 |
Structures |
|
Fundamentals of Structures
Introduction
A structure is a list of characteristics, as members, that describe an
object. A member of a structure can also perform one or more actions. A structure is like a class, but a
structure is made for relatively small objects.
Creating a Structure
To create a structure, use the same formula as a class
but with the Structure keyword. The basic formula to follow is:
[ Private | Public ] Structure structure-name
End Structure
If the structure is created in a webpage, you must include it
in a script section as seen for a class. The body of the structure starts after the Structure structure-name line and ends just above the End Structure line.
Like a class, a structure can have members as fields and/or properties.
Here is an example:
<script Language="VB" runat="server">
Public Structure Station
Public StationNumber As Integer
Public StationName As Integer
Public Line As String
Public DailyParking As Boolean
Public MeteredParking As Boolean
End Structure
</script>
A structure can also have methods and constructors but it
cannot have a default constructor (a constructor without parameters). Here is an
example of a structure with a constructor:
<script Language="VB" runat="server">
Public Structure Station
Public StationNumber As Integer
Public StationName As Integer
Public Line As String
Public DailyParking As Boolean
Public MeteredParking As Boolean
Public Sub New(ByVal number As Integer, ByVal name As Integer, ByVal line As String,
ByVal longTermParting As Boolean, ByVal shortTermParting As Boolean)
Me.StationNumber = number
Me.StationName = name
Me.Line = line
Me.DailyParking = longTermParting
Me.MeteredParking = shortTermParting
End Sub
End Structure
</script>
Using a Structure
A structure is primarily used like a class. To create an
object based on a structure, declare a variable for it. You do this as if the variable were of a primitive
type. By default, the memory allocated for a variable of a structure type is in
the stack. That is, you don't have to allocate memory for it using the New
operator. Still, if you want to dynamically allocate memory for the
variable, you can initialize it using the New operator.
Like a class, a structure can be passed as argument to a
procedure. An object of a structure type can be returned from a function. A
structure can be used to create a collection.
After declaring a variable for a structure, to access the
members of the structure, you can use the period operator. Here are examples of
using a structure:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Public Structure Station
Public StationNumber As Integer
Public StationName As String
Public Line As String
Public DailyParking As Boolean
Public MeteredParking As Boolean
Public Sub New(ByVal number As Integer,
ByVal name As String,
ByVal line As String,
ByVal longTermParting As Boolean,
ByVal shortTermParting As Boolean)
Me.StationNumber = number
Me.StationName = name
Me.Line = line
Me.DailyParking = longTermParting
Me.MeteredParking = shortTermParting
End Sub
End Structure
Public Function CreateRedLineStations() As Collection
