Module Module1
Sub Main()
Dim Letter As Char
Letter = "W"
End Sub
End Module
To indicate that the value of the variable must be
treated as Char, when initializing it, you can type c or C on the right
side of the double-quoted value. Here is an example:
Module Module1
Sub Main()
Dim Letter As Char
Letter = "W"c
End Sub
End Module
To convert a value to Char, you can call CChar().
A string is an empty text, a letter, a word or a group
of words considered "as is". To declare a string variable, you
can use the String data
type. Here is an example:
Module Module1
Sub Main()
Dim Sentence As String
End Sub
End Module
If you want, you can replace the AS String
expression with
the $ symbol when declaring a string
variable. This can be done as follows:
Module Module1
Sub Main()
Dim Sentence$
End Sub
End Module
After declaring a String variable, by default,
the compiler initializes it with an empty string. Otherwise, you can initialize
it with a string of your choice. To do this, include the string between
double quotes. Here is an example: Module Module1
Sub Main()
Dim Sentence$
Sentence$ = "He called me"
End Sub
End Module
If you want to include a double-quote character in the
string, you can double it. Here is an example:
Module Module1
Sub Main()
Dim Sentence$
Sentence$ = "Then she said, ""I don't love you no more."" and I left"
MsgBox(Sentence)
End Sub
End Module
This would produce:
To convert a value to a string, call CStr()
and enter the value or the expression in its parentheses.
If the value is an appropriate date or time, CStr() would produces a
string that represents that date or that time value.
Practical
Learning: Using Strings
|
|
- Start Microsoft Visual Basic
- To create a new application, on the main menu, click File -> New ->
Project or File -> New Project
- In the Templates list, click Console Application
- Set the Name to YugoNationalBank2 and click OK
- To use a few string variables, change the program as follows:
Module Module1
Sub Main()
Dim CustomerName As String
Dim AccountNumber As String
Dim InitialDeposit As Double
Dim Withdrawals As Double
Dim CurrentBalance As Double
Dim Result As String
Dim strDeposit As String
Dim strWithdrawals As String
CustomerName = InputBox("Enter Customer Name:")
AccountNumber = InputBox("Enter Account #:")
strDeposit = InputBox("Enter Initial Deposit:")
strWithdrawals = InputBox("Enter total withdrawals:")
InitialDeposit = CDbl(strDeposit)
Withdrawals = CDbl(strWithdrawals)
CurrentBalance = InitialDeposit - Withdrawals
Result = " =-= Yugo National Bank =-=" & vbCrLf & _
"Customer Name: " & CustomerName & vbCrLf & _
"Account Number: " & AccountNumber & vbCrLf & _
"Deposits: $" & InitialDeposit & vbCrLf & _
"Withdrawals: $" & Withdrawals & vbCrLf & _
"Current Balance: $" & CurrentBalance
MsgBox(Result)
End Sub
End Module
|
- Execute the application and provide the requested values. Here is an
example:
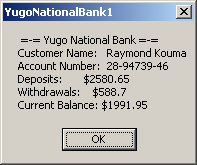
- Return to your programming environment
A date is a numeric value that represents the number
of days that have elapsed since a determined period. A time is a numeric
value that represents the number of seconds that have elapsed in a day.
To declare a variable that can hold either date
values, time values, or both, you can use the Date
data type. After the variable has been declared, you will configure it to
the appropriate value. Here are two examples:
Module Module1
Sub Main()
Dim DateOfBirth As Date
Dim KickOffTime As Date
End Sub
End Module
As mentioned above, the Date data type is used for
both date and time. Like the other values, there are rules you must
observe when dealing with date and time values. The rules are defined in
the Regional Settings Properties of the Control Panel of the computer on
which the application is run. The rules for the dates are defined in the
Date property page:
The rules for the time values are defined in the Time
property page:
When using date and time values in your applications,
you should not change these rules because the application will refer to
them when it is installed in someone else's computer.
