The simplest type of brush is referred to as solid. This
type of brush is simply equipped with a color and it is used to fill a shape
with it. To get a solid brush, you use the SolidBrush class defined in
the System.Drawing namespace. It has only one constructor declared with
the following syntax:
public: SolidBrush(Color color);
The color passed as argument must be a valid
definition of a Color:: Here is an example:
|
private: System::Void Form1_Paint(System::Object * sender, System::Windows::Forms::PaintEventArgs * e)
{
SolidBrush *brushBlue = new SolidBrush(Color::Blue);
e->Graphics->FillRectangle(brushBlue, 20, 20, 200, 160);
}
|
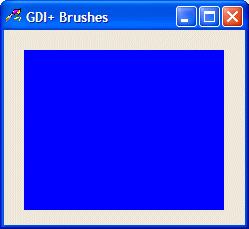 |
If you plan to use different colors to fill different
shapes, you don't have to create a new brush for each shape. At any time, before
re-using the same brush previously defined, you can simply change its Color:: For
this reason, the SolidBrush class is equipped with the Color property.
Here is an example of using it:
|
private: System::Void Form1_Paint(System::Object * sender, System::Windows::Forms::PaintEventArgs * e)
{
SolidBrush *colorizer = new SolidBrush(Color::Lime);
e->Graphics->FillRectangle(colorizer, 10, 10, 120, 120);
colorizer->Color = Color::Salmon;
e->Graphics->FillRectangle(colorizer, 140, 10, 120, 120);
colorizer->Color = Color::Aqua;
e->Graphics->FillRectangle(colorizer, 10, 140, 120, 120);
colorizer->Color = Color::Navy;
e->Graphics->FillRectangle(colorizer, 140, 140, 120, 120);
}
|
 |
Like most objects used in graphics programming, a brush
consumes the computer resources. Therefore, after using it, you can free the
resources it was using by calling the Dispose() method. Here is an example:
|
private: System::Void Form1_Paint(System::Object * sender, System::Windows::Forms::PaintEventArgs * e)
{
SolidBrush *colorizer = new SolidBrush(Color::Lime);
e->Graphics->FillRectangle(colorizer, 10, 10, 120, 120);
colorizer->Color = Color::Salmon;
e->Graphics->FillRectangle(colorizer, 140, 10, 120, 120);
colorizer->Color = Color::Aqua;
e->Graphics->FillRectangle(colorizer, 10, 140, 120, 120);
colorizer->Color = Color::Navy;
e->Graphics->FillRectangle(colorizer, 140, 140, 120, 120);
colorizer.Dispose();
}
|
|