 |
Conditional Selections
|
|
The Select...Case Statement
Introduction
If you have a large number of conditions to examine, the If...Then...Else statement will go through each one of them. The Visual Basic language offers the alternative of jumping to the statement that applies to the stated condition. This is done with the Select Case operator.
The formula of the Select Case statement is:
Select Case expression
Case expression1
statement1
Case expression2
statement2
Case expressionX
statementX
End Select
The statement starts with Select Case and ends with End Select. On the right side of Select Case, enter a value or an expression that will be checked.
The Type of Expression to Test
The value of expression can be Boolean value. In this case, one case would be True and the other would be False. Here is an example:
<!DOCTYPE html>
<html>
<head>
<script language="VB" runat="server">
Sub btnCreateRecordClick(ByVal sender As Object, ByVal e As EventArgs)
dim unitPrice As Double
dim markedPrice As Double
dim discountAmount As Double
unitPrice = CDbl(txtUnitPrice.Text)
Select Case chkApplyDiscount.Checked
Case True
discountAmount = unitPrice * 20.00 / 100.00
Case False
discountAmount = 0.00
End Select
markedPrice = unitPrice - discountAmount
lblItemNumber.Text = txtItemNumber.Text
lblItemName.Text = txtItemName.Text
lblUnitPrice.Text = FormatNumber(markedPrice)
End Sub
</script>
<title>Department Store - Inventory Creation</title>
</head>
<body>
<div align="center">
<form id="frmStoreItem" runat="server">
<h3>Department Store - Inventory Creation</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:TextBox id="txtItemNumber" text="000000" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:TextBox id="txtItemName" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td><asp:TextBox id="txtUnitPrice" text="0.00" runat="server" /></td>
</tr>
<tr>
<td><asp:CheckBox id="chkApplyDiscount" text="Apply 20% Discount"
TextAlign="Left" runat="server" /></td>
<td> </td>
</tr>
<tr>
<td> </td>
<td><asp:Button id="btnCreateRecord"
text="Create Record"
OnClick="btnCreateRecordClick"
runat="server"></asp:Button> </td>
</tr>
</table>
<h3>Department Store - Store Inventory</h3>
<table>
<tr>
<td>Item #:</td>
<td><asp:Label id="lblItemNumber" runat="server" /></td>
</tr>
<tr>
<td>Item Name:</td>
<td><asp:Label id="lblItemName" runat="server" /></td>
</tr>
<tr>
<td>Unit Price:</td>
<td><asp:Label id="lblUnitPrice" runat="server" /></td>
</tr>
</table>
</form>
</div>
</body>
</html>
Here is an example of using the webpage:
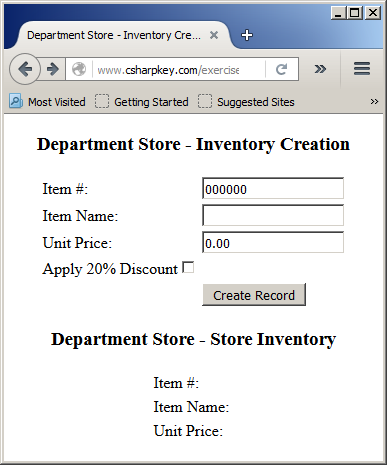
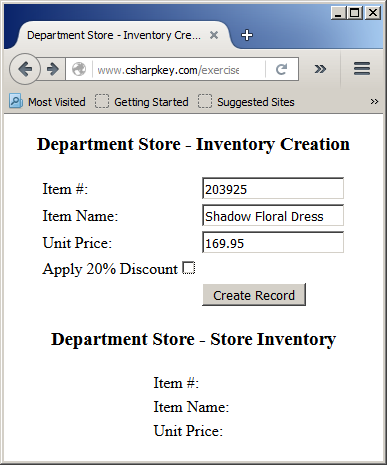
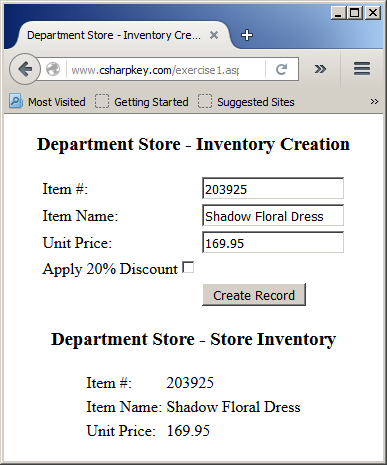
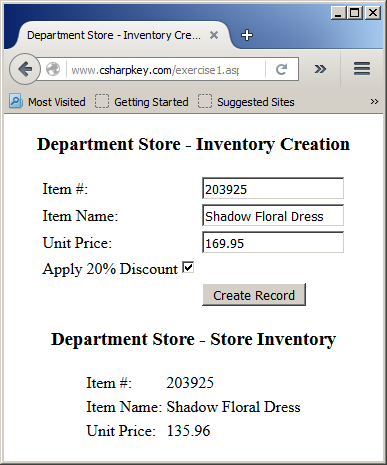
The expression can produce a character (Char type) or a string
(a String type). In this case, each Case would deal with a value in double-quotes. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub ddlLinesSelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
Select Case ddlLines.SelectedItem.Text
Case "Red Line"
pnlAllStations.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = False
pnlRedLine.Visible = True
Case "Green Line"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = True
Case "Blue Line"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = True
Case "Orange Line"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = False
pnlOrangeLine.Visible = True
Case "All Stations"
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlAllStations.Visible = True
End Select
End Sub
</script>
<style type="text/css">
#main-title
{
font-size: 1.28em;
font-weight: bold;
text-align: center;
font-family: Georgia, Garamond, Times New Roman, Times, serif;
}
h2 { text-align: center; }
#tblMetroLineSelection { width: 390px; }
#whole
{
margin: auto;
width: 395px;
}
</style>
<title>Metro System</title>
</head>
<body>
<form id="frmMetroSystem" runat="server">
<div id="whole">
<p id="main-title">Metro System</p>
<table id="tblMetroLineSelection" align="center">
<tr>
<td>
<asp:Label id="lblMetroLine" runat="server"
Text="Select the metro line:"></asp:Label>
</td>
<td>
<asp:DropDownList id="ddlLines" runat="server"
AutoPostBack="True"
OnSelectedIndexChanged="ddlLinesSelectedIndexChanged">
<asp:ListItem>Red Line</asp:ListItem>
<asp:ListItem>Green Line</asp:ListItem>
<asp:ListItem>Blue Line</asp:ListItem>
