 |
Counting and Looping |
|
Fundamentals of Loop Counters
Introduction
A loop is a technique used to repeat
an action. The Visual Basic language provides various techniques and
keywords to perform such actions called loops.
The For...To...Next Loop
Imagine you have a series of numbers you must visit to perform an action on
each. To assist you with this, the Visual Basic language provides a mechanism created with the For...Next expression. One of the loop counters you can use is For...To...Next.
Its formula is:
For counter = start To end
statement(s)
Next
You must use a variable by which the counting would proceed.
You can first declare the variable or you can directly use it after the For keyword
and you must specify its initial value there as the start point. Here is
an example:
<%
Dim i As Integer
. . .
For i = 1 To . . .
Next
%>
After the To keyword,
specify the last value of the counting as the end point. The value being
counted can be used as the index of a collection. In this case, when a
value is passed to the name of the collection, it produces the object. The
result can be a regular item of the collection. The loop ends with the Next keyword. Here are two examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim cost = 0.00
Dim period As Integer
Dim depreciation = 0.00
Dim salvageValue = 0.00
Dim estimatedLife = 0.00
Dim row As New TableRow()
Dim column As New TableCell()
Dim depreciations As New Collection
cost = txtCost.Text
salvageValue = txtSalvageValue.Text
estimatedLife = txtEstimatedLife.Text
For period = 1 To estimatedLife
depreciation = Math.Floor(SYD(cost, salvageValue, estimatedLife, period))
depreciations.Add(depreciation)
Next
lblSchedule.Visible = True
column.Text = "Year"
row.Cells.Add(column)
column = New TableCell()
column.Text = "Depreciation"
row.Cells.Add(column)
tblDepreciation.Rows.Add(row)
For period = 1 To estimatedLife
row = New TableRow()
column = New TableCell()
column.Text = period
row.Cells.Add(column)
column = New TableCell()
column.Text = depreciations(period)
row.Cells.Add(column)
tblDepreciation.Rows.Add(row)
Next
End Sub
</script>
<style>
#container
{
margin: auto;
width: 320px;
}
</style>
<title>Depreciation: Sum-of-Years Digits</title>
</head>
<body>
<form id="frmBusiness" runat="server">
<div id="container">
<h3>Sum-of-Years Digits</h3>
<table>
<tr>
<td><b>Original Value:</b></td>
<td><asp:TextBox id="txtCost" Width="75px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td><b>Salvage Value:</b></td>
<td><asp:TextBox id="txtSalvageValue" Width="55px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td><b>Estimated Life:</b></td>
<td><asp:TextBox id="txtEstimatedLife" Width="55px" runat="server"></asp:TextBox> Years
<asp:Button id="btnCalculate" Text="Calculate" OnClick="btnCalculateClick" runat="server" /></td>
</tr>
</table>
<br />
<b><asp:Label id="lblSchedule" Visible ="False" Text="Depreciation Schedule" runat="server"></asp:Label></b>
<br />
<asp:Table id="tblDepreciation" runat="server" BorderColor="#999999" BorderStyle="Solid" BorderWidth="1pt" Width="317px" GridLines="Both">
</asp:Table>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
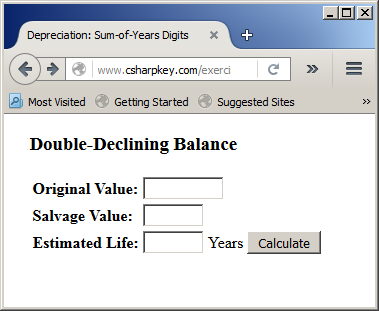
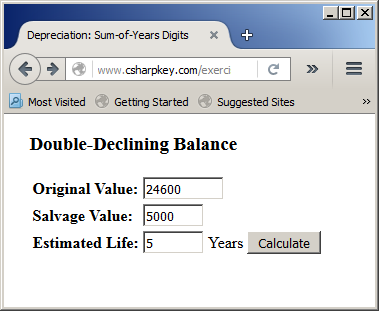
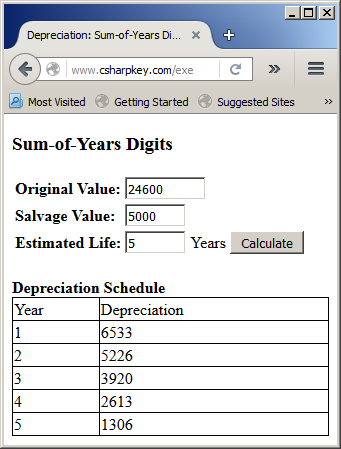
If the values are
coming from a collection, you can use its Count member as the last value of the loop. Here is an
example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim i As Integer
Dim zones As Collection
zones = New Collection
zones.Add("Pacific Standard Time (PST)")
zones.Add("Mountain Standard Time (MST)")
zones.Add("Central Standard Time (CST)")
zones.Add("Eastern Standard Time (EST)")
zones.Add("Atlantic Standard Time (AST)")
zones.Add("Alaskan Standard Time (AKST)")
zones.Add("Hawaii-Aleutian Standard Time (HST)")
zones.Add("Samoa standard time (UTC-11)")
zones.Add("Chamorro Standard Time (UTC+10)")
For i = 1 To zones.Count
lbxTimeZones.Items.Add(zones(i))
Next
End Sub
</script>
<title>United States Time Zones</title>
</head>
<body>
