 |
Date and Time Values |
|
Introduction to Date Values
Introduction
A date is a non-spatial value that uses a combination of numbers, symbols, and strings (or names). Both the Visual Basic language and the .NET Framework provide tremendous support for date and time values.
The Date Data Type
To support dates and time values, the Visual Basic library
includes a shared (or static) class named DateAndTime. Because this is a
shared class, all of its members are directly accessible when you write VB code.
If a member you want to access has the same name as a class or an enumeration in
the .NET Framework, then you must qualify it.
In the Visual Basic language, the DateAndTime class is
represented by a data type named Date. To support dates and time values, the .NET
Framework provides a
structure named DateTime that is defined in the System namespace.
The Date data type and the DateTime structure
support dates and time starting from January 1st, 0001 at midnight
(0:00:00 AM) up to December 31st, 9999 at midnight minus 1 second (1!:59:59 PM).
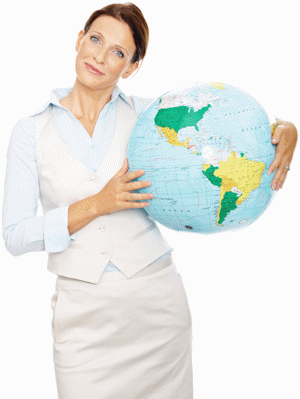
Creating a Date Value
To create a date or a time value, declare a variable using
either the Date data type or the DateTime structure. Here is an example:
<%
Dim DateHired As Date
%>
There are various ways you can initialize a date or
time variable. The numbers are represented with:
- Y (or y) for a digit in a year
- M (or m) for a digit in a month
- D (or d) for a digit in a day
- A separator: This can be - or /. Only one type of separator can be used in
a value
When initializing the variable, if you want to provide a constant value, you can include it
between # and #. The value can use the
following formula: MM-DD-YYYY: Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim DateHired As Date
DateHired = #08-22-2018#
Response.Write("Date Hired: " & DateHired)
%>
</body>
</html>
This would produce:
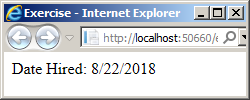
You can also use the date formula as MM/DD/YYYY.
As an alternative to initiate the variable, the Visual Basic
language provides a function named DateSerial. Its syntax is:
Public Shared Function DateSerial(Year As Integer,
Month As Integer,
Day As Integer) As Date
Here is an example:
<%
Dim DateHired = DateSerial(2018, 8, 22)
Response.Write("Date Hired: " & DateHired)
%>
As one more alternative, the DateTime structure is
equipped with the following contstructor:
Public Sub New(year As Integer, month As Integer, day As Integer)
Here is an example:
<%
Dim DateHired As Date
DateHired = New DateTime(2018, 8, 22)
Response.Write("Date Hired: " & DateHired)
%>
</body>
</html>
Converting a Value to Date or Time
To let you convert a value to date or time, the Visual Basic
language provides a function named CDate. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim show = "05-30-2018"
Dim depart = CDate("07/23/2017")
Dim d = CDate(show)
Response.Write("The TV show will premiere on " & d & ".")
Response.Write("<br>The cruise session will start on " & depart & ".")
%>
</body>
</html>
This would produce:
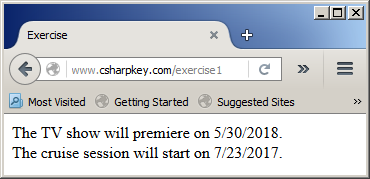
As an alternative, you can use a function named DateValue. Its
syntax is:
Public Shared Function DateValue(StringDate As String) As Date
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim show = "05-30-2018"
Dim depart = DateValue("07/23/2017")
Dim d = DateValue(show)
Response.Write("The TV show will premiere on " & d & ".")
Response.Write("<br>The cruise session will start on " & depart & ".")
%>
</body>
</html>
The Current System Date
To let you get the current date of the computer that a
visitor is using, use a property named Today:
Public Shared Property Today As Date
As an alternative, the Visual Basic language provides a
property named DateString:
Public Shared Property DateString As String
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim dteStart = DateSerial(2016, 8, 1)
txtStartDate.Text = dteStart
End Sub
Sub btnSubmitTimeSheetClick(ByVal sender As Object, ByVal e As EventArgs)
End Sub
</script>
<title>Employee Time Sheet</title>
</head>
<body>
<h3>Employee Time Sheet</h3>
<form id="frmTimeSheet" runat="server">
<table style="width: 500px">
<tr>
<td Width="100px"><b>Employee #:</b></td>
<td Width="100px">
<asp:TextBox id="txtEmployeeNumber" Width="70px"
runat="server" AutoPostBack="True"></asp:TextBox></td>
<td><asp:TextBox id="txtEmployeeName"
runat="server" Width="192px"></asp:TextBox></td>
</tr>
</table>
<table>
<tr>
<td Width="100px"><b>Start Date:</b></td>
<td><asp:TextBox id="txtStartDate" Width="70px"
runat="server"></asp:TextBox></td>
<td><b>End Date:</b></td>
<td><asp:TextBox id="txtEndDate" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table width="520">
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmitTimeSheet" Text="Submit Time Sheet"
OnClick="btnSubmitTimeSheetClick"
runat="server"></asp:Button></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of displaying the webpage:
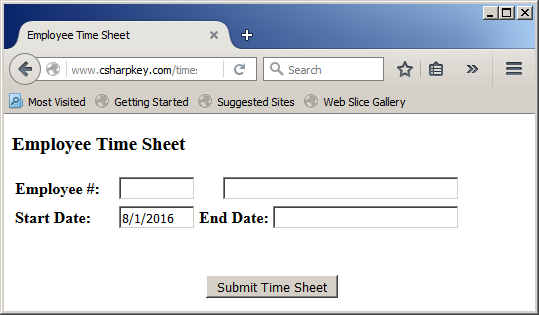
Formatting the Display of Dates
Introduction
To assist you in controlling how a date value should
display, the Visual Basic language provides a function named FormatDateTime. Its
syntax is:
Public Shared Function FormatDateTime(Expression As Date,
NamedFormat As DateFormat) As String
As we saw in previous lessons, the language also provides a
function named Format. As a reminder, its syntax is:
Public Shared Function Format(Expression As Object, Style As String) As String
In both cases, the first argument is the date value that will be formatted.
