- Start a new project with its default form
- Save it in a new folder named BodyMonitor1
- Save the unit as Exercise and save the project as BodyMonitor
- Open Image Editor. Design a 32 x 32 icon and its associated 16 x 16 icon as follows:

- Save it as BMon
- Open the Project Options dialog. In the Application property page, set the title to Body Monitor Simulation
- Change the icon to the above
- Change the form’s properties as follows:
Name: frmMain
Caption: Body Monitoring
ShowHint: true
Position: poScreenCenter
- Save All
- On the Win32 tab of the Component Palette, click the ProgressBar button
and click on the form
- Change its properties as follows:
Height: 225
Left: 16
Max: 250
Name: pgrBlood
Orientation: pbVertical
Position: 128
Smooth: true
Top: 24
Width: 18
- In the same way, add other progress bars and design the form as follows:
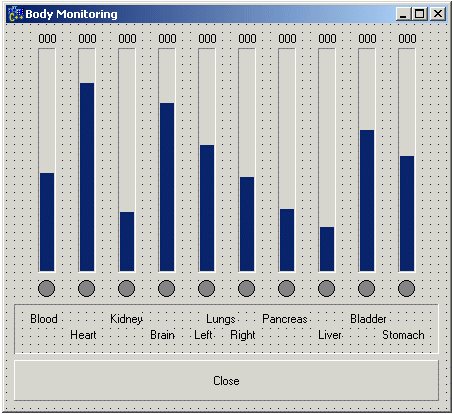 |
Control |
Name |
Caption |
Additional Properties |
Label |
lblHeart |
000 |
|
Label |
lblKidney |
000 |
|
Label |
lblBrain |
000 |
|
Label |
lblLLung |
000 |
|
Label |
lblRLung |
000 |
|
Label |
lblPancreas |
000 |
|
Label |
lblLiver |
000 |
|
Label |
lblBladder |
000 |
|
Label |
lblStomach |
000 |
|
Label |
lblBlood |
000 |
|
ProgressBar |
pgrBlood |
|
Max: 650
Position: 288 |
ProgressBar |
pgrHeart |
|
Max: 240
Position: 204 |
ProgressBar |
pgrKidney |
|
Max: 450
Position: 120 |
ProgressBar |
pgrBrain |
|
Max: 1000
Position: 760 |
ProgressBar |
pgrLLung |
|
Max: 750
Position: 428 |
ProgressBar |
pgrRLung |
|
Max: 750
Position: 320 |
ProgressBar |
pgrPancreas |
|
Max: 800
Position: 224 |
ProgressBar |
pgrLiver |
|
Max: 1200
Position: 240 |
ProgressBar |
pgrBladder |
|
Max: 550
Position: 350 |
ProgressBar |
pgrStomach |
|
Max: 1250
Position: 650 |
Shape |
shpBlood |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Blood Monitoring |
Shape |
shpHeart |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Heart Monitoring |
Shape |
shpKidney |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Kidney Monitoring |
Shape |
shpBrain |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Brain Monitoring |
Shape |
shpLLung |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Left Lung |
Shape |
shpRLung |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Right Lung |
Shape |
shpPancreas |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Pancreas Monitoring |
Shape |
shpLiver |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Liver Monitoring |
Shape |
shpBladder |
|
Brush: Color: clGray
Shape: stCircle |
Shape |
shpStomach |
|
Brush: Color: clGray
Shape: stCircle
Hint: Start Stomach Monitoring |
Bevel |
|
|
|
Label |
|
Blood |
|
Label |
|
Heart |
|
Label |
|
Kidney |
|
Label |
|
Brain |
|
Label |
|
Lungs |
|
Label |
|
Left |
|
Label |
|
Right |
|
Label |
|
Pancreas |
|
Label |
|
Liver |
|
Label |
|
Bladder |
|
Label |
|
Stomach |
|
Panel |
pnlClose |
Close |
|
|
- Add 10 timers
to the form and configure them as follows:
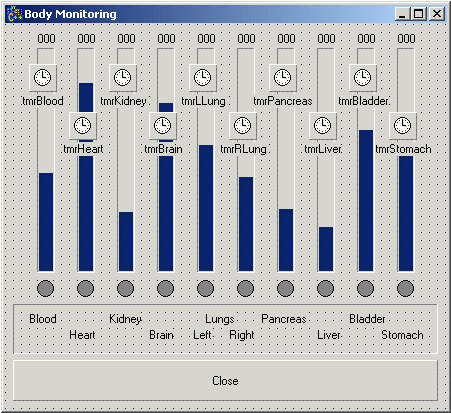 |
Control |
Name |
Enabled |
Interval |
Timer |
tmrBlood |
False |
650 |
Timer |
tmrHeart |
False |
240 |
Timer |
tmrKidney |
False |
450 |
Timer |
tmrBrain |
False |
1000 |
Timer |
tmrLLung |
False |
750 |
Timer |
tmrRLung |
False |
750 |
Timer |
tmrPancreas |
False |
800 |
Timer |
tmrLiver |
False |
1200 |
Timer |
tmrBladder |
False |
550 |
Timer |
tmrStomach |
False |
1250 |
|
- Double-click an unoccupied area on the form and implement its OnCreate() event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::FormCreate(TObject *Sender)
{
SendMessage(pgrBlood->Handle, PBM_SETBARCOLOR, 0, clRed);
SendMessage(pgrHeart->Handle, PBM_SETBARCOLOR, 0, clGreen);
SendMessage(pgrKidney->Handle, PBM_SETBARCOLOR, 0, clYellow);
SendMessage(pgrBrain->Handle, PBM_SETBARCOLOR, 0, clGray);
SendMessage(pgrLLung->Handle, PBM_SETBARCOLOR, 0, clFuchsia);
SendMessage(pgrRLung->Handle, PBM_SETBARCOLOR, 0, clFuchsia);
SendMessage(pgrPancreas->Handle, PBM_SETBARCOLOR, 0, clBlue);
SendMessage(pgrLiver->Handle, PBM_SETBARCOLOR, 0, clAqua);
SendMessage(pgrBladder->Handle, PBM_SETBARCOLOR, 0, clLime);
SendMessage(pgrStomach->Handle, PBM_SETBARCOLOR, 0, clNavy);
}
//---------------------------------------------------------------------------
|
- Save all
- On the form, double-click the tmrBlood timer to access its OnTimer event and implement it as follows:
//---------------------------------------------------------------------------
