The Select Case Statement
|
|
|
If you have a large number of conditions to examine, the
If...Then...Else statement will go through each one of them. The
Visual Basic language offers the alternative of jumping to the statement
that applies to the state of a condition. This is referred to as a select
case condition. It uses the keywords Select and
Case.
|
The formula of the Select Case statement is:
Select Case Expression
Case Expression1
Statement1
Case Expression2
Statement2
Case Expression_X
Statement_X
End Select
The statement starts with Select Case and ends
with End Select. On the right side of Select Case, enter a
value, the Expression factor, that will be used as a tag. The value
of Expression can be a Boolean value (a Boolean type), a
character or a string (a String type), a natural number (a Byte,
an Integer, or a Long type), a decimal number (a Single
or a Double type), a date or time value (a Date type), an
enumeration (an Enum type), or else (a Variant type).
Inside the Select Case and the End Select
lines, you provide one or more sections that each contains a
Case keyword followed by a value. The value on
the right side of a Case, Expresion1,
Expresion2, or Expresion_X, must be the same type as the value
of Expression or it can be implied from it. After the case and its
expression, you can write a statement.
When this section of code is accessed, the value of
Expression is considered. Then the value of Expression is
compared to each Expression_X of each case:
- If the value of Expression1 is equal to that of
Expression, then Statement1 is executed.
If the value of
Expression1 is not equal to that of Expression, then the
interpreter moves to Expression2
- If the value of Expression2 is equal to that of
Expression, then Statement2 is executed
- This will continue down to the last Expression_X
Here is an example:
Private Sub cmdFunction_Click()
Dim Answer As Byte
Answer = CByte(InputBox( _
"One of the following is not a Visual Basic keyword" & vbCrLf & _
"1) Function" & vbCrLf & _
"2) Except" & vbCrLf & _
"3) ByRef" & vbCrLf & _
"4) Each" & vbCrLf & vbCrLf & _
"Your Answer? "))
Select Case Answer
Case 1
MsgBox("Wrong: Function is a Visual Basic keyword." & vbCrLf & _
"It is used to create a procedure of a function type")
Case 2
MsgBox("Correct: Except is not a keyword in " & vbCrLf & _
"Visual Basic but __except is a C++ " & vbCrLf & _
"keyword used in Exception Handling")
Case 3
MsgBox("Wrong: ByRef is a Visual Basic keyword used " & vbCrLf & _
"to pass an argument by reference to a procedure")
Case 4
MsgBox("Wrong: The ""Each"" keyword is used in " & vbCrLf & _
"Visual Basic in a type of looping " & vbCrLf & _
"used to ""scan"" a list of item.")
End Select
End Sub
Here is an example of running the program:
The above code supposes that one of the cases will
match the value of the Expression factor. This is not always so. If
you anticipate that there could be no match between the Expression
and one of the Expressions, you can use a Case Else
statement at the end of the list. The statement would then look like this:
Select Case Expression
Case Expression1
Statement1
Case Expression2
Statement2
Case Expressionk
Statementk
Case Else
Statementk
End Select
In this case, the statement after the Case Else
will execute if none of the previous expressions matches the Expression
factor. Here is an example:
Private Sub cmdFunction_Click()
Dim Answer As Byte
Answer = CByte(InputBox( _
"One of the following is not a Visual Basic keyword" & vbCrLf & _
"1) Function" & vbCrLf & _
"2) Except" & vbCrLf & _
"3) ByRef" & vbCrLf & _
"4) Each" & vbCrLf & vbCrLf & _
"Your Answer? "))
Select Case Answer
Case 1
MsgBox("Wrong: Function is a Visual Basic keyword." & vbCrLf & _
"It is used to create a procedure of a function type")
Case 2
MsgBox("Correct: Except is not a keyword in " & vbCrLf & _
"Visual Basic but __except is a C++ " & vbCrLf & _
"keyword used in Exception Handling")
Case 3
MsgBox("Wrong: ByRef is a Visual Basic keyword used " & vbCrLf & _
"to pass an argument by reference to a procedure")
Case 4
MsgBox("Wrong: The ""Each"" keyword is used in " & vbCrLf & _
"Visual Basic in a type of looping " & vbCrLf & _
"used to ""scan"" a list of item.")