The second argument specifies the format.
The DateTime structure provides additional means.
The Short Date Format
To display the short date format, pass the
second argument of the FormatDateTime() function as DateFormat.ShortDate.
Here is an example:
<%
Dim happy As Date
happy = DateString
Response.Write("Happy Birthday: " & FormatDateTime(happy, DateFormat.ShortDate))
%>
As an alternative, pass the second argument of the Format() function as d (lowercase). Here is an example:
<%
Dim happy As Date
happy = DateString
Response.Write("Happy Birthday: " & Format(happy, "d"))
%>
The Long Date Format
To display the long date format, pass the
second argument of the FormatDateTime() function as DateFormat.LongDate.
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim dteStart = DateSerial(2016, 8, 1)
txtStartDate.Text = FormatDateTime(dteStart, DateFormat.ShortDate)
txtEndDate.Text = FormatDateTime(dteStart, DateFormat.LongDate)
End Sub
Sub btnSubmitTimeSheetClick(ByVal sender As Object, ByVal e As EventArgs)
End Sub
</script>
<title>Employee Time Sheet</title>
</head>
<body>
<h3>Employee Time Sheet</h3>
<form id="frmTimeSheet" runat="server">
<table style="width: 500px">
<tr>
<td Width="100px"><b>Employee #:</b></td>
<td Width="100px">
<asp:TextBox id="txtEmployeeNumber" Width="70px"
runat="server" AutoPostBack="True"></asp:TextBox></td>
<td><asp:TextBox id="txtEmployeeName"
runat="server" Width="230px"></asp:TextBox></td>
</tr>
</table>
<table>
<tr>
<td Width="100px"><b>Start Date:</b></td>
<td><asp:TextBox id="txtStartDate" Width="70px"
runat="server"></asp:TextBox></td>
<td><b>End Date:</b></td>
<td><asp:TextBox id="txtEndDate" Width="180px"
runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table width="520">
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmitTimeSheet" Text="Submit Time Sheet"
OnClick="btnSubmitTimeSheetClick"
runat="server"></asp:Button></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of displaying the webpage:
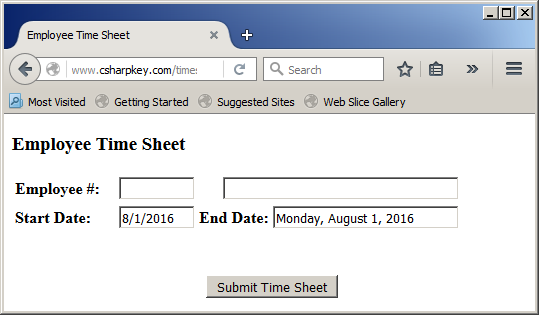
As an alternative, pass the second argument of the Format() function as D (uppercase). Here is an example:
<%
Dim happy As Date
happy = DateString
Response.Write("Happy Birthday: " & Format(happy, "D"))
%>
Here is an example of displaying the webpage:

The Format() function provides more options to
control has a date or one of its components (day, month, and/or year) appear.
The Parts of a Date
Introduction to the Part Name of a Date Value
The Visual Basic language provides various options to get the day,
the month, or the year of an existing date value. One of the functions used is named
DatePart. It is available in two versions whose syntaxes are:
Public Shared Function DatePart(Interval As DateInterval,
DateValue As Date,
FirstDayOfWeekValue As FirstDayOfWeek,
FirstWeekOfYearValue As FirstWeekOfYear) As Integer
Public Shared Function DatePart(Interval As String,
DateValue As Object,
DayOfWeek As FirstDayOfWeek,
WeekOfYear As FirstWeekOfYear) As Integer
The first argument, required, specifies the type of
value to get. The second argument, also required, is the date from which to get
the value.
The Format() function provides other ways to
get the parts of a date value.
The Day of a Date
To get the day in the month of a
date value, you can call a function named Day. Its syntax is:
Public Shared Function Day(DateValue As Date) As Integer
This function takes a date as argument and produces its
day. Here is an example:
<%
Dim dob As Date = #02/12/1975#
Dim d = Day(dob)
Response.Write("Day: " & d)
%>
As an alternative, the DateTime structure is equipped with a property named Day:
Public ReadOnly Property Day As Integer
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim current As Date = #11/24/2006#
Dim day = current.Day
Select Case day
Case 1, 21, 31
Response.Write("The date occurs on the " & day & "<sup>st</sup>")
Case 2, 22
Response.Write("The date occurs on the " & day & "<sup>nd</sup>")
Case 3, 23
Response.Write("The date occurs on the " & day & "<sup>rd</sup>")
Case Else
Response.Write("The date occurs on the " & day & "<sup>th</sup>")
End Select
%>
</body>
</html>
This would produce:
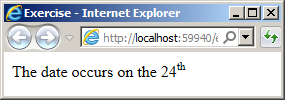
As one more alternative, you can call the DatePart()
function. Pass the second argument as either DateInterval.Day or "d". Here is an example:
<%
Dim dob As Date = #02/12/1975#
Dim d = DatePart(DateInterval.Day, dob)
Response.Write("Day: " & d)
d = DatePart("d", dob)
Response.Write("<br>Day: " & d)
%>
One more alternative is to cass the Format() function
and pass the second argument as "dd". Here is an example:
<%
Dim dob As Date = #02/12/1975#
Dim d = Format(dob, "dd")
Response.Write("<br>Day: " & d)
%>
The Month Name of a Date
To let you get the name of the month of a date, the
Visual Basic language provides a function named
Month. Its syntax is:
Public Shared Function MonthName(Month As Integer, Abbreviate As Boolean) As String
The first argument is required and it is just the numeric position
of a
month within a year. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Response.Write("Month: " & MonthName(8))
%>
</body>
</html>
This would produce:
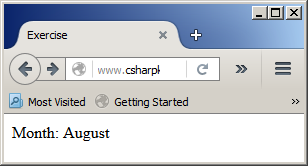
As you can see, if you provide only the first argument, the
full name of the month is produced. If you want a short name for the month, pass
the second argument as True.