__fastcall TfrmMain::TfrmMain(TComponent* Owner)
: TForm(Owner)
{
Randomize();
}
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrBloodTimer(TObject *Sender)
{
int BloodLevel = random(650);
pgrBlood->Position = BloodLevel;
if( BloodLevel > 480 )
shpBlood->Brush->Color = clRed;
else
shpBlood->Brush->Color = clGreen;
lblBlood->Caption = lblBlood->Caption.sprintf("%d.%d",
BloodLevel/100, random(50));
}
//---------------------------------------------------------------------------
|
- Again, on the form, double-click the tmrHeart timer to access its OnTimer event event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrHeartTimer(TObject *Sender)
{
int HeartLevel = random(240);
pgrHeart->Position = HeartLevel;
if( HeartLevel > 180 )
shpHeart->Brush->Color = clRed;
else
shpHeart->Brush->Color = clGreen;
lblHeart->Caption = lblHeart->Caption.sprintf("%d\260", HeartLevel);
}
//---------------------------------------------------------------------------
|
- In the same way, initiate the OnTimer event of the tmrKidney timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrKidneyTimer(TObject *Sender)
{
int KidneyLevel = random(450);
pgrKidney->Position = KidneyLevel;
if( KidneyLevel > 400 )
shpKidney->Brush->Color = clRed;
else
shpKidney->Brush->Color = clGreen;
lblKidney->Caption = lblKidney->Caption.sprintf("%d\045", KidneyLevel);
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrBrain timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrBrainTimer(TObject *Sender)
{
int BrainLevel = random(1000);
pgrBrain->Position = BrainLevel;
if( BrainLevel > 550 )
shpBrain->Brush->Color = clRed;
else
shpBrain->Brush->Color = clGreen;
lblBrain->Caption = lblBrain->Caption.sprintf("<%d>", BrainLevel-450);
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrLLung timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrLLungTimer(TObject *Sender)
{
int LLungLevel = random(750);
pgrLLung->Position = LLungLevel;
if( LLungLevel > 600 )
shpLLung->Brush->Color = clRed;
else
shpLLung->Brush->Color = clGreen;
lblLLung->Caption = lblLLung->Caption.sprintf("%d.%d\"",
LLungLevel, 2 + random(5));
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrRLung timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrRLungTimer(TObject *Sender)
{
int RLungLevel = random(750);
pgrRLung->Position = RLungLevel;
if( RLungLevel > 500 )
shpRLung->Brush->Color = clRed;
else
shpRLung->Brush->Color = clGreen;
lblRLung->Caption = lblRLung->Caption.sprintf("%d.%d\"",
RLungLevel, 2 + random(5));
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrPancreas timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrPancreasTimer(TObject *Sender)
{
int PancreasLevel = random(800);
pgrPancreas->Position = PancreasLevel;
if( PancreasLevel > 600 )
shpPancreas->Brush->Color = clRed;
else
shpPancreas->Brush->Color = clGreen;
lblPancreas->Caption = lblPancreas->Caption.sprintf("\273%d",
PancreasLevel);
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrLiver timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrLiverTimer(TObject *Sender)
{
int LiverLevel = random(1200);
pgrLiver->Position = LiverLevel;
if( LiverLevel > 1100 )
shpLiver->Brush->Color = clRed;
else
shpLiver->Brush->Color = clGreen;
lblLiver->Caption = lblLiver->Caption.sprintf("%d\264", LiverLevel);
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrBladder timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrBladderTimer(TObject *Sender)
{
int BladderLevel = random(550);
pgrBladder->Position = BladderLevel;
if( BladderLevel > 450 )
shpBladder->Brush->Color = clRed;
else
shpBladder->Brush->Color = clGreen;
lblBladder->Caption = lblBladder->Caption.sprintf("\247%d\252",
BladderLevel);
}
//---------------------------------------------------------------------------
|
- Initiate the OnTimer event of the tmrStomach timer event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::tmrStomachTimer(TObject *Sender)
{
int StomachLevel = random(1250);
pgrStomach->Position = StomachLevel;
if( StomachLevel > 1100 )
shpStomach->Brush->Color = clRed;
else
shpStomach->Brush->Color = clGreen;
lblStomach->Caption = lblStomach->Caption.sprintf("%d\274",
StomachLevel);
}
//---------------------------------------------------------------------------
|
- On the form, click the shape control above the Blood label. In the Object Inspector, click the Events tab. Double-click the right field to OnMouseDown and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpBloodMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrBlood->Enabled )
