" method="post" runat="server">
<table>
<tr>
<td>What type of property do you want to purchase?</td>
<td>
<asp:DropDownList id="TypesOfHouses"
AutoPostBack="True"
OnSelectedIndexChanged="HouseSelected" runat="server">
<asp:ListItem>Condominium</asp:ListItem>
<asp:ListItem>Townhouse</asp:ListItem>
<asp:ListItem>Single Family</asp:ListItem>
<asp:ListItem>Unknown</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
</table>
<table>
<tr>
<td valign="top">Description:</td>
<td>
<asp:TextBox ID="txtDescription"
TextMode="Multiline"
Columns="40"
Rows="3"
runat="server"></asp:TextBox></td>
</tr>
</form>
</body>
</html>
Here are examples of using the web page:
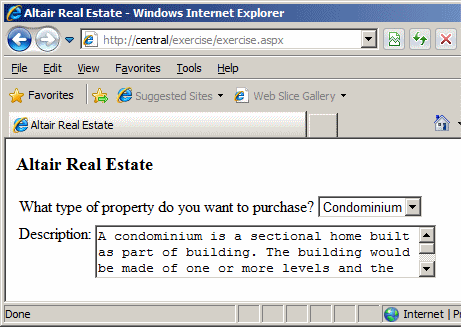
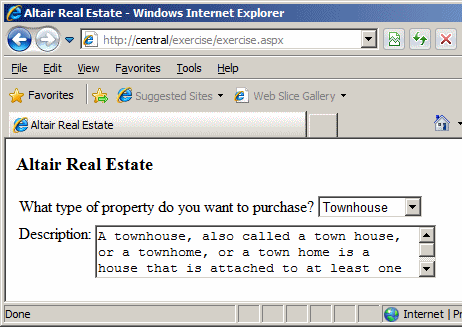