-
Double-click the OK button to access its
OnClick() event.
Implement the event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::BitBtn1Click(TObject *Sender)
{
Close();
}
//---------------------------------------------------------------------------
|
-
To bring back the form, press F12.
-
Press Esc a few times to make sure that no control
but the form itself is selected.
-
On the Object Inspector, click the Events tab.
-
Double-click the empty section on the right side
of OnCreate to access the form's to access
the form's OnCreate() event.
-
To make the table available when the form comes
up, implement the event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::FormCreate(TObject *Sender)
{
Table1->Open();
}
//---------------------------------------------------------------------------
|
- We will use a control-independent function that
can reset the values of the combo boxes.
When a user needs to fill out the time sheet, we will set all times to
0, which would allow the user to proceed step-by-step in selecting the
appropriate start and end sections of each shift.
If the Class Explorer is not visible, on the main menu, click View
ClassExplorer.
On the Class Explorer, expand everything by clicking the + buttons if
necessary.
Right-click frmTimeSheet and click New Method...
- Set the Method Name to ResetShifts
- Set the Function Result to void
- Click the __fastcall check box and click OK.
- Implement the method as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::ResetShifts()
{
cbxMondayIn1->ItemIndex = 24;
cbxMondayOut1->ItemIndex = 24;
cbxMondayIn2->ItemIndex = 24;
cbxMondayOut2->ItemIndex = 24;
cbxTuesdayIn1->ItemIndex = 24;
cbxTuesdayOut1->ItemIndex = 24;
cbxTuesdayIn2->ItemIndex = 24;
cbxTuesdayOut2->ItemIndex = 24;
cbxWednesdayIn1->ItemIndex = 24;
cbxWednesdayOut1->ItemIndex = 24;
cbxWednesdayIn2->ItemIndex = 24;
cbxWednesdayOut2->ItemIndex = 24;
cbxThursdayIn1->ItemIndex = 24;
cbxThursdayOut1->ItemIndex = 24;
cbxThursdayIn2->ItemIndex = 24;
cbxThursdayOut2->ItemIndex = 24;
cbxFridayIn1->ItemIndex = 24;
cbxFridayOut1->ItemIndex = 24;
cbxFridayIn2->ItemIndex = 24;
cbxFridayOut2->ItemIndex = 24;
cbxSaturdayIn1->ItemIndex = 24;
cbxSaturdayOut1->ItemIndex = 24;
cbxSaturdayIn2->ItemIndex = 24;
cbxSaturdayOut2->ItemIndex = 24;
cbxSundayIn1->ItemIndex = 24;
cbxSundayOut1->ItemIndex = 24;
cbxSundayIn2->ItemIndex = 24;
cbxSundayOut2->ItemIndex = 24;
}
//---------------------------------------------------------------------------
|
- To make the combo boxes display the reset time
values, call the ResetShifts() method from the OnCreate event pf the
form as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::FormCreate(TObject *Sender)
{
Table1->Open();
ResetShifts();
}
//---------------------------------------------------------------------------
|
- Also call the ResetShifts() method from the
btnResetShifts OnClick event. On the form, double-click the Reset
button and implement its OnClick event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::btnResetClick(TObject *Sender)
{
ResetShifts();
}
//---------------------------------------------------------------------------
|
- To test the form, press F9 and make sure that each
combo box displays 12:00 PM
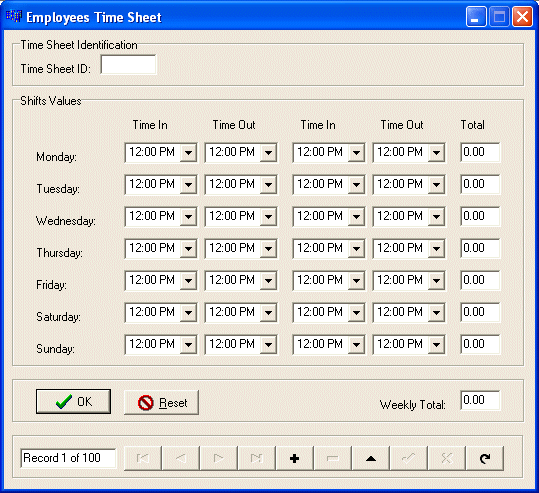
- The first thing we are going to do is to validate
the time values that the user selects on a combo box, depending on the
combo box. Consider a day like Monday. The starting time that the user
selects should not be higher than the end time. This means that a user
cannot state that he worked from 12PM to 9AM in one day. Logically,
such a sequence doesn't make sense. Therefore, the first time in for
Monday must not occur after the first time out for Monday.
