The Edit control is equipped with many events. Some of
these events are from its parent class the TCustomEdit
class and some others are from ancestor classes.
An edit control shows that it has focus when the caret
blinks in it. To use an edit control, the user can click it. When this
happens the control fires an OnClick event. If the form or
container has many controls, the user can press Tab continuously until the
caret displays in the edit box. In this case, the control would fire an
OnEnter event.
After using the edit control, the user can click another
control or the user can press Tab. In both cases, the edit control that had
the caret loses focus. At this time, the control fires an OnExit
event.
Practical
Learning: Using the Lost Focus
|
|
- On the form, click the Radius edit control
- In the Object Inspector, click Events and double-click OnExit
- Implement the event as follows:
//---------------------------------------------------------------------------
#include <vcl.h>
#include <math.h>
#pragma hdrstop
#include "Evaluation.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TfrmEvaluation *frmEvaluation;
//---------------------------------------------------------------------------
__fastcall TfrmEvaluation::TfrmEvaluation(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void __fastcall TfrmEvaluation::edtRadiusExit(TObject *Sender)
{
double dRadius = 0.00;
try {
dRadius = StrToFloat(edtRadius->Text);
edtDiameter->Text = FloatToStrF(dRadius * 2,
ffFixed, 12, 5);
edtCircumference->Text = FloatToStrF(dRadius * 2 * M_PI,
ffFixed, 12, 5);
edtArea->Text = FloatToStrF(dRadius * dRadius * 4.0 * M_PI,
ffFixed, 12, 5);
edtVolume->Text = FloatToStrF(dRadius * dRadius *
dRadius * 4.0 * M_PI / 3.00,
ffFixed, 12, 5);
}
catch (EConvertError *)
{
ShowMessage(L"The value you provided for the radius is not acceptable");
}
catch(...)
{
}
}
//---------------------------------------------------------------------------
- Press F9 to execute
- In the radius edit box, enter a number, such as 48.69 and press Tab

- Close the form and return to your programming environment
- On the main menu, click File -> Close All
- When asked whether you want to save, if you had decided to save the
project, click Yes. Otherwise, click No
The OnChange() event occurs as the user is typing
text in the control. This happens as the user is changing the content of an
edit control; this sends a message that the content of the edit box has
changed or has been updated. You can use this event to check, live, what the
user is doing in the edit box. For example, if you create a dialog with a
first and last names edit boxes, you can use another edit box to display the
full name. The controls could be drawn as follows:
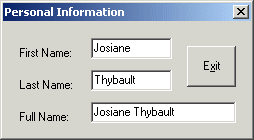
You can implement the OnChange event of the first
name edit box as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmPersInfo::edtFirstNameChange(TObject *Sender)
{
edtFullName->Text = edtFirstName->Text + " " + edtLastName->Text;
}
//---------------------------------------------------------------------------
When the second edit box is being edited, you can
implement its OnChange event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmPersInfo::edtLastNameChange(TObject *Sender)
{
edtFullName->Text = edtFirstName->Text + " " + edtLastName->Text;
}
//---------------------------------------------------------------------------
Practical
Learning: Changing the Text of an Edit Control
|
|
- To create a new application, on the main menu, click File -> New ->
VCL Forms Application - C++Builder
- In the Tool Palette, click Standard
- Click TEdit and click the form
- In the Object Inspector, click Text and press Delete
- Click Font and click its ellipsis button
- Select the following options:
Font: Georgia Font style: Bold
Size: 24 Color: Blue
- Click OK
- On the form, right-click the edit control -> Edit -> Copy
- Right-click the form -> Edit -> Paste
- On the form, double-click the first text box to generate its
OnChange event
- Implement it as follows:
//---------------------------------------------------------------------------
void __fastcall TForm1::Edit2Change(TObject *Sender)
{
Edit2->Text = Edit1->Text;
}
//---------------------------------------------------------------------------
- Press F9 to test the application
- When the form comes up, type a few characters
- Besides the character keys, press the Backspace, the Space, the
Shift, the Home, and other keys while typing
- Close the form and return to your programming environment
- On the main menu, click File -> Close All
- When asked whether you want to save the project, click No
Using the Keyboard Events
|
|
The edit control recognizes all the events associated
with the keyboard and these are particularly important because the edit box
is the most commonly used control for typing. All three keyboard events are
dealt with sequentially when the user types. When the user presses a key to
type, the control fires an OnKeyDown event. As soon as the user releases the
key, the control fires an OnKeyUp event. If you want to find out what key
the user had pressed, use the OnKeyPress event.
Practical
Learning: Using a Keyboard Event
|
|
- To create a new application, in the Tool Palette, click C++Builder
Projects and double-click the VCL Forms Application icon
- In the Tool Palette, click Standard
- Click TEdit and click the form
- On the form, make sure the edit control is still selected.
