Practical
Learning: Creating the Application
|
|
- Start a new Windows Application named PayrollProcessing2
- In the Solution Explorer, right-click Form1.vb and click Rename
- Type PayrollProcessing.vb and press Enter
- Design the form as follows:
- On the form, double-click the Calculate button and implement its event
as follows:
Private Sub btnCalculateClick(ByVal sender As System.Object,
ByVal e As System.EventArgs)
Handles btnCalculate.Click
Dim Monday1 As Double, Monday2 As Double
Dim Tuesday1 As Double, Tuesday2 As Double
Dim Wednesday1 As Double, Wednesday2 As Double
Dim Thursday1 As Double, Thursday2 As Double
Dim Friday1 As Double, Friday2 As Double
Dim Saturday1 As Double, Saturday2 As Double
Dim Sunday1 As Double, Sunday2 As Double
Dim TotalHoursWeek1 As Double, TotalHoursWeek2 As Double
Dim RegularHours1 As Double, RegularHours2 As Double
Dim OvertimeHours1 As Double, OvertimeHours2 As Double
Dim RegularAmount1 As Double, RegularAmount2 As Double
Dim OvertimeAmount1 As Double, OvertimeAmount2 As Double
Dim RegularHours As Double, OvertimeHours As Double
Dim RegularAmount As Double, OvertimeAmount As Double
Dim TotalEarnings As Double
Dim HourlySalary As Double
Dim OvertimeSalary As Double
' Retrieve the hourly salary
Try
HourlySalary = CDbl(txtHourlySalary.Text)
Catch ex As Exception
MsgBox("The value you typed for the salary is invalid \n" &
"Please try again")
txtHourlySalary.Focus()
End Try
' Retrieve the value of each day worked
Try
Monday1 = CDbl(txtMonday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtMonday1.Focus()
End Try
Try
Tuesday1 = CDbl(txtTuesday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtTuesday1.Focus()
End Try
Try
Wednesday1 = CDbl(txtWednesday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtWednesday1.Focus()
End Try
Try
Thursday1 = CDbl(txtThursday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtThursday1.Focus()
End Try
Try
Friday1 = CDbl(txtFriday1.Text)
Catch ex As FormatException
MsgBox("You typed an invalid value\n" &
"Please try again")
txtFriday1.Focus()
End Try
Try
Saturday1 = CDbl(txtSaturday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtSaturday1.Focus()
End Try
Try
Sunday1 = CDbl(txtSunday1.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtSunday1.Focus()
End Try
Try
Monday2 = CDbl(txtMonday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtMonday2.Focus()
End Try
Try
Tuesday2 = CDbl(txtTuesday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtTuesday2.Focus()
End Try
Try
Wednesday2 = CDbl(txtWednesday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtWednesday2.Focus()
End Try
Try
Thursday2 = CDbl(txtThursday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtThursday2.Focus()
End Try
Try
Friday2 = CDbl(txtFriday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtFriday2.Focus()
End Try
Try
Saturday2 = CDbl(txtSaturday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtSaturday2.Focus()
End Try
Try
Sunday2 = CDbl(txtSunday2.Text)
Catch ex As Exception
MsgBox("You typed an invalid value\n" &
"Please try again")
txtSunday2.Focus()
End Try
' Calculate the total number of hours for each week
TotalHoursWeek1 = Monday1 + Tuesday1 + Wednesday1 +
Thursday1 + Friday1 + Saturday1 + Sunday1
TotalHoursWeek2 = Monday2 + Tuesday2 + Wednesday2 +
Thursday2 + Friday2 + Saturday2 + Sunday2
' The overtime is paid time and half
OvertimeSalary = HourlySalary * 1.5
' If the employee worked under 40 hours, there is no overtime
If TotalHoursWeek1 < 40 Then
RegularHours1 = TotalHoursWeek1
RegularAmount1 = HourlySalary * RegularHours1
OvertimeHours1 = 0.0
OvertimeAmount1 = 0.0
' If the employee worked over 40 hours, calculate the overtime
ElseIf TotalHoursWeek1 >= 40 Then
RegularHours1 = 40
RegularAmount1 = HourlySalary * 40
OvertimeHours1 = TotalHoursWeek1 - 40
OvertimeAmount1 = OvertimeHours1 * OvertimeSalary
End If
If TotalHoursWeek2 < 40 Then
RegularHours2 = TotalHoursWeek2
RegularAmount2 = HourlySalary * RegularHours2
OvertimeHours2 = 0.0
OvertimeAmount2 = 0.0
ElseIf TotalHoursWeek2 >= 40 Then
RegularHours2 = 40
RegularAmount2 = HourlySalary * 40
OvertimeHours2 = TotalHoursWeek2 - 40
OvertimeAmount2 = OvertimeHours2 * OvertimeSalary
End If
RegularHours = RegularHours1 + RegularHours2
OvertimeHours = OvertimeHours1 + OvertimeHours2
RegularAmount = RegularAmount1 + RegularAmount2
OvertimeAmount = OvertimeAmount1 + OvertimeAmount2
TotalEarnings = RegularAmount + OvertimeAmount
txtRegularTime.Text = FormatNumber(RegularHours)
txtOvertime.Text = FormatNumber(OvertimeHours)
