Some times if you are asked to create an application
for a store that sells a specific number of items based on a fixed list,
you may request the list of items so you can create its elements in the
application. Here is an example:
For such an application, when the list of items
changes, you may have to modify the application, recompile, and
redistribute (or re-install it). One solution to this problem would
consist of providing placeholders for an employee to fill out and process
the order with them. Another problem that can be common with this type of
application is that, while the form may be crowded with too many objects,
most of the time, some items are rarely ordered.
In this exercise, to make this type of application a
little more flexible, we will use a few combo boxes that allow the user to
simply select the items that are valid for the order.
Prerequisites:
Practical Learning: Introducing Buttons
|
|
- Start a new MFC Application named GCS1
- Create it as a Single Document
- Set the File Extension to gcs and the Filter Name to
Georgetown Cleaning Services Files (*.gcs)
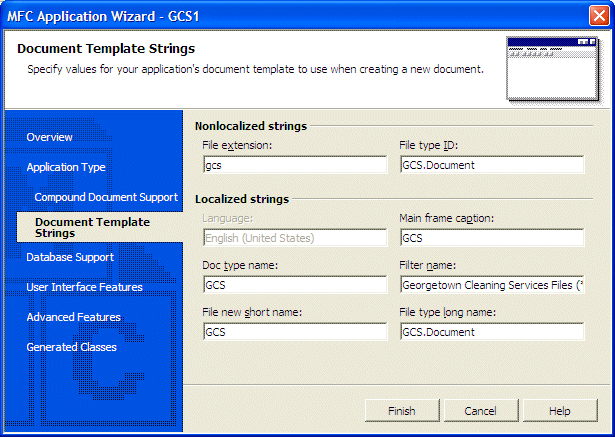
- Uncheck the Maximize Box check box
- Set the Base Class to CFormView and click Finish
- In the Resource View, expand GCS1, followed by GDC1.rc and the Dialog
folder
- Double-click IDD_GCS1_Form and design it as follows:
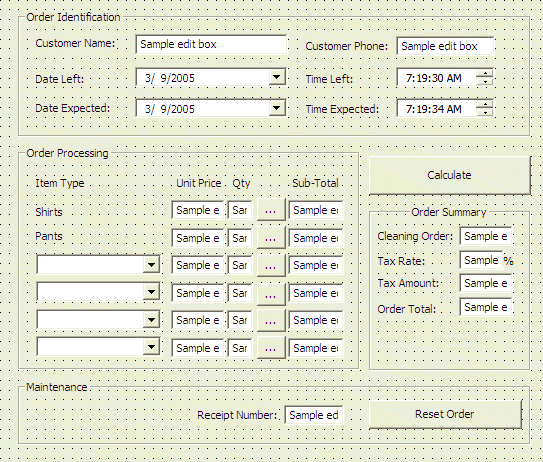 |
Control |
ID |
Caption |
Additional Properties |
Group Box |
 |
|
Order Identification |
|
Static Text |
 |
|
Customer Name: |
|
Edit Control |
 |
IDC_CUSTOMERNAME |
|
|
Static Text |
 |
|
Customer Phone: |
|
Edit Control |
 |
IDC_CUSTOMERPHONE |
|
|
Static Text |
 |
|
Date Left: |
|
Date Time Picker |
 |
IDC_DATELEFT |
|
|
Static Text |
 |
|
Time Left: |
|
Date Time Picker |
 |
IDC_TIMELEFT |
|
|
Static Text |
 |
|
Date Expected: |
|
Date Time Picker |
 |
IDC_DATEEXPECTED |
|
|
Static Text |
 |
|
Time Expected: |
|
Date Time Picker |
 |
IDC_TIMEEXPECTED |
|
|
Group Box |
 |
|
Order Processing |
|
Static Text |
 |
|
Item Type |
|
Static Text |
 |
|
Unit Price |
|
Static Text |
 |
|
Qty |
|
Static Text |
 |
|
Sub Total |
|
Static Text |
 |
|
Shirts |
|
Edit Control |
 |
IDC_SHIRTS_UNITPRICE |
|
Align Text: Right |
Edit Control |
 |
IDC_SHIRTS_QTY |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC_SHIRTS |
... |
|
Edit Control |
 |
IDC_SHIRTS_SUBTOTAL |
|
Align Text: Right |
Static Text |
 |
|
Pants |
|
Edit Control |