The Numeric Month of a Date
To get the numeric position of a month in a date, you can
call a function named Month. Its syntax is:
Public Shared Function Month(DateValue As Date) As Integer
Here is an example:
<%
Dim vote = #02/20/2016#
Response.Write("Month: " & Month(vote))
%>
As you can see, the Month() function returns an
integer that is the position or index of the month. You can pass that return value
to the MonthName() function. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim vote = #02/20/2016#
Response.Write("Month: " & MonthName(Month(vote)))
%>
</body>
</html>
This would produce:
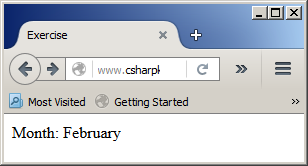
As an alternative, the DateTime structure is equipped with a property named Month:
Public ReadOnly Property Month As Integer
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim current As Date = #11/24/2006#
Dim day = current.Day
Select Case day
Case 1, 21, 31
Response.Write("The date occurs on the " & day & "<sup>st</sup>")
Case 2, 22
Response.Write("The date occurs on the " & day & "<sup>nd</sup>")
Case 3, 23
Response.Write("The date occurs on the " & day & "<sup>rd</sup>")
Case Else
Response.Write("The date occurs on the " & day & "<sup>th</sup>")
End Select
Response.Write(" in " & MonthName(current.Month))
%>
</body>
</html>
This would produce:
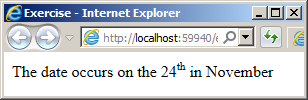
As one more alternative, you can call the DatePart() function.
Pass the first argument as either DateInterval.Month, "m", or "M" Here is an example:
<%
Dim vote = #02/20/2016#
Response.Write("Month: " & DatePart(DateInterval.Month, vote))
%>
As another alternative, you can call the Format function
and pass the second argument as "MM". Here is an example:
<%
Dim vote = #02/20/2016#
Response.Write("Month: " & Format(vote, "MM"))
%>
You can call the Day(), the Month(), the MonthName(), or the DatePart()
function as many times as you want to create a combination of values. Here are
examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim dteStart = DateSerial(2016, 8, 1)
Response.Write("Date Considered: " & Month(dteStart) & "-" & Day(dteStart))
Response.Write("<br>Important Date: " & DatePart(DateInterval.Month, dteStart)
& "-" & DatePart(DateInterval.Day, dteStart))
Response.Write("<br>To Note in Calendar: " & MonthName(Month(dteStart))
& ", " & DatePart(DateInterval.Day, dteStart))
%>
</body>
</html>
This would produce:
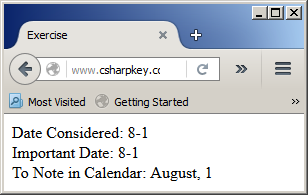
One of the
advantages of the Format() function is that it already has that
functionality built-in. This means that you can combine letters and symbols in
the second argument of that function. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim dteStart = DateSerial(2016, 8, 1)
txtStartDate.Text = FormatDateTime(dteStart, DateFormat.ShortDate)
txtEndDate.Text = FormatDateTime(dteStart, DateFormat.LongDate)
lblWeek1Monday.Text = Format(dteStart, "MM-dd")
End Sub
Sub btnSubmitTimeSheetClick(ByVal sender As Object, ByVal e As EventArgs)
End Sub
</script>
<style>
#container
{
margin: auto;
width: auto;
}
</style>
<title>Salary Estimation</title>
</head>
<body>
<div id="container">
<h3>Employee Time Sheet</h3>
<form id="frmTimeSheet" runat="server">
<table style="width: 500px">
<tr>
<td Width="100px"><b>Employee #:</b></td>
<td Width="100px">
<asp:TextBox id="txtEmployeeNumber" Width="70px"
runat="server" AutoPostBack="True"></asp:TextBox></td>
<td><asp:TextBox id="txtEmployeeName"
runat="server" Width="230px"></asp:TextBox></td>
</tr>
</table>
<table>
<tr>
<td Width="100px"><b>Start Date:</b></td>
<td><asp:TextBox id="txtStartDate" Width="70px"
runat="server"></asp:TextBox></td>
<td><b>End Date:</b></td>
<td><asp:TextBox id="txtEndDate" Width="180px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table>
<tr>
<td> </td>
<td><asp:Label id="lblWeek1Monday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Tuesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Wednesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Thursday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Friday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Saturday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Sunday" runat="server"></asp:Label></td>
</tr>
<tr>
<td><b>Week 1:</b></td>
<td><asp:TextBox id="txtWeek1Monday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Tuesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Wednesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Thursday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Friday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Saturday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Sunday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr>