In the
Object Inspector, click Text, type write.exe and press
Enter
- Still in the Object Inspector, click Events
- Double-click the right side of OnKeyPress
- In the top section of the file, under #include <vcl.h>, type
#include <stdio.h>
- Scroll down and implement the event as follows:
//---------------------------------------------------------------------------
void __fastcall TForm1::Edit1KeyPress(TObject *Sender, wchar_t &Key)
{
char strURL[100];
if( Key == VK_RETURN )
{
sprintf(strURL, "%s", Edit1->Text.t_str());
ShellExecute(Handle,
"open",
Edit1->Text.t_str(),
NULL,
NULL,
SW_SHOWNORMAL);
}
}
//---------------------------------------------------------------------------
- Press F9 to execute and test the application
- When the form opens, press Enter
- Close WordPad
- In the text box, replace the text with the address of a web site,
such as http://www.embarcadero.com,
and press Enter
- Close the form and return to your programming environment
- On the main menu, click File -> Close All
- When asked whether you want to save the project, click No
Additional Characteristics of Edit Controls
|
|
To use an edit control, the user can enter text or
change the text in it. To keep track of the changes, the TCustomEdit
class is equipped with a Boolean property named Modified
and that its child controls inherit:
__property bool Modified = {read=GetModified,write=SetModified};
You can programmatically acknowledge that the control
has been modified by setting the Modified property to true.
Whenever the user changes the text in the edit control,
the control fires an event named OnChange. This event is of type a
TNotifyEvent type. This event is useful if you want to display a
notification that the text is not as it was when the form opened.
The CharCase property of an edit control allows
the content of a text box to apply a character case of your choice. The
CharCase property is controlled by the TEditCharCase enumerator
defined as follows:
enum TEditCharCase { ecNormal, ecUpperCase, ecLowerCase };
By default, it is set to ecNormal, which respects
whatever character case is typed in an edit box. Otherwise, you can set it
to ecUpperCase or ecLowerCase to set the edit box’ characters
to uppercase or lowercase respectively.
Text typed in an edit box appears with its corresponding
characters unless you changed the effect of the CharCase property
from ecNormal. This allows the user to see and be able to read the
characters of an edit. If you prefer to make them un-readable, you can use
the PasswordChar property. Although this property is a char type of
data, changing it actually accomplishes two things. Its default value is #0,
which means that the characters typed in it will be rendered in their
alphabetic corresponding and readable characters. If you change it, for
example to *, any character typed in it would be un-readable and be replaced
by the value of this property. You can use any alphabetic character or digit
to represent the characters that would be typed but you must provide only
one character. Alternatively, you can specify an ASCII character instead. To
do this, type # followed by the number representing the ASCII character. For
example, #98 would display b for each character.
Selecting the Content of an Edit Control
|
|
When using a form, the user can press Tab to move from
one control to another. By default, when such a control receives focus from
the user pressing Tab, the whole text in an edit control is selected. This
is controlled by the AutoSelect property. If you do not want that,
you can set the AutoSelect Boolean property to false.
One of the actions the user may be asked to perform on
an edit box is to type text in it. If the control already contains text, the
user can read it. Another common action the user performs is to select text
contained in the control. The user must have the option to select only part
of the text or its whole content. To do this, the user can click and hold
the mouse on one end of the selection and drag to the desired end. This
allows for partial selection. The area where the user start dragging during
selection is represented by the SelStart property. When the user ends
the selections, the length of the text selected is represented by the
SelLength property. The portion of text selected is represented by the
SelText property, which is an AnsiString type. These properties allow
you either to programmatically select text or to get information about text
that the user would have selected in the edit box.
The edit control is based on the TEdit class
whose immediate parent is TCustomEdit. Like every VCL class, it has a
constructor and a destructor. The constructor can be used to dynamically
create an edit control. The TEdit class provides other methods
derived from the control’s parent or from ancestor classes.
We saw earlier the properties related to the selection
of text from an edit control performed either by you or by a user.
Alternatively, the user may want to select the whole content of the control.
To programmatically select the whole text of an edit control, call the
SelectAll() method. Its syntax is:
void __fastcall SelectAll();
If the user or another control or action had selected
text on the edit control, you can delete the selection using the
ClearSelection() method of the TCustomEdit class. Its
syntax is:
void __fastcall ClearSelection(void);
This method first finds if some text is selected. If so,
the selected text would be discarded. If nothing is selected, the method
would not do anything. To delete a selected text, you can write:
//---------------------------------------------------------------------------
void __fastcall TForm1::btnClearSelectionClick(TObject *Sender)
{
Edit1->ClearSelection();
}
//---------------------------------------------------------------------------
The contents of an edit box can be empty or contain some
text. If you want it empty, use the Clear() method of the
TCustomEdit class. Its syntax is:
virtual void __fastcall Clear(void);
This would delete the whole contents of the control:
//---------------------------------------------------------------------------
void __fastcall TForm1::btnClearClick(TObject *Sender)
{
Edit1->Clear();
}
//---------------------------------------------------------------------------
The edit control natively supports regular operations
performed on a text control such as undoing an action, cutting or copying
text from the control, pasting text from another control. The methods used
to accomplish these assignments are Undo(), CutToClipboard(),
CopyToClipboard(), PasteFromClipboard(). Their syntaxes are:
void __fastcall Undo(void);
void __fastcall CutToClipboard(void);
void __fastcall CopyToClipboard(void);
void __fastcall PasteFromClipboard(void);
|
|