Dim metro As Station
Dim stations As New Collection
metro = New Station(2014, "Shady Grove", "Red", True, True)
stations.Add(metro)
metro = New Station(1660, "Rockville", "Red", True, True)
stations.Add(metro)
metro = New Station(9722, "Twinbrook", "Red", True, True)
stations.Add(metro)
stations.Add(New Station(2048, "White Flint", "Red", True, True))
stations.Add(New Station(8294, "Grosvenor-Strathmore", "Red", True, True))
stations.Add(New Station(2864, "Medical Center", "Red", False, False))
stations.Add(New Station(2814, "Bethesda", "Red", False, False))
stations.Add(New Station(9204, "Friendship Heights", "Red", False, False))
stations.Add(New Station(8648, "Tenleytown-AU", "Red", False, True))
stations.Add(New Station(2522, "Van Ness - UDC", "Red", False, False))
stations.Add(New Station(9741, "Cleveland Park", "Red", False, False))
stations.Add(New Station(9279, "Dupont Circle", "Red", False, False))
stations.Add(New Station(7974, "Farragut North", "Red", False, False))
stations.Add(New Station(9294, "Metro Center", "Red", False, False))
stations.Add(New Station(1359, "Gallery Pl - Chinatown", "Red", False, False))
stations.Add(New Station(8200, "Judiciary Square", "Red", False, False))
stations.Add(New Station(1802, "Union Station", "Red", False, False))
stations.Add(New Station(2014, "NoMa-Gallaudet U", "Red", False, False))
stations.Add(New Station(8802, "Glenmont", "Red", True, True))
stations.Add(New Station(1116, "Rhode Island Ave-Brentwood", "Red", True, True))
stations.Add(New Station(3948, "Brookland-CUA", "Red", False, True))
stations.Add(New Station(9794, "Fort Totten", "Red", True, True))
stations.Add(New Station(8270, "Takoma", "Red", False, True))
stations.Add(New Station(9274, "Silver Spring", "Red", False, False))
stations.Add(New Station(1417, "Forest Glen", "Red", True, True))
stations.Add(New Station(9737, "Wheaton", "Red", True, False))
Return stations
End Function
Sub btnFindStationByNumberClick(ByVal sender As Object, ByVal e As EventArgs)
Dim selection As Station
Dim stations = CreateRedLineStations()
If Not String.IsNullOrEmpty(txtStationNumber.Text) Then
For Each stt As Station In stations
If stt.StationNumber = txtStationNumber.Text Then
selection = stt
Exit For
End If
Next
lblStationByNumber.Text = selection.StationNumber
lblStationByName.Text = selection.StationName
lblStationByLine.Text = selection.Line
chkHasDailyParking.Checked = selection.DailyParking
chkHasMeteredParking.Checked = selection.MeteredParking
pnlRequest.Visible = False
pnlStationByNumber.Visible = True
End If
End Sub
Sub btnFindStationByNameClick(ByVal sender As Object, ByVal e As EventArgs)
Dim selection As Station
Dim stations = CreateRedLineStations()
If Not String.IsNullOrEmpty(txtStationName.Text) Then
For Each stt As Station In stations
If LCase(stt.StationName).Contains(LCase(txtStationName.Text)) Then
selection = stt
Exit For
End If
Next
lblStationByNumber.Text = selection.StationNumber
lblStationByName.Text = selection.StationName
lblStationByLine.Text = selection.Line
chkHasDailyParking.Checked = selection.DailyParking
chkHasMeteredParking.Checked = selection.MeteredParking