 |
IDC_PANTS_UNITPRICE |
|
Align Text: Right |
Edit Control |
 |
IDC_PANTS_QTY |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC_PANTS |
... |
|
Edit Control |
 |
IDC_PANTS_SUBTOTAL |
|
Align Text: Right |
ComboBox |
 |
IDC_ITEM1 |
|
Data: None;Women Suit;Dress;Regular Skirt;Skirt With Hook;Men 's Suit 2Pc;Men 's Suit 3Pc;Sweaters;Silk Shirt;Tie;Coat;Jacket;Swede; |
Edit Control |
 |
IDC_UNITPRICE1 |
|
Align Text: Right |
Edit Control |
 |
IDC_QUANTITY1 |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC1 |
... |
|
Edit Control |
 |
IDC_SUBTOTAL1 |
|
Align Text: Right |
ComboBox |
 |
IDC_ITEM2 |
|
Data: None;Women Suit;Dress;Regular Skirt;Skirt With Hook;Men 's Suit 2Pc;Men 's Suit 3Pc;Sweaters;Silk
Shirt;Tie;Coat;Jacket;Swede; |
Edit Control |
 |
IDC_UNITPRICE2 |
|
Align Text: Right |
Edit Control |
 |
IDC_QUANTITY2 |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC2 |
|
|
Edit Control |
 |
IDC_SUBTOTAL2 |
|
Align Text: Right |
ComboBox |
 |
IDC_ITEM3 |
|
Data: None;Women Suit;Dress;Regular Skirt;Skirt With Hook;Men 's Suit 2Pc;Men 's Suit 3Pc;Sweaters;Silk
Shirt;Tie;Coat;Jacket;Swede; |
Edit Control |
 |
IDC_UNITPRICE3 |
|
Align Text: Right |
Edit Control |
 |
IDC_QUANTITY3 |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC3 |
|
|
Edit Control |
 |
IDC_SUBTOTAL3 |
|
Align Text: Right |
ComboBox |
 |
IDC_ITEM4 |
|
Data: None;Women Suit;Dress;Regular Skirt;Skirt With Hook;Men 's Suit 2Pc;Men 's Suit 3Pc;Sweaters;Silk
Shirt;Tie;Coat;Jacket;Swede; |
Edit Control |
 |
IDC_UNITPRICE4 |
|
Align Text: Right |
Edit Control |
 |
IDC_QUANTITY4 |
|
Align Text: Right
Number: True |
Button |
 |
IDC_CALC4 |
|
|
Edit Control |
 |
IDC_SUBTOTAL4 |
|
Align Text: Right |
Button |
 |
IDC_CALCULATE |
Calculate |
|
Group Box |
 |
|
Order Summary |
Horizontal Alignment: Center |
Static Text |
 |
|
Cleaning Order: |
|
Edit Control |
 |
IDC_CLEANINGORDER |
|
Align Text: Right |
Static Text |
 |
|
Tax Rate: |
|
Edit Control |
 |
IDC_TAXRATE |
|
Align Text: Right |
Static Text |
 |
|
% |
|
Static Text |
 |
|
Tax Amount: |
|
Edit Control |
 |
IDC_TAXAMOUNT |
|
Align Text: Right |
Static Text |
 |
|
Order Price: |
|
Edit Control |
 |
IDC_ORDERTOTAL |
|
Align Text: Right |
Group Box |
 |
|
Maintenance |
|
Static Text |
 |
|
Receipt Number: |
|
Edit Control |
 |
IDC_RECEIPTNBR |
|
|
Button |
 |
IDC_RESET |
Reset Order |
|
|
- If you are using MSVC 6, press Ctrl + W to access the ClassWizard.