To implement this algorithm, on the form, double-click the combo box
at the intersection of Monday and the left Time In label to access its
OnChange event.
- Implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayIn1Change(TObject *Sender)
{
// InSelection is the time that has just been selected
int In1Selection = cbxMondayIn1->ItemIndex;
// OutSelection is the time that is displaying the next combo box
int Out1Selection = cbxMondayOut1->ItemIndex;
// Whenever the user decides to change the time on this one,
// make sure the new time doesn't occur after the next time value
// If the user tries this, set the Monday 's first time in to value
// of the next combo box
if( Out1Selection < In1Selection )
cbxMondayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
|
-
When it comes to the first time out value, we need
to fulfill two conditions: a)the value of the first time out must not
be lower than that of the first time in; b) the value of the first
time out must not be higher than that of the second time in.
To implement this behavior, on the form, double-click the combo box at
the intersection of Monday and the left Time Out label.
- Implement its OnChange() event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayOut1Change(TObject *Sender)
{
// Get the selected value of the first Time In
int In1Selection = cbxMondayIn1->ItemIndex;
// Get the selected value of the first Time Out
int Out1Selection = cbxMondayOut1->ItemIndex;
// Get the selected value of the second Time In
int In2Selection = cbxMondayIn2->ItemIndex;
// Is the new value selected less than that of
// the first Time In
if( Out1Selection < In1Selection )
cbxMondayOut1->ItemIndex = 24; // If so, set it to 12:00 PM
// If the selected value happens to occur after the second Time In
if( Out1Selection > In2Selection )
cbxMondayOut1->ItemIndex = In2Selection; // Set it to that of Time In2
}
//---------------------------------------------------------------------------
|
-
These two algorithms will be applied to all the
other combo boxes. Therefore, implement their OnChange events
accordingly. When you have finished, their should look as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayIn1Change(TObject *Sender)
{
// InSelection is the time that has just been selected
int In1Selection = cbxMondayIn1->ItemIndex;
// OutSelection is the time that is displaying the next combo box
int Out1Selection = cbxMondayOut1->ItemIndex;
// Whenever the user decides to change the time on this one,
// make sure the new time doesn't occur after the next time value
// If the user tries this, set the Monday 's first time in to value
// of the next combo box
if( Out1Selection < In1Selection )
cbxMondayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayOut1Change(TObject *Sender)
{
// Get the selected value of the first Time In
int In1Selection = cbxMondayIn1->ItemIndex;
// Get the selected value of the first Time Out
int Out1Selection = cbxMondayOut1->ItemIndex;
// Get the selected value of the second Time In
int In2Selection = cbxMondayIn2->ItemIndex;
// Is the new value selected less than that of
// the first Time In
if( Out1Selection < In1Selection )
cbxMondayOut1->ItemIndex = 24; // If so, set it to 12:00 PM
// If the selected value happens to occur after the second Time In
if( Out1Selection > In2Selection )
cbxMondayOut1->ItemIndex = In2Selection; // Set it to that of Time In2
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayIn2Change(TObject *Sender)
{
int In2Selection = cbxMondayIn2->ItemIndex;
int Out1Selection = cbxMondayOut1->ItemIndex;
int Out2Selection = cbxMondayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxMondayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxMondayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayOut2Change(TObject *Sender)
{
int Out2Selection = cbxMondayOut2->ItemIndex;
int In2Selection = cbxMondayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxMondayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayIn1Change(TObject *Sender)
{
int In1Selection = cbxTuesdayIn1->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxTuesdayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayOut1Change(TObject *Sender)
{
int In1Selection = cbxTuesdayIn1->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
int In2Selection = cbxTuesdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxTuesdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxTuesdayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayIn2Change(TObject *Sender)
{
int In2Selection = cbxTuesdayIn2->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
int Out2Selection = cbxTuesdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxTuesdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxTuesdayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxTuesdayOut2->ItemIndex;
int In2Selection = cbxTuesdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxTuesdayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayIn1Change(TObject *Sender)
{
int In1Selection = cbxWednesdayIn1->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxWednesdayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayOut1Change(TObject *Sender)
{
int In1Selection = cbxWednesdayIn1->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
