Practical
Learning: Creating a GUI Application Using a Text Editor
|
|
- Start Notepad
- Type the following code:
using System;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
using System.Data.SqlClient;
public class Departments : Form
{
private string serverName;
private DataGridView dgvDepartments;
private Label lblDepartmentCode;
private TextBox txtDepartmentCode;
private Label lblDepartment;
private TextBox txtDepartment;
private Button btnAdd;
private GroupBox gbxNewDepartment;
private Button btnClose;
public Departments()
{
InitializeComponent();
}
private void InitializeComponent()
{
dgvDepartments = new DataGridView();
dgvDepartments.TabIndex = 1;
dgvDepartments.Size = new System.Drawing.Size(268, 145);
dgvDepartments.Location = new System.Drawing.Point(12, 12);
dgvDepartments.ColumnHeadersHeightSizeMode = DataGridViewColumnHeadersHeightSizeMode.AutoSize;
lblDepartmentCode = new Label();
lblDepartmentCode.TabIndex = 1;
lblDepartmentCode.AutoSize = true;
lblDepartmentCode.Text = "Department Code:";
lblDepartmentCode.Size = new System.Drawing.Size(93, 13);
lblDepartmentCode.Location = new System.Drawing.Point(15, 27);
txtDepartmentCode = new TextBox();
txtDepartmentCode.TabIndex = 2;
txtDepartmentCode.Size = new System.Drawing.Size(68, 20);
txtDepartmentCode.Location = new System.Drawing.Point(114, 24);
lblDepartment = new Label();
lblDepartment.TabIndex = 3;
lblDepartment.AutoSize = true;
lblDepartment.Text = "Department:";
lblDepartment.Size = new System.Drawing.Size(65, 13);
lblDepartment.Location = new System.Drawing.Point(15, 53);
txtDepartment = new TextBox();
txtDepartment.TabIndex = 4;
txtDepartment.Size = new System.Drawing.Size(141, 20);
txtDepartment.Location = new System.Drawing.Point(114, 50);
btnAdd = new Button();
btnAdd.Text = "Add";
btnAdd.TabIndex = 5;
btnAdd.Location = new System.Drawing.Point(180, 85);
btnAdd.Size = new System.Drawing.Size(75, 23);
btnAdd.UseVisualStyleBackColor = true;
btnAdd.Click += new System.EventHandler(this.btnAddClick);
gbxNewDepartment = new GroupBox();
gbxNewDepartment.Text = "New Department";
gbxNewDepartment.Size = new System.Drawing.Size(268, 124);
gbxNewDepartment.Location = new System.Drawing.Point(12, 168);
gbxNewDepartment.Controls.Add(this.btnAdd);
gbxNewDepartment.Controls.Add(this.txtDepartment);
gbxNewDepartment.Controls.Add(this.lblDepartment);
gbxNewDepartment.Controls.Add(this.txtDepartmentCode);
gbxNewDepartment.Controls.Add(this.lblDepartmentCode);
btnClose = new Button();
btnClose.TabIndex = 3;
btnClose.Text = "Close";
btnClose.Size = new System.Drawing.Size(75, 23);
btnClose.Location = new System.Drawing.Point(192, 304);
btnClose.UseVisualStyleBackColor = true;
btnClose.Click += new System.EventHandler(this.btnCloseClick);
AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
AutoScaleMode = AutoScaleMode.Font;
ClientSize = new System.Drawing.Size(292, 337);
Controls.Add(this.dgvDepartments);
Controls.Add(this.gbxNewDepartment);
Controls.Add(this.btnClose);
StartPosition = FormStartPosition.CenterScreen;
Text = "Departments";
Load += new System.EventHandler(this.DepartmentsLoad);
}
private void ShowDepartments()
{
using (SqlConnection cntExercise =
new SqlConnection("Data Source=" + serverName +
