|
Consider a floating number such as 12.155. As you can
see, this number is between integer 12 and integer 13
|
In the same way, consider a number such as –24.06. As
this number is negative, it is between –24 and –25, with –24 being
greater.
In arithmetic, the ceiling of a number is the closest
integer that is greater or higher than the number considered. In the first
case, the ceiling of 12.155 is 13 because 13 is the closest integer
greater than or equal to 12.155. The ceiling of –24.06 is –24.
In C++, the function used to obtain the ceiling of a
number uses the following syntax:
double ceil(double Value);
The function takes one argument, which is the floating
number to be evaluated, and the function returns a double-precision number
that is the integer that is greater than or equal to Value. Here is an
example:
//---------------------------------------------------------------------------
#include <vcl.h>
#include <math.h>
#pragma hdrstop
#include "Exercise.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TfrmExercice *frmExercice;
//---------------------------------------------------------------------------
__fastcall TfrmExercice::TfrmExercice(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void __fastcall TfrmExercice::btnCeilingClick(TObject *Sender)
{
double Number = edtNumber->Text.ToDouble();
double Ceiling = ceil(Number);
edtCeiling->Text = UnicodeString(Ceiling);
}
//---------------------------------------------------------------------------
Here is an example of running this code:
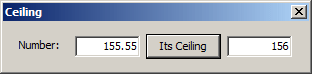

In the VCL, the function used to get the ceiling of a
number is named Ceil and its syntax is:
int __fastcall Ceil(Extended Value);
The Ceil() function takes an argument that
represents a long double value. The function returns the greater or equal
integer of Value. To use the Ceil() function, include the
math.hpp header to your program. Here is an example:
//---------------------------------------------------------------------------
#include <vcl.h>
#include <math.hpp>
#pragma hdrstop
#include "Exercise.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TForm1 *Form1;
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
}
//---------------------------------------------------------------------------
void __fastcall TForm1::btnCeilingClick(TObject *Sender)
{
Extended Number = edtNumber->Text.ToDouble();
edtCeiling->Text = Ceil(Number);
}
//---------------------------------------------------------------------------
Here is an example of running this code:
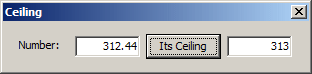
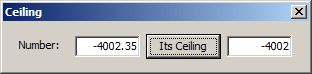