- Start a new project with the default form
- To save it, on the Standard toolbar, click the Save All button
- Click the Create New Folder button. Type Operations and press Enter twice
- Click Unit1 to select it. Type Main and press Enter
- Type Operations as the name of the project and press Enter
- On the Standard tab of the Component Palette, click the Panel control

- On the form, click and drag from the top left section to the middle center
section
- On the Object Inspector, click Caption and press Delete to delete the caption of the
panel
- On the Component Palette, double-click the RadioButton control

- On the Object Inspector, click Caption and type &Addition
- Click Name and type rdoAddition
- Move the new radio button to the top section of and inside the panel
- On the Component Palette, click the Radio Button
- Click inside the panel
- Click Name and type rdoSubtraction
- Click Caption and type &Subtraction
- In the same way, add another RadioButton control to the panel. Set its Caption to &Multiplication and its Name to rdoMultiplication
- Add one more RadioButton
to the panel
- Set its Name to rdoDivision and its Caption to &Division
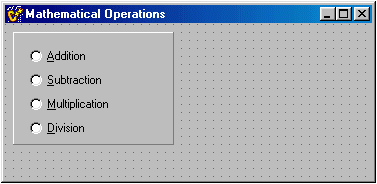
- Move the panel to the right section of the form
- Notice that it moves with its “children”. You will redesign the form as follows:
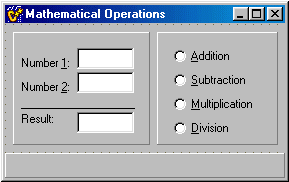
- Add another panel
with four labels
captioned
Number &1:
Number &2:
------------------
Result:
- Add three Edit controls
inside the panel and on the right side of the corresponding labels. These edit boxes will be named
edtNumber1, edtNumber2, and edtResult
- Add another panel to an empty area of the form
- While the new and last panel is selected, click the Align field. Click its arrow and select
alBottom
- On the form double-click the Addition radio button to access its OnClick event
- Implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmOperations::rdoAdditionClick(TObject *Sender)
{
double Num1;
double Num2;
double Result;
if( edtNumber1->Text == "" )
Num1 = 0;
else
Num1 = StrToFloat(edtNumber1->Text);
if( edtNumber2->Text == "" )
Num2 = 0;
else
Num2 = StrToFloat(edtNumber2->Text);
Result = Num1 + Num2;
edtResult->Text = Result;
}
//---------------------------------------------------------------------------
|
- Click the arrow on the top section of the Object Inspector and select rdoSubtraction
- Click the Events tab
- Double-click the empty box on the right side of OnClick
- Implement the function just like the Click event of the addition but change the operation as follows:
Result = Num1 - Num2;
- On the top combo box of the Object Inspector, select rdoMultiplication
- Double-click the box of the OnClick field
- Implement the Click event like the previous two but change the operation as follows:
Result = Num1 * Num2;
- In the same way, access the OnClick event for the rdoDivision control and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmOperations::rdoDivisionClick(TObject *Sender)
{
double Num1;
double Num2;
double Result;
if( edtNumber1->Text == "" )
Num1 = 0;
else
Num1 = StrToFloat(edtNumber1->Text);
if( edtNumber2->Text == "" )
{
MessageBox(NULL, "The Number 2 Edit Box should not be empty",
"Algebraic Operations", MB_OK);
edtNumber2->SetFocus();
}
else if( edtNumber2->Text == 0 )
{
MessageBox(0, "Cannot divide by zero",
"Algebraic Operations", MB_OK);
edtNumber2->SetFocus();
}
else
{
Num2 = StrToFloat(edtNumber2->Text);
Result = Num1 / Num2;
edtResult->Text = Result;
}
}
//---------------------------------------------------------------------------
|
- To display the form, press F12
- Double-click the panel on the bottom section of the form to access its OnClick event and implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmOperations::Panel3Click(Tobject *Sender)
{
Close();
}
//---------------------------------------------------------------------------
|
- To test the form, press F9
- After using it, close the form and save your project
|
|