int In2Selection = cbxWednesdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxWednesdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxWednesdayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayIn2Change(TObject *Sender)
{
int In2Selection = cbxWednesdayIn2->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
int Out2Selection = cbxWednesdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxWednesdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxWednesdayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxWednesdayOut2->ItemIndex;
int In2Selection = cbxWednesdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxWednesdayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayIn1Change(TObject *Sender)
{
int In1Selection = cbxThursdayIn1->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxThursdayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayOut1Change(TObject *Sender)
{
int In1Selection = cbxThursdayIn1->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
int In2Selection = cbxThursdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxThursdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxThursdayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayIn2Change(TObject *Sender)
{
int In2Selection = cbxThursdayIn2->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
int Out2Selection = cbxThursdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxThursdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxThursdayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxThursdayOut2->ItemIndex;
int In2Selection = cbxThursdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxThursdayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayIn1Change(TObject *Sender)
{
int In1Selection = cbxFridayIn1->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxFridayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayOut1Change(TObject *Sender)
{
int In1Selection = cbxFridayIn1->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
int In2Selection = cbxFridayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxFridayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxFridayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayIn2Change(TObject *Sender)
{
int In2Selection = cbxFridayIn2->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
int Out2Selection = cbxFridayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxFridayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxFridayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayOut2Change(TObject *Sender)
{
int Out2Selection = cbxFridayOut2->ItemIndex;
int In2Selection = cbxFridayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxFridayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayIn1Change(TObject *Sender)
{
int In1Selection = cbxSaturdayIn1->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxSaturdayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayOut1Change(TObject *Sender)
{
int In1Selection = cbxSaturdayIn1->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
int In2Selection = cbxSaturdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxSaturdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxSaturdayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayIn2Change(TObject *Sender)
{
int In2Selection = cbxSaturdayIn2->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
int Out2Selection = cbxSaturdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxSaturdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxSaturdayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxSaturdayOut2->ItemIndex;
int In2Selection = cbxSaturdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxSaturdayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayIn1Change(TObject *Sender)
{
int In1Selection = cbxSundayIn1->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxSundayIn1->ItemIndex = Out1Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayOut1Change(TObject *Sender)
{
int In1Selection = cbxSundayIn1->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
int In2Selection = cbxSundayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxSundayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxSundayOut1->ItemIndex = In2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayIn2Change(TObject *Sender)
{
int In2Selection = cbxSundayIn2->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
int Out2Selection = cbxSundayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxSundayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxSundayIn2->ItemIndex = Out2Selection;
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayOut2Change(TObject *Sender)
{
int Out2Selection = cbxSundayOut2->ItemIndex;
int In2Selection = cbxSundayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxSundayOut2->ItemIndex = 34;
}
//---------------------------------------------------------------------------
|
- Now that we can control the sequence of the time values that the
user can select, we can calculate the actual time frames.
The time values of our time sheet are set as strings. We need a
mechanism that can convert such a string to a double-precision number
in order to involve such a time value into an arithmetic calculation.
To do this, we will get the time value that a user has selected. We
will pass that string to a method that would convert it to a double
and return that number.
