 |
GDI+ Examples: Collision Detection II |
|
|
This is another implementation of
collision detection. This one uses a Windows
control, namely a picture box, to represent the object that is moving.
|
Application:
Introducing the Tree View Control
|
|
- Start Microsoft Visual Studio or Microsoft Visual C# Express
- To create an application, on the main menu, click File -> New Project...
- Set the project type to Windows Form Application
- Change the name to CollisionDetection2
- Click OK
- In the Solution Explorer, right-click Form1.cs and click Rename
- Type CollisionDetection.cs and press Enter
- Design the form as follows:

|
Control |
(Name) |
BackColor |
BorderStyle |
Enabled |
Interval |
PictureBox |
 |
pbxGreen |
192, 255, 192 |
Fixed3D |
|
|
PictureBox |
 |
pbxBrown |
SandyBrown |
Fixed3D |
|
|
Timer |
 |
tmrMover |
|
|
True |
2 |
|
- Double-click the middle of the form to generate its Load event
- Change the file as follows:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace CollisionDetection2
{
public partial class CollisionDetection : Form
{
static int xGreen;
static int xBrown;
static int yGreen;
static int yBrown;
static bool IsMovingDownGreen;
static bool IsMovingDownBrown;
static bool IsMovingRightGreen;
static bool IsMovingRightBrown;
public CollisionDetection()
{
InitializeComponent();
}
private void CollisionDetection_Load(object sender, EventArgs e)
{
xGreen = 10;
yGreen = 10;
xBrown = 1100;
yBrown = 500;
IsMovingDownGreen = false;
IsMovingDownBrown = false;
IsMovingRightGreen = false;
IsMovingRightBrown = false;
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.DoubleBuffer, true);
}
}
}
- Double-click the timer
- Implement the event as follows:
private void tmrMover_Tick(object sender, EventArgs e)
{
// If the status of the origin indicates that it is moving right,
// then increase the horizontal axis
if (IsMovingRightGreen == true)
xGreen++;
else // If it's moving left, then decrease the horizontal movement
xGreen--;
// If the status of the origin indicates that it is moving down,
// then increase the vertical axis
if (IsMovingDownGreen == true)
yGreen++;
else // Otherwise, decrease it
yGreen--;
// Collision: if the axis hits the right side of the screen,
// then set the horizontal moving status to "Right", which will be used
// by the above code
if ((xGreen + pbxGreen.Width) >= ClientSize.Width)
IsMovingRightGreen = false;
if ((xGreen + pbxGreen.Width) <= 100)
IsMovingRightGreen = true; ;
if ((yGreen + pbxGreen.Height) >= ClientSize.Height)
IsMovingDownGreen = false;
if ((yGreen + pbxGreen.Height) <= 50)
IsMovingDownGreen = true;
BackColor = Color.Black;
pbxGreen.Location = new Point(xGreen, yGreen);
// -----------------------------------------------------------
if (IsMovingRightBrown == true)
xBrown += 2;
else // If it's moving left, then decrease the horizontal movement
xBrown--;
// If the status of the origin indicates that it is moving down,
// then increase the vertical axis
if (IsMovingDownBrown == true)
yBrown += 2;
else // Otherwise, decrease it
yBrown--;
// Collision: if the axis hits the right side of the screen,
// then set the horizontal moving status to "Right", which will be used
// by the above code
if ((xBrown + pbxBrown.Width) >= ClientSize.Width)
IsMovingRightBrown = false;
if ((xBrown + pbxBrown.Width) <= 100)
IsMovingRightBrown = true; ;
if ((yBrown + pbxBrown.Height) >= ClientSize.Height)
IsMovingDownBrown = false;
if ((yBrown + pbxBrown.Height) <= 50)
IsMovingDownBrown = true;
pbxBrown.Location = new Point(xBrown, yBrown);
if (pbxBrown.Bounds.IntersectsWith(pbxGreen.Bounds))
{
tmrMover.Enabled = false;
/* Random rndNumber = new Random();
xGreen = rndNumber.Next(Width);
xBrown = rndNumber.Next(Width);
yGreen = rndNumber.Next(Height);
yBrown = rndNumber.Next(Height);*/
}
}
- To execute, press F5


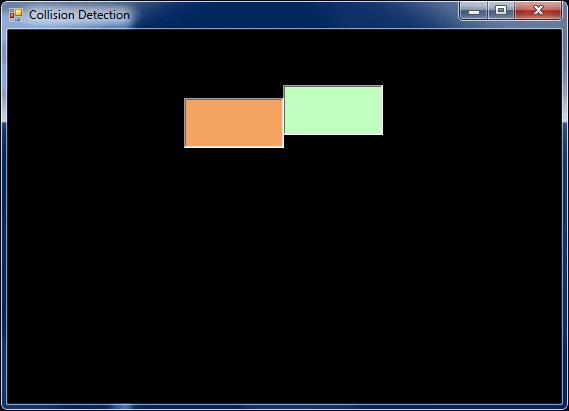
- Close the form
|
|