pnlRequest.Visible = False
pnlStationByNumber.Visible = True
End If
End Sub
</script>
<style>
#main-title
{
font-size: 1.18em;
font-weight: bold;
text-align: center;
font-family: Georgia, Garamond, 'Times New Roman', Times, serif;
}
.tables { width: 300px; }
#whole
{
margin: auto;
width: 305px;
}
</style>
<title>Metro System</title>
</head>
<body>
<form id="frmMetro" runat="server">
<div id="whole">
<p id="main-title">Metro System</p>
<asp:Panel id="pnlRequest" Visible="True" runat="server">
<table class="tables">
<tr>
<td>Station #:</td>
<td><asp:TextBox id="txtStationNumber" Width="65px" runat="server" />
<asp:Button id="btnFindStationByNumber" runat="server"
Text="Find" Width="85px" OnClick="btnFindStationByNumberClick" /></td>
</tr>
<tr>
<td>Station Name:</td>
<td><asp:TextBox id="txtStationName" runat="server" />
<asp:Button id="btnFindStationByName" runat="server"
Text="Find" Width="85px" OnClick="btnFindStationByNameClick" /></td>
</tr>
<tr>
<td>Line:</td>
<td><asp:TextBox id="txtLine" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td><asp:CheckBox id="chkDailyParking"
Text="Only stations with long term parking" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td><asp:CheckBox id="chkMeteredParking"
Text="Only stations with short term parking" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td>
</td>
</tr>
</table>
</asp:Panel>
<asp:Panel id="pnlStationByNumber" Visible="False" runat="server">
<table class="tables">
<tr>
<td>Station #:</td>
<td><asp:Label id="lblStationByNumber" Width="75px" runat="server" /></td>
</tr>
<tr>
<td>Station Name:</td>
<td><asp:Label id="lblStationByName" runat="server" Width="75px" /></td>
</tr>
<tr>
<td>Line:</td>
<td><asp:Label id="lblStationByLine" runat="server" Width="75px" /></td>
</tr>
<tr>
<td> </td>
<td><asp:CheckBox id="chkHasDailyParking"
Text="Has Daily Parking" runat="server" /></td>
</tr>
<tr>
<td> </td>
<td><asp:CheckBox id="chkHasMeteredParking"
Text="Has Metered Parking" runat="server" /></td>
</tr>
</table>
</asp:Panel>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
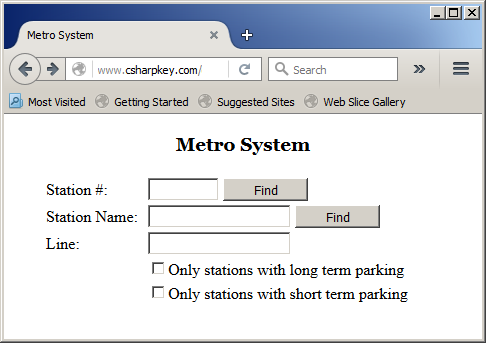
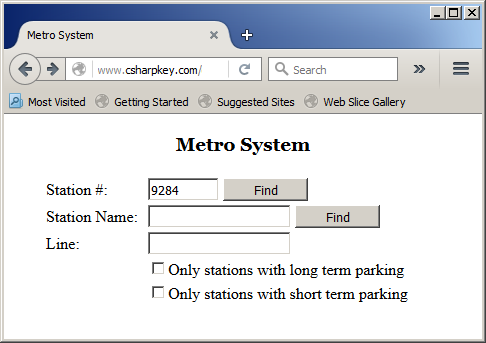
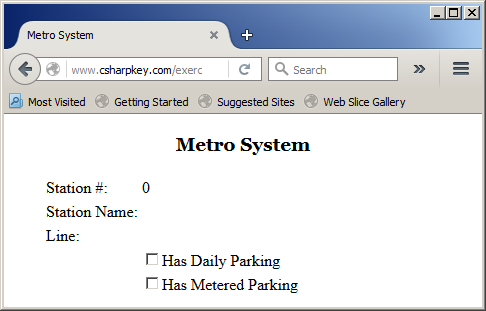
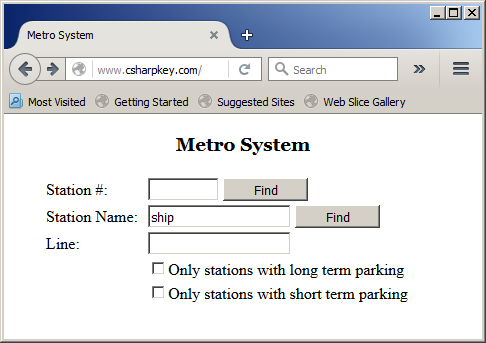
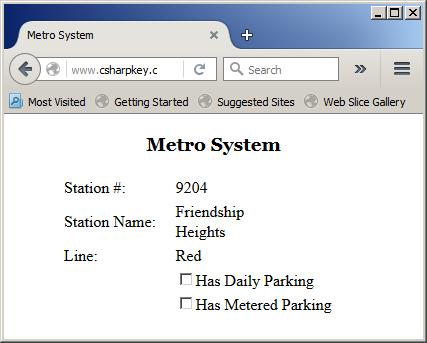
Although there are many similarities in the behaviors of classes and structures, you should use a structure when the object you are creating is meant to represent relatively small values.
Built-In Structures: The Numeric Types
Introduction
To support the numeric primitive data types (integers, decimal numbers, and their variants), the .NET Framework has a structure for each of them. To support the routine operations of regular variables, each structure that represents these primitive types is equipped with some member variables and methods.
Conversion to a String
To let you convert the value of a variable from its type to a string,
each structure of a primitive type is equipped with a method named
ToString. This method is overloaded with various versions. One of the
versions of this method takes no argument.