Case Else
MsgBox("Invalid Selection")
End Select
End Sub
Here is an example of running the program:
As mentioned in our introduction, the Select Case
can use a value other than an integer. For example you can use a
character:
Private Sub cmdFunction_Click()
Dim Gender As String
Gender = "M"
Select Case Gender
Case "F"
MsgBox("Female")
Case "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
End Sub
This would produce:
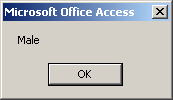
Notice that in this case we are using only upper case
characters. If want to validate lower case characters also, we may have to
create additional case sections for each. Here is an example:
Private Sub cmdFunction_Click()
Dim Gender As String
Gender = "f"
Select Case Gender
Case "f"
MsgBox("Female")
Case "F"
MsgBox("Female")
Case "m"
MsgBox("Male")
Case "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
End Sub
This would produce:
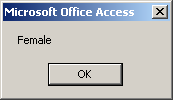
Instead of using one value for a case, you can apply
more than one. To do this, on the right side of the Case keyword,
you can separate the expressions with commas. Here are examples:
Private Sub cmdFunction_Click()
Dim Gender As String
Gender = "F"
Select Case Gender
Case "f", "F"
MsgBox("Female")
Case "m", "M"
MsgBox("Male")
Case Else
MsgBox("Unknown")
End Select
End Sub
Validating a Range of
Cases
|
|
You can use a range of values for a case. To do this,
on the right side of a Case, enter the lower
value, followed by To, followed by the higher value. Here is an
example:
Private Sub cmdFunction_Click()
Dim Age As Integer
Age = 24
Select Case Age
Case 0 To 17
MsgBox("Teen")
Case 18 To 55
MsgBox("Adult")
Case Else
MsgBox("Senior")
End Select
End Sub
This would produce:
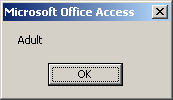
Checking Whether a Value
IS
|
|
Consider the following procedure:
Private Sub cmdFunction_Click()
Dim Number As Integer
Number = 448
Select Case Number
Case -602
MsgBox "-602"
Case 24
MsgBox "24"
Case 0
MsgBox "0"
End Select
End Sub
Obviously, this Select Case statement will work
in rare cases only when the expression of a case exactly matches the value
sought for. In reality, for this type of scenario, you could validate a
range of values. The Visual Basic language provides an alternative. You
can check whether the value of the Expression responds to a
criterion instead of an exact value. To create it, you use the Is
operator with the following formula:
Is Operator Value
You start with the Is keyword. It is followed
by one of the Boolean operators we know already: =, <>, <, <=, >, or >=.
On the right side of the Boolean operator, type the desired value. Here
are examples:
Private Sub cmdFunction_Click()
Dim Number As Integer
Number = -448
Select Case Number
Case Is < 0
MsgBox("The number is negative")
Case Is > 0
MsgBox("The number is positive")
Case Else
MsgBox("0")
End Select
End Sub
This would produce:
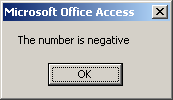
Although we used a natural number here, you can use
any appropriate logical comparison that can produce a True or a
False result. You can also combine it with the other alternatives we
saw previously, such as separating the expressions of a case with commas.