On the Class Explorer, right-click the frmTimeSheet folder and click
Add Method.
- Set the name Method Name to ConvertTimeToDouble
- In the Arguments edit box, type AnsiString Value
- Set the Function Result to double and click the __fastcall check
box.
- Click OK and implement its method as follows:
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::ConvertTimeToDouble(AnsiString Value)
{
int HPortion; // Hour portion of the string as an integer
double MinPortion; // Minute portion of the string as a decimal value
// Remove the empty space on the left of AM/PM
Value = Value.Delete(6, 1);
// Get the hour portion of the string
AnsiString ValueHour = Value.SubString(1, 2);
// Get the minute portion of the string
AnsiString ValueMin = Value.SubString(4, 2);
// Get the AM/PM portion of the string
AnsiString ValueAMPM = Value.SubString(6, 2);
// Does the time occur in the afternoon?
if( ValueAMPM == "PM" )
{
// Is it at noon = 12 PM?
if( ValueHour == "12" )
HPortion = ValueHour.ToInt(); // Convert it to a 12-hour integer
else
HPortion = 12 + ValueHour.ToInt(); // Convert it to a 24-hour integer
}
// Since the time occured in the morning
else
HPortion = ValueHour.ToInt(); // Convert it to a 12-hour integer
// Does the time occur at the exact hour?
if( ValueMin == "00" )
MinPortion = 0.00; // Set the minute to 0
else // Otherwise
MinPortion = 0.50; // set it at half the hour
// Now we have a complete floating number that represents the hour
return HPortion + MinPortion;
}
//---------------------------------------------------------------------------
|
- Whenever the user selects a new or different value on one of the
combo box, it is possible that the time frame has changed. We need to
get all values of the corresponding day, calculate the equivalent time
frame and display its decimal value in the right edit box. To take
care of this situation, we will use a particular method for each day.
In the Class Explorer, right-click frmTimeSheet and click New Method.
- Set the Method Name to UpdateMondayTime
- Set the Function Result to double and click the __fastcall check
box.
- Click OK and implement the method as follows:
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateMondayTime()
{
double Monday, MondayIn1, MondayOut1, MondayIn2, MondayOut2;
// Get the decimal equivalent of the first Time In
MondayIn1 = ConvertTimeToDouble(cbxMondayIn1->Text);
// Get the decimal equivalent of the first Time Out
MondayOut1 = ConvertTimeToDouble(cbxMondayOut1->Text);
// Get the decimal equivalent of the second Time In
MondayIn2 = ConvertTimeToDouble(cbxMondayIn2->Text);
// Get the decimal equivalent of the second Time Out
MondayOut2 = ConvertTimeToDouble(cbxMondayOut2->Text);
// Calculate the time worked for the first shift
double FirstShift = MondayOut1 - MondayIn1;
// Calculate the time worked for the second shift
double SecondShift = MondayOut2 - MondayIn2;
// Calculate the total time for the day
Monday = FirstShift + SecondShift;
// Display the result in the Monday edit box
edtMonday->Text = FloatToStr(Monday, ffFixed, 4, 2);
return Monday;
}
//---------------------------------------------------------------------------
|
- In the same way, create an update method for each day and implement
them as follows:
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateTuesdayTime()
{
double Tuesday, TuesdayIn1, TuesdayOut1, TuesdayIn2, TuesdayOut2;
TuesdayIn1 = ConvertTimeToDouble(cbxTuesdayIn1->Text);
TuesdayOut1 = ConvertTimeToDouble(cbxTuesdayOut1->Text);
TuesdayIn2 = ConvertTimeToDouble(cbxTuesdayIn2->Text);