Another version of this method takes as argument a
string. This string holds an expression used to format the value of the variable
that called the method. The syntax of this method is:
Public Function ToString(format As String) As String
The argument is passed as a string and it can be one of the following characters:
Character |
Description |
c |
C |
Currency values |
d |
D |
Decimal numbers |
e |
E |
Scientific numeric display such as 1.45e5 |
f |
F |
Fixed decimal numbers |
d |
D |
General and most common type of numbers |
n |
N |
Natural numbers |
r |
R |
Roundtrip formatting |
s |
S |
Hexadecimal formatting |
p |
P |
Percentages |
Parsing a String
As you may know already:
- To convert a value to an integer, depending on the desired end result, you can call the CByte(), the CSByte(), the CShort(), the CUShort, the CInt(), the CUInt(), the CLng(), or the CULng() function
- To convert a value to a float-point number with single-precision, you can call the CSng() function
- To convert a value to a float-point number with double-precision, you can call the CDbl() function
- To convert a value to a decimal, you can call the CDec() function
- To convert any value to an Object type, call the CObj() function
To support these operations in the .NET Framework, each structure
of a primitive data type is equipped with a shared method named Parse. To use it, pass the value in the parenthesis of the method.
When you call the Parse() method, if a bad value is passed, the webpage may produce an error. To assist you with this type of problem, each
structure of a primitive type is equipped with a method named TryParse.
This method is overloaded with two versions that each returns a bool. One
of the versions of this method takes two arguments: a string and a variable
passed as reference.
The Minimum and Maximum Values of a Primitive Type
To help you find out the minimum value that a data type can
hold, each structure of a numeric type is equipped with a constant member
named MinValue. In the same way, the maximum value that a data type can
support is represented by a constant field named MaxValue. You can check
these minimum and maximum values as follow:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Numeric Primitive Types - Minimum and Maximum Values</title>
</head>
<body>
<h2>Numeric Primitive Types - Minimum and Maximum Values</h2>
<%
Response.Write("<table border=6><tr><td><b>VB Type</b></td><td><b>.NET Structure</b></td><td><b>Minimum</b></td><td><b>Maximum</b></td></tr>")
Response.Write("<tr><td>Byte</td><td>Byte</td><td>" & Byte.MinValue & "</td><td>" & Byte.MaxValue & "</td></tr>")
Response.Write("<tr><td>SByte</td><td>SByte</td><td>" & SByte.MinValue & "</td><td>" & SByte.MaxValue & "</td></tr>")
Response.Write("<tr><td>Short</td><td>Int16</td><td>" & Short.MinValue & "</td><td>" & Short.MaxValue & "</td></tr>")
Response.Write("<tr><td>UShort</td><td>UInt16</td><td>" & UShort.MinValue & "</td><td>" & UShort.MaxValue & "</td></tr>")
Response.Write("<tr><td>Integer</td><td>Int32</td><td>" & Integer.MinValue & "</td><td>" & Integer.MaxValue & "</td></tr>")
Response.Write("<tr><td>UInteger</td><td>UInt32</td><td>" & UInteger.MinValue & "</td><td>" & UInteger.MaxValue & "</td></tr>")
Response.Write("<tr><td>Long</td><td>Int64</td><td>" & Long.MinValue & "</td><td>" & Long.MaxValue & "</td></tr>")
Response.Write("<tr><td>ULong</td><td>UInt64</td><td>" & ULong.MinValue & "</td><td>" & ULong.MaxValue & "</td></tr>")
Response.Write("<tr><td>Single</td><td>Single</td><td>" & Single.MinValue & "</td><td>" & Single.MaxValue & "</td></tr>")
Response.Write("<tr><td>Double</td><td>Double</td><td>" & Double.MinValue & "</td><td>" & Double.MaxValue & "</td></tr>")
Response.Write("<tr><td>Decimal</td><td>Decimal</td><td>" & Decimal.MinValue & "</td><td>" & Decimal.MaxValue & "</td></tr>")
Response.Write("</table>")
%>
</body>
</html>
This would produce:
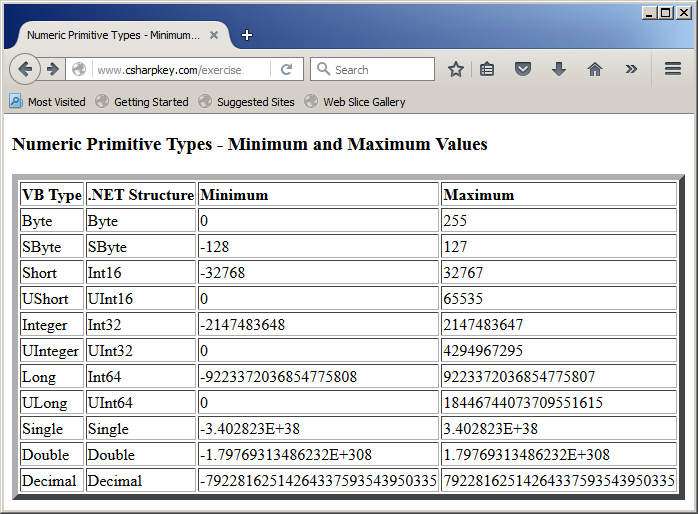