<asp:ListItem>Orange Line</asp:ListItem>
<asp:ListItem>All Stations</asp:ListItem>
</asp:DropDownList>
</td>
</tr>
</table>
<asp:Panel id="pnlAllStations" runat="server" Visible="True">
<h2>All Stations</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxAllStations" Height="400px" style="margin: 0 auto" runat="server">
<asp:ListItem>Shady Grove</asp:ListItem><asp:ListItem>Rockville</asp:ListItem>
<asp:ListItem>Twinbrook</asp:ListItem><asp:ListItem>White Flint</asp:ListItem>
<asp:ListItem>Grosvenor-Strathmore</asp:ListItem><asp:ListItem>Medical Center</asp:ListItem>
<asp:ListItem>Bethesda</asp:ListItem><asp:ListItem>Friendship Heights</asp:ListItem>
<asp:ListItem>Tenleytown-AU</asp:ListItem><asp:ListItem>Van Ness-UDC</asp:ListItem>
<asp:ListItem>Greebelt</asp:ListItem><asp:ListItem>Cleveland Park</asp:ListItem>
<asp:ListItem>Woodley Park-Zoo/Adams Morgan</asp:ListItem>
<asp:ListItem>Dupont Circle</asp:ListItem><asp:ListItem>Farragut North</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem><asp:ListItem>Gallery Pl-Chinatown</asp:ListItem>
<asp:ListItem>Judiciary Square</asp:ListItem><asp:ListItem>Union Station</asp:ListItem>
<asp:ListItem>NoMa-Gallaudet U</asp:ListItem><asp:ListItem>New Carrolton</asp:ListItem>
<asp:ListItem>Rhode Island Ave-Brentwood</asp:ListItem>
<asp:ListItem>Brookland-CUA</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Takoma</asp:ListItem><asp:ListItem>Silver Spring</asp:ListItem>
<asp:ListItem>Forest Glen</asp:ListItem><asp:ListItem>Wheaton</asp:ListItem>
<asp:ListItem>Glenmont</asp:ListItem><asp:ListItem>Franconia-Springfield</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlRedLine" Visible="False" runat="server">
<h2>Red Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxRedLine" Height="300px" runat="server">
<asp:ListItem>Shady Grove</asp:ListItem><asp:ListItem>Rockville</asp:ListItem>
<asp:ListItem>Twinbrook</asp:ListItem><asp:ListItem>White Flint</asp:ListItem>
<asp:ListItem>Grosvenor-Strathmore</asp:ListItem><asp:ListItem>Medical Center</asp:ListItem>
<asp:ListItem>Bethesda</asp:ListItem><asp:ListItem>Friendship Heights</asp:ListItem>
<asp:ListItem>Tenleytown-AU</asp:ListItem><asp:ListItem>Van Ness-UDC</asp:ListItem>
<asp:ListItem>Cleveland Park</asp:ListItem>
<asp:ListItem>Woodley Park-Zoo/Adams Morgan</asp:ListItem>
<asp:ListItem>Dupont Circle</asp:ListItem><asp:ListItem>Farragut North</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem><asp:ListItem>Gallery Pl-Chinatown</asp:ListItem>
<asp:ListItem>Judiciary Square</asp:ListItem><asp:ListItem>Union Station</asp:ListItem>
<asp:ListItem>NoMa-Gallaudet U</asp:ListItem><asp:ListItem>Glenmont</asp:ListItem>
<asp:ListItem>Rhode Island Ave-Brentwood</asp:ListItem>
<asp:ListItem>Brookland-CUA</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Takoma</asp:ListItem><asp:ListItem>Silver Spring</asp:ListItem>
<asp:ListItem>Forest Glen</asp:ListItem><asp:ListItem>Wheaton</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlOrangeLine" Visible="False" runat="server">
<h2>Orange Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxOrangeLine" Height="130px" runat="server">
<asp:ListItem>New Carrolton</asp:ListItem><asp:ListItem>Landover</asp:ListItem>
<asp:ListItem>Stadium-Armory</asp:ListItem><asp:ListItem>Eastern Market</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem>
<asp:ListItem>Rosslyn</asp:ListItem><asp:ListItem>Vienna</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlGreenLine" Visible="False" runat="server">
<h2>Green Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxLineGreen" Height="105px" runat="server">
<asp:ListItem>Greenbelt</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Gallery Pl-Chinatown</asp:ListItem><asp:ListItem>Archives</asp:ListItem>
<asp:ListItem>Waterfront</asp:ListItem><asp:ListItem>Branch Ave</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlBlueLine" Visible="False" runat="server">
<h2>Blue Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxBlueLine" Height="105px" runat="server">
<asp:ListItem>Largo Town Center</asp:ListItem><asp:ListItem>Stadium-Armory</asp:ListItem>
<asp:ListItem>Eastern Market</asp:ListItem><asp:ListItem>Metro Center</asp:ListItem>
<asp:ListItem>Rosslyn</asp:ListItem><asp:ListItem>Franconia-Springfield</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
</div>
</form>