If you are using MSVC .NET, right-click each control and click Add Variable
- Add the control variables as follows:
Identifier |
Value Variable |
Control Variable |
Type |
Name |
IDC_CUSTOMERNAME |
CString |
m_CustomerName |
|
IDC_CUSTOMERPHONE |
CString |
m_CustomerPhone |
|
IDC_DATELEFT |
|
|
m_DateLeft |
IDC_TIMELEFT |
|
|
m_TimeLeft |
IDC_DATEEXPECTED |
|
|
m_DateExpected |
IDC_TIMEEXPECTED |
|
|
m_TimeExpected |
IDC_SHIRTS_UNITPRICE |
double |
m_ShirtsUnitPrice |
|
IDC_SHIRTS_QTY |
int |
m_ShirtsQuantity |
|
IDC_SHIRTS_SUBTOTAL |
double |
m_ShirtsSubTotal |
|
IDC_PANTS_UNITPRICE |
double |
m_PantsUnitPrice |
|
IDC_PANTS_QTY |
int |
m_PantsQuantity |
|
IDC_PANTS_SUBTOTAL |
double |
m_PantsSubTotal |
|
IDC_ITEM1 |
|
|
m_Item1 |
IDC_UNITPRICE1 |
double |
m_UnitPrice1 |
|
IDC_QUANTITY1 |
int |
m_Quantity1 |
|
IDC_SUBTOTAL1 |
double |
m_SubTotal1 |
|
IDC_ITEM2 |
|
|
m_Item2 |
IDC_UNITPRICE2 |
double |
m_UnitPrice2 |
|
IDC_QUANTITY2 |
int |
m_Quantity2 |
|
IDC_SUBTOTAL2 |
double |
m_SubTotal2 |
|
IDC_ITEM3 |
|
|
m_Item3 |
IDC_UNITPRICE3 |
double |
m_UnitPrice3 |
|
IDC_QUANTITY3 |
int |
m_Quantity3 |
|
IDC_SUBTOTAL3 |
double |
m_SubTotal3 |
|
IDC_ITEM4 |
|
|
m_Item4 |
IDC_UNITPRICE4 |
double |
m_UnitPrice4 |
|
IDC_QUANTITY4 |
int |
m_Quantity4 |
|
IDC_SUBTOTAL4 |
double |
m_SubTotal4 |
|
IDC_CLEANINGORDER |
CString |
m_CleaningOrder |
|
IDC_TAXRATE |
double |
m_TaxRate |
|
IDC_TAXAMOUNT |
CString |
m_TaxAmount |
|
IDC_ORDERTOTAL |
CString |
m_OrderTotal |
|
IDC_RECEIPTNBR |
CString |
m_ReceiptNumber |
|
- Access the form view and double-click the Start New Cleaning Order button
- Implement its event as follows:
void CGCS1View::OnBnClickedReset()
{
// TODO: Add your control notification handler code here
UpdateData();
// Reset the form
m_CustomerName = "";
m_CustomerPhone = "";
CTime tmeCurrent = CTime::GetCurrentTime();
m_DateLeft.SetTime(&tmeCurrent);
m_TimeLeft.SetTime(&tmeCurrent);
m_DateExpected.SetTime(&tmeCurrent);
m_TimeExpected.SetTime(&tmeCurrent);
m_ShirtsUnitPrice = 0.95;
m_ShirtsQuantity = 0;
m_ShirtsSubTotal = 0.00;
m_PantsUnitPrice = 2.75;
m_PantsQuantity = 0;
m_PantsSubTotal = 0.00;
m_Item1.SetWindowText("None");
m_UnitPrice1 = 0.00;
m_Quantity1 = 0;
m_SubTotal1 = 0.00;
m_Item2.SetWindowText("None");
m_UnitPrice2 = 0.00;
m_Quantity2 = 0;
m_SubTotal2 = 0.00;
m_Item3.SetWindowText("None");
m_UnitPrice3 = 0.00;
m_Quantity3 = 0;
m_SubTotal3 = 0.00;
m_Item4.SetWindowText("None");
m_UnitPrice4 = 0;
m_Quantity4 = 0;
m_SubTotal4 = 0;
m_CleaningOrder = "0.00";
m_TaxRate = 5.75;
m_TaxAmount = "0.00";
m_OrderTotal = "0.00";
CTime tmeLeft;
this->m_TimeLeft.GetTime(tmeLeft);
CString strReceiptNumber = tmeLeft.Format("%H%M%S");
this->m_ReceiptNumber = strReceiptNumber;
UpdateData(FALSE);
