The dialog box on this exercise displays three
progress bars: one holds the value of the current hour, another holds the
value oVisual C# Examples - Progressive Clockf the minutes in the current hour, the last displays the seconds of
the current minute. We also use a label on the right side of each progress
bar to display its corresponding value.
To start this application, you can use a form. Then add the necessary controls to it as we will design
shortly. Although the hour holds 24 values while the minutes and the
seconds hold 60 values each, we will use the same dimensions (especially
the same width) for all progress controls. Because a progress control has
no mechanism or message to trigger the change of its position, we will use
a timer to handle such a message.
Practical Learning: Starting the Exercise |
|
- Start a new Visual C# project as a Windows Forms Application
- Name it ProgressClock
- Design the form as follows:
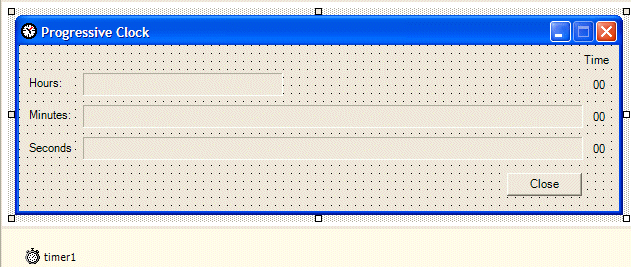 |
Control |
Name |
Text |
Other Properties |
Label |
|
Time |
|
Label |
|
Hours: |
|
ProgressBar |
pgrHours |
|
Anchor: Top, Left, Right
Maximum: 23
Step: 1 |
Label |
lblHours |
00 |
|
Label |
|
Minute: |
|
ProgressBar |
pgrMinutes |
|
Anchor: Top, Right
Maximum: 59
Step: 1 |
Label |
lblMinutes |
00 |
Anchor: Top, Right |
Label |
|
Second: |
|
ProgressBar |
pgrSeconds |
|
Anchor: Top, Right
Maximum: 59
Step: 1 |
Label |
lblSeconds |
00 |
Anchor: Top, Right |
Button |
btnClose |
Close |
|
Timer |
|
|
Enabled: True
Interval: 20 |
|
- Double-click the Timer to generate its Tick() event and implement it as follows:
private void timer1_Tick(object sender, System.EventArgs e)
{
DateTime curTime = DateTime.Now;
int H = curTime.Hour;
int M = curTime.Minute;
int S = curTime.Second;
pgrHours.Value = H;
pgrMinutes.Value = M;
pgrSeconds.Value = S;
lblHours.Text = H.ToString();
lblMinutes.Text = M.ToString();
lblSeconds.Text = S.ToString();
}
|
- Return to the form and click an unoccupied area of its body
- In the Properties window, click the Events button and generate the
Resize event of the form
- Implement it as follows:
private void Form1_Resize(object sender, System.EventArgs e)
{
this.pgrHours.Width = 2 * this.pgrMinutes.Width / 5;
}
|
- On the form, double-click the Close button and implement it as
follows:
private void btnClose_Click(object sender, System.EventArgs e)
{
Close();
}
|
- Test the application
- After using it, close it and return to your programming environment.
|
|