</body>
</html>
The expression can produce an integer (a Byte, an SByte, a Short,
a UShort, an Integer, a UInteger, a Long, or a ULongtype). In this case, each Case would deal with one of the numeric outcomes. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<style type="text/css">
#tblPreparation { width: 450px; }
#tblEvaluation { width: 300px; }
#pre-section
{
margin: auto;
width: 455px;
}
#eval-section
{
margin: auto;
width: 255px;
}
.main-title
{
font-size: 0.65cm;
font-weight: bold;
text-align: center;
font-family: Garamond, Georgia, Times New Roman, serif;
}
</style>
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim incomeTax As Double
Dim hourlySalary As Double, timeWorked As Double
Dim grossSalary As Double, netPay As Double
Dim payrollFrequency As Integer
hourlySalary = CDbl(TxtHourlySalary.Text)
timeWorked = CDbl(TxtTimeWorked.Text)
grossSalary = hourlySalary * timeWorked
payrollFrequency = RblPayrollFrequency.SelectedIndex
Select Case payrollFrequency
Case 0
incomeTax = 99.1 + (grossSalary * 0.25)
Case 1
incomeTax = 35.5 + (grossSalary * 0.15)
Case 2
incomeTax = 38.4 + (grossSalary * 0.15)
Case 3
incomeTax = 76.8 + (grossSalary * 0.15)
End Select
netPay = grossSalary - incomeTax
LblEmployeeName.Text = TxtEmployeeName.Text
LblHourlySalary.Text = TxtHourlySalary.Text
LblTimeWorked.Text = TxtTimeWorked.Text
LblGrossSalary.Text = formatnumber(grossSalary)
Select Case payrollFrequency
Case 0
LblPayrollFrequency.Text = "Weekly"
Case 1
LblPayrollFrequency.Text = "Biweekly"
Case 2
LblPayrollFrequency.Text = "Semimonthly"
Case 3
LblPayrollFrequency.Text = "Monthly"
End Select
LblIncomeTax.Text = formatnumber(incomeTax)
LblNetPay.Text = formatnumber(netPay)
PnlPreparation.Visible = False
PnlEvaluation.Visible = True
End Sub
</script>
<title>Payroll Preparation</title>
</head>
<body>
<form id="frmPayroll" runat="server">
<div id="pre-section">
<asp:Panel id="PnlPreparation" runat="server">
<p class="main-title">Payroll Preparation</p>
<table id="tblPreparation">
<tr>
<td><p><b>Employee Time Sheet</b></p>
<table>
<tr>
<td style="width: 160px;">Employee Name:</td>
<td>
<asp:TextBox id="TxtEmployeeName"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>Hourly Salary:</td>
<td>
<asp:TextBox id="TxtHourlySalary" runat="server"
Width="50px">0.00</asp:TextBox>
</td>
</tr>
<tr>
<td>Time Worked:</td>
<td>
<asp:TextBox id="TxtTimeWorked" runat="server"
Width="50px">0.00</asp:TextBox>
</td>
</tr>
<tr>
<td> </td>
<td> </td>
</tr>
</table>
</td>
<td style="width: 140px;"><p><b>Payroll Frequency</b></p>
<asp:RadioButtonList id="RblPayrollFrequency" runat="server">
<asp:ListItem>Weekly</asp:ListItem>
<asp:ListItem>Biweekly</asp:ListItem>
<asp:ListItem>Semimonthly</asp:ListItem>
<asp:ListItem>Monthly</asp:ListItem>
</asp:RadioButtonList>
</td>
</tr>
<tr>
<td colspan="2">
<p style="text-align: center">
<asp:Button id="BtnCalculate" runat="server" style="height: 32px;"
Text="Calculate" Width="182px" OnClick="BtnCalculateClick" /></p>
</td>
</tr>
</table>
</asp:Panel>
</div>
<div id="eval-section">
<asp:Panel id="PnlEvaluation" Visible="False" runat="server">
<p class="main-title">Payroll Evaluation</p>
<table id="tblEvaluation" border=5>
<tr>
<td>Employee Name:</td>
<td><asp:Label id="LblEmployeeName"
runat="server"></asp:Label></td>
</tr>
<tr>
<td>Hourly Salary:</td>
<td><asp:Label id="LblHourlySalary" runat="server"
Width="75px">0.00</asp:Label></td>
</tr>
<tr>
<td>Time Worked:</td>
<td><asp:Label id="LblTimeWorked"
runat="server" Width="75px">0.00</asp:Label></td>
</tr>
<tr>
<td>Gross Salary:</td>
<td><asp:Label id="LblGrossSalary"
runat="server" Width="75px">0.00</asp:Label></td>
</tr>
<tr>
<td>Payroll Frequency:</td>
<td><asp:Label id="LblPayrollFrequency"
runat="server" Width="75px">0</asp:Label></td>
</tr>
<tr>
<td>Income Tax:</td>
<td><asp:Label id="LblIncomeTax" runat="server"
Width="75px">0.00</asp:Label>
</td>
</tr>
<tr>
<td>Net Pay:</td>
<td><asp:Label id="LblNetPay" runat="server"
Width="75px">0.00</asp:Label></td>
</tr>
</table>
</asp:Panel>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
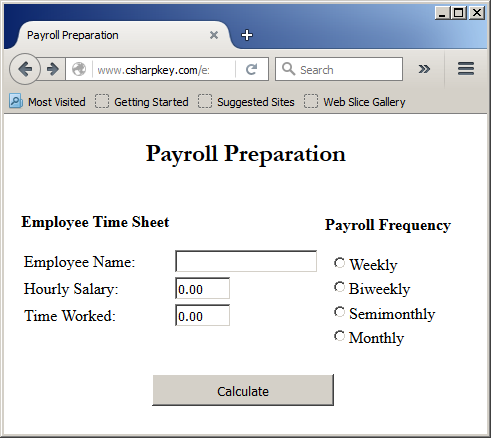
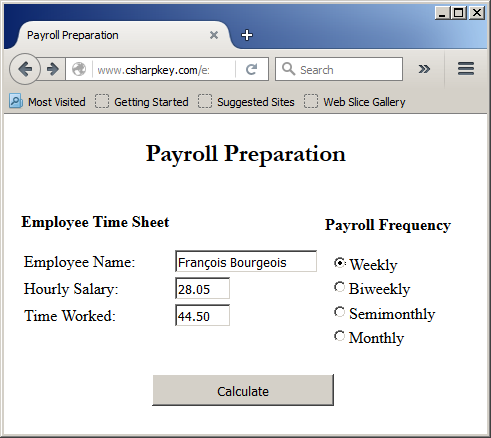

The expression can be an enumeration. In this case, the expression would be the name of the enumeration. Each Case would deal with one of the members of the enumeration.