}
|
- In the Class View, expand CGCS1View and double-click OnInitialUpdate
- To initialize the date pickers and other controls, make the following
changes:
void CGCS1View::OnInitialUpdate()
{
CFormView::OnInitialUpdate();
GetParentFrame()->RecalcLayout();
ResizeParentToFit();
this->m_DateLeft.SetFormat(_T("dddd dd MMM yyyy"));
this->m_DateExpected.SetFormat(_T("dddd dd MMM yyyy"));
UpdateData(FALSE);
OnBnClickedReset();
}
|
- In the Class View, expand the CMainFrame node and double-click
PreCreateWindow
- To customize the title of the application and set the size of the frame,
make the following changes:
// MainFrm.cpp : implementation of the CMainFrame class
//
#include "stdafx.h"
#include "GCS1.h"
#include "MainFrm.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CMainFrame
IMPLEMENT_DYNCREATE(CMainFrame, CFrameWnd)
BEGIN_MESSAGE_MAP(CMainFrame, CFrameWnd)
ON_WM_CREATE()
END_MESSAGE_MAP()
static UINT indicators[] =
{
ID_SEPARATOR, // status line indicator
ID_INDICATOR_CAPS,
ID_INDICATOR_NUM,
ID_INDICATOR_SCRL,
};
// CMainFrame construction/destruction
CMainFrame::CMainFrame()
{
// TODO: add member initialization code here
}
CMainFrame::~CMainFrame()
{
}
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
if (!m_wndToolBar.CreateEx(this, TBSTYLE_FLAT, WS_CHILD | WS_VISIBLE | CBRS_TOP
| CBRS_GRIPPER | CBRS_TOOLTIPS | CBRS_FLYBY | CBRS_SIZE_DYNAMIC) ||
!m_wndToolBar.LoadToolBar(IDR_MAINFRAME))
{
TRACE0("Failed to create toolbar\n");
return -1; // fail to create
}
m_wndToolBar.SetWindowText(_T("Standard Toolbar"));
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators)/sizeof(UINT)))
{
TRACE0("Failed to create status bar\n");
return -1; // fail to create
}
// TODO: Delete these three lines if you don't want the toolbar to be dockable
m_wndToolBar.EnableDocking(CBRS_ALIGN_ANY);
EnableDocking(CBRS_ALIGN_ANY);
DockControlBar(&m_wndToolBar);
this->CenterWindow();
this->SetWindowText(_T("Georgetown Cleaning Services - Customer Order"));
return 0;
}
BOOL CMainFrame::PreCreateWindow(CREATESTRUCT& cs)
{
if( !CFrameWnd::PreCreateWindow(cs) )
return FALSE;
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
cs.style = WS_OVERLAPPED | WS_CAPTION | FWS_ADDTOTITLE
| WS_THICKFRAME | WS_MINIMIZEBOX | WS_SYSMENU;
// The new width of the window's frame
cs.cx = 580;
// The new height of the window's frame
cs.cy = 620;
// Remove the Untitled thing
cs.style &= ~FWS_ADDTOTITLE;
return TRUE;
}
// CMainFrame diagnostics
#ifdef _DEBUG
void CMainFrame::AssertValid() const
{
CFrameWnd::AssertValid();
}
void CMainFrame::Dump(CDumpContext& dc) const
{
CFrameWnd::Dump(dc);
}
#endif //_DEBUG
// CMainFrame message handlers
|
- Execute the application to preview it

- Close the window and return to MSVC
|
|