<td> </td>
<td><asp:Label id="lblWeek2Monday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Tuesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Wednesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Thursday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Friday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Saturday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Sunday" runat="server"></asp:Label></td>
</tr>
<tr>
<td><b>Week 2:</b></td>
<td><asp:TextBox id="txtWeek2Monday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Tuesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Wednesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Thursday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Friday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Saturday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Sunday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table width="520">
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmitTimeSheet" Text="Submit Time Sheet"
OnClick="btnSubmitTimeSheetClick"
runat="server"></asp:Button></td>
</tr>
</table>
</form>
</div>
</body>
</html>
This would produce:
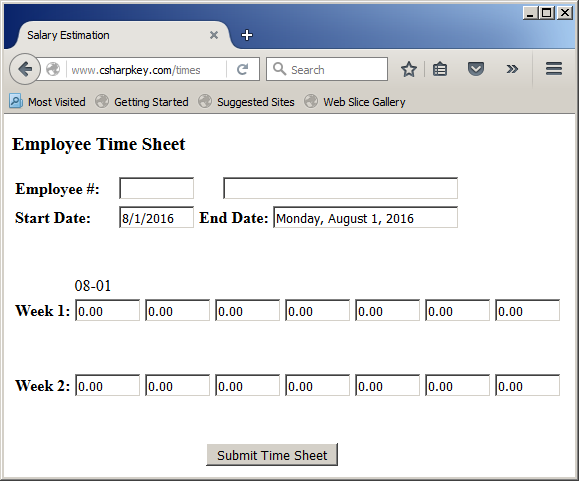
The Year of a Date
To let you get the year of a date, the Visual Basic
language provides a function named Year. Its syntax is:
Public Shared Function Year(DateValue As Date) As Integer
Here is an example:
<%
Dim dteStart = DateSerial(2016, 8, 1)
Response.Write("Year Considered: " & Year(dteStart))
%>
As an alternative, the DateTime structure is equipped with a
property named Year:
Public ReadOnly Property Year As Integer
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim current As Date = #11/24/2006#
Dim day = current.Day
Select Case day
Case 1, 21, 31
Response.Write("The date occurs on the " & day & "<sup>st</sup>")
Case 2, 22
Response.Write("The date occurs on the " & day & "<sup>nd</sup>")
Case 3, 23
Response.Write("The date occurs on the " & day & "<sup>rd</sup>")
Case Else
Response.Write("The date occurs on the " & day & "<sup>th</sup>")
End Select
Response.Write(" day of " & MonthName(current.Month))
Response.Write(" in " & current.Year)
%>
</body>
</html>
This would produce:
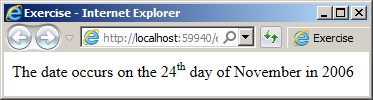
Another way to get the year value is to call the DatePart()
function. Pass the first argument as either DateInterval.Year or "yyyy". Here
is an example:
<%
Dim dteStart = DateSerial(2016, 8, 1)
Response.Write("Year Considered: " & DatePart(DateInterval.Year, dteStart))
%>
One more alternative is to call the Format() function and pass the
second argument as "yyy".
The Week Day
To let you get the index of a day of the week in which a
date occurs, the Visual Basic language provides the Weekday() function. Its
syntax is:
Public Shared Function Weekday(DateValue As Date,
DayOfWeek As FirstDayOfWeek) As Integer
The first argument is required and is a date that will be
considered. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim dteStart = DateSerial(2016, 8, 1)
Response.Write("On " & dteStart & ", the day of the week is " & Weekday(dteStart))
%>
</body>
</html>
This would produce:

The date of August 1st, 2016 is on a Monday. This means that
the date system of the Visual Basic language considers Sunday as the first day
of the week. If you want the week of your application to start on a different
day, pass the second argument, wihch is a member of the FirstDayOfWeek enumeration.
The members are: FirstDayOfWeek.System as 0, FirstDayOfWeek.Sunday as 1, FirstDayOfWeek.Monday as 2, FirstDayOfWeek.Tuesday as
3, FirstDayOfWeek.Wednesday as 4, FirstDayOfWeek.Thursday as 5, FirstDayOfWeek.Friday as
6, and FirstDayOfWeek.Saturday as 7.
To let you get the name of a day in a week, the Visual
Basic language provides a function named WeekdayName. Its syntax is:
Public Shared Function WeekdayName(Weekday As Integer,
Abbreviate As Boolean,
FirstDayOfWeekValue As FirstDayOfWeek) As String
The first argument is the only one required. It is the
numeric index of a day in a week. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Response.Write("Week Day Name: " & WeekDayName(1))
%>
</body>
</html>
This would produce:
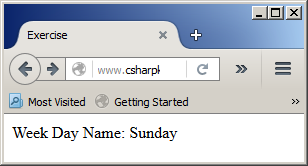
As you can see, by default, this function produces the full
name of a week day. If you want a short name, pass the second argument as True.
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Response.Write("Week Day Name: " & WeekDayName(4, True))
%>
</body>
</html>
This would produce:
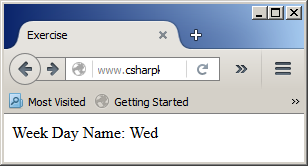
The third argument follows the same logic as the second argument of the Weekday() function.