TuesdayOut2 = ConvertTimeToDouble(cbxTuesdayOut2->Text);
double FirstShift = TuesdayOut1 - TuesdayIn1;
double SecondShift = TuesdayOut2 - TuesdayIn2;
Tuesday = FirstShift + SecondShift;
edtTuesday->Text = FloatToStrF(Tuesday, ffFixed, 4, 2);
return Tuesday;
}
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateWednesdayTime()
{
double Wednesday, WednesdayIn1, WednesdayOut1, WednesdayIn2, WednesdayOut2;
WednesdayIn1 = ConvertTimeToDouble(cbxWednesdayIn1->Text);
WednesdayOut1 = ConvertTimeToDouble(cbxWednesdayOut1->Text);
WednesdayIn2 = ConvertTimeToDouble(cbxWednesdayIn2->Text);
WednesdayOut2 = ConvertTimeToDouble(cbxWednesdayOut2->Text);
double FirstShift = WednesdayOut1 - WednesdayIn1;
double SecondShift = WednesdayOut2 - WednesdayIn2;
Wednesday = FirstShift + SecondShift;
edtWednesday->Text = FloatToStrF(Wednesday, ffFixed, 4, 2);
return Wednesday;
}
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateThursdayTime()
{
double Thursday, ThursdayIn1, ThursdayOut1, ThursdayIn2, ThursdayOut2;
ThursdayIn1 = ConvertTimeToDouble(cbxThursdayIn1->Text);
ThursdayOut1 = ConvertTimeToDouble(cbxThursdayOut1->Text);
ThursdayIn2 = ConvertTimeToDouble(cbxThursdayIn2->Text);
ThursdayOut2 = ConvertTimeToDouble(cbxThursdayOut2->Text);
double FirstShift = ThursdayOut1 - ThursdayIn1;
double SecondShift = ThursdayOut2 - ThursdayIn2;
Thursday = FirstShift + SecondShift;
edtThursday->Text = FloatToStrF(Thursday, ffFixed, 4, 2);
return Thursday;
}
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateFridayTime()
{
double Friday, FridayIn1, FridayOut1, FridayIn2, FridayOut2;
FridayIn1 = ConvertTimeToDouble(cbxFridayIn1->Text);
FridayOut1 = ConvertTimeToDouble(cbxFridayOut1->Text);
FridayIn2 = ConvertTimeToDouble(cbxFridayIn2->Text);
FridayOut2 = ConvertTimeToDouble(cbxFridayOut2->Text);
double FirstShift = FridayOut1 - FridayIn1;
double SecondShift = FridayOut2 - FridayIn2;
Friday = FirstShift + SecondShift;
edtFriday->Text = FloatToStrF(Friday, ffFixed, 4, 2);
return Friday;
}
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateSaturdayTime()
{
double Saturday, SaturdayIn1, SaturdayOut1, SaturdayIn2, SaturdayOut2;
SaturdayIn1 = ConvertTimeToDouble(cbxSaturdayIn1->Text);
SaturdayOut1 = ConvertTimeToDouble(cbxSaturdayOut1->Text);
SaturdayIn2 = ConvertTimeToDouble(cbxSaturdayIn2->Text);
SaturdayOut2 = ConvertTimeToDouble(cbxSaturdayOut2->Text);
double FirstShift = SaturdayOut1 - SaturdayIn1;
double SecondShift = SaturdayOut2 - SaturdayIn2;
Saturday = FirstShift + SecondShift;
edtSaturday->Text = FloatToStrF(Saturday, ffFixed, 4, 2);
return Saturday;
}
//---------------------------------------------------------------------------
double __fastcall TfrmTimeSheet::UpdateSundayTime()
{
double Sunday, SundayIn1, SundayOut1, SundayIn2, SundayOut2;
SundayIn1 = ConvertTimeToDouble(cbxSundayIn1->Text);
SundayOut1 = ConvertTimeToDouble(cbxSundayOut1->Text);
SundayIn2 = ConvertTimeToDouble(cbxSundayIn2->Text);
SundayOut2 = ConvertTimeToDouble(cbxSundayOut2->Text);
double FirstShift = SundayOut1 - SundayIn1;
double SecondShift = SundayOut2 - SundayIn2;
Sunday = FirstShift + SecondShift;
edtSunday->Text = FloatToStrF(Sunday, ffFixed, 4, 2);
return Sunday;
}
//---------------------------------------------------------------------------
|
- Now that we can find out how much time the employee worked for a
day, we can calculate the total weekly hours and display its value in
the Total Weekly Hour edit box. To perform this calculation, we will
retrieve the value that each Update...Time method can produce, add the
resulting values to obtain a total, format and display that total.