<h3>United States Time Zones</h3>
<form id="frmTimeZones" runat="server">
<asp:ListBox id="lbxTimeZones" Height="160px" runat="server"></asp:ListBox>
</form>
</body>
</html>
This would produce:
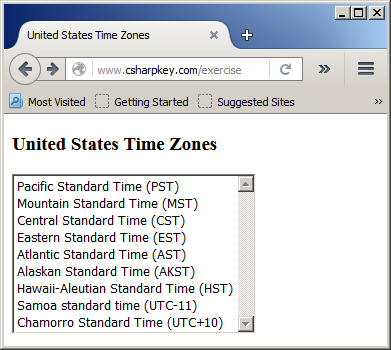
You can also use a loop to get an object from a collection. Such an object would hold the members of a class. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script Language="VB" runat="server">
Public Class Employee
Public EmployeeNumber As String
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim staff As Employee
Dim employees As New Collection
Dim worker As New Employee
staff = New Employee
staff.EmployeeNumber = "953-084"
staff.FirstName = "Anthony"
staff.LastName = "Walters"
staff.HourlySalary = 18.52
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "204-815"
staff.FirstName = "Jeannine"
staff.LastName = "Rocks"
staff.HourlySalary = 22.74
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "728-411"
staff.FirstName = "Aaron"
staff.LastName = "Gibson"
staff.HourlySalary = 35.08
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "528-492"
staff.FirstName = "Stephen"
staff.LastName = "Brothers"
staff.HourlySalary = 24.93
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "303-415"
staff.FirstName = "Laura"
staff.LastName = "Edom"
staff.HourlySalary = 15.75
employees.Add(staff)
Response.Write("<h3>Employees Records</h3>")
Response.Write("<table border=5><tr><td><b>Employee #<b></td><td><b>First Name</b></td>")
Response.Write("<td><b>Last Name</b></td><td><b>Salary</b></td></tr>")
For index As Integer = 1 To employees.Count
worker = employees(index)
Response.Write("<tr><td>" & worker.EmployeeNumber &
"</td><td>" & worker.FirstName &
"</td><td>" & worker.LastName &
"</td><td>" & worker.HourlySalary & "</td><tr>")
Next
Response.Write("</table>")
End Sub
</script>
<title>Exercise</title>
</head>
<body>
</body>
</html>
This would produce:
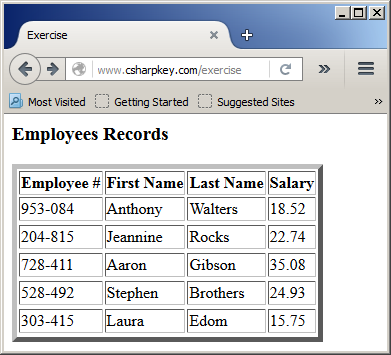
Options on Loops Counters
The Explicit Next Item
If you want to explicitly indicate that you accessing the next item for each subsequent loop, type the looing variable after the Next keywork. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Public Structure Road
Public Sub New(ByVal name As String, ByVal type As String,
ByVal length As Single, ByVal where As String)
RoadName = name
Category = type
Distance = length
Location = where
End Sub
Public Property RoadName As String
Public Property Category As String
Public Property Distance As Single
Public Property Location As String
End Structure
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim index As Integer
Dim route As New Road
Dim roads As New Collection
roads.Add(New Road("MD 410", "Road", 13.92, "From East Bethesda To Pennsy Drive In Landover Hills"))
roads.Add(New Road("I-5", "Interstate", 796.432, "From Mexico-United States border to South of Oregon"))
roads.Add(New Road("NE 14", "State Highway", 203.54, "From Superior, KS To SD 37"))
roads.Add(New Road("I-296", "Interstate", 3.43, "Michigan"))
roads.Add(New Road("US 2", "Road", 2571.0, "Western Segment From Washington Rte 529 to I 75 in Michigan; Eastern Segment From US 11 in NY to I-95 in Maine."))
Response.Write("<h2 style='text-align: center'>Road System</h2>")
Response.Write("<table border=1><tr><td><b>Road Name</b></td><td><b>Road Type</b></td><td><b>Distance</b></td><td><b>Location</b></td></tr>")
For index = 1 To roads.Count
Response.Write("<tr><td style='width: 90px'>" & roads(index).RoadName & "</td><td style='width: 100px'>" & roads(index).Category &
"</td><td style='width: 100px'>" & roads(index).Distance & " miles</td><td>" & roads(index).Location & "</td></tr>")
Next index
Response.Write("</table>")
End Sub
</script>
<title>Road System</title>
</head>
<body>
</body>
</html>
This would produce:
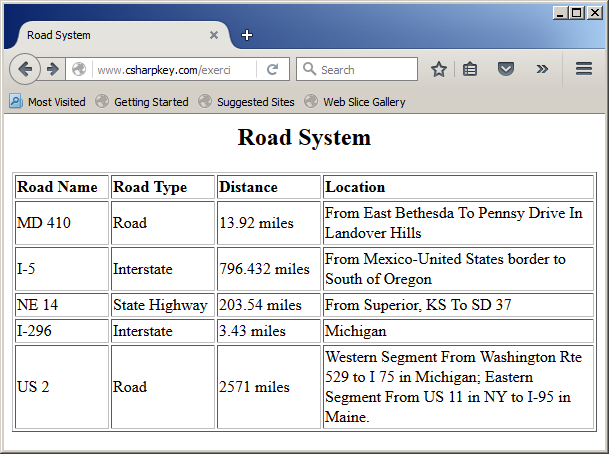
Stepping the Counting Loop
The regular formula of a loop increments the counting by
1 at the end of each statement. If you want to control how the
incrementing processes, you can set your own, using the Step option.