Value Comparisons
As you may know already, to compare the values of two variables, you can use a Boolean operator. To support these operations, each structure of a numeric data type is equipped with a method named CompareTo that is overloaded with two versions. One of the implementations of the CompareTo() method
compares a value or the value of a variable of the same type, with the variable
that calls the method. This method takes one argument that is the same type as
the variable that is calling it.
The CompareTo() method returns an integer. The end result is as follows:
- If the first value is greater than the second value, the
method returns 1
- If the first value is lower than the second value, the method
returns -1
- If both values are equal, the method returns 0
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<title>Exercise</title>
</head>
<body>
<%
Dim variable1 = 248
Dim variable2 = 72937
Dim result = variable1.CompareTo(variable2)
Response.Write(variable1 & " compared to " & variable2 & " produces " & result)
%>
</body>
</html>
This would produce:
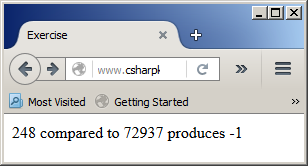
Another version of the CompareTo() method allows you
to compare the value of a variable with a variable whose type is not the same as
the variable that called it. The syntax of this version is:
public int CompareTo(object value);
Built-In Structures: The Case of Floating-Point Numbers
Introduction
Remember that the Visual Basic language supports
floating-point numbers in the Single, the Double, and the Decimal data types.
The .NET Framework provides structures of the same names. After declaring and initializing a variable, to check whether the variable is holding a value that is not a number, you can access its
NaN constant. To let you check this characteristic, the structures have
method named IsNaN.
Operations on Floating-Point Numbers
When it comes to the division of floating-point numbers on two constants, you can get a positive or a negative number. In highly precise calculations, you may have to deal with an approximate number whose exact value is not known. In the Double and the
Single structures, this constant is named Epsilon. For the single-precision type, the epsilon is equal to 1.445. For a double-precision type, the epsilon constant is equivalent to 4.94065645841247e-324. As you can see, this number is extremely low.
When dealing with real numbers, some operations produce very little or very large numbers. In algebra, the smallest number is called negative infinity. In the .NET Framework, the negative infinity is represented by a constant named
NegativeInfinity.
To find out if a variable holds a negative infinity value, you can call the
IsNegativeInfinity() method from the variable. The syntaxes of this method are:
Public Shared Function IsNegativeInfinity(f As Single) As Boolean
Public Shared Function IsNegativeInfinity(d As Double) As Boolean
On the other extreme, the possible largest number is named positive infinity. This constant is represented in the .NET Framework by the
PositiveInfinity value. To access this constant, type float or double, followed by the period, followed by the name of this constant. To find out if a variable's value is a positive infinity, you can call its
IsPositiveInfinity() method. The syntaxes of this method are:
Public Shared Function IsPositiveInfinity(f As Single) As Boolean
Public Shared Function IsPositiveInfinity(d As Double) As Boolean
To check whether the value of a variable is one of the infinities, you can call its
IsInfinity() method. The syntaxes of this method are:
pPublic Shared Function IsInfinity(f As Single) As Boolean
Public Shared Function IsInfinity(d As Double) As Boolean
The Boolean Type
Introduction
As seen in previous lessons, the Boolean data type is used to represent a value
considered as being True or False. The Visual Basic Boolean data type is represented in the .NET Framework by a structure named Boolean.
