|
Using Visual Basic .NET Functions
|
|
There is no doubt that Microsoft Visual Basic .NET has
more genuine built-in functions that any other language of the Microsoft
Visual Studio .NET (As opposed to other languages, Visual C++ .NET
is the only one that has direct access to Win32 functions without any type
of, or very little, code configuration). This is because MSVB .NET inherited an already
rich library of functions from its parent Visual Basic 6. The function
available to Visual Basic .NET are so flexible you may want to use them in
your application as if you were using MSVB without MSVB. This is easily
possible in Visual Studio .NET.
Imagine you want to perform some calculations from a
Visual C++ .NET application and you have designed a form as follows:
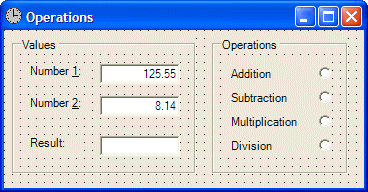
To use a Visual Basic .NET function in your code, you
must first include its DLL in the file where you would need it. To do
that, simply type
#using <Microsoft.VisualBasic.dll>
Once this is done, you can enjoy the vast array of
functions of MSVB .NET
|
|
|
#pragma once
#using <Microsoft.VisualBasic.dll>
namespace UsingVB
{
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
/// <summary>
/// Summary for Form1
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public __gc class Form1 : public System::Windows::Forms::Form
{
public:
Form1(void)
{
InitializeComponent();
}
protected:
void Dispose(Boolean disposing)
{
if (disposing && components)
{
components->Dispose();
}
__super::Dispose(disposing);
}
private: System::Windows::Forms::GroupBox * grpValues;
private: System::Windows::Forms::TextBox * txtResult;
private: System::Windows::Forms::Label * label3;
private: System::Windows::Forms::TextBox * txtNumber2;
private: System::Windows::Forms::Label * label2;
private: System::Windows::Forms::TextBox * txtNumber1;
private: System::Windows::Forms::Label * label1;
private: System::Windows::Forms::GroupBox * grpOperations;
private: System::Windows::Forms::RadioButton * rdoDivision;
private: System::Windows::Forms::RadioButton * rdoMultiplication;
private: System::Windows::Forms::RadioButton * rdoSubtraction;
private: System::Windows::Forms::RadioButton * rdoAddition;
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container * components;
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
{
this->grpValues = new System::Windows::Forms::GroupBox();
this->txtResult = new System::Windows::Forms::TextBox();
this->label3 = new System::Windows::Forms::Label();
this->txtNumber2 = new System::Windows::Forms::TextBox();
this->label2 = new System::Windows::Forms::Label();
this->txtNumber1 = new System::Windows::Forms::TextBox();
this->label1 = new System::Windows::Forms::Label();
this->grpOperations = new System::Windows::Forms::GroupBox();
this->rdoDivision = new System::Windows::Forms::RadioButton();
this->rdoMultiplication = new System::Windows::Forms::RadioButton();
this->rdoSubtraction = new System::Windows::Forms::RadioButton();
this->rdoAddition = new System::Windows::Forms::RadioButton();
this->grpValues->SuspendLayout();
this->grpOperations->SuspendLayout();
this->SuspendLayout();
//
// grpValues
//
this->grpValues->Controls->Add(this->txtResult);
this->grpValues->Controls->Add(this->label3);
this->grpValues->Controls->Add(this->txtNumber2);
this->grpValues->Controls->Add(this->label2);
this->grpValues->Controls->Add(this->txtNumber1);
this->grpValues->Controls->Add(this->label1);
this->grpValues->Location = System::Drawing::Point(8, 16);
this->grpValues->Name = S"grpValues";
this->grpValues->Size = System::Drawing::Size(184, 136);
this->grpValues->TabIndex = 9;
this->grpValues->TabStop = false;
this->grpValues->Text = S"Values";
//
// txtResult
//
this->txtResult->Location = System::Drawing::Point(88, 98);
this->txtResult->Name = S"txtResult";
this->txtResult->Size = System::Drawing::Size(80, 20);
this->txtResult->TabIndex = 11;
this->txtResult->Text = S"";
this->txtResult->TextAlign = System::Windows::Forms::HorizontalAlignment::Right;
//
// label3
//
this->label3->Location = System::Drawing::Point(16, 98);
this->label3->Name = S"label3";
this->label3->Size = System::Drawing::Size(56, 16);
this->label3->TabIndex = 10;
this->label3->Text = S"Result:";
//
// txtNumber2
//
this->txtNumber2->Location = System::Drawing::Point(88, 58);
this->txtNumber2->Name = S"txtNumber2";
this->txtNumber2->Size = System::Drawing::Size(80, 20);
this->txtNumber2->TabIndex = 9;
this->txtNumber2->Text = S"8.14";
this->txtNumber2->TextAlign = System::Windows::Forms::HorizontalAlignment::Right;
//
// label2
//
this->label2->Location = System::Drawing::Point(16, 58);
this->label2->Name = S"label2";
this->label2->Size = System::Drawing::Size(64, 16);
this->label2->TabIndex = 8;
this->label2->Text = S"Number &2:";
//
// txtNumber1
//
this->txtNumber1->Location = System::Drawing::Point(88, 26);
this->txtNumber1->Name = S"txtNumber1";
this->txtNumber1->Size = System::Drawing::Size(80, 20);
this->txtNumber1->TabIndex = 7;