The formula to follow is:
For counter = start To end Step increment
statement(s)
Next
You can set the incrementing value to your
choice. If the value of increment is positive, the counter will be
added to its value. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim month As Integer
Dim months As New Collection
months.Add("January") : months.Add("February")
months.Add("March") : months.Add("April")
months.Add("May") : months.Add("June")
months.Add("July") : months.Add("August")
months.Add("September") : months.Add("October")
months.Add("November") : months.Add("December")
For month = 1 To months.Count
lbxMonths.Items.Add(months(month))
Next
For month = 1 To months.Count Step 3
lbxSemester.Items.Add(months(month))
Next
End Sub
</script>
<title>Months of the Year</title>
<style type="text/css">
table { width: 300px; }
</style>
</head>
<body>
<form id="frmMonths" runat="server">
<table>
<tr>
<td><p><b>Months of the Year</b></p></td>
<td> </td>
<td><p><b>Months that start a semester</b></p></td>
</tr>
<tr>
<td><asp:ListBox id="lbxMonths" Width="170px"
runat="server" Height="210px"></asp:ListBox></td>
<td> </td>
<td style="vertical-align: top">
<asp:ListBox id="lbxSemester" Width="220px"
runat="server"></asp:ListBox></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of using the webpage:
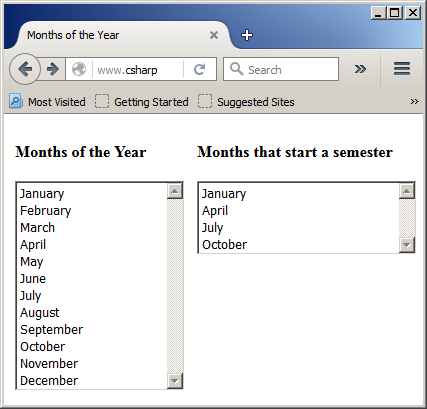
On the other hand, if you want to loop from a higher value to the a lower value, set a negative value to the increment
factor and use the following formula:
For counter = end To start Step decrement
statement(s)
Next
Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim month As Integer
Dim months As New Collection
months.Add("January") : months.Add("February")
months.Add("March") : months.Add("April")
months.Add("May") : months.Add("June")
months.Add("July") : months.Add("August")
months.Add("September") : months.Add("October")
months.Add("November") : months.Add("December")
For month = 1 To months.Count
lbxMonths.Items.Add(months(month))
Next
For month = months.Count To 1 Step -1
lbxBackwards.Items.Add(months(month))
Next
End Sub
</script>
<title>Months of the Year</title>
<style type="text/css">
table { width: 300px; }
</style>
</head>
<body>
<form id="frmMonths" runat="server">
<table>
<tr>
<td><p><b>Months of the Year</b></p></td>
<td> </td>
<td><p><b>Months Backwards</b></p></td>
</tr>
<tr>
<td><asp:ListBox id="lbxMonths" Width="170px"
runat="server" Height="210px"></asp:ListBox></td>
<td> </td>
<td style="vertical-align: top">
<asp:ListBox id="lbxBackwards" Width="170px" Height="210px"
runat="server"></asp:ListBox></td>
</tr>
</table>
</form>
</body>
</html>
This would produce:
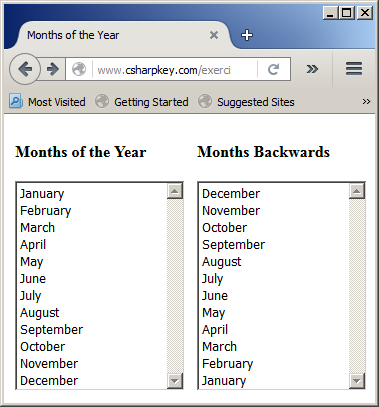
Loop Counters and Procedures
Introduction
You can create a looping statement in a procedure or in an event of a webcontrol. When you do this, you may let the whole loop to be processed. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub CreateWeekDays()
Dim days As New Collection
days.Add("Monday")
days.Add("Tuesday")
days.Add("Wednesday")
days.Add("Thursday")
days.Add("Friday")
days.Add("Saturday")
days.Add("Sunday")
Response.Write("<b>Working Days</b>")
For d = 1 To days.Count
Response.Write("<br>" & days(d))
Next
End Sub
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
CreateWeekDays()
End Sub
</script>
<title>Employees Payroll</title>
</head>
<body>
</body>
</html>
This would produce:
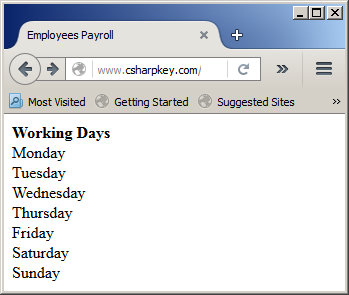
In some cases, you may want to exit a conditional statement or a loop before its end. To assist with with this, the Visual Basic language provides the Exit keyword. It can be used in the body of a procedure or a loop.