The True and the False Strings
The True value of a
Boolean variable is represented in the Boolean structure by the TrueString field and the False value is represented by the
FalseString member variable.
Parsing a Boolean Variable
You probably know already that, to convert a value to Boolean, you can pass the value to the CBool() function. To support this operation in the .NET Framework, the
Boolean structure is equipped with the Parse() shared method.
Built-In Structures: The Char Type
Introduction
As far as computers or operating systems are concerned,
every readable or non-readable symbol used in an application is a character.
All those symbols are considered objects of type Char. To support characters, the .NET Framework provides a structure named Char. The Visual Basic Char type is just a custom name of that structure.
Converting a Character to the Opposite Case
An alphabetic character can be converted from
one case to the other. To let you convert a character to lowercase, the Char structure is equipped with a method named ToLower. It is overloaded with two versions. One of them gets a character as argument and converts it. Its syntax is:
Public Shared Function ToLower(c As Char) As Char
On the other hande, to let you convert a character to uppercase, the Char structure is equipped with the ToUpper() method. It too comes in two versions. One of them receives a character as argument and convers it. That version uses the following syntax:
Public Shared Function ToUpper(c As Char) As Char
Categories of Characters
The Char structure (remember that everything reviewed here applies directly to the Visual Basic Char data type)
considers symbols into various categories:
Letters: An alphabetical letter is a readable character recognized by
a human language. To let you find out whether a character is a letter, the Char
structure is equipped with a shared method named IsLetter. It is
overloaded with two versions.
Digits: A digit is a symbol used in a number. It can be 0, 1, 2, 3,
4, 5, 6, 7, 8, or 9. To let you find out whether a character is a digit, the Char
structure is equipped with the IsDigit() shared method that is
overloaded with two versions. In the same way, the Char structure
provides various methods to test the category of characters being used. All
these methods are Shared and they are given in two versions. Each has a version
that takes one argument as a character. If the argument is the type sought, the
method returns true. Otherwise it returns false. The methods are:
Method |
Returns True if the argument is |
IsLetter(c As Char) |
A letter |
IsLower(c As Char) |
A lowercase letter |
IsUpper(c As Char) |
An uppercase letter |
IsDigit(c As Char) |
A digit |
IsNumber(c As Char) |
A digit or any other type of number |
IsLetterOrDigit(c As Char) |
A letter or a digit |
IsControl(c As Char) |
A control character (Ctrl, Shift, Enter, Del, Ins, etc) |
IsPunctuation(c As Char) |
A punctuation such as , . - ! ' " ( ) | # \ / % & * > @ < ? |
IsSymbol(c As Char) |
A symbol such as | + ? = ^ ? $ |
IsWhiteSpace(c As Char) |
An empty space such as created by pressing the SPACE bar |
IsSeparator(c As Char) |
An empty space or the end of a line |
IsHighSurrogate(c As Char) |
A Unicode character between U+D800 and U+DBFF |
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub txtNewPasswordTextChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim digits = 0
Dim symbols = 0
Dim lowercaseLetters = 0
Dim uppercaseLetters = 0
Dim length = Len(txtNewPassword.Text)
For i As Integer = 0 To length - 1
If Char.IsDigit(txtNewPassword.Text.Chars(i)) Then
digits += 1
End If
If Char.IsSymbol(txtNewPassword.Text.Chars(i)) Or Char.IsPunctuation(txtNewPassword.Text.Chars(i)) Then
symbols += 1
End If
Next
txtCharactersCount.Text = length
txtDigits.Text = str(digits)
txtSymbols.Text = str(symbols)
txtLowercaseLetters.Text = str(lowercaseLetters)
txtUppercaseLetters.Text = str(uppercaseLetters)
End Sub
Sub btnSubmitClick(ByVal sender As Object, ByVal e As EventArgs)
Dim original As String
Dim confirm As String
original = txtNewPassword.Text
confirm = txtConfirmPassword.Text
If original.Equals(confirm) = True Then
lblMessage.Text = "The passwords match."
