|
Scaling a picture consists of changing its size, either
to widen, to enlarge, to narrow, to heighten, or to shrink it. Although
there are various complex algorithms you can use to perform this operation,
the Bitmap class provides a shortcut you can use. Of course, in order to
scale a picture, you must first have one.
|
To support picture scaling, the Bitmap class provides
the following constructor:
public:
Bitmap(Image^ original, int width, int height);
The original argument is the Image, such
as a Bitmap object, that you want to scale. The width and
the height arguments represent the new size you want to apply to
the picture. After this constructor has been used, you get a bitmap with
the new size. Here is an example of using it:

public ref class Picture : public System::Windows::Forms::Form
{
public:
. . . No Change
System::ComponentModel::Container ^components;
Bitmap ^ bmpPicture;
. . . No Change
System::Void Picture_Load(System::Object^ sender,
System::EventArgs^ e)
{
bmpPicture = gcnew Bitmap(10, 10);
}
System::Void Picture_Paint(System::Object^ sender,
System::Windows::Forms::PaintEventArgs^ e)
{
e->Graphics->DrawImage(bmpPicture, 120, 12);
}
System::Void btnLoadPicture_Click(System::Object^ sender,
System::EventArgs^ e)
{
OpenFileDialog ^ dlgOpen = gcnew OpenFileDialog;
if( dlgOpen->ShowDialog() == ::DialogResult::OK )
{
String ^ strFilename = dlgOpen->FileName;
bmpPicture = gcnew Bitmap(strFilename);
int width = bmpPicture->Width;
int height = bmpPicture->Height;
txtWidth->Text = width.ToString();
txtHeight->Text = height.ToString();
Invalidate();
}
}
System::Void btnResize_Click(System::Object^ sender, System::EventArgs^ e)
{
int width, height;
try {
width = int::Parse(txtWidth->Text);
}
catch(FormatException ^)
{
MessageBox::Show(L"The value you entered for the width is not valid");
}
try {
height = int::Parse(txtHeight->Text);
}
catch(FormatException ^)
{
MessageBox::Show(L"The value you entered for the height is not valid");
}
Bitmap ^ bmpNew = gcnew Bitmap(bmpPicture, width, height);
bmpPicture = bmpNew;
Invalidate();
}
};
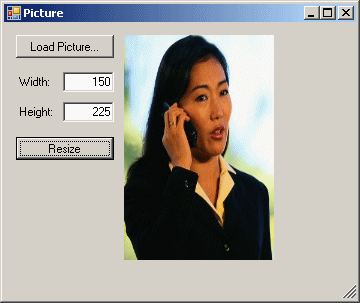
You can also specify both the width and the height as
the size. To do this, you can use the following constructor:
public:
Bitmap(Image^ original, Size newSize);
As you can see, the scaling operation could produce a
kind of distorted picture. An alternative would be to keep the ration of
both dimensions while changing the value of one.