Exiting a Procedure
Exiting a procedure consists of interrupting its flow and jumping to the End line of the procedure. To do this anywhere in the body of a procedure, type Exit Sub. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub CreateWeekDays()
Dim days As New Collection
days.Add("Monday")
days.Add("Tuesday")
days.Add("Wednesday")
days.Add("Thursday")
days.Add("Friday")
Exit Sub
days.Add("Saturday")
days.Add("Sunday")
Response.Write("<b>Working Days</b>")
For d = 1 To days.Count
Response.Write("<br>" & days(d))
Next
End Sub
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
CreateWeekDays()
End Sub
</script>
<title>Employees Payroll</title>
</head>
<body>
</body>
</html>
This would produce:
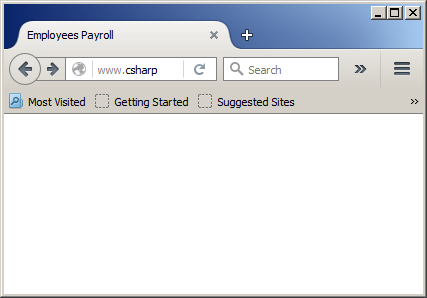
Exiting a For Loop Counter
You can also exit a For loop. To do this, in the section where you want to stop, type Exit For. This would stop the flow of the loop and jump to the line immediately after the Next keyword. This feature of the Visual Basic language is very valuable when looking for a specific value or object in a collection. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Public Class Employee
Public EmployeeNumber As String
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim staff As Employee
Dim worker As New Employee
Dim employeeFound As Boolean
Dim employees As New Collection
employeeFound = False
staff = New Employee
staff.EmployeeNumber = "953-084"
staff.FirstName = "Anthony"
staff.LastName = "Walters"
staff.HourlySalary = 18.52
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "204-815"
staff.FirstName = "Jeannine"
staff.LastName = "Rocks"
staff.HourlySalary = 22.74
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "728-411"
staff.FirstName = "Aaron"
staff.LastName = "Gibson"
staff.HourlySalary = 35.08
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "528-492"
staff.FirstName = "Stephen"
staff.LastName = "Brothers"
staff.HourlySalary = 24.93
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "303-415"
staff.FirstName = "Laura"
staff.LastName = "Edom"
staff.HourlySalary = 15.75
employees.Add(staff)
' Check every record in the collection of employees
For index As Integer = 1 To employees.Count
' Get a reference to the current record
worker = employees(index)
' If the employee number of the current record is the same as the user typed, ...
If worker.EmployeeNumber = txtEmployeeNumber.Text Then
' ... display the first and last names
txtEmployeeName.Text = worker.LastName & ", " & worker.FirstName
' Since the employee has been found, make a note.
employeeFound = True
' Now that the employee has found, there is no reason to keep looking. Exit the loop
Exit For
End If
' As long as we haven't finished with the loop and we haven't
' found the employee, continue with the loop
Next
If employeeFound = False Then
txtEmployeeName.Text = ""
txtEmployeeNumber.Text = ""
End If
End Sub
Sub btnSubmitTimeSheetClick(ByVal sender As Object, ByVal e As EventArgs)
End Sub
</script>
<title>Employee Time Sheet</title>
</head>
<body>
<h3>Employee Time Sheet</h3>
<form id="frmTimeSheet" runat="server">
<table>
<tr>
<td><b>Employee #:</b></td>
<td><asp:TextBox id="txtEmployeeNumber" Width="60px" runat="server" AutoPostBack="True"></asp:TextBox></td>
<td><asp:TextBox id="txtEmployeeName" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<table>
<tr>
<td><b>Monday</b></td>
<td><b>Tuesday</b></td>
<td><b>Wednesday</b></td>
<td><b>Thursday</b></td>
<td><b>Friday</b></td>
<td><b>Saturday</b></td>
<td><b>Sunday</b></td>
</tr>
<tr>
<td><asp:TextBox id="txtMonday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtTuesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtWednesday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtThursday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtFriday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtSaturday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
<td><asp:TextBox id="txtSunday" Text="0.00" Width="60px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br>
<table width="520">
<tr>
<td style="text-align: center">
<asp:Button id="btnSubmitTimeSheet" Text="Submit Time Sheet"
OnClick="btnSubmitTimeSheetClick" runat="server"></asp:Button></td>
</tr>
</table>
</form>
</body>
</html>
Here is an example of using the webpage:
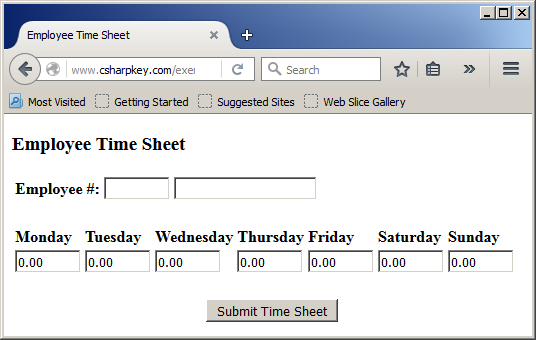
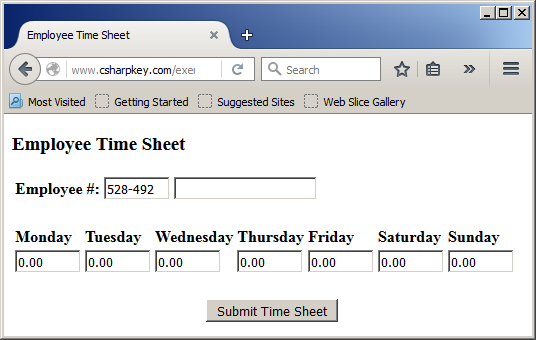
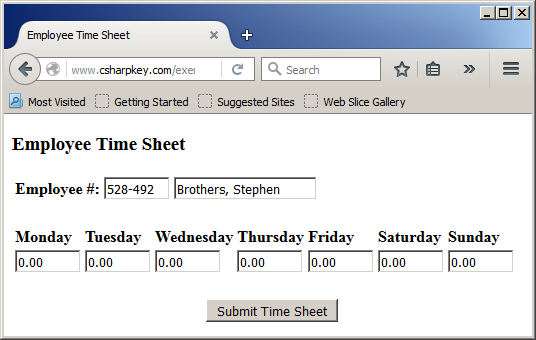
Loops Repeaters
Introduction
The Visual Basic language presents many variations of loops. They combine the Do and the Loop
keywords.