Else
lblMessage.Text = "The passwords don't match."
End If
End Sub
</script>
<title>Password Validation</title>
</head>
<body>
<h2 style="text-align: center">Password Validation</h2>
<form id="frmValidation" runat="server">
<div align="center">
<table>
<tr>
<td>New Password:</td>
<td>
<asp:TextBox id="txtNewPassword" AutoPostBack="True"
OnTextChanged="txtNewPasswordTextChanged"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>Confirm Password:</td>
<td>
<asp:TextBox id="txtConfirmPassword" runat="server"></asp:TextBox>
</td>
</tr>
</table>
<table>
<tr>
<td></td>
<td>
<asp:TextBox id="txtCharactersCount" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Characters</td>
<td> </td>
<td> </td>
</tr>
<tr>
<td> </td>
<td>
<asp:TextBox id="txtUppercaseLetters" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Uppercase Letters</td>
<td>
<asp:TextBox id="txtLowercaseLetters" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Lowercase Letters</td>
</tr>
<tr>
<td> </td>
<td>
<asp:TextBox id="txtDigits" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Digits</td>
<td>
<asp:TextBox id="txtSymbols" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Symbols</td>
</tr>
</table>
<table>
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmit" runat="server" Text="Submit Password"
OnClick="btnSubmitClick" Width="130px" />
</td>
</tr>
</table>
<p style="text-align: center"><asp:Label id="lblMessage" runat="server"></asp:Label></p>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:

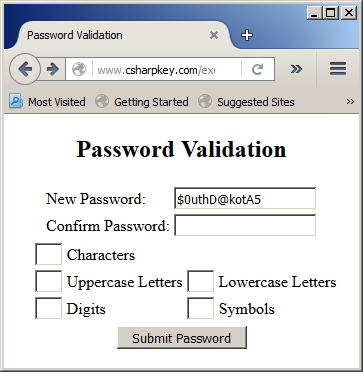
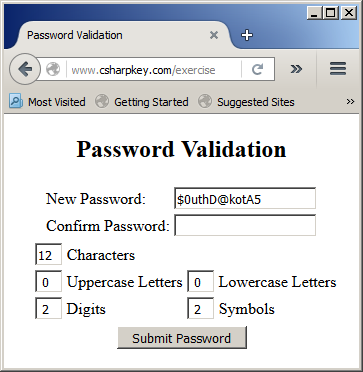
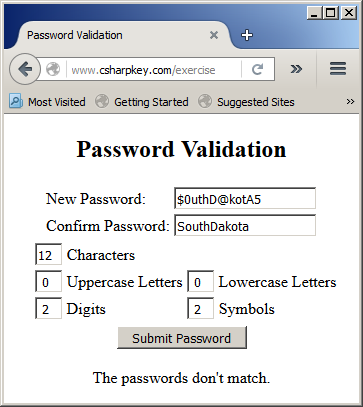
The Type of Character in a String
Alternatively, you can scan a
string to find out the type of character used in a certain position within the
string. To support this, the Char structure has various methods that are
second versions to the methods we saw for characters passed as arguments. Each
of these second versions takes two arguments. The first argument is passed as a string. The second
argument is the index where the character is positioned. If the character at
that position is the category sought, the method returns true. Otherwise it returns false.