What Case Else?
If you anticipate that there could be no match
between the expression and one of the values in the cases, you can use a
Case Else statement at the end of the list. The statement would then
look like this:
Select Case expression
Case expression1
statement1
Case expression2
statement2
. . .
Case expression-n
statement-n
Case Else
statement-else
End Select
In this case, the statement after the Case Else
will execute if none of the values of the cases matches the expression.
Combining Cases
When creating a Select Case situation, you may end up using two or more cases that proceed to the same outcome. Here is an
example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Select Case txtMetroLine.Text
Case "R"
pnlAllStations.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = False
pnlRedLine.Visible = True
Case "Green"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = True
Case "Blue"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = True
Case "Red"
pnlAllStations.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = False
pnlRedLine.Visible = True
Case "G"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = True
Case "Orange"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = False
pnlOrangeLine.Visible = True
Case "B"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = True
Case Else
' "All Stations"
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlAllStations.Visible = True
End Select
End Sub
</script>
<style type="text/css">
#main-title
{
font-size: 1.28em;
font-weight: bold;
text-align: center;
font-family: Georgia, Garamond, Times New Roman, Times, serif;
}
h2 { text-align: center; }
#tblMetroLineSelection { width: 390px; }
#whole
{
margin: auto;
width: 395px;
}
</style>
<title>Metro System</title>
</head>
<body>
<form id="frmMetroSystem" runat="server">
<div id="whole">
<p id="main-title">Metro System</p>
<table id="tblMetroLineSelection" align="center">
<tr>
<td>
<asp:Label id="lblLineLetter" runat="server"
Text="Type the color (Red, Green, etc) of a metro line or simply enter its line letter (R, G, B, etc) or type :"></asp:Label>
</td>
</tr>
<tr>
<td>
<asp:TextBox id="txtMetroLine" runat="server"></asp:TextBox>
<asp:Button id="btnFind" Text="Find Metro Stations"
OnClick="btnFindClick" runat="server"></asp:Button>
</td>
</tr>
</table>
<asp:Panel id="pnlAllStations" runat="server" Visible="True">
<h2>All Stations</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxAllStations" Height="400px" style="margin: 0 auto" runat="server">
<asp:ListItem>Shady Grove</asp:ListItem><asp:ListItem>Rockville</asp:ListItem>
<asp:ListItem>Twinbrook</asp:ListItem><asp:ListItem>White Flint</asp:ListItem>
<asp:ListItem>Grosvenor-Strathmore</asp:ListItem><asp:ListItem>Medical Center</asp:ListItem>
<asp:ListItem>Bethesda</asp:ListItem><asp:ListItem>Friendship Heights</asp:ListItem>
<asp:ListItem>Tenleytown-AU</asp:ListItem><asp:ListItem>Van Ness-UDC</asp:ListItem>
<asp:ListItem>Greebelt</asp:ListItem><asp:ListItem>Cleveland Park</asp:ListItem>
<asp:ListItem>Woodley Park-Zoo/Adams Morgan</asp:ListItem>
<asp:ListItem>Dupont Circle</asp:ListItem><asp:ListItem>Farragut North</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem><asp:ListItem>Gallery Pl-Chinatown</asp:ListItem>
<asp:ListItem>Judiciary Square</asp:ListItem><asp:ListItem>Union Station</asp:ListItem>
<asp:ListItem>NoMa-Gallaudet U</asp:ListItem><asp:ListItem>New Carrolton</asp:ListItem>
<asp:ListItem>Rhode Island Ave-Brentwood</asp:ListItem>
<asp:ListItem>Brookland-CUA</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Takoma</asp:ListItem><asp:ListItem>Silver Spring</asp:ListItem>
<asp:ListItem>Forest Glen</asp:ListItem><asp:ListItem>Wheaton</asp:ListItem>
<asp:ListItem>Glenmont</asp:ListItem><asp:ListItem>Franconia-Springfield</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlRedLine" Visible="False" runat="server">
<h2>Red Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxRedLine" Height="300px" runat="server">
<asp:ListItem>Shady Grove</asp:ListItem><asp:ListItem>Rockville</asp:ListItem>
<asp:ListItem>Twinbrook</asp:ListItem><asp:ListItem>White Flint</asp:ListItem>
<asp:ListItem>Grosvenor-Strathmore</asp:ListItem><asp:ListItem>Medical Center</asp:ListItem>
<asp:ListItem>Bethesda</asp:ListItem><asp:ListItem>Friendship Heights</asp:ListItem>
<asp:ListItem>Tenleytown-AU</asp:ListItem><asp:ListItem>Van Ness-UDC</asp:ListItem>
<asp:ListItem>Cleveland Park</asp:ListItem>
<asp:ListItem>Woodley Park-Zoo/Adams Morgan</asp:ListItem>
<asp:ListItem>Dupont Circle</asp:ListItem><asp:ListItem>Farragut North</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem><asp:ListItem>Gallery Pl-Chinatown</asp:ListItem>