The Do...Loop While Loop
One of the formulas to perform a loop uses the Do... Loop While approach. The formula to follow is:
Do
statement(s)
Loop While condition
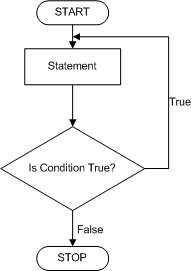
The statement(s) would execute first. Then the condition would be checked. If the condition
is true, then the statement(s) would execute again. The check-execution
routine would continue as long as the condition is true. If/Once the condition becomes false, the statement will not execute anymore and the code will move beyond the loop. Here is an example:
<%@ Page Language="VB" %>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim pers = 0
Dim index = 0
Dim periods = 0.00
Dim payment = 0.00
Dim compounded = 4
Dim interest = 0.00
Dim futureValue = 0.00
Dim interestRate = 0.00
Dim presentValue = 0.00
Dim row As New TableRow()
Dim column As New TableCell()
Dim payments As New Collection
Dim interests As New Collection
futureValue = txtFutureValue.Text
interestRate = CDbl(txtInterestRate.Text) / 100.0
If rblCompounded.SelectedIndex = 0 Then
compounded = 365
ElseIf rblCompounded.SelectedIndex = 1 Then
compounded = 52
ElseIf rblCompounded.SelectedIndex = 2 Then
compounded = 12
ElseIf rblCompounded.SelectedIndex = 3 Then
compounded = 4
Else ' If rblCompounded.SelectedIndex = 4 Then
compounded = 1
End If
periods = CDbl(txtPeriods.Text)
presentValue = futureValue / Math.Pow(1 + interestRate / compounded, (periods * compounded))
index = 1
Do
payment = futureValue / Math.Pow(1 + interestRate / compounded, (periods * compounded) - index)
payments.Add(payment)
index += 1
Loop While index <= periods * compounded
interest = payments(1) - presentValue
interests.Add(interest)
index = 2
Do
interest = payments(index) - payments(index - 1)
interests.Add(interest)
index += 1
Loop While index <= periods * compounded
txtPresentValue.Text = FormatNumber(presentValue)
index = 1
lblPayments.Visible = True
column.Text = "Period"
column.Width = 80
row.Cells.Add(column)
column = New TableCell()
column.Text = "Interest"
column.Width = 100
row.Cells.Add(column)
tblPayments.Rows.Add(row)
column = New TableCell()
column.Text = "Amount"
column.Width = 120
row.Cells.Add(column)
tblPayments.Rows.Add(row)
index = 1
Do
row = New TableRow()
column = New TableCell()
column.Text = index
row.Cells.Add(column)
column = New TableCell()
column.Text = FormatNumber(interests(index))
row.Cells.Add(column)
column = New TableCell()
column.Text = FormatNumber(payments(index))
row.Cells.Add(column)
tblPayments.Rows.Add(row)
index += 1
Loop While index <= periods * compounded
End Sub
</script>
<style>
#container
{
margin: auto;
width: 345px;
}
</style>
<title>Business Mathematics - Investment</title>
</head>
<body>
<form id="frmBusiness" runat="server">
<div id="container">
<h3>Business Mathematics - Investment</h3>
<table style="width: 400px">
<tr>
<td style="width: 140px"><b>Future Value:</b></td>
<td><asp:TextBox id="txtFutureValue" Width="75px" runat="server"></asp:TextBox></td>
<td rowspan="5">
<asp:RadioButtonList id="rblCompounded" runat="server" Width="120px">
<asp:ListItem>Daily</asp:ListItem>
<asp:ListItem>Weekly</asp:ListItem>
<asp:ListItem Selected="True">Monthly</asp:ListItem>
<asp:ListItem>Quarterly</asp:ListItem>
<asp:ListItem>Yearly</asp:ListItem>
</asp:RadioButtonList></td>
</tr>
<tr>
<td><b>Interest Rate:</b></td>
<td><asp:TextBox id="txtInterestRate" Width="55px" runat="server"></asp:TextBox>%</td>
</tr>
<tr>
<td><b>Number of Periods:</b></td>
<td><asp:TextBox id="txtPeriods" Width="55px" runat="server"></asp:TextBox>Years</td>
</tr>
<tr>
<td> </td>
<td>
<asp:Button id="btnCalculate" Text="Calculate" OnClick="btnCalculateClick" runat="server" /></td>
</tr>
</table>
<br />
<table>
<tr>
<td style="width: 140px"><b>Present Value:</b></td>
<td><asp:TextBox id="txtPresentValue" Width="120px" runat="server"></asp:TextBox></td>