The methods are:
Method |
Returns true if the character at index i within string s is |
IsLetter(s As String, index As Integer) |
A letter |
IsLower(s As String, index As Integer) |
A lowercase letter |
IsUpper(s As String, index As Integer) |
An uppercase letter |
IsDigit(s As String, index As Integer) |
A digit |
IsNumber(s As String, index As Integer) |
A digit or any other type of number |
IsLetterOrDigit(s As String, index As Integer) |
A letter or a digit |
IsControl(s As String, index As Integer) |
A control character (Ctrl, Shift, Enter, Del, Ins, etc) |
IsPunctuation(s As String, index As Integer) |
A punctuation such as , . - ! ' " ( ) | # \ / % & * > @ < ? |
IsSymbol(s As String, index As Integer) |
A symbol such as | + ? = ^ $ |
IsWhiteSpace(s As String, index As Integer) |
An empty space such as created by pressing the SPACE bar |
IsSeparator(s As String, index As Integer) |
An empty space or the end of a line |
IsHighSurrogate(c As Char) |
A Unicode character between U+D800 and U+DBFF |
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub txtNewPasswordTextChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim digits = 0
Dim symbols = 0
Dim lowercaseLetters = 0
Dim uppercaseLetters = 0
Dim length = Len(txtNewPassword.Text)
For i As Integer = 0 To length - 1
If Char.IsDigit(txtNewPassword.Text.Chars(i)) Then
digits += 1
End If
If Char.IsSymbol(txtNewPassword.Text.Chars(i)) Or Char.IsPunctuation(txtNewPassword.Text.Chars(i)) Then
symbols += 1
End If
If Char.IsLower(txtNewPassword.Text, i) Then
lowercaseLetters += 1
End If
If Char.IsUpper(txtNewPassword.Text, i) Then
uppercaseLetters += 1
End If
Next
txtCharactersCount.Text = length
txtDigits.Text = str(digits)
txtSymbols.Text = str(symbols)
txtLowercaseLetters.Text = str(lowercaseLetters)
txtUppercaseLetters.Text = str(uppercaseLetters)
End Sub
Sub btnSubmitClick(ByVal sender As Object, ByVal e As EventArgs)
Dim original As String
Dim confirm As String
original = txtNewPassword.Text
confirm = txtConfirmPassword.Text
If original.Equals(confirm) = True Then
lblMessage.Text = "The passwords match."
Else
lblMessage.Text = "The passwords don't match."
End If
End Sub
</script>
<title>Password Validation</title>
</head>
<body>
<h2 style="text-align: center">Password Validation</h2>
<form id="frmValidation" runat="server">
<div align="center">
<table>
<tr>
<td>New Password:</td>
<td>
<asp:TextBox id="txtNewPassword" AutoPostBack="True"
OnTextChanged="txtNewPasswordTextChanged"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>Confirm Password:</td>
<td>
<asp:TextBox id="txtConfirmPassword" runat="server"></asp:TextBox>
</td>
</tr>
</table>
<table>
<tr>
<td></td>
<td>
<asp:TextBox id="txtCharactersCount" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Characters</td>
<td> </td>
<td> </td>
</tr>
<tr>
<td> </td>
<td>
<asp:TextBox id="txtUppercaseLetters" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Uppercase Letters</td>
<td>
<asp:TextBox id="txtLowercaseLetters" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Lowercase Letters</td>
</tr>
<tr>
<td> </td>
<td>
<asp:TextBox id="txtDigits" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Digits</td>
<td>
<asp:TextBox id="txtSymbols" runat="server" Width="22px"></asp:TextBox>
</td>
<td>Symbols</td>
</tr>
</table>
<table>
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmit" runat="server" Text="Submit Password"
OnClick="btnSubmitClick" Width="130px" />
</td>
</tr>
</table>
<p style="text-align: center"><asp:Label id="lblMessage" runat="server"></asp:Label></p>
</div>
</form>
</body>
</html>
Here is an example of running the program:

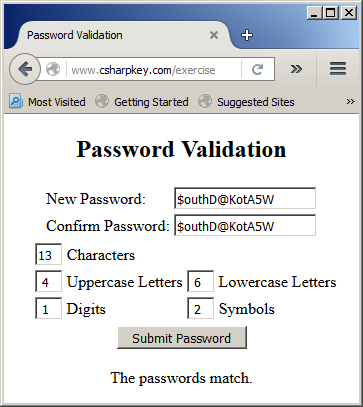
In the same way, you can create a class that is based on more than one interface but it can be based on only one class.
|