";Database='Exercise1';Integrated Security=yes;"))
{
SqlCommand cmdDepartments =
new SqlCommand("SELECT ALL * FROM Management.Departments;", cntExercise);
SqlDataAdapter odaDepartments = new SqlDataAdapter(cmdDepartments);
DataSet dsDepartments = new DataSet("DepartmentsSet");
cntExercise.Open();
odaDepartments.Fill(dsDepartments);
dgvDepartments.DataSource = dsDepartments.Tables[0];
}
}
private void DepartmentsLoad(object sender, EventArgs e)
{
// Replace EXPRESSION with the name of the computer
// on which Microsoft SQL Server is installed
serverName = "EXPRESSION";
ShowDepartments();
}
private void btnAddClick(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(txtDepartmentCode.Text))
{
MessageBox.Show("You must enter a department code.",
"Exercise", MessageBoxButtons.OK,
MessageBoxIcon.Information);
return;
}
if (string.IsNullOrEmpty(txtDepartment.Text))
{
MessageBox.Show("You must enter the name of a department.",
"Exercise", MessageBoxButtons.OK,
MessageBoxIcon.Information);
return;
}
using (SqlConnection cntExercise =
new SqlConnection("Data Source=" + serverName +
";Database='Exercise1';Integrated Security=yes;"))
{
SqlCommand cmdDepartments =
new SqlCommand("INSERT INTO Management.Departments VALUES(" +
"'" + txtDepartmentCode.Text + "', '" +
txtDepartment.Text + "');",
cntExercise);
cntExercise.Open();
cmdDepartments.ExecuteNonQuery();
txtDepartmentCode.Text = "";
txtDepartment.Text = "";
}
ShowDepartments();
}
private void btnCloseClick(object sender, EventArgs e)
{
Close();
}
}
- To start a new file, on the main menu, click File -> New
- When asked whether you want to save, click Save
- In the top combo box, select the C: drive
- On the Toolbar, click New Folder
- Type ClientServer and press Enter twice (to display
the folder in the top combo box)
- Change the name of the file to "Departments.cs"
- Click Save
- In the empty document, type the following:
using System;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
using System.Data.SqlClient;
public class Employees : Form
{
private string serverName;
private DataGridView dgvEmployees;
private Label lblEmployeeNumber;
private MaskedTextBox txtEmployeeNumber;
private Label lblFirstName;
private TextBox txtFirstName;
private Label lblLastName;
private TextBox txtLastName;
private Label lblDepartment;
private ComboBox cbxDepartments;
private Label lblTitle;
private TextBox txtTitle;
private Button btnAdd;
private GroupBox gbxNewEmployee;
private Button btnClose;
public Employees()
{
InitializeComponent();
}
private void InitializeComponent()
{
dgvEmployees = new DataGridView();
dgvEmployees.TabIndex = 0;
dgvEmployees.Size = new System.Drawing.Size(373, 145);
dgvEmployees.Location = new System.Drawing.Point(12, 12);
dgvEmployees.Anchor = AnchorStyles.Top | AnchorStyles.Bottom |
AnchorStyles.Left | AnchorStyles.Right;
dgvEmployees.ColumnHeadersHeightSizeMode = DataGridViewColumnHeadersHeightSizeMode.AutoSize;
lblEmployeeNumber = new Label();
lblEmployeeNumber.TabIndex = 0;
lblEmployeeNumber.AutoSize = true;
lblEmployeeNumber.Text = "Employee #:";
lblEmployeeNumber.Size = new System.Drawing.Size(66, 13);
lblEmployeeNumber.Location = new System.Drawing.Point(15, 22);
txtEmployeeNumber = new MaskedTextBox();
