If you have used tables in SQL Server, Microsoft
Access, Paradox, and other database environments, you probably know that
their tables automatically save data as the user changes records and moves
through cells. Although it is one of the most regularly used objects of
databases in Microsoft Visual .NET, the DataGrid control doesn't
inherently provide this functionality, but for a good reason. By default,
the DataGrid control is not meant for databases only. When a DataGrid
control displays data, it allows a user to enter new data but doesn't save
it.
Data entered in a DataGrid is extremely easy to
save. This is simply taken care of by calling the Update method of your
data adapter.
|
Practical
Learning: Performing Data Entry
|
|
- Open SQL Data Analyzer
- If you haven't yet (only if you haven't), create
the AlleyTechno database
- If you haven't yet (only if you haven't), create
the Departments table
- If you haven't yet (only if you haven't), create
the Employees table
- Open Visual C++ .NET and create a new Windows Forms Application named DataEntry1
- From Server Explorer, open the server that holds the above database then
expand the AlleyTechno database followed by the Tables node
- Drag the Employees table and drop it on the form
- Right-click the newly added sqlDataAdapter1 and click Generate Dataset...
- While the New radio button is selected, change the name of the dataset to
dsEmployees
- Click OK
- From the Toolbox, click DataGrid and click the form
- Move the DataGrid control to the upper-left section of the form and set
its Anchor property to Top, Bottom, Left, Right
- Right-click the DataGrid and click Auto Format
- In the Auto Format dialog box, select Colorful 2
- Click OK
- Set the DataGrid control's Data Source to dsEmployees1.Employees
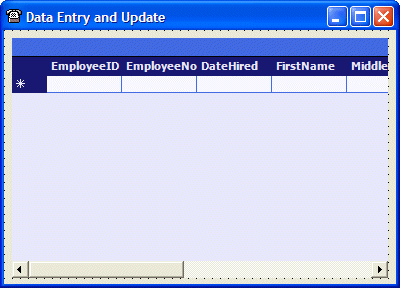
- Double-click an empty area of the form and change the Load event as
follows:
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
this->sqlDataAdapter1->Fill(this->dsEmployees1);
}
|
- Press Ctrl + F5 to test the application
- Enter a new record and navigate to a different record. Close the
form and execute the application again. Notice that the record you
added was not saved
- To allow the user to create a new record and/or to update new
changes, on the form, click the DataGrid control
- In the Properties window, click the Events button

- Double-click the CurrentCellChanged event and implement it as
follows:
private: System::Void dataGrid1_CurrentCellChanged(System::Object * sender,
System::EventArgs * e)
{
this->sqlDataAdapter1->Update(this->dsEmployees1);
}
|
- Press Ctrl + F5 to test the application
- Create a new record by entering new information
|
|