Operations on Date Values
Adding a Number to a Date Value
Date addition consists of adding a number of days, months, or years, to a date value. To
support this operation, the Visual Basic language provides a function named
DateAdd. It is overloaded in two versions. The syntax of one of them is:
Public Shared Function DateAdd(Interval As String,
Number As Integer,
DateValue As Object) As Date
The other version uses the following syntax:
Public Shared Function DateAdd(Interval As DateInterval,
Number As Integer,
DateValue As Date) As Date
The first argument specifies the type of value that will
be added. It can be one of the following values:
Date Interval |
String |
Description |
DateInterval.Day |
d |
A number of days to add to the date value |
DateInterval.DayOfYear |
y |
A number of days of a year will be added to the date value |
DateInterval.Week |
w |
A number of weeks will be added to the date value |
DateInterval.Week |
ww |
A number of weeks will be added to the date value |
DateInterval.Month |
m |
A number of months will be added to the date value |
DateInterval.Year |
yyyy |
A number of years to add be added to the date value |
DateInterval.quarter |
q |
A number of quarters of a year will be added to the date
value |
Here is an example
that adds a number of years to a date value:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim dob As Date = #02/12/1975#
Dim happy = DateString
Dim passed = DateAdd("yyyy", 2, dob)
Response.Write("Happy Birthday: " & passed)
%>
</body>
</html>
This would produce:
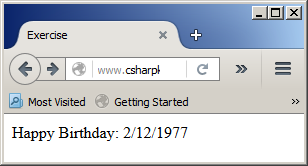
Once a number has been added to a date value, the function
produces a new date. You can format it any way you want. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim dteStart = DateSerial(2016, 8, 29)
txtStartDate.Text = FormatDateTime(dteStart, DateFormat.ShortDate)
txtEndDate.Text = FormatDateTime(DateAdd(DateInterval.Day, 13, dteStart), DateFormat.LongDate)
lblWeek1Monday.Text = Format(dteStart, "MM-dd")
lblWeek1Tuesday.Text = Format(DateAdd(DateInterval.Day, 1, dteStart), "MM-dd")
lblWeek1Wednesday.Text = Format(DateAdd(DateInterval.Day, 2, dteStart), "MM-dd")
lblWeek1Thursday.Text = Format(DateAdd(DateInterval.Day, 3, dteStart), "MM-dd")
lblWeek1Friday.Text = Format(DateAdd(DateInterval.Day, 4, dteStart), "MM-dd")
lblWeek1Saturday.Text = Format(DateAdd(DateInterval.Day, 5, dteStart), "MM-dd")
lblWeek1Sunday.Text = Format(DateAdd(DateInterval.Day, 6, dteStart), "MM-dd")
lblWeek2Monday.Text = Format(DateAdd(DateInterval.Day, 7, dteStart), "MM-dd")
lblWeek2Tuesday.Text = Format(DateAdd(DateInterval.Day, 8, dteStart), "MM-dd")
lblWeek2Wednesday.Text = Format(DateAdd(DateInterval.Day, 9, dteStart), "MM-dd")
lblWeek2Thursday.Text = Format(DateAdd(DateInterval.Day, 10, dteStart), "MM-dd")
lblWeek2Friday.Text = Format(DateAdd(DateInterval.Day, 11, dteStart), "MM-dd")
lblWeek2Saturday.Text = Format(DateAdd(DateInterval.Day, 12, dteStart), "MM-dd")
lblWeek2Sunday.Text = Format(DateAdd(DateInterval.Day, 13, dteStart), "MM-dd")
End Sub
Sub btnSubmitTimeSheetClick(ByVal sender As Object, ByVal e As EventArgs)
End Sub
</script>
<style>
#container
{
margin: auto;
width: auto;
}
</style>
<title>Salary Estimation</title>
</head>
<body>
<div id="container">
<h3>Employee Time Sheet</h3>
<form id="frmTimeSheet" runat="server">
<table style="width: 500px">
<tr>
<td Width="100px"><b>Employee #:</b></td>
<td Width="100px">
<asp:TextBox id="txtEmployeeNumber" Width="70px"
runat="server" AutoPostBack="True"></asp:TextBox></td>
<td><asp:TextBox id="txtEmployeeName"
runat="server" Width="230px"></asp:TextBox></td>
</tr>
</table>
<table>
<tr>
<td Width="100px"><b>Start Date:</b></td>
<td><asp:TextBox id="txtStartDate" Width="70px"
runat="server"></asp:TextBox></td>
<td><b>End Date:</b></td>
<td><asp:TextBox id="txtEndDate" Width="180px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table>
<tr>
<td> </td>
<td><asp:Label id="lblWeek1Monday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Tuesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Wednesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Thursday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Friday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Saturday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek1Sunday" runat="server"></asp:Label></td>
</tr>
<tr>
<td><b>Week 1:</b></td>
<td><asp:TextBox id="txtWeek1Monday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Tuesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Wednesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Thursday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Friday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Saturday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek1Sunday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr>
<td> </td>
<td><asp:Label id="lblWeek2Monday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Tuesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Wednesday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Thursday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Friday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Saturday" runat="server"></asp:Label></td>
<td><asp:Label id="lblWeek2Sunday" runat="server"></asp:Label></td>
</tr>
<tr>
<td><b>Week 2:</b></td>
<td><asp:TextBox id="txtWeek2Monday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Tuesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Wednesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Thursday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Friday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Saturday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWeek2Sunday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<br>
<table width="520">
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmitTimeSheet" Text="Submit Time Sheet"
OnClick="btnSubmitTimeSheetClick"
runat="server"></asp:Button></td>
</tr>
</table>
</form>
</div>
</body>
</html>
This would produce
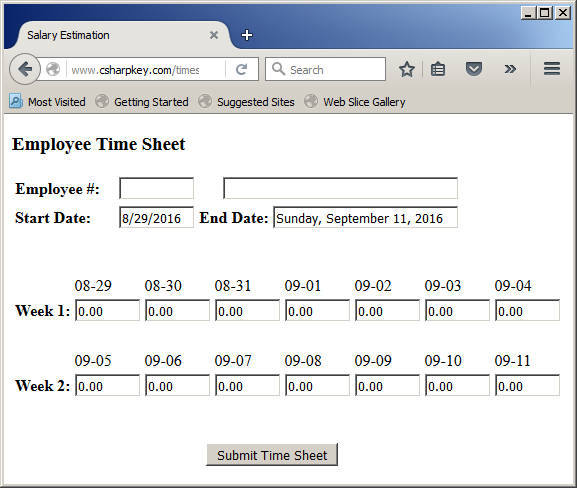
To let you add a number of years to a date, the DateTime structure
is equipped with a method named AddYears. Its syntax is:
Public Function AddYears(value As Integer) As Date
To let you add a number of months to a date, the DateTime structure
is equipped with a method named AddMonths. Its syntax is:
Public Function AddMonths(months As Integer) As Date
To let you add a number of days to a date, the DateTime structure
is equipped with a method named AddDays. Its syntax is:
Public Function AddDays(value As Double) As Date
To add a number of days, pass it as an integer. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim vacationFrom As Date
Dim vacationEnd As Date
vacationFrom = #12-19-2016#
vacationEnd = vacationFrom.AddDays(25)
Response.Write("The boss will be on vacation for 2 weeks from " &
FormatDateTime(vacationFrom, DateFormat.LongDate) &
" to " & FormatDateTime(vacationEnd, DateFormat.LongDate) & ".")