</tr>
</table>
<br />
<b><asp:Label id="lblPayments" Visible ="False" Text="Schedule of Payments" runat="server"></asp:Label></b>
<br />
<asp:Table id="tblPayments" runat="server" BorderColor="Black" BorderStyle="Dotted" BorderWidth="1pt" GridLines="Both"></asp:Table>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
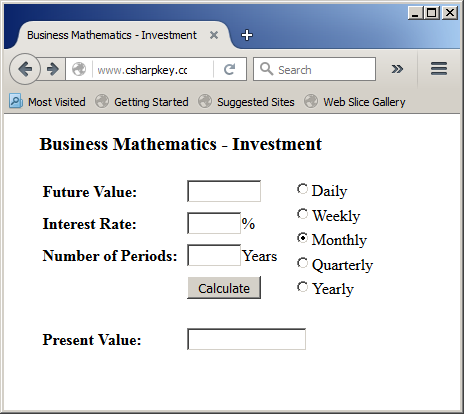
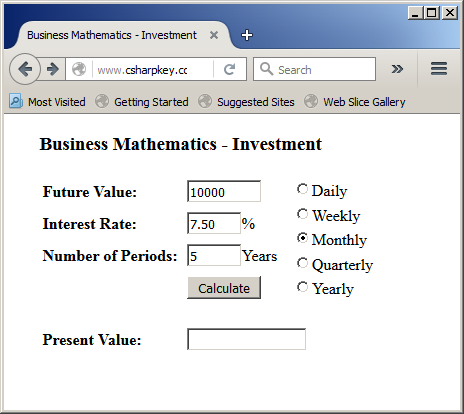
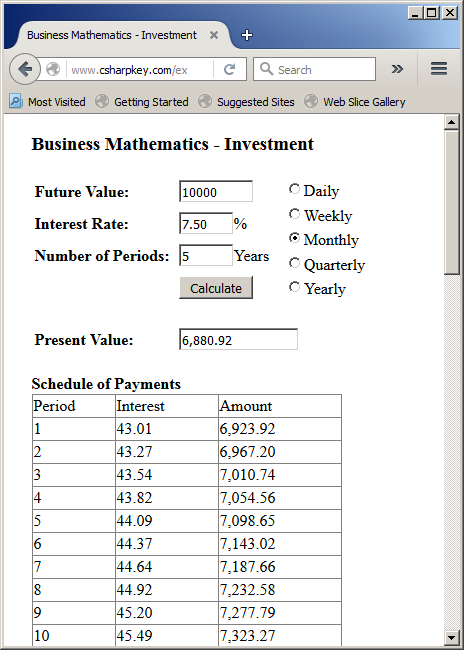
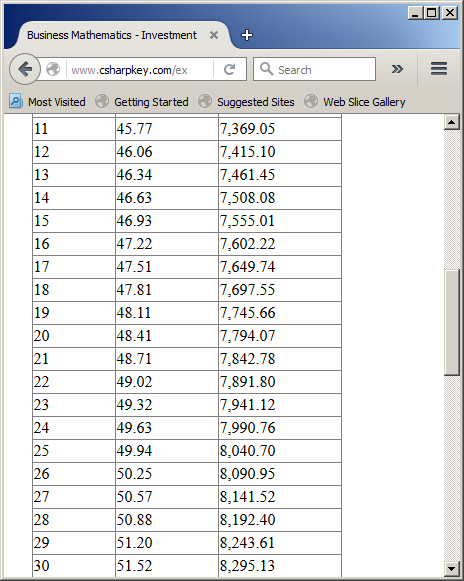
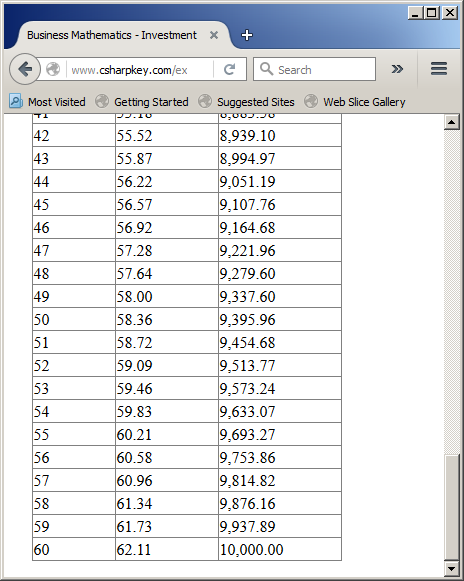
As you may guess already,
the condition must provide a way for it to be true or to be false.
Otherwise, the looping would execute continually.
The Do...Loop Until Loop
An alternative to the Do... Loop While uses the following formula:
Do
statement(s)
Loop Until condition
Once again, the statement(s) section executes first. After executing the statement(s), the
condition is checked. If the condition is true, the statement(s) section executes again. This will continue until the condition becomes false.
Once the condition becomes false, the loop stops and the flow continues with the section under the Loop Until
line. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim cost = 0
Dim period = 1
Dim bookValue = 0.0
Dim salvageValue = 0.0
Dim estimatedLife = 0.0
Dim depreciationRate = 0
Dim row As New TableRow()
Dim yearlyDepreciation = 0.0
Dim column As New TableCell()
cost = txtCost.Text
salvageValue = txtSalvageValue.Text
estimatedLife = txtEstimatedLife.Text
depreciationRate = 100.0 / estimatedLife
yearlyDepreciation = SLN(cost, salvageValue, estimatedLife)
bookValue = cost
lblDepreciation.Visible = True
column.Text = "<b>Year</b>"
column.Width = 80
row.Cells.Add(column)
column = New TableCell()
column.Text = "<b>Depreciation Rate</b>"
column.Width = 120
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = "<b>Yearly Depreciation</b>"
column.Width = 120
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = "<b>Book Value</b>"
column.Width = 100
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = "<b>Accumulated Depreciation</b>"
column.Width = 100
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
Do
row = New TableRow()
column = New TableCell()
column.Text = period
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = depreciationRate & "%"
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = FormatNumber(yearlyDepreciation)
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = FormatNumber(Format(bookValue - (yearlyDepreciation * period), "#,000"))
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
column = New TableCell()
column.Text = Format(yearlyDepreciation * period, "#,000")
row.Cells.Add(column)
tblDepreciations.Rows.Add(row)
period += 1
Loop Until period = estimatedLife + 1
End Sub
</script>
<style>
#container
{
margin: auto;
width: 400px;
}
</style>
<title>Depreciation: The Straight-Line Method</title>
</head>
<body>
<form id="frmBusiness" runat="server">
<div id="container">
<h3>Straight-Line Method</h3>
<table>
<tr>
<td><b>Asset Original Value:</b></td>
<td><asp:TextBox id="txtCost" Width="75px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td><b>Salvage Value:</b></td>
<td><asp:TextBox id="txtSalvageValue" Width="55px" runat="server"></asp:TextBox></td>
</tr>
<tr>
<td><b>Estimated Life:</b></td>
<td><asp:TextBox id="txtEstimatedLife" Width="55px" runat="server"></asp:TextBox>
<asp:Button id="btnCalculate" Text="Calculate"
OnClick="btnCalculateClick" runat="server" /></td>
</tr>
</table>
<p><b><asp:Label id="lblDepreciation" Visible="false" Text="Depreciation Schedule" runat="server"></asp:Label></b></p>
<asp:Table id="tblDepreciations" runat="server" BorderColor="#333333" BorderStyle="Double" BorderWidth="2pt" GridLines="Both"></asp:Table>
</div>
</form>
</body>
</html>
This would produce:
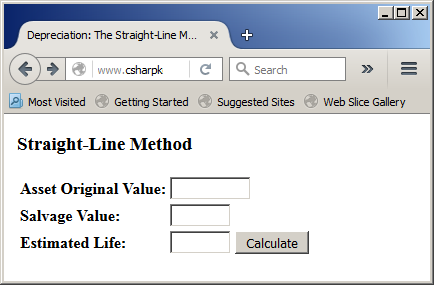