<asp:ListItem>Judiciary Square</asp:ListItem><asp:ListItem>Union Station</asp:ListItem>
<asp:ListItem>NoMa-Gallaudet U</asp:ListItem><asp:ListItem>Glenmont</asp:ListItem>
<asp:ListItem>Rhode Island Ave-Brentwood</asp:ListItem>
<asp:ListItem>Brookland-CUA</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Takoma</asp:ListItem><asp:ListItem>Silver Spring</asp:ListItem>
<asp:ListItem>Forest Glen</asp:ListItem><asp:ListItem>Wheaton</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlOrangeLine" Visible="False" runat="server">
<h2>Orange Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxOrangeLine" Height="130px" runat="server">
<asp:ListItem>New Carrolton</asp:ListItem><asp:ListItem>Landover</asp:ListItem>
<asp:ListItem>Stadium-Armory</asp:ListItem><asp:ListItem>Eastern Market</asp:ListItem>
<asp:ListItem>Metro Center</asp:ListItem>
<asp:ListItem>Rosslyn</asp:ListItem><asp:ListItem>Vienna</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlGreenLine" Visible="False" runat="server">
<h2>Green Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxLineGreen" Height="105px" runat="server">
<asp:ListItem>Greenbelt</asp:ListItem><asp:ListItem>Fort Totten</asp:ListItem>
<asp:ListItem>Gallery Pl-Chinatown</asp:ListItem><asp:ListItem>Archives</asp:ListItem>
<asp:ListItem>Waterfront</asp:ListItem><asp:ListItem>Branch Ave</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
<asp:Panel id="pnlBlueLine" Visible="False" runat="server">
<h2>Blue Line</h2>
<table align="center"><tr><td>
<asp:ListBox ID="lbxBlueLine" Height="105px" runat="server">
<asp:ListItem>Largo Town Center</asp:ListItem><asp:ListItem>Stadium-Armory</asp:ListItem>
<asp:ListItem>Eastern Market</asp:ListItem><asp:ListItem>Metro Center</asp:ListItem>
<asp:ListItem>Rosslyn</asp:ListItem><asp:ListItem>Franconia-Springfield</asp:ListItem>
</asp:ListBox>
</td></tr></table>
</asp:Panel>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
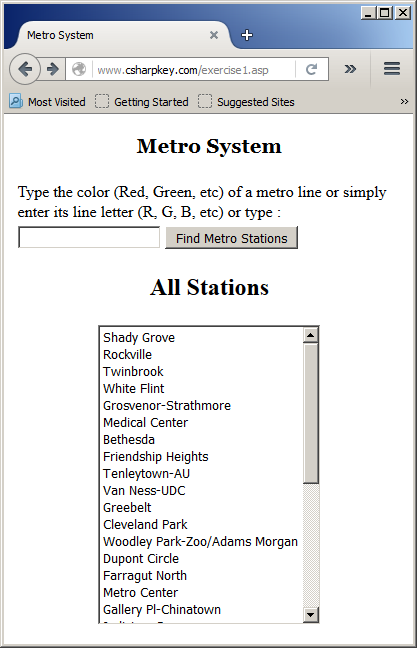
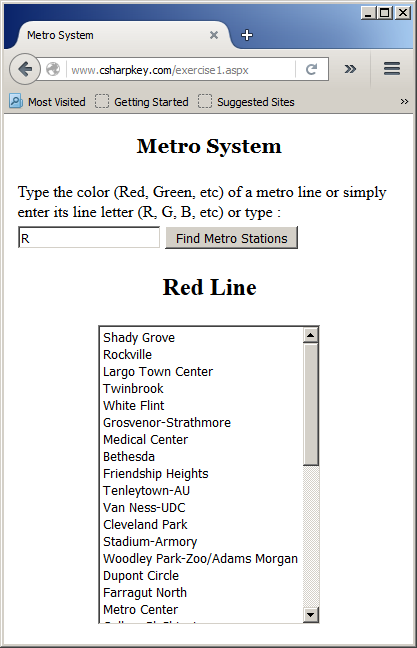
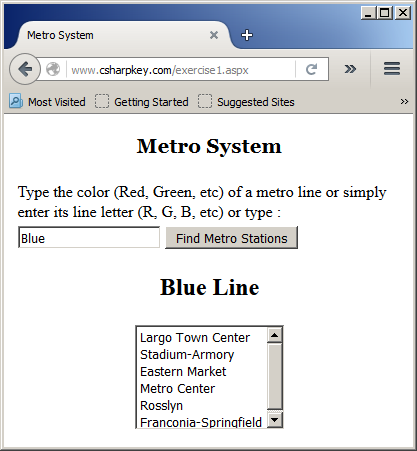
If you have different values for cases and those values are similar outcomes, you can combine those cases. To do this, on the right side of the Case
keyword, type each but separate them with commas. Here are examples:
<script runat="server">
Sub btnFindClick(ByVal sender As Object, ByVal e As EventArgs)
Select Case txtMetroLine.Text
Case "R", "Red"
pnlAllStations.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = False
pnlRedLine.Visible = True
Case "G", "Green"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = True
Case "B", "Blue"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlBlueLine.Visible = True
Case "O", "Orange"
pnlAllStations.Visible = False
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlGreenLine.Visible = False
pnlOrangeLine.Visible = True
Case Else
' "All Stations"
pnlRedLine.Visible = False
pnlBlueLine.Visible = False
pnlOrangeLine.Visible = False
pnlGreenLine.Visible = False
pnlAllStations.Visible = True
End Select
End Sub
</script>
Applying a Range of Values for a Case
You can use a range of values for a case. To do this, on the right side of Case,
enter the lower value, followed by To, followed by the higher value.
Here is an example:
<script runat="server">
Sub Find()
Dim nbr As Integer
nbr = 24
Select Case nbr
Case 0 To 17
Response.Write("Teen")
Case 18 To 55
Response.Write("Adult")
Case Else
Response.Write("Senior")
End Select
End Sub
End Sub
</script>
Checking Whether a Case Value IS
Instead of specifying the exact value for a case, you can check whether the value
of the expression responds to a Boolean expression instead of an exact value. To create
it, you use the Is keyword with the following formula:
Is operator value
You start with the Is keyword. It is followed by a
Boolean operator (=, <>, <, <=, >, or >=). On the right side of the boolean operator, type the desired value. Here
are examples:
<script runat="server">
Sub Find()
Dim Number As Short
Number = -448
Select Case Number
Case Is < 0
Response.Write("The number is negative")
Case Is > 0
Response.Write("The number is positive")
Case Else
Response.Write("0",
MsgBoxStyle.OkOnly Or MsgBoxStyle.Information,
"Conditional Selections")
End Select
End Sub
</script>
Although we used a natural number here, you can use any
appropriate logical comparison that can produce a True or a False result. You
can also combine it with the other alternatives we saw previously, such as
separating the expressions of a case with commas.