this->txtNumber1->Text = S"125.55";
this->txtNumber1->TextAlign = System::Windows::Forms::HorizontalAlignment::Right;
//
// label1
//
this->label1->Location = System::Drawing::Point(16, 26);
this->label1->Name = S"label1";
this->label1->Size = System::Drawing::Size(64, 16);
this->label1->TabIndex = 6;
this->label1->Text = S"Number &1:";
//
// grpOperations
//
this->grpOperations->Controls->Add(this->rdoDivision);
this->grpOperations->Controls->Add(this->rdoMultiplication);
this->grpOperations->Controls->Add(this->rdoSubtraction);
this->grpOperations->Controls->Add(this->rdoAddition);
this->grpOperations->Location = System::Drawing::Point(208, 16);
this->grpOperations->Name = S"grpOperations";
this->grpOperations->Size = System::Drawing::Size(136, 136);
this->grpOperations->TabIndex = 8;
this->grpOperations->TabStop = false;
this->grpOperations->Text = S"Operations";
//
// rdoDivision
//
this->rdoDivision->CheckAlign = System::Drawing::ContentAlignment::MiddleRight;
this->rdoDivision->Location = System::Drawing::Point(16, 96);
this->rdoDivision->Name = S"rdoDivision";
this->rdoDivision->TabIndex = 3;
this->rdoDivision->Text = S"Division";
this->rdoDivision->CheckedChanged += new System::EventHandler(this, rdoDivision_CheckedChanged);
//
// rdoMultiplication
//
this->rdoMultiplication->CheckAlign = System::Drawing::ContentAlignment::MiddleRight;
this->rdoMultiplication->Location = System::Drawing::Point(16, 72);
this->rdoMultiplication->Name = S"rdoMultiplication";
this->rdoMultiplication->TabIndex = 2;
this->rdoMultiplication->Text = S"Multiplication";
this->rdoMultiplication->CheckedChanged += new System::EventHandler(this, rdoMultiplication_CheckedChanged);
//
// rdoSubtraction
//
this->rdoSubtraction->CheckAlign = System::Drawing::ContentAlignment::MiddleRight;
this->rdoSubtraction->Location = System::Drawing::Point(16, 48);
this->rdoSubtraction->Name = S"rdoSubtraction";
this->rdoSubtraction->TabIndex = 1;
this->rdoSubtraction->Text = S"Subtraction";
this->rdoSubtraction->CheckedChanged += new System::EventHandler(this, rdoSubtraction_CheckedChanged);
//
// rdoAddition
//
this->rdoAddition->CheckAlign = System::Drawing::ContentAlignment::MiddleRight;
this->rdoAddition->Location = System::Drawing::Point(16, 24);
this->rdoAddition->Name = S"rdoAddition";
this->rdoAddition->TabIndex = 0;
this->rdoAddition->Text = S"Addition";
this->rdoAddition->CheckedChanged += new System::EventHandler(this, rdoAddition_CheckedChanged);
//
// Form1
//
this->AutoScaleBaseSize = System::Drawing::Size(5, 13);
this->ClientSize = System::Drawing::Size(360, 174);
this->Controls->Add(this->grpValues);
this->Controls->Add(this->grpOperations);
this->Name = S"Form1";
this->Text = S"Form1";
this->grpValues->ResumeLayout(false);
this->grpOperations->ResumeLayout(false);
this->ResumeLayout(false);
}
private: System::Void rdoAddition_CheckedChanged(System::Object * sender, System::EventArgs * e)
{
double Number1, Number2, Result;
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber1->Text) )
Number1 = 0.00;
else
Number1 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber1->Text);
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber2->Text) )
Number2 = 0.00;
else
Number2 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber2->Text);
Result = Number1 + Number2;
this->txtResult->Text = Result.ToString(S"F");
}
private: System::Void rdoSubtraction_CheckedChanged(System::Object * sender, System::EventArgs * e)
{
double Number1, Number2, Result;
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber1->Text) )
Number1 = 0.00;
else
Number1 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber1->Text);
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber2->Text) )
Number2 = 0.00;
else
Number2 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber2->Text);
Result = Number1 - Number2;
this->txtResult->Text = Result.ToString(S"F");
}
private: System::Void rdoMultiplication_CheckedChanged(System::Object * sender, System::EventArgs * e)
{
double Number1, Number2, Result;
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber1->Text) )
Number1 = 0.00;
else
Number1 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber1->Text);
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber2->Text) )
Number2 = 0.00;
else
Number2 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber2->Text);
Result = Number1 * Number2;
this->txtResult->Text = Result.ToString();
}
private: System::Void rdoDivision_CheckedChanged(System::Object * sender, System::EventArgs * e)
{
double Number1, Number2, Result;
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber1->Text) )
Number1 = 0.00;
else
Number1 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber1->Text);
if( !Microsoft::VisualBasic::Information::IsNumeric(this->txtNumber2->Text) )
Number2 = 0.00;
else
Number2 = Microsoft::VisualBasic::Conversion::Val(this->txtNumber2->Text);
Result = Number1 / Number2;
this->txtResult->Text = Result.ToString();
}
};
}