txtEmployeeNumber.TabIndex = 1;
txtEmployeeNumber.Mask = "000-000";
txtEmployeeNumber.Size = new System.Drawing.Size(53, 20);
txtEmployeeNumber.Location = new System.Drawing.Point(114, 22);
lblFirstName = new Label();
lblFirstName.TabIndex = 2;
lblFirstName.AutoSize = true;
lblFirstName.Text = "First Name:";
lblFirstName.Size = new System.Drawing.Size(60, 13);
lblFirstName.Location = new System.Drawing.Point(15, 53);
txtFirstName = new TextBox();
txtFirstName.TabIndex = 3;
txtFirstName.Size = new System.Drawing.Size(141, 20);
txtFirstName.Location = new System.Drawing.Point(114, 50);
lblLastName = new Label();
lblLastName.TabIndex = 4;
lblLastName.AutoSize = true;
lblLastName.Text = "Last Name:";
lblLastName.Size = new System.Drawing.Size(61, 13);
lblLastName.Location = new System.Drawing.Point(15, 79);
txtLastName = new TextBox();
txtLastName.TabIndex = 5;
txtLastName.Size = new System.Drawing.Size(141, 20);
txtLastName.Location = new System.Drawing.Point(114, 76);
lblDepartment = new Label();
lblDepartment.TabIndex = 6;
lblDepartment.AutoSize = true;
lblDepartment.Text = "Department:";
lblDepartment.Size = new System.Drawing.Size(65, 13);
lblDepartment.Location = new System.Drawing.Point(15, 105);
cbxDepartments = new ComboBox();
cbxDepartments.TabIndex = 7;
cbxDepartments.FormattingEnabled = true;
cbxDepartments.Size = new System.Drawing.Size(141, 21);
cbxDepartments.Location = new System.Drawing.Point(114, 101);
btnAdd = new Button();
btnAdd.Text = "Add";
btnAdd.TabIndex = 10;
btnAdd.UseVisualStyleBackColor = true;
btnAdd.Size = new System.Drawing.Size(75, 23);
btnAdd.Location = new System.Drawing.Point(281, 154);
btnAdd.Click += new System.EventHandler(btnAdd_Click);
lblTitle = new Label();
lblTitle.TabIndex = 8;
lblTitle.Text = "Title:";
lblTitle.AutoSize = true;
lblTitle.Size = new System.Drawing.Size(30, 13);
lblTitle.Location = new System.Drawing.Point(15, 131);
txtTitle = new TextBox();
txtTitle.TabIndex = 9;
txtTitle.Size = new System.Drawing.Size(242, 20);
txtTitle.Location = new System.Drawing.Point(114, 128);
gbxNewEmployee = new GroupBox();
gbxNewEmployee.Text = "New Employee";
gbxNewEmployee.Size = new System.Drawing.Size(373, 193);
gbxNewEmployee.Location = new System.Drawing.Point(12, 168);
gbxNewEmployee.Anchor = AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right;
gbxNewEmployee.Controls.Add(lblEmployeeNumber);
gbxNewEmployee.Controls.Add(txtEmployeeNumber);
gbxNewEmployee.Controls.Add(lblFirstName);
gbxNewEmployee.Controls.Add(txtFirstName);
gbxNewEmployee.Controls.Add(lblLastName);
gbxNewEmployee.Controls.Add(txtLastName);
gbxNewEmployee.Controls.Add(lblDepartment);
gbxNewEmployee.Controls.Add(cbxDepartments);
gbxNewEmployee.Controls.Add(lblTitle);
gbxNewEmployee.Controls.Add(txtTitle);
gbxNewEmployee.Controls.Add(btnAdd);
btnClose = new Button();
btnClose.TabIndex = 2;
btnClose.Text = "Close";
btnClose.UseVisualStyleBackColor = true;
btnClose.Size = new System.Drawing.Size(75, 23);
btnClose.Location = new System.Drawing.Point(293, 376);
btnClose.Anchor = AnchorStyles.Bottom | AnchorStyles.Right;
btnClose.Click += new System.EventHandler(btnClose_Click);
Text = "Employees";
ClientSize = new System.Drawing.Size(401, 412);