Select...Case and Conditional Built-In Functions
To functionaly apply select cases, the Visual Basic language provides a function named Choose
that can check a condition and take an action. The Choose() function is
presented as follows:
Function Choose(ByVal Index As Double,
many parameters) As Object
This function takes two required arguments. The first
argument is equivalent to the expression of our Select Case
formula. The first argument must be a number (a Byte, an
SByte, a Short, UShort, an Integer, a UInteger, a Long, a ULong,
a Single, a Double, or a Decimal value). In place of the Case sections, for the second argument, provide the equivalent expression-x
as a list of values. The values are separated by commas. Here is an example:
Choose(Number, "Teen", "Adult", "Senior")
As mentioned already, the values of the second argument are
provided as a list. Each member of the list uses an index. The first member of the list, which is the second argument of this
function, has an index of 1. The second value of the argument, which would be the third
argument of the function, has an index of 2. You can continue adding the values
of the second argument as you see fit.
When the Choose() function has been called, it
returns a value of type Object. You can retrieve that value, store it in
a variable and use it as you see fit.
Options on Using Conditional Selections
Nesting a Conditional Statement
A conditional statement can be created in the body of a Case
statement. In fact, a Select statement can be created in a Case or a Case Else
section. This is referred to as nesting.
Conditional Selections and Conditional Statements
A conditional statement can be created or nested in a case of a conditional selection.
Here are examples of nesting If...ElseIf...Else conditional statements in Select Cases:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim withheldAmount As Double
Dim allowanceRate As Double
Dim salary As Double
Dim exemptions As Double
Dim withholdingAllowances As Double
Dim taxableGrossWages As Double
allowanceRate = 76.9
salary = CDbl(txtSalary.Text)
exemptions = CDbl(txtExemptions.Text)
withholdingAllowances = allowanceRate * exemptions
taxableGrossWages = salary - withholdingAllowances
Select Case ddlMaritalsStatus.SelectedItem.Text
Case "Single"
If taxableGrossWages <= 44.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 44.0) And (taxableGrossWages <= 222.0) Then
withheldAmount = (taxableGrossWages - 44.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 222.0) And (taxableGrossWages <= 764.0) Then
withheldAmount = 17.8 + ((taxableGrossWages - 222.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 764.0) And (taxableGrossWages <= 1789.0) Then
withheldAmount = 99.1 + ((taxableGrossWages - 764.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 1789.0) And (taxableGrossWages <= 3685.0) Then
withheldAmount = 355.05 + ((taxableGrossWages - 1789.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 3685.0) And (taxableGrossWages <= 7958.0) Then
withheldAmount = 886.23 + ((taxableGrossWages - 3685.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 7958.0) And (taxableGrossWages <= 7990.0) Then
withheldAmount = 2296.32 + ((taxableGrossWages - 7958.0) * 35.0 / 100.0)
Else
withheldAmount = 2307.52 + ((taxableGrossWages - 7990.0) * 39.6 / 100.0)
End If
Case "Married"
If taxableGrossWages <= 165.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 165.0) And (taxableGrossWages <= 520.0) Then
withheldAmount = (taxableGrossWages - 165.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 520.0) And (taxableGrossWages <= 1606.0) Then
withheldAmount = 35.5 + ((taxableGrossWages - 520.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 1606.0) And (taxableGrossWages <= 3073.0) Then
withheldAmount = 198.4 + ((taxableGrossWages - 1606.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 3073.0) And (taxableGrossWages <= 4597.0) Then
withheldAmount = 565.15 + ((taxableGrossWages - 3073.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 4597.0) And (taxableGrossWages <= 8079.0) Then
withheldAmount = 991.87 + ((taxableGrossWages - 4597.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 8079.0) And (taxableGrossWages <= 9105.0) Then
withheldAmount = 2140.93 + ((taxableGrossWages - 8079.0) * 35.0 / 100.0)
Else
withheldAmount = 2500.03 + ((taxableGrossWages - 9105.0) * 39.6 / 100.0)
End If
End Select
txtAllowances.Text = FormatNumber(withholdingAllowances)
txtTaxableGrossWages.Text = FormatNumber(taxableGrossWages)
txtFederalIncomeTax.Text = FormatNumber(withheldAmount)
End Sub
</script>
<title>Federal Withholding Tax</title>