By default, a Date variable is initialized with
January 1st, 0001 at midnight as the starting value. After declaring a Date variable, you can initialize it
with an appropriate date or time or date and time value. The value can be
included in double-quotes. Here is an example:
Module Module1
Sub Main()
Dim DateOfBirth As Date
DateOfBirth = "08/14/1982"
MsgBox(DateOfBirth)
End Sub
End Module
If you intend to use only a date value for a Date
variable, its corresponding time part would be set to midnight of the same
date. Based on this, the above program would produce:
8/14/1982 12:00:00 AM
If you intend to use only a time value for your Date
variable, the date part of the variable would be set to January 1st, 0001.
A Date value can also be included between two # signs.
Here is an example:
Module Module1
Sub Main()
Dim DateOfBirth As Date
Dim KickOffTime As Date
DateOfBirth = "08/14/1982"
KickOffTime = #6:45:00 PM#
MsgBox(DateOfBirth)
MsgBox(KickOffTime)
End Sub
End Module
To convert a value to a date or a time value, write
an appropriate date or a recognizable time in the parentheses of CDate().
If the value is appropriate, CDate() produces a Date value.
An Object can be any type of data that you want
to use in your program. In most cases, but sparingly, it can be used to
declare a variable of any type. Here are examples:
Module Module1
Sub Main()
Dim CountryName As Object
Dim NumberOfPages As Object
Dim UnitPrice As Object
CountryName = "Australia"
NumberOfPages = 744
UnitPrice = 248.95
MsgBox(CountryName)
MsgBox(NumberOfPages)
MsgBox(UnitPrice)
End Sub
End Module
If you don't specify a data type or can't figure
out what data type you want to use, you can use the Object data
type. As you can see from the result of the above program, the vbc
compiler knows how to convert the value of any Object variable to the
appropriate type. On the other hand, to convert a value or an expression to the
Object
type, you can use CObj().
A constant is a value that doesn't change. There are two types of constants you will use in your programs: those
supplied to you and those you define yourself.
To create a constant to use in your program type the Const
keyword followed by a name for the variable, followed by the assignment operator
"=", and followed by the value that the constant will hold. Here is an example:
Module Module1
Sub Main()
Const DateOfBirth = #12/5/1974#
MsgBox(DateOfBirth)
End Sub
End Module
When defining a constant like this, the compiler would know
the type of data to apply to the variable. In this case the DateOfBirth constant
holds a Date value. Still, to be more explicit, you can indicate the type
of value of the constant by following its name with the As keyword and the
desired data type. Based on this, the above program would be:
Inports System
Module Module1
Sub Main()
Const DateOfBirth As Date = #12/5/1974#
Console.Write("Date of Birth: ")
Console.WriteLine(DateOfBirth)
End Sub
End Module
As mentioned earlier, the second category of constants are
those that are built in the VBasic language. Because there are many of them and
used in different circumstances, when we need one, we will introduce and then
use it.
So far, to initialize a variable, we were using a known value.
Alternatively, you can use the Nothing constant to initialize a variable, simply
indicating that it holds a value that is not (yet) defined. Here is an example:
Module Module1
Sub Main()
Dim DateOfBirth As Date = Nothing
End Sub
End Module
If you use the Nothing keyword to initialize a variable, the
variable is actually initialized to the default value of its type. For example,
a number would be assigned 0, a date would be assigned January 1, 0001 at
midnight.
The Scope and Lifetime of a Variable |
|
The scope of a variable determines the areas of code
where the variable is available. You may have noticed that, so far, we
declared all our variables only inside of Main(). Actually, the
VBasic language allows you to declare variables outside of Main()
(and outside of a particular procedure).
A variable declared inside of a procedure such as Main()
is referred to as a local variable. Such as variable cannot be accessed by
another part (such as another procedure) of the program.
A variable that is declared outside of any procedure
is referred to as global. To declare a global variable, use the same
formula as we have done so far. For example, just above the Sub Main()
line, you can type Dim, followed by the name of the variable, the As
keyword, its type and an optional initialization. Here is an example:
Module Module1
Dim DateOfBirth As Date
Sub Main()
End Sub
End Module
As mentioned above, you can initialize the global
variable when or after declaring it. Here are two examples:
Module Module1
Dim UnitPrice As Double
Dim DateOfBirth As Date = #12/5/1974#
Sub Main()
UnitPrice = 24.95
MsgBox(DateOfBirth)
MsgBox(UnitPrice)
End Sub
End Module
As we will see when studying procedures, a global
variable can be accessed by any other procedure (or even class) of the
same file. In most cases, a global variable must be declared inside of a
module, that is, between the Module ModName and the End
Module lines but outside of a procedure. Based on this, such a
variable is said to have module scope because it is available to anything
inside of that module.