Controls.Add(dgvEmployees);
Controls.Add(gbxNewEmployee);
Controls.Add(btnClose);
StartPosition = FormStartPosition.CenterScreen;
Load += new System.EventHandler(Employees_Load);
}
private void ShowEmployees()
{
using (SqlConnection cntExercise =
new SqlConnection("Data Source=" + serverName +
";Database='Exercise1';Integrated Security=yes;"))
{
SqlCommand cmdEmployees =
new SqlCommand("SELECT ALL * FROM Personnel.Employees;", cntExercise);
SqlDataAdapter odaEmployees = new SqlDataAdapter(cmdEmployees);
DataSet dsEmployees = new DataSet("EmployeesSet");
cntExercise.Open();
odaEmployees.Fill(dsEmployees);
dgvEmployees.DataSource = dsEmployees.Tables[0];
}
}
private void Employees_Load(object sender, EventArgs e)
{
// Replace EXPRESSION with the name of the computer
// on which Microsoft SQL Server is installed
serverName = "EXPRESSION";
ShowEmployees();
using (SqlConnection cntExercise =
new SqlConnection("Data Source=" + serverName +
";Database='Exercise1';Integrated Security=yes;"))
{
SqlCommand cmdDepartments =
new SqlCommand("SELECT ALL * FROM Management.Departments;", cntExercise);
cntExercise.Open();
SqlDataReader rdrDepartment = cmdDepartments.ExecuteReader();
while(rdrDepartment.Read())
cbxDepartments.Items.Add(rdrDepartment.GetString(0) + " - " + rdrDepartment.GetString(1));
}
}
private void btnAdd_Click(object sender, EventArgs e)
{
if (string.IsNullOrEmpty( txtEmployeeNumber.Text))
{
MessageBox.Show("You must enter an employee number.",
"Exercise", MessageBoxButtons.OK,
MessageBoxIcon.Information);
return;
}
if (string.IsNullOrEmpty(txtLastName.Text))
{
MessageBox.Show("You must enter thelast name of the employee.",
"Exercise", MessageBoxButtons.OK,
MessageBoxIcon.Information);
return;
}
using (SqlConnection cntExercise =
new SqlConnection("Data Source=" + serverName +
";Database='Exercise1';Integrated Security=yes;"))
{
SqlCommand cmdDepartments =
new SqlCommand("INSERT INTO Personnel.Employees VALUES(" +
"'" + txtEmployeeNumber.Text + "', '" +
txtFirstName.Text + "', '" +
txtLastName.Text + "', '" +
cbxDepartments.Text.Substring(0, 5) + "', '" +
txtTitle.Text + "');",
cntExercise);
cntExercise.Open();
cmdDepartments.ExecuteNonQuery();
txtEmployeeNumber.Text = "";
txtFirstName.Text = "";
txtLastName.Text = "";
cbxDepartments.Text = "";
txtTitle.Text = "";
}
ShowEmployees();
}
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
}
- To create a new file, on the main menu, click File -> New
- When asked whether you want to save, click Save
- Make sure the top combo box displays ClientServer.
Set the name
of the file to "Employees.cs"
- Click Save
- In the empty document, type the following:
using System;
using System.Data;
using System.Drawing;
using System.Windows.Forms;
public class Exercise : Form
{
private Button btnDepartments;
private Button btnEmployees;
private Button btnClose;
public Exercise()
{
InitializeComponent();
}
private void InitializeComponent()
{
btnDepartments = new Button();
btnDepartments.TabIndex = 0;
btnDepartments.Text = "Departments...";
btnDepartments.UseVisualStyleBackColor = true;
btnDepartments.Size = new System.Drawing.Size(259, 65);
btnDepartments.Location = new System.Drawing.Point(21, 12);