</head>
<body>
<form id="frmWithholding" runat="server">
<div align="center">
<h3>Federal Withholding Tax</h3>
<table>
<tr>
<td style="text-align: left">
<asp:Label id="lblMaritalStatus" runat="server" Text="Marital Status:" /></td>
<td style="text-align: left">
<asp:DropDownList id="ddlMaritalsStatus" Width="90px" runat="server">
<asp:ListItem>Single</asp:ListItem>
<asp:ListItem>Married</asp:ListItem>
</asp:DropDownList></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblSalary" runat="server" Text="Salary:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtSalary" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblExemptions" runat="server" Text="Exemptions:" /></td>
<td style="text-align: left">
<asp:TextBox id="txtExemptions" Width="85px" Text="0" runat="server"></asp:TextBox>
</tr>
<tr>
<td> </td>
<td style="text-align: left">
<asp:Button id="btnCalculate" Width="90px"
text="Calculate"
runat="server" OnClick="btnCalculateClick"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblAllowances" runat="server" Text="Allowances:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtAllowances" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblTaxableGrossWages" runat="server" Text="Taxable Gross Wages:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtTaxableGrossWages" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblFederalIncomeTax" runat="server" Text="Federal Income Tax:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtFederalIncomeTax" Width="85px"
text="0.00" runat="server" /></td>
</tr>
</table>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
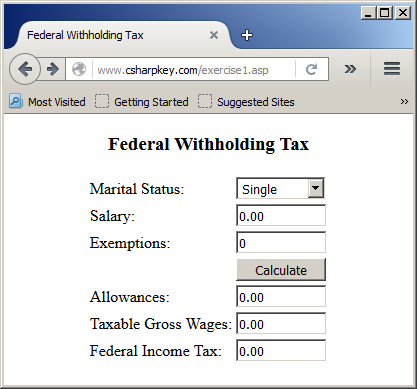
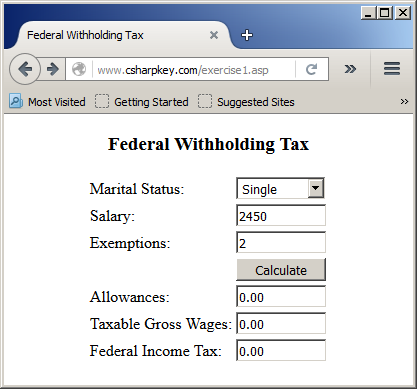
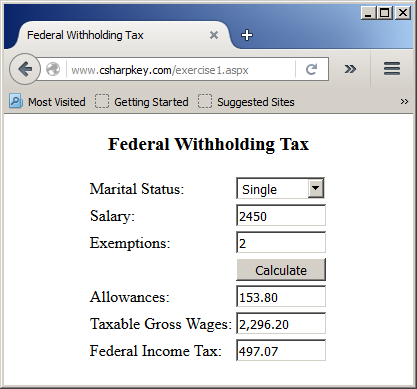
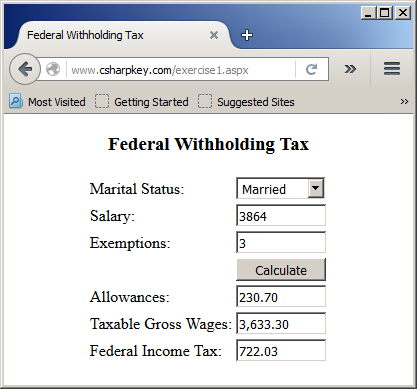
In the same way, you can nest a conditional statement that
itself is nested in a matching pattern that itself is nested in another
conditional statement or in another matching pattern, etc. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub btnCalculateClick(ByVal sender As Object, ByVal e As EventArgs)
Dim withheldAmount As Double
Dim allowanceRate As Double
Dim salary As Double
Dim exemptions As Double
Dim withholdingAllowances As Double
Dim taxableGrossWages As Double
allowanceRate = 76.9
salary = CDbl(txtSalary.Text)
exemptions = CDbl(txtExemptions.Text)
withholdingAllowances = allowanceRate * exemptions
taxableGrossWages = salary - withholdingAllowances
Select Case ddlPayrollFrequency.SelectedItem.Text
Case "Weekly"
Select Case ddlMaritalsStatus.SelectedItem.Text
Case "Single"
If taxableGrossWages <= 44.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 44.0) And (taxableGrossWages <= 222.0) Then
withheldAmount = (taxableGrossWages - 44.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 222.0) And (taxableGrossWages <= 764.0) Then
withheldAmount = 17.8 + ((taxableGrossWages - 222.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 764.0) And (taxableGrossWages <= 1789.0) Then
withheldAmount = 99.1 + ((taxableGrossWages - 764.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 1789.0) And (taxableGrossWages <= 3685.0) Then
withheldAmount = 355.05 + ((taxableGrossWages - 1789.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 3685.0) And (taxableGrossWages <= 7958.0) Then
withheldAmount = 886.23 + ((taxableGrossWages - 3685.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 7958.0) And (taxableGrossWages <= 7990.0) Then
withheldAmount = 2296.32 + ((taxableGrossWages - 7958.0) * 35.0 / 100.0)
Else
withheldAmount = 2307.52 + ((taxableGrossWages - 7990.0) * 39.6 / 100.0)
End If
Case "Married"
If taxableGrossWages <= 165.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 165.0) And (taxableGrossWages <= 520.0) Then
withheldAmount = (taxableGrossWages - 165.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 520.0) And (taxableGrossWages <= 1606.0) Then
withheldAmount = 35.5 + ((taxableGrossWages - 520.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 1606.0) And (taxableGrossWages <= 3073.0) Then
withheldAmount = 198.4 + ((taxableGrossWages - 1606.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 3073.0) And (taxableGrossWages <= 4597.0) Then
withheldAmount = 565.15 + ((taxableGrossWages - 3073.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 4597.0) And (taxableGrossWages <= 8079.0) Then
withheldAmount = 991.87 + ((taxableGrossWages - 4597.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 8079.0) And (taxableGrossWages <= 9105.0) Then
withheldAmount = 2140.93 + ((taxableGrossWages - 8079.0) * 35.0 / 100.0)
Else