txtRegularAmount.Text = FormatNumber(RegularAmount)
txtOvertimeAmount.Text = FormatNumber(OvertimeAmount)
txtNetPay.Text = FormatNumber(TotalEarnings)
End Sub
|
- In the Class Name combo box, select calTimeFrame
- In the Method Name combo box, select DateSelected and implement the
event as follows:
Private Sub calTimeFrameDateSelected(ByVal sender As Object,
ByVal e As System.Windows.Forms.DateRangeEventArgs)
Handles calTimeFrame.DateSelected
Dim DateStart As DateTime = e.Start
If DateStart.DayOfWeek <> DayOfWeek.Monday Then
MsgBox("The first day of your time frame must be a Monday")
End If
End Sub
|
- Execute the application and try selecting a range of dates
- Close the form and return to your programming environment
- Display the form
- On the form, click the calendar control and drag its right border so it
can display two months:
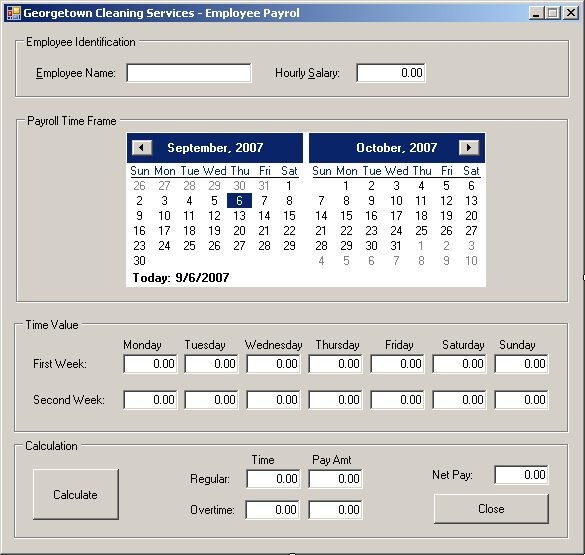
- Right-click the form and click View Code
- Under the previous End Sub line, create the following procedure:
Private Sub EnableForInvalidDate(ByVal Enable As Boolean)
If Enable = True Then
txtMonday1.Enabled = True
txtMonday2.Enabled = True
txtTuesday1.Enabled = True
txtTuesday2.Enabled = True
txtWednesday1.Enabled = True
txtWednesday2.Enabled = True
txtThursday1.Enabled = True
txtThursday2.Enabled = True
txtFriday1.Enabled = True
txtFriday2.Enabled = True
txtSaturday1.Enabled = True
txtSaturday2.Enabled = True
txtSunday1.Enabled = True
txtSunday2.Enabled = True
txtRegularTime.Enabled = True
txtRegularAmount.Enabled = True
txtOvertime.Enabled = True
txtOvertimeAmount.Enabled = True
txtNetPay.Enabled = True
btnCalculate.Enabled = True
ElseIf Enable = False Then
txtMonday1.Enabled = False
txtMonday2.Enabled = False
txtTuesday1.Enabled = False
txtTuesday2.Enabled = False
txtWednesday1.Enabled = False
txtWednesday2.Enabled = False
txtThursday1.Enabled = False
txtThursday2.Enabled = False
txtFriday1.Enabled = False
txtFriday2.Enabled = False
txtSaturday1.Enabled = False
txtSaturday2.Enabled = False
txtSunday1.Enabled = False
txtSunday2.Enabled = False
txtRegularTime.Enabled = False
txtRegularAmount.Enabled = False
txtOvertime.Enabled = False
txtOvertimeAmount.Enabled = False
txtNetPay.Enabled = False
btnCalculate.Enabled = False
End If
End Sub
|
- In the Class Name combo box, select calTimeFrame
- Change the DateSelected event as follows:
Private Sub calTimeFrameDateSelected(ByVal sender As Object,
ByVal e As System.Windows.Forms.DateRangeEventArgs)
Handles calTimeFrame.DateSelected
Dim DateStart As DateTime = e.Start
' Each payroll will cover 2 weeks
' This application assumes that the company started
' implementing the time sheet on January 1st, 2007
' Our week day will go from Monday of the first week
' to Sunday of the following week
' The first date of the time frame must be a Monday
If DateStart.DayOfWeek <> DayOfWeek.Monday Then
MsgBox("The first day of your time frame must be a Monday")
EnableForInvalidDate(False)
Exit Sub
Else
EnableForInvalidDate(True)
End If
' Now that the user has selected a date that starts on Monday,
' We will check that it corresponds to
' 2 weeks by 2 weeks after January 1st, 2007
' To start, we must get the difference of days between
' the selected starting date and January 1st, 2007
Dim tmDifference As TimeSpan =
DateStart.Subtract(New DateTime(2007, 1, 1))
Dim days As Integer = tmDifference.Days
' Now that we have the number of days,
' this number must be divisible by 14 (2 weeks)
If (days Mod 14) <> 0 Then
MsgBox("Invalid starting period - Please try again")
EnableForInvalidDate(False)
Exit Sub
Else
EnableForInvalidDate(True)
End If
' Now that we have a valid starting period,
' let's help the user and select the time period
Dim range As DateTime = e.Start.AddDays(-1)
calTimeFrame.SelectionEnd = range.AddDays(14)
End Sub
|
- In the Class Name combo box, select btnClose
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCloseClick(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnClose.Click
End
End Sub
|
- Execute the application and try selecting a range of dates
- Close the form and return to your programming environment
- On the form, click the calendar control
- In the Properties window, change the following values:
BackColor: 255, 192, 128
ForeColor: Maroon
TitleBackColor: 192, 64, 0
TitleForeColor: Yellow
TrailingForeColor: 255, 255, 192
- Save the form
- On the form, click the calendar control and, in the Properties window,
double-click ShowToday to set its value to False
- Execute the application to test it

- Close the form and return to your programming environment
|
|