btnDepartments.Font = new System.Drawing.Font("Century Schoolbook",
21.75F,
System.Drawing.FontStyle.Bold,
System.Drawing.GraphicsUnit.Point,
0);
btnDepartments.Click += new System.EventHandler(this.btnDepartments_Click);
btnEmployees = new Button();
btnEmployees.TabIndex = 1;
btnEmployees.Text = "Employees...";
btnEmployees.UseVisualStyleBackColor = true;
btnEmployees.Size = new System.Drawing.Size(259, 65);
btnEmployees.Location = new System.Drawing.Point(21, 94);
btnEmployees.Font = new System.Drawing.Font("Century Schoolbook",
21.75F,
System.Drawing.FontStyle.Bold,
System.Drawing.GraphicsUnit.Point,
0);
btnEmployees.Click += new System.EventHandler(this.btnEmployees_Click);
btnClose = new Button();
btnClose.TabIndex = 2;
btnClose.Text = "Close";
btnClose.UseVisualStyleBackColor = true;
btnClose.Size = new System.Drawing.Size(259, 65);
btnClose.Location = new System.Drawing.Point(21, 176);
btnClose.Font = new System.Drawing.Font("Century Schoolbook",
21.75F,
System.Drawing.FontStyle.Bold,
System.Drawing.GraphicsUnit.Point,
0);
btnClose.Click += new System.EventHandler(this.btnClose_Click);
ClientSize = new System.Drawing.Size(301, 263);
Controls.Add(this.btnClose);
Controls.Add(this.btnEmployees);
Controls.Add(this.btnDepartments);
StartPosition = FormStartPosition.CenterScreen;
Text = "Client/Server Exercise";
}
private void btnDepartments_Click(object sender, EventArgs e)
{
Departments depts = new Departments();
depts.Show();
}
private void btnEmployees_Click(object sender, EventArgs e)
{
Employees empls = new Employees();
empls.Show();
}
private void btnClose_Click(object sender, EventArgs e)
{
Close();
}
}
public class Program
{
[STAThread]
static int Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Exercise());
return 0;
}
}
- To close Notepad, on the main menu, click File -> Exit
- When asked whether you want to save, click Save
- Set the file name to "Exercise.cs"
- Click Save
- To open the Command Prompt (Start -> All Programs -> Accessories ->
Command Prompt)
- To switch to the root, type CD\ and press Enter
- To switch to the folder that contains the files of this project,
type CD ClientServer and press Enter
- To build and compile the project, type the following:
C:\Windows\Microsoft.NET\Framework\v4.0.30319\csc /r:System.dll
/r:System.Drawing.dll /r:System.Data.dll /r:System.Xml.dll /r:System.Windows.For
ms.dll /target:winexe Departments.cs Employees.cs Exercise.cs
- Press Enter
- To execute, type Exercise and press Enter
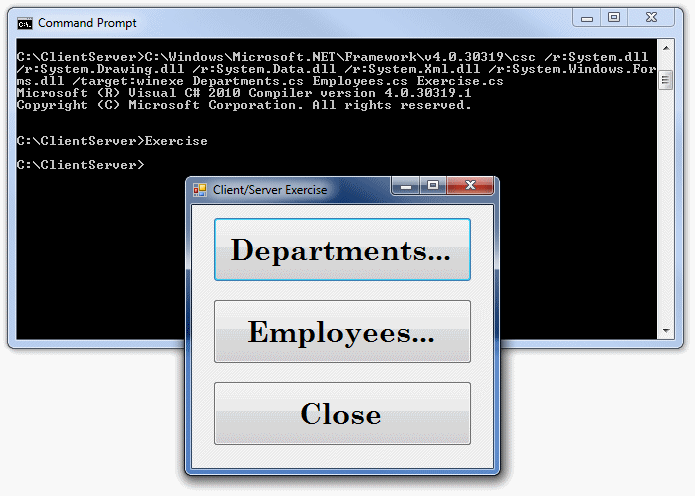
You can copy the executable that was created, named
Exercise.exe, and paste it in the computers that need to access the database.
Practical
Learning: Using the Application
|
|
- Click Departments
- Create a few deparments as follows:
Department Code |
Department |
HMNRS |
Human Resources |
ITECM |
Information Technology and Management |
ACCNT |
Accounting |
- Close the Departments form
- Click Employees
- Create a few employees as follows:
Employee # |
First Name |
Last Name |
Department |
Title |
270-284 |
Paul |
Motto |
HMNRS |
General Manager |
579-485 |
Joan |
Stohl |
ITECM |
Webmaster |
852-662 |
Thomas |
Pratt |
HMNRS |
Intern |
279-484 |
Martine |
Schlamme |
ACCNT |
Accountant |
- Close the forms
|
|