withheldAmount = 2500.03 + ((taxableGrossWages - 9105.0) * 39.6 / 100.0)
End If
End Select
Case "Biweekly"
Select Case ddlMaritalsStatus.SelectedItem.Text
Case "Single"
If taxableGrossWages <= 88.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 88.0) And (taxableGrossWages <= 443.0) Then
withheldAmount = (taxableGrossWages - 88.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 443.0) And (taxableGrossWages <= 1529.0) Then
withheldAmount = 35.5 + ((taxableGrossWages - 443.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 1529.0) And (taxableGrossWages <= 3579.0) Then
withheldAmount = 198.4 + ((taxableGrossWages - 1529.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 3579.0) And (taxableGrossWages <= 7369.0) Then
withheldAmount = 710.9 + ((taxableGrossWages - 3579.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 7369.0) And (taxableGrossWages <= 15915.0) Then
withheldAmount = 1772.1 + ((taxableGrossWages - 7369.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 15915.0) And (taxableGrossWages <= 15981.0) Then
withheldAmount = 4592.28 + ((taxableGrossWages - 15915.0) * 35.0 / 100.0)
Else
withheldAmount = 4615.38 + ((taxableGrossWages - 15981.0) * 39.6 / 100.0)
End If
Case "Married"
If taxableGrossWages <= 331.0 Then
withheldAmount = 0.0
ElseIf (taxableGrossWages > 331.0) And (taxableGrossWages <= 1040.0) Then
withheldAmount = (taxableGrossWages - 331.0) * 10.0 / 100.0
ElseIf (taxableGrossWages > 1040.0) And (taxableGrossWages <= 3212.0) Then
withheldAmount = 70.9 + ((taxableGrossWages - 1040.0) * 15.0 / 100.0)
ElseIf (taxableGrossWages > 3212.0) And (taxableGrossWages <= 6146.0) Then
withheldAmount = 396.7 + ((taxableGrossWages - 3212.0) * 25.0 / 100.0)
ElseIf (taxableGrossWages > 6146.0) And (taxableGrossWages <= 9194.0) Then
withheldAmount = 1130.2 + ((taxableGrossWages - 6146.0) * 28.0 / 100.0)
ElseIf (taxableGrossWages > 9194.0) And (taxableGrossWages <= 16158.0) Then
withheldAmount = 1983.64 + ((taxableGrossWages - 9194.0) * 33.0 / 100.0)
ElseIf (taxableGrossWages > 16158.0) And (taxableGrossWages <= 18210.0) Then
withheldAmount = 4281.76 + ((taxableGrossWages - 16158.0) * 35.0 / 100.0)
Else
withheldAmount = 4999.96 + ((taxableGrossWages - 18210.0) * 39.6 / 100.0)
End If
End Select
End Select
txtAllowances.Text = FormatNumber(withholdingAllowances)
txtTaxableGrossWages.Text = FormatNumber(taxableGrossWages)
txtFederalIncomeTax.Text = FormatNumber(withheldAmount)
End Sub
</script>
<title>Federal Withholding Tax</title>
</head>
<body>
<form id="frmWithholding" runat="server">
<div align="center">
<h3>Federal Withholding Tax</h3>
<table>
<tr>
<td style="text-align: left">
Payroll Frequency:</td>
<td style="text-align: left">
<asp:DropDownList id="ddlPayrollFrequency" Width="90px" runat="server">
<asp:ListItem>Weekly</asp:ListItem>
<asp:ListItem>Biweekly</asp:ListItem>
</asp:DropDownList></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblMaritalStatus" runat="server" Text="Marital Status:" /></td>
<td style="text-align: left">
<asp:DropDownList id="ddlMaritalsStatus" Width="90px" runat="server">
<asp:ListItem>Single</asp:ListItem>
<asp:ListItem>Married</asp:ListItem>
</asp:DropDownList></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblSalary" runat="server" Text="Salary:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtSalary" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblExemptions" runat="server" Text="Exemptions:" /></td>
<td style="text-align: left">
<asp:TextBox id="txtExemptions" Width="85px" Text="0" runat="server"></asp:TextBox>
</tr>
<tr>
<td> </td>
<td style="text-align: left">
<asp:Button id="btnCalculate" Width="90px"
text="Calculate"
runat="server" OnClick="btnCalculateClick"></asp:Button></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblAllowances" runat="server" Text="Allowances:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtAllowances" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblTaxableGrossWages" runat="server" Text="Taxable Gross Wages:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtTaxableGrossWages" Width="85px"
text="0.00" runat="server" /></td>
</tr>
<tr>
<td style="text-align: left">
<asp:Label id="lblFederalIncomeTax" runat="server" Text="Federal Income Tax:" />
</td>
<td style="text-align: left">
<asp:TextBox id="txtFederalIncomeTax" Width="85px"
text="0.00" runat="server" /></td>
</tr>
</table>
</div>
</form>
</body>
</html>
Here is an example of using the webpage:
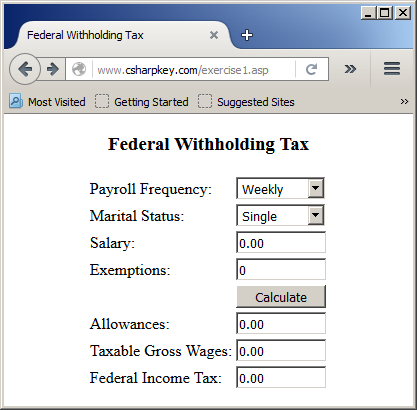
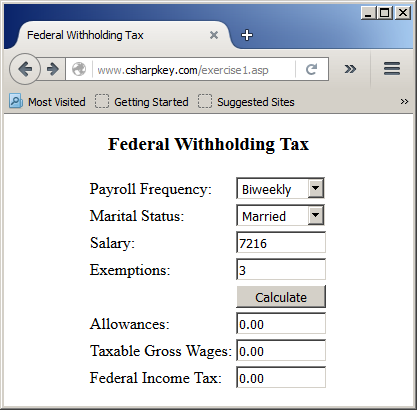
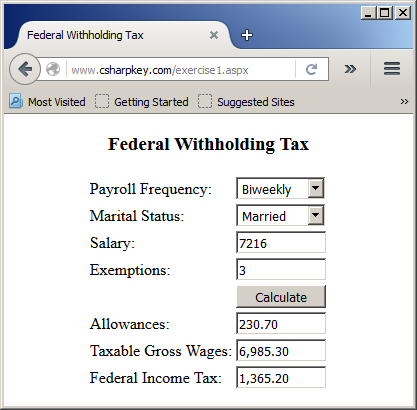
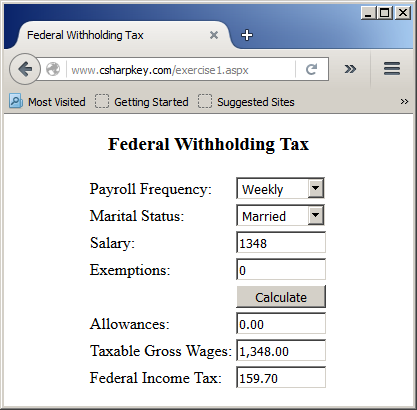
|