%>
</body>
</html>
This would produce
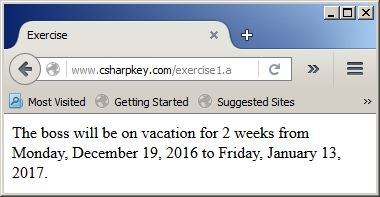
To add a number of days and a fraction of an additional day,
pass a decimal number.
The Difference Between Two Date Values
To let you find the difference between two dates, the
Visual Basic language provides a function named DateDiff. Its syntax is:
Public Shared Function DateDiff(Interval As DateInterval,
Date1 As Date,
Date2 As Date,
DayOfWeek As FirstDayOfWeek,
WeekOfYear As FirstWeekOfYear) As Long
The first argument specifies the type of value the
function must produce. This argument uses the same value as those of the
DateAdd() function. The second argument is the starting date. The third
argument is the end date. Those three arguments are the only ones required. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim dteStart = DateSerial(2006, 8, 15)
Dim dteEnd = DateSerial(2018, 12, 1)
Dim days = DateDiff(DateInterval.Day, dteStart, dteEnd)
Dim months = DateDiff(DateInterval.Month, dteStart, dteEnd)
Dim years = DateDiff(DateInterval.Year, dteStart, dteEnd)
Response.Write("It took " & days & " days, or " & months &
" months, or " & years & " years from " & dteStart & " to " & dteEnd & ".")
%>
</body>
</html>
This would produce
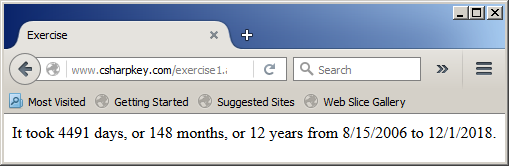
To subtract a number of years (or months, or days) to a date,
call the DateTime.AddYears() (DateTime.AddMonths() or DateTime.AddDays())
and pass a negative value. To subtract a number of and a portion, pass a
floating-point number.
Introduction to Time Values
Overview
To support time values, the Visual Basic language uses the
same Date data type used for dates. In the same way, the DateTime
structure of the .NET Framework is used for time values.
Creating a Time Value
You can use the Date data type to declare a
time-based variable and initialize it. The value of the variable can be
included between # and # and it can use one of the following formulas:
hh:mm
hh:mm:ss
hh:mm:ss[.fractional seconds]
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim meetingTime As Date
meetingTime = #09:35#
Response.Write("The meeting will start at " & meetingTime & ".")
%>
</body>
</html>
This would produce
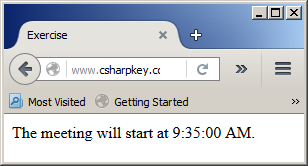
As an alternative to create a time value, the Visual Basic
language provides a function named TimeSerial. Its syntax is:
Public Shared Function TimeSerial(Hour As Integer,
Minute As Integer, Second As Integer) As Date
Here is an example of calling this function:
<%
Dim meetingTime As Date
meetingTime = TimeSerial(9, 35, 0)
Response.Write("The meeting will start at " & meetingTime & ".")
%>
The Current System Time
To let you get the current time of the computer that a
visitor is using, the Visual Basic language provides a property named TimeString:
Public Shared Property TimeString As String
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Response.Write("The current time is " & TimeString)
%>
</body>
</html>
As an option, the Visual Basic language provides a
property named TimeOfDay:
Public Shared Property TimeOfDay As Date
Here is an example of using it:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Response.Write("Time of Day: " & TimeOfDay)
%>
</body>
</html>
The Parts of a Time Value
The AM/PM Sides of a Time Value
As you may know, the hours of a time value use a 24-hour
range from 0 to 23 but divided in two sections of 12 hours each. To express
the first section, you can write the number between 0 and 11 as we have done
in the above two examples. To re-enforce the idea that the time occurs in
the morning, end the value with AM. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim late = #12:46 AM#
Dim occurring = #03:18 AM#
Response.Write("The late night TV show will end at " & late & ".")
Response.Write("<br>The event will occur in local time at " & occurring & ".")
%>
</body>
</html>
This would produce
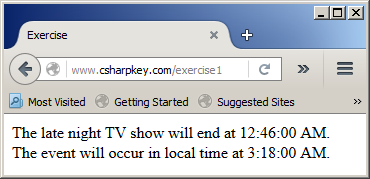
The last section, also referred to as
afternoon, can be expressed in two ways:
- If you are assigning a constant time value to a variable, you can express the hour value from 12, 1, 2, etc
up to 11; but then you must
end the value with PM. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim course = #12:35 PM#
Dim game = #03:25 PM#
Response.Write("The course will start at " & course & ".")
Response.Write("<br>The game will take place at " & game & ".")
%>
</body>
</html>
This would produce
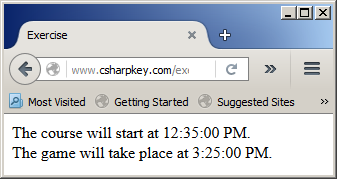
In the absence of the PM section, the time is considered to occur in the
morning
- You can use the military time in which the hour is expressed as a value
between 0 and 23 (0 to 11 in the morning and 12 to 23 in the
afternoon/evening) and you must omit PM. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim course = #10:25#
' This value may not work with some compilers
Dim game = #17:25#
Dim arrival = TimeSerial(19, 48, 0)
Response.Write("The course will start at " & course & ".")