Details on Declaring Variables |
|
Declaring a Series of Variables |
|
Because a program can use different
variables, you can declare each variable on its own line. Here is an
example:
Module Module1
Sub Main()
Dim NumberOfPages As Integer
Dim TownName As String
Dim IsUppercase As Boolean
Dim MagazinePrice As Double
End Sub
End Module
It is important to know that different variables
can be declared with the same data type as in the following example:
Module Module1
Sub Main()
Dim NumberOfPages As Integer
Dim Category As Integer
Dim IsUppercase As Boolean
Dim MagazinePrice As Double
End Sub
End Module
When two variables use the same data type,
instead of declaring each on its own line, you can declare two or
more of these variables on the same line. There are two techniques
you can use:
- You can write the names of both variables on the same line,
separated by a comma, and ending the line with the common data type:
Module Module1
Sub Main()
Dim NumberOfPages, Category As Integer
Dim IsUppercase As Boolean
Dim MagazinePrice As Double
End Sub
End Module
In this case, both variables NumberOfPages and Category, are
the same type, Integer
|
- You can also declare, on the same line, variables that use different
data types. To do this, type the Dim keyword followed by the variable
name and its data type with the As keyword. Then type a comma,
followed by the other variable(s) and data type(s). Here is an example
Module Module1
Sub Main()
Dim NumberOfPages, Category As Integer
Dim IsUppercase As Boolean, MagazinePrice As Double
End Sub
End Module
In this case, the IsUppercase is of Boolean type while the
MagazinePrice is a Double |
We have indicated that when a variable is
declared, it receives a default initialization unless you decide you
specify its value. Whether such a variable has been initialized or not, at
any time, you can change its value by reassigning it a new one. Here is an
example:
Module Module1
Sub Main()
' Initializing a variable when declaring it
Dim Number As Double = 155.82
MsgBox(Number)
' Changing the value of a variable after using it
Number = 46008.39
MsgBox(Number)
End Sub
End Module
In the same way, we saw that a variable could be
declared at module scope outside of Main() and then initialize or change
its value when judged necessary. Here is an example:
Module Module1
Dim UnitPrice As Double
Dim DateOfBirth As Date = #12/5/1974#
Dim Number As Double
Sub Main()
' Initializing a variable
Number = 155.82
MsgBox(Number)
' Changing the value of a variable after using it
Number = 46008.39
MsgBox(Number)
End Sub
End Module
When declaring a variable, as the programmer, you
should have an idea of how you want to use the variable and what type of
values the variable should hold. In some cases, you may want the variable
to hold a constant value and not be able to change it. We saw earlier that
such a variable could be declared as a constant. An alternative is to
declare it with the ReadOnly keyword. While a constant variable can
be declared locally, a ReadOnly variable cannot. It must be declared
globally.
As done for a constant, when declaring a ReadOnly
variable, you should initialize. If you don't, the compiler would assign
the default value based on its type. For example, a numeric-based
variable would be initialized with 0 and a String variable would be
initialized with an empty string. As done so far, to initialize the
variable, use the assignment operator followed by the desired value.
Like a constant, after declaring and optionally
initializing a ReadOnly variable, you cannot change its value. Based on
this, the following code would produce an error:
Module Module1
Dim UnitPrice As Double
Dim DateOfBirth As Date = #12/5/1974#
ReadOnly Number As Double = 155.82
Sub Main()
' Initializing a variable
Number = 155.82
MsgBox(Number)
' Changing the value of a variable after using it
Number = 46008.39 ' Error: You cannot assign a value to
' a ReadOnly variable after initializing it
MsgBox(Number)
End Sub
End Module
This means that a ReadOnly variable must be
assigned a value once, when initializing it.
|