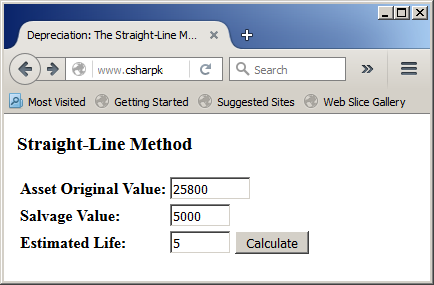
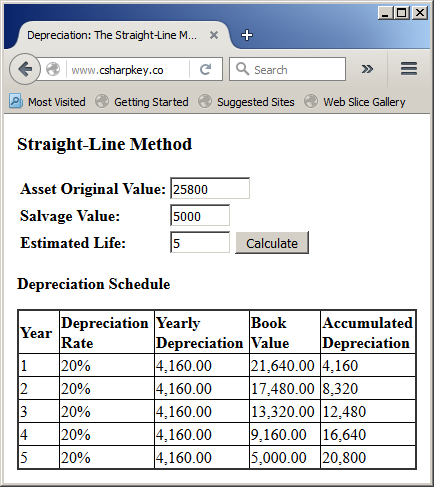
The Do While... Loop Loop
The Visual Basic language provides another loop option that use the Do While... Loop
expression. Its formula is:
Do While condition
statement(s)
Loop
This time, the condition id checked first. If
the condition is true, then the statement(s) execute(s). Then the condition is checked again. If the Condition is false, or once/when the condition becomes
false, the statement(s) section is(are) skipped and the flow continues with the code below the Loop keyword. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Public Class Element
Public AtomicNumber As Integer
Public ChemicalSymbol As String
Public ElementName As String
Public AtomicMass As String
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim elm As Element
Dim current As Element
Dim periodicTable As New Collection
elm = New Element
elm.AtomicNumber = 1
elm.ChemicalSymbol = "H"
elm.ElementName = "Hydrogen"
elm.AtomicMass = 1.0079
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 2
elm.ChemicalSymbol = "He"
elm.ElementName = "Helium"
elm.AtomicMass = 4.002682
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 3
elm.ChemicalSymbol = "Li"
elm.ElementName = "Lithium"
elm.AtomicMass = 6.941
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 4
elm.ChemicalSymbol = "Be"
elm.ElementName = "Berylium"
elm.AtomicMass = 9.0122
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 5
elm.ChemicalSymbol = "B"
elm.ElementName = "Boron"
elm.AtomicMass = 10.811
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 6
elm.ChemicalSymbol = "C"
elm.ElementName = "Carbon"
elm.AtomicMass = 12.011
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 7
elm.ChemicalSymbol = "N"
elm.ElementName = "Nitrogen"
elm.AtomicMass = 14.007
periodicTable.Add(elm)
elm = New Element
elm.AtomicNumber = 8
elm.ChemicalSymbol = "O"
elm.ElementName = "Oxygen"
elm.AtomicMass = 15.999
periodicTable.Add(elm)
Response.Write("<h3>Chemistry - Periodic Table</h3>")
Response.Write("<table border=3><tr><td><b>Atomic #<b></td><td><b>Symbol</b></td>")
Response.Write("<td><b>Element</b></td><td><b>Mass</b></td></tr>")
nbr = 1
Do While nbr <= periodicTable.Count
current = periodicTable(nbr)
Response.Write("<tr><td>" & current.AtomicNumber &
"</td><td>" & current.ChemicalSymbol &
"</td><td>" & current.ElementName &
"</td><td>" & current.AtomicMass & "</td><tr>")
nbr += 1
Loop
End Sub
</script>
<title>Chemistry - Periodic Table</title>
</head>
<body>
</body>
</html>
This would produce:
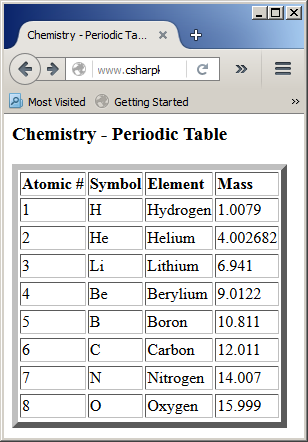
The Do Until... Loop Statement
An alternative to the Do While... Loop loop
uses the following formula:
Do Until condition
statement(s)
Loop
This loop works like the Do While... Loop expression. The condition is examined first. If the
condition is true, then the statement(s) section executes. Here is an example:
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class DoubleDecliningBalance
Inherits Form
Dim lblCost As Label
Dim txtCost As TextBox
Dim lblPercent As Label
Dim lblDepreciationRate As Label
Dim txtDepreciationRate As TextBox
Dim lblEstimatedLife As Label
Dim txtEstimatedLife As TextBox
Dim lblYears As Label
Dim lblFactor As Label
Dim txtFactor As TextBox
Dim lblDepreciation As Label
Dim lvwDepreciations As ListView
Dim WithEvents btnCalculate As button
Public Sub New()
InitializeComponent()
End Sub
Private Sub InitializeComponent()
lblCost = New Label()
lblCost.AutoSize = True
lblCost.Location = New Point(18, 19)
lblCost.Text = "Original Value:"
Controls.Add(lblCost)
txtCost = New TextBox()
txtCost.Location = New Point(125, 16)
Controls.Add(txtCost)
lblDepreciationRate = New Label()
lblDepreciationRate.AutoSize = True
lblDepreciationRate.Location = New Point(18, 45)
lblDepreciationRate.Text = "Depreciation Rate:"