Response.Write("<br>The game will take place at " & game & ".")
Response.Write("<br>The train will arrive at " & arrival & ".")
%>
</body>
</html>
This would produce
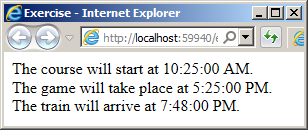
To let you extract the parts of a time value, the Visual Basic language provides
the the DatePart() function. The Format() function provides other
options. The language also includes functions to get the hours, the minutes, and the seconds
portions.
The Hour, the Minute, and the Second of a Time
To let you get the hours, the minutes, and the seconds
portions of a time value, the Visual Basic language provides functions named Hour, Minute, and Second.
Their syntaxes are:
Public Shared Function Hour(TimeValue As Date) As Integer
Public Shared Function Minute(TimeValue As Date) As Integer
Public Shared Function Second(TimeValue As Date) As Integer
Here are examples of calling them:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim occurrence As Date = #12:35:48 AM#
Response.Write("The event occurs at " & Hour(occurrence) & "." &
Minute(occurrence) & "." & Second(occurrence))
%>
</body>
</html>
This would produce:
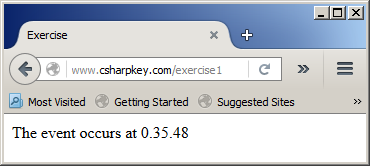
An alternative is to call the DatePart()
function. Pass the first argument as
- DateInterval.Hour or "h" for the hour
- DateInterval.Minute or "n" for the hour
- DateInterval.Second or "h" for the hour
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim occurrence As Date = #12:35:48 AM#
Response.Write("The event occurs at " &
DatePart(DateInterval.Hour, occurrence) & "." &
DatePart(DateInterval.Minute, occurrence) & "." &
DatePart(DateInterval.Second, occurrence))
%>
</body>
</html>
One more alternative is to cass the Format() function
and pass the second argument as "%h" for the hour, "%m" for the minute, or "%s" for the second. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim occurrence As Date = #10:35:48 AM#
Response.Write("The event occurs at " &
Format(occurrence, "%h") & "." &
Format(occurrence, "%m") & "." &
Format(occurrence, "%s"))
%>
</body>
</html>
Converting a Value to Time
To convert an appropriately-formatted value to time, you can
call the same CDate() function used for date values.
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim late As String = "12:35 AM"
Dim occurring As String = "#03:18 AM#"
Dim d = CDate(late)
Dim o = CDate(occurring)
Response.Write("The late night TV show will end at " & d & ".")
Response.Write("<br>The event occur in local time at " & o & ".")
%>
</body>
</html>
If the time is provided as a string, you can convert it
using a function named TimeValue. Its syntax is
Public Shared Function TimeValue(StringTime As String) As Date
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim late = "12:35 AM"
Dim occurring = TimeValue("03:18 AM")
Dim d = TimeValue(late)
Response.Write("The late night TV show will end at " & d & ".")
Response.Write("<br>The event occur in local time at " & occurring & ".")
%>
</body>
</html>
Formatting the Display of a Time Value
Introduction
To let you control how a time value should
appear, you can use the same FormatDateTime() used for date value. The Format() function
also is available with additional options. The DateTime structure provides
other options.
The Short Time Format
To display a time value in a short format, pass the
second argument of the FormatDateTime() function as DateFormat.ShortTime.
Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim late As String = "12:35 AM"
Dim occurring As String = "#03:18 AM#"
Dim d = CDate(late)
Dim o = CDate(occurring)
Response.Write("The late night TV show will end at " & FormatDateTime(d, DateFormat.ShortTime) & ".")
Response.Write("<br>The event occur in local time at " & FormatDateTime(o, DateFormat.ShortTime) & ".")
%>
</body>
</html>
This would produce
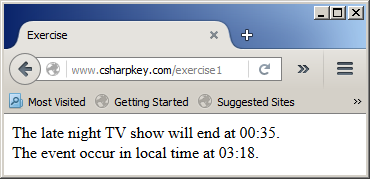
Indeed, a short time value uses the following format:
hh:mm AM/PM. To show that format, pass the second argument of the Format() function as
t (lowercase). Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim occurrence As Date = #10:35:48 AM#
Response.Write("The event occurs at " & Format(occurrence, "t"))
occurrence = #17:29#
Response.Write("<br>The event occurs at " & Format(occurrence, "t"))
%>
</body>
</html>
This would produce
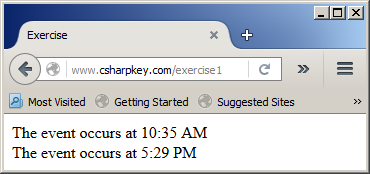
The Long Date Format
To display a time value in long format, pass the
second argument of the FormatDateTime() function as LongTime.
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim late As String = "12:35 AM"
Dim occurring As String = "#03:18 PM#"
Dim d = CDate(late)
Dim o = CDate(occurring)
Response.Write("The late night TV show will end at " &
FormatDateTime(d, DateFormat.LongTime) & ".")
Response.Write("<br>The event occur in local time at " &
FormatDateTime(o, DateFormat.LongTime) & ".")
%>
</body>
</html>
Here is an example of displaying the webpage:
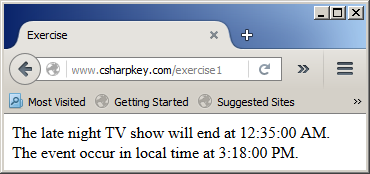
Introduction to Date and Time Values
Overview
The values of date and time and usually considered in
one unit. To declare a variable for such a value, you can use either the Date
data type or the DateTime structure.