Select...Case and the Conditional Built-In
Functions
|
|
With the Select...Case statement, we saw how to
check different values against a central one and take action when one of
those matches the tag. Here is an example:
Private Sub cmdFunction_Click()
Dim Number As Integer, MembershipType As String
Number = 2
Select Case Number
Case 1
MembershipType = "Teen"
Case 2
MembershipType = "Adult"
Case Else
MembershipType = "Senior"
End Select
MsgBox "Membership Type: " & MembershipType
End Sub
This would produce:
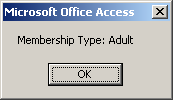
Select...Case-Related Functions: Choose()
|
|
We have learned how to check whether a condition is
True or False and take an action. Here is an example:
Private Sub cmdFunction_Click()
Dim Status As Integer, EmploymentStatus As String
Status = 1
EmploymentStatus = "Unknown"
If Status = 1 Then
EmploymentStatus = "Full Time"
End If
MsgBox "Employment Status: " & EmploymentStatus
End Sub
To provide an alternative to this operation, the
Visual Basic language provides a function named Choose. Its syntax
is:
Public Function Choose( _
ByVal Index As Double, _
ByVal ParamArray Choice() As Variant _
) As Variant
This function takes two required arguments. The fist
argument is equivalent to the ConditionToCheck of our If...Then
formula. For the Choose() function, this first argument must be a
number. This is the value against which the second argument will be
compared. Before calling the function, you must know the value of the
first argument. To take care of this, you can first declare a variable and
initialize it with the desired value. Here is an example:
Private Sub cmdFunction_Click()
Dim Status As Byte, EmploymentStatus As String
Status = 1
EmploymentStatus = Choose(Status, ...)
MsgBox ("Employment Status: " & EmploymentStatus)
End Sub
The second argument can be the Statement of our
formula. Here is an example:
Choose(Status, "Full Time")
The second argument is actually a list of values and
each value has a specific position referred to as its index. To use this
function as an alternative to the If...Then...Else operation, you
can pass two values for the second argument. To use the function in an
If...Then...Else implementation, pass two values for the second
argument. Here is an example:
Choose(Status, "Full Time", "Part Time")
The second argument to the function, which is the
first value of the Choose argument, has an index of 1. The third
argument to the function, which is the second value of the Choose
argument, has an index of 2.
When the Choose() function is called, if the
first argument has a value of 1, then the second argument is validated. If
the first argument has a value of 2, then the third argument is validated.
As mentioned already, you can retrieve the returned value of the function
and use it however you want. Here is an example:
Private Sub cmdFunction_Click()
Dim Status As Integer, EmploymentStatus As String
Status = 2
EmploymentStatus = Choose(Status, "Full Time", "Part Time")
MsgBox "Employment Status: " & EmploymentStatus
End Sub
This would produce:
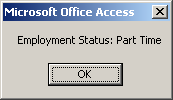
To use the Choose function as an alternative to
the If...Then...ElseIf...ElseIf condition, you can pass as many
values as you judge necessary for the second argument. The index of the
first member of the second argument would be 1. The index of the second
member of the second argument would be 2, and so on. When the function is
called, it would first get the value of the first argument, then it would
check the indexes of the available members of the second argument. The
member whose index matches the first argument would be executed. Here is
an example:
Private Sub cmdFunction_Click()
Dim Status As Byte, EmploymentStatus As String
Status = 3
EmploymentStatus = Choose(Status, _
"Full Time", _
"Part Time", _
"Contractor", _
"Seasonal")
MsgBox "Employment Status: " & EmploymentStatus
End Sub
This would produce:
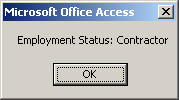
So far, we have used only strings for the values of
the second argument of the Choose() function. In reality, the
values of the second argument can be almost anything. One value can be a
constant. Another value can be a string. Yet another value can come from
calling a function. Here is an example:
Private Function ShowContractors$()
ShowContractors$ = "=-= List of Contractors =-=" & vbCrLf & _
"Martin Samson" & vbCrLf & _
"Geneviève Lam" & vbCrLf & _
"Frank Viel" & vbCrLf & _
"Henry Rickson" & vbCrLf & _
"Samuel Lott"
End Function
Private Sub cmdFunction_Click()
Dim Status As Byte, Result$
Status = 3
Result = Choose(Status, _
"Employment Status: Full Time", _
"Employment Status: Part Time", _
ShowContractors, _
"Seasonal Employment")
MsgBox Result
End Sub
This would produce:

The values of the second argument can even be of
different types.