Practical Learning: Introducing Buttons
|
|
- Start Microsoft Visual Basic and create a Windows Application named
Algebra1
- In the Solution Explorer, right-click Form1.vb and click Rename
- Type Exercise.vb and press Enter
- Click the body of the form to make sure it is selected.
In the Properties window, change the following characteristics
FormBorderStyle: FixedDialog
Text: Factorial, Permutation, and Combination
Size: 304, 235
StartPosition: CenterScreen
MaximizeBox: False
MinimizeBox: False
- In the Containers section of the Toolbox, click TabControl and click the
form
- On the form, right-click the right side of tabPage2 and click Add Page
- Design
the form as follows:
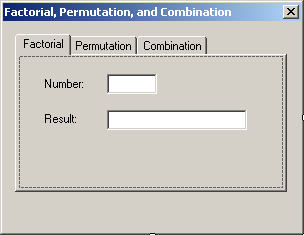 |
Control |
Text |
Name |
Additional Properties |
TabControl |
|
tclAlgebra |
HotTrack: True
Location: 12, 12
Size: 304, 235 |
TabPage |
Factorial |
tabFactorial |
|
Label |
Number: |
|
Location: 22, 21 |
TextBox |
|
txtNumber |
TextAlign: Right
Location: 88, 18
Size: 50, 20 |
Label |
Result: |
|
Location: 22, 56 |
TextBox |
|
txtFactorial |
TextAlign: Right
Location: 88, 54
Size: 140, 20 |
|
|
Control |
Text |
Name |
Location |
Size |
TabPage |
Permutation |
tabPermutation |
|
|
Label |
n: |
|
22, 21 |
|
TextBox |
|
txtPermutationN |
88, 18 |
50, 20 |
Label |
r: |
|
22, 56 |
|
TextBox |
|
txtPermutationR |
88, 54 |
50, 20 |
Label |
P(n, r): |
|
22, 92 |
|
TextBox |
|
txtPermutation |
88, 90 |
140, 20 |
|
|
Control |
Text |
Name |
Location |
Size |
TabPage |
Combination |
tabCombination |
|
|
Label |
n: |
|
22, 21 |
|
TextBox |
|
txtCombinationN |
88, 18 |
50, 20 |
Label |
r: |
|
22, 56 |
|
TextBox |
|
txtCombinationR |
88, 54 |
50, 20 |
Label |
C(n, r): |
|
22, 92 |
|
TextBox |
|
txtCombination |
88, 90 |
140, 20 |
|
- Right-click the form and click View Code
- Create the following functions:
Public Class Exercise
Private Function Factorial(ByVal x As Long) As Long
If x <= 1 Then
Return 1
Else
Factorial = x * Factorial(x - 1)
End If
End Function
Private Function Permutation(ByVal n As Long, ByVal r As Long) As Long
If r = 0 Then Permutation = 0
If n = 0 Then Permutation = 0
If (r >= 0) And (r <= n) Then
Permutation = Factorial(n) / Factorial(n - r)
Else
Permutation = 0
End If
End Function
Private Function Combinatorial(ByVal a As Long, ByVal b As Long) As Long
If a <= 1 Then Combinatorial = 1
Combinatorial = Factorial(a) / (Factorial(b) * Factorial(a - b))
End Function
End Class
- Click the Exercise.vb [Design] tab
- In the combo box on top of the Properties window, select tabFactorial
- From the Common Controls section of the Toolbox, click Button and click
on the right side of the top text box
- Access each tab page and add a button to it
- Add a button to the form under the tab control
- Complete the design of the form as follows:
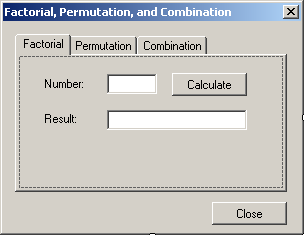 |
Control |
Text |
Name |
Button |
Calculate |
btnCalcFactorial |
Button |
Close |
btnClose |
|
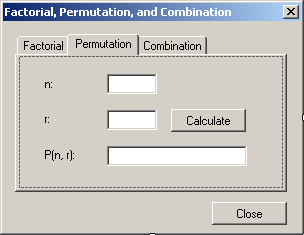 |
Control |
Text |
Name |
Button |
Calculate |
btnCalcPermutation |
|
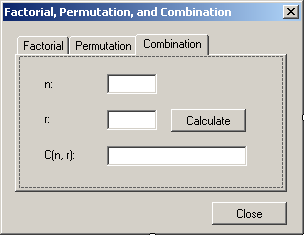 |
Control |
Text |
Name |
Button |
Calculate |
btnCalcCombination |
|
- Right-click the form and click View Code
- In the Class Name combo box, select btnCalc Factorial
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCalcFactorial_Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCalcFactorial.Click
Dim number As Long
Dim Result As Long
Try
number = CLng(txtFactNumber.Text)
Result = Factorial(number)
txtFactorial.Text = Result.ToString()
Catch ex As Exception
MsgBox("Invalid Number")
End Try
End Sub
- In the Class Name combo box, select btnCalc Permutation
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCalcPermutation_Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCalcPermutation.Click
Dim n As Long
Dim r As Long
Dim Result As Long
Try
n = CLng(txtPermutationN.Text)
Catch ex As Exception
MsgBox("Invalid Number")
End Try
Try
r = CLng(txtPermutationR.Text)
Result = Permutation(n, r)
txtPermutation.Text = Result.ToString()
Catch ex As Exception
MsgBox("Invalid Number")
End Try
End Sub
- In the Class Name combo box, select btnCalc Combination
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnCalcCombination_Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnCalcCombination.Click
Dim n As Long
Dim r As Long
Dim Result As Long
Try
n = CLng(txtCombinationN.Text)
Catch ex As Exception
MsgBox("Invalid Number")
End Try
Try
r = CLng(txtCombinationR.Text)
Result = Combinatorial(n, r)
txtCombination.Text = Result.ToString()
Catch ex As Exception
MsgBox("Invalid Number")
End Try
End Sub
- In the Class Name combo box, select btn Close
- In the Method Name combo box, select Click and implement the event as
follows:
Private Sub btnClose_Click(ByVal sender As Object,
ByVal e As System.EventArgs)
Handles btnClose.Click
End
End Sub
- Execute the application to test the calculations
- Close the form and return to your programming environment
Download
|
|