In the Class Explorer, right-click frmTimeSheet and click Add Method
- Set the Method Name to CalcTotalTime
- Set its Function Result to void and click the __fastcall check
button.
- Click OK and implemented the method as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::CalcTotalTime()
{
double Mon = UpdateMondayTime();
double Tue = UpdateTuesdayTime();
double Wed = UpdateWednesdayTime();
double Thu = UpdateThursdayTime();
double Fri = UpdateFridayTime();
double Sat = UpdateSaturdayTime();
double Sun = UpdateSundayTime();
double Total = Mon + Tue + Wed + Thu + Fri + Sat + Sun;
edtWeeklyTotal->Text = FloatToStrF(Total, ffFixed, 4, 2);
}
//---------------------------------------------------------------------------
|
- We could have called this method using a button. To make the
employees job easier, we want the time sheet to update itself.
Whenever the user has changed the value of one of the combo boxes, we
will call the CalcTotalTime() method to recalculate things, update the
total time for each day, and update the total time for the week.
At the end of the OnChange event of each combo box, call the
CalcTotalTime() method as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayIn1Change(TObject *Sender)
{
// InSelection is the time that has just been selected
int In1Selection = cbxMondayIn1->ItemIndex;
// OutSelection is the time that is displaying the next combo box
int Out1Selection = cbxMondayOut1->ItemIndex;
// Whenever the user decides to change the time on this one,
// make sure the new time doesn't occur after the next time value
// If the user tries this, set the Monday 's first time in to value
// of the next combo box
if( Out1Selection < In1Selection )
cbxMondayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayOut1Change(TObject *Sender)
{
// Get the selected value of the first Time In
int In1Selection = cbxMondayIn1->ItemIndex;
// Get the selected value of the first Time Out
int Out1Selection = cbxMondayOut1->ItemIndex;
// Get the selected value of the second Time In
int In2Selection = cbxMondayIn2->ItemIndex;
// Is the new value selected less than that of
// the first Time In
if( Out1Selection < In1Selection )
cbxMondayOut1->ItemIndex = 24; // If so, set it to 12:00 PM
// If the selected value happens to occur after the second Time In
if( Out1Selection > In2Selection )
cbxMondayOut1->ItemIndex = In2Selection; // Set it to that of Time In2
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayIn2Change(TObject *Sender)
{
int In2Selection = cbxMondayIn2->ItemIndex;
int Out1Selection = cbxMondayOut1->ItemIndex;
int Out2Selection = cbxMondayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxMondayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxMondayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxMondayOut2Change(TObject *Sender)
{
int Out2Selection = cbxMondayOut2->ItemIndex;
int In2Selection = cbxMondayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxMondayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayIn1Change(TObject *Sender)
{
int In1Selection = cbxTuesdayIn1->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxTuesdayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayOut1Change(TObject *Sender)
{
int In1Selection = cbxTuesdayIn1->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
int In2Selection = cbxTuesdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxTuesdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxTuesdayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayIn2Change(TObject *Sender)
{
int In2Selection = cbxTuesdayIn2->ItemIndex;
int Out1Selection = cbxTuesdayOut1->ItemIndex;
int Out2Selection = cbxTuesdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxTuesdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxTuesdayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxTuesdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxTuesdayOut2->ItemIndex;
int In2Selection = cbxTuesdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxTuesdayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayIn1Change(TObject *Sender)
{
int In1Selection = cbxWednesdayIn1->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxWednesdayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayOut1Change(TObject *Sender)
{
int In1Selection = cbxWednesdayIn1->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
int In2Selection = cbxWednesdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxWednesdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxWednesdayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayIn2Change(TObject *Sender)
{
int In2Selection = cbxWednesdayIn2->ItemIndex;
int Out1Selection = cbxWednesdayOut1->ItemIndex;