Controls.Add(lblDepreciationRate)
txtDepreciationRate = New TextBox()
txtDepreciationRate.Width = 60
txtDepreciationRate.Location = New Point(125, 43)
Controls.Add(txtDepreciationRate)
lblPercent = New Label()
lblPercent.AutoSize = True
lblPercent.Location = New Point(188, 43)
lblPercent.Text = "%"
Controls.Add(lblPercent)
lblEstimatedLife = New Label()
lblEstimatedLife.AutoSize = True
lblEstimatedLife.Location = New Point(18, 73)
lblEstimatedLife.Text = "Estimated Life:"
Controls.Add(lblEstimatedLife)
txtEstimatedLife = New TextBox()
txtEstimatedLife.Width = 60
txtEstimatedLife.Location = New Point(125, 70)
Controls.Add(txtEstimatedLife)
lblYears = New Label()
lblYears.AutoSize = True
lblYears.Location = New Point(188, 71)
lblYears.Text = "Years"
Controls.Add(lblYears)
lblFactor = New Label()
lblFactor.AutoSize = True
lblFactor.Location = New Point(18, 101)
lblFactor.Text = "Factor:"
Controls.Add(lblFactor)
txtFactor = New TextBox()
txtFactor.Location = New Point(125, 98)
txtFactor.Text = "2"
Controls.Add(txtFactor)
btnCalculate = New Button()
btnCalculate.Location = New Point(235, 97)
btnCalculate.Text = "Calculate"
Controls.Add(btnCalculate)
lblDepreciation = New Label()
lblDepreciation.AutoSize = True
lblDepreciation.Location = New Point(18, 142)
lblDepreciation.Text = "Depreciation Schedule"
Controls.Add(lblDepreciation)
lvwDepreciations = New ListView()
lvwDepreciations.Width = 580
lvwDepreciations.GridLines = True
lvwDepreciations.View = View.Details
lvwDepreciations.FullRowSelect = True
lvwDepreciations.Location = New Point(15, 160)
lvwDepreciations.Columns.Add("Year", 40, HorizontalAlignment.Right)
lvwDepreciations.Columns.Add("Book Value at Beginning of Year", 170, HorizontalAlignment.Right)
lvwDepreciations.Columns.Add("Depreciation Rate", 100, HorizontalAlignment.Right)
lvwDepreciations.Columns.Add("Depreciation for Year", 120, HorizontalAlignment.Right)
lvwDepreciations.Columns.Add("Book Value at End of Year", 145, HorizontalAlignment.Right)
Controls.Add(lvwDepreciations)
Me.Width = 620
Me.Height = 300
Me.Text = "Depreciation: Double-Declining Balance"
End Sub
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs) Handles btnCalculate.Click
Dim year = 0
Dim cost = 0.0
Dim factor = 0
Dim period = 1
Dim deprec = 0.0
Dim bookValue = 0.0
Dim depreciation = 0.0
Dim estimatedLife = 0.0
Dim depreciationRate = 0.0
Dim depreciations As New Collection
Dim bookValuesEnd As New Collection
Dim bookValuesBeginning As New Collection
cost = txtCost.Text
depreciationRate = txtDepreciationRate.Text
estimatedLife = txtEstimatedLife.Text
factor = CType(txtFactor.Text, Integer)
depreciation = DDB(cost, depreciationRate, estimatedLife, period, factor)
year = 1
Do Until year = estimatedLife + 1
deprec = Math.Floor(DDB(cost, depreciationRate, estimatedLife, year, factor))
depreciations.Add(deprec)
year += 1
Loop
bookValuesBeginning.Add(cost)
year = 2
Do Until year = estimatedLife + 1
bookValue = Math.Floor(bookValuesBeginning(year - 1) - depreciations(year - 1))
bookValuesBeginning.Add(bookValue)
year += 1
Loop
bookValuesEnd.Add(bookValuesBeginning(2))
year = 2
Do Until year = estimatedLife + 1
bookValue = Math.Floor(bookValuesEnd(year - 1) - depreciations(year))
bookValuesEnd.Add(bookValue)
year += 1
Loop
lvwDepreciations.Items.Clear()
year = 1
Do Until year = estimatedLife + 1
Dim lviDepreciation = New ListViewItem(year)
lviDepreciation.SubItems.Add(Format(bookValuesBeginning(year), "#,000"))
lviDepreciation.SubItems.Add(depreciationRate & "%")
lviDepreciation.SubItems.Add(Format(depreciations(year), "N"))
lviDepreciation.SubItems.Add(Format(bookValuesEnd(year), "#,000"))
lvwDepreciations.Items.Add(lviDepreciation)
year += 1
Loop
End Sub
<STAThread()>
Public Shared Sub Main()
Application.Run(New DoubleDecliningBalance())
End Sub
End Class
This would produce:
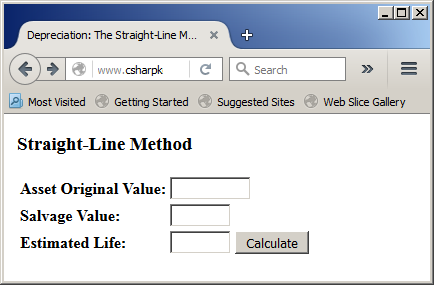
|