A dynamic link library or DLL is a program that
another program needs for its functionality. It usually has an extension
of .dll although it could sometimes have another extension. DLLs are
created for various reasons, the most regular of which allows different
programs to "call" the same DLL.
|
- Start Borland C++ Builder if you didn't yet. From the Standard
toolbar, click the New button
.
- From the New Items dialog box, click DLL Wizard

- Click OK.
- On the DLL Wizard, click the C++ radio button and only the Use VCL
check box

- Click OK
- To save the current project, on the main menu, click File -> Save
All
- To make
things easier, from the Save Unit1 As dialog box, locate the common
folder called Programs and
display it in the Save In combo box.
- Click the Create New Folder button, type DLLExercise and press
Enter.
- Double-click the new folder to display it in the Save In combo box.
In the File Name edit box, change the name of the unit to DLLUnit and
click Save.
- Change the name of the project to DLLProject and click Save.
- Examine and read the whole file, especially the commented section.
- On top of the DLLEntryPoint() function, declare the needed functions
and implement them as follows:
//---------------------------------------------------------------------------
#include <vcl.h>
#include <windows.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
//---------------------------------------------------------------------------
// This function is used to calculate the total area of a parallelepid rect
double BoxArea(double L, double H, double W);
// This function is used to calculate the volume of a parallelepid rectangle
double BoxVolume(double L, double H, double W);
//---------------------------------------------------------------------------
int WINAPI DllEntryPoint(HINSTANCE hinst, unsigned long reason, void* lpReserved)
{
return 1;
}
//---------------------------------------------------------------------------
double BoxArea(double L, double H, double W)
{
return 2 * ((L*H) + (L*W) + (H*W));
}
//---------------------------------------------------------------------------
double BoxVolume(double L, double H, double W)
{
return L * H * W;
}
//---------------------------------------------------------------------------
|
- Because the above functions cannot be accessed from outside the DLL,
we will provide a function that can pass data from outside and to the
external programs.
Declare such a function as follows:
//---------------------------------------------------------------------------
#include <vcl.h>
#include <windows.h>
#pragma hdrstop
//---------------------------------------------------------------------------
#pragma argsused
//---------------------------------------------------------------------------
// This function is used to calculate the total area of a parallelepid rect
double BoxArea(double L, double H, double W);
// This function is used to calculate the volume of a rectangular parallelepid
double BoxVolume(double L, double H, double W);
// This function is used to get the dimensions of a rectangular parallelepid
// calculate the area and volume, then pass the calculated values to the
// function that called it.
extern "C" __declspec(dllexport)void BoxProperties(double Length, double Height,
double Width, double& Area, double& Volume);
//---------------------------------------------------------------------------
int WINAPI DllEntryPoint(HINSTANCE hinst, unsigned long reason, void* lpReserved)
{
return 1;
}
//---------------------------------------------------------------------------
double BoxArea(double L, double H, double W)
{
return 2 * ((L*H) + (L*W) + (H*W));
}
//---------------------------------------------------------------------------
double BoxVolume(double L, double H, double W)
{
return L * H * W;
}
//---------------------------------------------------------------------------
void BoxProperties(double L, double H, double W, double& A, double& V)
{
A = BoxArea(L, H, W);
V = BoxVolume(L, H, W);
}
//---------------------------------------------------------------------------
|
- To save your project, on the main menu, click File -> Save All.
- To build the DLL, on the main menu, click Project -> Build
DLLProject.
During the building process, a dialog box will display the evolution.
If there is a mistake somewhere, correct it. When everything goes fine,
the dialog box will disappear.
- To get the DLL ready for use, you will have to import it using the Implib utility. To do that, access the Command Prompt (it depends on
the operating system you are using. In Win2000/XP you can click Start
-> Programs -> Accessories -> Command Prompt). Type CD\ to
remove whatever directory is displaying.
- Display the directory where the DLL project was created. For this
exercise, this would be:
C:\Programs\DLLExercise
(this means you will type CD Programs\DLLExercise).
- Once you are inside the folder of the DLL project, type implib
DLLProject.lib DLLProject.dll and press Enter
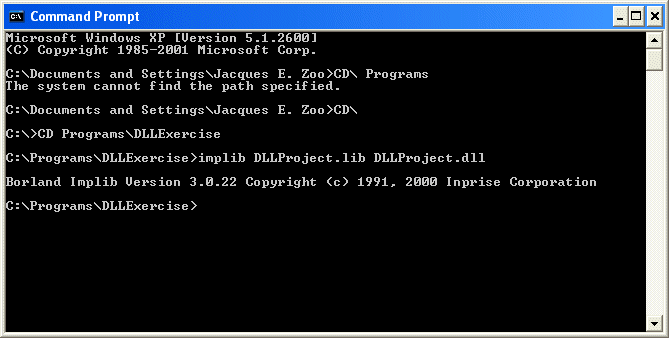
- When the DLL has been imported, type Exit and press Enter.
- Save your project
|
|
|