int Out2Selection = cbxWednesdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxWednesdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxWednesdayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxWednesdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxWednesdayOut2->ItemIndex;
int In2Selection = cbxWednesdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxWednesdayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayIn1Change(TObject *Sender)
{
int In1Selection = cbxThursdayIn1->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxThursdayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayOut1Change(TObject *Sender)
{
int In1Selection = cbxThursdayIn1->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
int In2Selection = cbxThursdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxThursdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxThursdayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayIn2Change(TObject *Sender)
{
int In2Selection = cbxThursdayIn2->ItemIndex;
int Out1Selection = cbxThursdayOut1->ItemIndex;
int Out2Selection = cbxThursdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxThursdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxThursdayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxThursdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxThursdayOut2->ItemIndex;
int In2Selection = cbxThursdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxThursdayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayIn1Change(TObject *Sender)
{
int In1Selection = cbxFridayIn1->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxFridayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayOut1Change(TObject *Sender)
{
int In1Selection = cbxFridayIn1->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
int In2Selection = cbxFridayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxFridayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxFridayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayIn2Change(TObject *Sender)
{
int In2Selection = cbxFridayIn2->ItemIndex;
int Out1Selection = cbxFridayOut1->ItemIndex;
int Out2Selection = cbxFridayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxFridayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxFridayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxFridayOut2Change(TObject *Sender)
{
int Out2Selection = cbxFridayOut2->ItemIndex;
int In2Selection = cbxFridayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxFridayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayIn1Change(TObject *Sender)
{
int In1Selection = cbxSaturdayIn1->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxSaturdayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayOut1Change(TObject *Sender)
{
int In1Selection = cbxSaturdayIn1->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
int In2Selection = cbxSaturdayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxSaturdayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxSaturdayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayIn2Change(TObject *Sender)
{
int In2Selection = cbxSaturdayIn2->ItemIndex;
int Out1Selection = cbxSaturdayOut1->ItemIndex;
int Out2Selection = cbxSaturdayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxSaturdayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxSaturdayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSaturdayOut2Change(TObject *Sender)
{
int Out2Selection = cbxSaturdayOut2->ItemIndex;
int In2Selection = cbxSaturdayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxSaturdayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayIn1Change(TObject *Sender)
{
int In1Selection = cbxSundayIn1->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
if( Out1Selection < In1Selection )
cbxSundayIn1->ItemIndex = Out1Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayOut1Change(TObject *Sender)
{
int In1Selection = cbxSundayIn1->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
int In2Selection = cbxSundayIn2->ItemIndex;
if( Out1Selection < In1Selection )
cbxSundayOut1->ItemIndex = 24;
if( Out1Selection > In2Selection )
cbxSundayOut1->ItemIndex = In2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayIn2Change(TObject *Sender)
{
int In2Selection = cbxSundayIn2->ItemIndex;
int Out1Selection = cbxSundayOut1->ItemIndex;
int Out2Selection = cbxSundayOut2->ItemIndex;
if( In2Selection < Out1Selection )
cbxSundayIn2->ItemIndex = 24;
if( In2Selection > Out2Selection )
cbxSundayIn2->ItemIndex = Out2Selection;
CalcTotalTime();
}
//---------------------------------------------------------------------------
void __fastcall TfrmTimeSheet::cbxSundayOut2Change(TObject *Sender)
{
int Out2Selection = cbxSundayOut2->ItemIndex;
int In2Selection = cbxSundayIn2->ItemIndex;
if( Out2Selection < In2Selection )
cbxSundayOut2->ItemIndex = 34;
CalcTotalTime();
}
//---------------------------------------------------------------------------
|
- Test your program.
|