{
tmrBlood->Enabled = False;
shpBlood->Brush->Color = clGray;
shpBlood->Hint = "Start Blood Monitoring";
}
else
{
tmrBlood->Enabled = True;
shpBlood->Hint = "Stop Blood Monitoring";
}
}
//---------------------------------------------------------------------------
|
- In the same way, initiate the OnMouseDown event of the shape above the Heart label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpHeartMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrHeart->Enabled )
{
tmrHeart->Enabled = False;
shpHeart->Brush->Color = clGray;
shpHeart->Hint = "Start Heart Monitoring";
}
else
{
tmrHeart->Enabled = True;
shpHeart->Hint = "Stop Heart Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Also, initiate the OnMouseDown event of the shape above the Kidney label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpKidneyMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrKidney->Enabled )
{
tmrKidney->Enabled = False;
shpKidney->Brush->Color = clGray;
shpKidney->Hint = "Start Kidney Monitoring";
}
else
{
tmrKidney->Enabled = True;
shpKidney->Hint = "Stop Kidney Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Brain label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpBrainMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrBrain->Enabled )
{
tmrBrain->Enabled = False;
shpBrain->Brush->Color = clGray;
shpBrain->Hint = "Start Brain Monitoring";
}
else
{
tmrBrain->Enabled = True;
shpBrain->Hint = "Stop Brain Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Left label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpLLungMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrLLung->Enabled )
{
tmrLLung->Enabled = False;
shpLLung->Brush->Color = clGray;
shpLLung->Hint = "Start Left Lung Monitoring";
}
else
{
tmrLLung->Enabled = True;
shpLLung->Hint = "Stop Left Lung Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Right label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpRLungMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrRLung->Enabled )
{
tmrRLung->Enabled = False;
shpRLung->Brush->Color = clGray;
shpRLung->Hint = "Start Right Lung Monitoring";
}
else
{
tmrRLung->Enabled = True;
shpRLung->Hint = "Stop Right Lung Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Pancreas label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpPancreasMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrPancreas->Enabled )
{
tmrPancreas->Enabled = False;
shpPancreas->Brush->Color = clGray;
shpPancreas->Hint = "Start Pancreas Monitoring";
}
else
{
tmrPancreas->Enabled = True;
shpPancreas->Hint = "Stop Pancreas Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Liver label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpLiverMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrLiver->Enabled )
{
tmrLiver->Enabled = False;
shpLiver->Brush->Color = clGray;
shpLiver->Hint = "Start Liver Monitoring";
}
else
{
tmrLiver->Enabled = True;
shpLiver->Hint = "Stop Liver Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Bladder label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpBladderMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrBladder->Enabled )
{
tmrBladder->Enabled = False;
shpBladder->Brush->Color = clGray;
shpBladder->Hint = "Start Bladder Monitoring";
}
else
{
tmrBladder->Enabled = True;
shpBladder->Hint = "Stop Bladder Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Initiate the OnMouseDown event of the shape above the Stomach label and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::shpStomachMouseDown(TObject *Sender,
TMouseButton Button, TShiftState Shift, int X, int Y)
{
if( tmrStomach->Enabled )
{
tmrStomach->Enabled = False;
shpStomach->Brush->Color = clGray;
shpStomach->Hint = "Start Stomach Monitoring";
}
else
{
tmrStomach->Enabled = True;
shpStomach->Hint = "Stop Stomach Monitoring";
}
}
//---------------------------------------------------------------------------
|
- Double-click the (bottom) panel to access its OnClick event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmMain::Panel1Click(TObject *Sender)
{
Close();
}
//---------------------------------------------------------------------------
|
- Execute the application
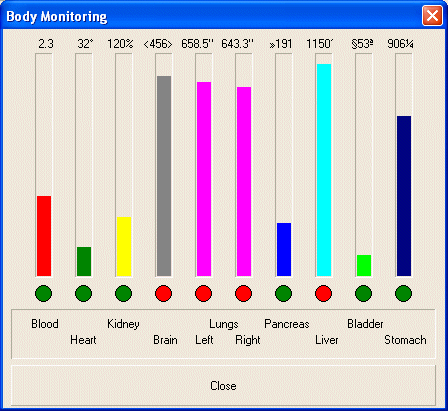
- To test a progress bar, click the shape button under it. To stop it, click its shape button again
- After using the form, close it and return to Bcb
- Save All
|
|