The timer in VCL applications is made available through
the TTimer class. The TTimer class is (directly) derived
from TComponent:
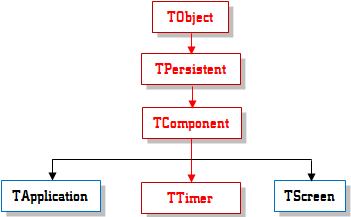
To add it to your application at design time, from the
System property page of the Tool Palette, click Timer
and click on the form.
Characteristics of a Timer
|
|
A timer is an object used to count lapses of time and
send a message when it has finished counting. The amount of time allocated
for counting is called an interval. The Interval is probably the most
important characteristic of the timer because it measures and controls the
total time needed to perform a complete count. The Interval is
measured in milliseconds. Like any counter, the lower the value, the faster
the count will finish, and the higher the value, the longer the count (if
you ask one kid to count from 1 to 10 and you ask another to count from 1 to
20 and shout when finished, the first kid would finish first and would shout
first). The amount of interval you specify will depend on what you are
trying to do.
One of the ways you can use a timer is to decide when it
should start counting. In some applications, you may want the control to
work full-time while in some other applications, you may want the control to
work only in response to an intermediate event. The ability to stop and
start a timer is set using the Enabled Boolean property. When, or as
soon as, this property is set to true, the control starts counting. If,
when, or as soon as, the Enabled property is set to false, the
control stops and resets its counter to 0.
Practical
Learning: Using Timer Controls
|
|
- On the Tool Palette, click System
- Click TTimer

- Click the form
- On the form, double-click the TTimer1 control to access its OnTimer
event
- Change its code as follows:
//---------------------------------------------------------------------------
void __fastcall TfrmTraffic::Timer1Timer(TObject *Sender)
{
// If the current color is red
if( Light == CurrentLight::Red )
{
// Change the amount of time to 1500 seconds
Timer1->Interval = 1500;
// Change the color to orange
imgLight->Picture->LoadFromFile(L"OrangeLight.bmp");
// Update the light tag
Light = CurrentLight::Orange;
}
// But if the color is orange
else if( Light == CurrentLight::Orange )
{
// Change the amount of time
Timer1->Interval = 7500;
// Change the color to green
imgLight->Picture->LoadFromFile(L"GreenLight.bmp");
// Update the light sentinel
Light = CurrentLight::Green;
}
// Otherwise, if the color is green
else // if( Light == CurrentLight::Green )
{
// Change the amount of time
Timer1->Interval = 5000;
// Change the color to red
imgLight->Picture->LoadFromFile(L"RedLight.bmp");
// Update the light tag
Light = CurrentLight::Red;
}
}
//---------------------------------------------------------------------------
- Save all
- Press F9 to test the program
|
|