Characteristics of the SpinEdit Control
|
|
The Minimum and Maximum Values
|
|
The spin edit control is used to display integer values
in the edit box section of the control. The displaying value is controlled
by the MinValue and the MaxValue properties that can visually
be set in the Object Inspector. You can also specify their values
programmatically as follows:
//---------------------------------------------------------------------------
void __fastcall TForm1::FormCreate(TObject *Sender)
{
SpinEdit1->MinValue = -12;
SpinEdit1->MaxValue = 228;
}
//---------------------------------------------------------------------------
By default, the values of a spin edit control increase
by 1 and decrease by –1. If that value is not appropriate for your
application, you can change it using the Increment property in the Object
inspector.
When a spin edit control appears at startup, the control
displays the value 0 even if its MinValue is set to a value other
than 0. To set the default position of the spin edit control, use the
Value property. You can control it at design time or at
runtime. If you set the Value property to a value that is
less than the MinValue, the value would be automatically set to the
minimum value. If you try to set it to a value greater than the MaxValue
in the Object Inspector, the Value would be reset to the MaxValue.
Practical
Learning: Using the Spin Button
|
|
- On the form, double-click the spin edit control
- Implement the event as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmPublisher::sedQuantityChange(TObject *Sender)
{
int Quantity;
double UnitPrice, TotalPrice;
Quantity = sedQuantity->Text.ToInt();
if( Quantity < 20 )
UnitPrice = 5.00;
else if( Quantity < 50 )
UnitPrice = 3.50;
else if( Quantity < 100 )
UnitPrice = 2.25;
else if( Quantity < 500 )
UnitPrice = 1.85;
else
UnitPrice = 0.95;
TotalPrice = Quantity * UnitPrice;
edtUnitPrice->Text = edtUnitPrice->Text.FormatFloat("#,##0.00", UnitPrice);
edtTotalPrice->Text = edtTotalPrice->Text.FormatFloat("#,##0.00", TotalPrice);
}
//---------------------------------------------------------------------------
- Save all
- To test the form, on the main menu, click Run -> Run
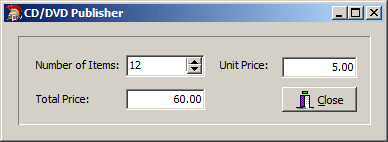

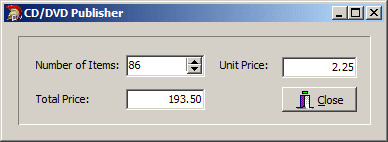


- After experimenting with the spin button, close the form and return
to your programming environment
|
|