If you declare a date/time variable, to initialize
it, include the value between # and #. The value can use one of the following formulas:
If you declare the variable using the DateTime
structure, you
can use the following constructor to initialize the variable:
Public Sub New(year As Integer,
month As Integer,
day As Integer,
hour As Integer,
minute As Integer,
second As Integer)
Here is an example:
<%
Dim dteStart As New DateTime(2018, 08, 14, 8, 12, 16)
Response.Write("The event starts at " & dteStart)
%>
The Current Date and Time
To let you get the combination of the current date and the
current time, the
DateTime structure is equipped with a property named Now:
Public Shared ReadOnly Property Now As Date
The Date Part of a Date/Time Value
To let you extract the date part of a date or of a date/time
value, the DateAndTime class is equipped with a property named Date:
Public ReadOnly Property Date As Date
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim current As Date = Now
Response.Write("Today and the current time are " & current)
Response.Write("<br>The date part is " & current.Date)
%>
</body>
</html>
A Date/Time From Parts
One you have a date/time value, you can extract the year,
the month, the day, the hour, the minute, and/or the seconds using any of the
functions we reviewed already.
Operations on Date/Time Values
Introduction
The concepts of the operations we reviewed for date values
are the same for time values. The exception is that, when the dates are
considered, the operations performed on time values can affect the dates.
Date/Time Addition
To add a portion to a time value, you use the same DateAdd()
function we reviewed for date values. To add a number of hours, pass
the first argument as DateInterval.Hour. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim courseStart = #08:15 AM#
Dim length = 2
Dim courseEnd = DateAdd(DateInterval.Hour, length, courseStart)
Response.Write("The course will start at " &
courseStart & " and end at " & courseEnd & ".")
%>
</body>
</html>
This would produce
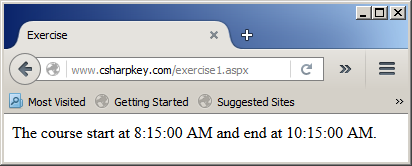
To add a number of minutes, pass
the first argument as DateInterval.Minute, and to add a number of seconds, pass
the first argument as DateInterval.Second..
In the same way, you can perform the addition on a value
that includes both the date and the time values. If the resulting date occurs
after mignight of the first date, the ending date would be different. Here is an
example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim gameStart = #07-31-2016 10:20 PM#
Dim length = 4
Dim gameEnd = DateAdd(DateInterval.Hour, length, gameStart)
Response.Write("The game started on " &
FormatDateTime(gameStart, DateFormat.ShortDate) & " at " &
FormatDateTime(gameStart, DateFormat.ShortTime) & " and ended on " &
FormatDateTime(gameEnd, DateFormat.ShortDate) & " at " &
FormatDateTime(gameEnd, DateFormat.ShortTime) & ".")
%>
</body>
</html>
This would produce
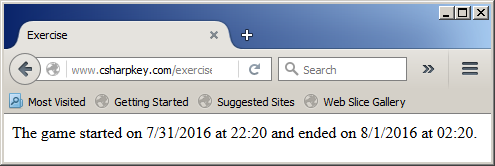
To let you add a number of hours to a date/time value, the DateTime structure
is equipped with a method named. Its syntax is:
Public Function AddHours(value As Double) As Date
To add just a number of hours, pass a natural number. To add
a number of hours and a fraction of the last hour, pass a decimal number. Here
is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim countStart = #08:15 AM#
Dim countEnd = countStart.AddHours(3.58)
Response.Write("The count started at " &
countStart & " and ended at " & countEnd & ".")
%>
</body>
</html>
This would produce:
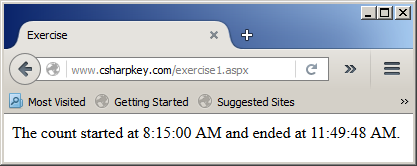
To add a number of minutes to a date/time value, call the DateTime.AddMinutes() method.
Its syntax:
<form method="post" action="index.aspx" runat="server">
</form>
Date/Time Subtraction
To find the difference between two time values, you can use
the same DateDiff() function we reviewed for date values. To get the difference in hours, pass
the first argument as DateInterval.Hour. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim courseStart = #08:15 AM#
Dim courseEnd = #10:15 AM#
Dim lapse = DateDiff(DateInterval.Hour, courseStart, courseEnd)
Response.Write("The course lasted " & lapse & " hours.")
%>
</body>
</html>
This would produce
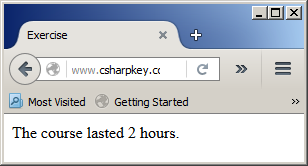
To get the difference in a number of minutes, pass
the first argument as DateInterval.Minute, and to get the difference in seconds, pass
the first argument as DateInterval.Second..
In the same way, you can involve different date/time values
in a subtraction. Even if the dates occur on different days, the operation will
still work well. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim departure = #12-31-2017 10:28 PM#
Dim arrival = #01-01-2018 06:14 AM#
Dim hours = DateDiff(DateInterval.Hour, departure, arrival)
Dim length = DateDiff(DateInterval.Minute, departure, arrival)
Response.Write("The airplane left the airport on " &
FormatDateTime(departure, DateFormat.ShortDate) & " at " &
Format(departure, "t") & " and landed on " &
FormatDateTime(arrival, DateFormat.ShortDate) & " at " &
Format(arrival, "t") & ".")
Response.Write("<br>The flight lasted " & hours &
" hours and " & (length Mod 60) & " minutes.")
%>
</body>
</html>
This would produce
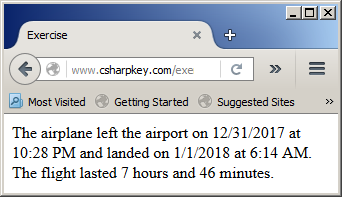
|