 |
Introduction to Databases: A Database as an Array of Values |
|
|
A database is a list of values. The values can be organized to make
it easy to retrieve and optionally manipulate them. A computer database is a
list of values that are stored in a one or more computers, usually as one or more
files.
The values can then be accessed when needed. Probably the most fundamental type
of list of values can be created, and managed, as an array.
|
Practical
Learning: Introducing Databases
|
|
- Start Microsoft Visual C++
- Create a Windows Forms Application named YugoNationalBank1
Before creating an array, you must decide what type of
values each element of the array would be. A simple array can be made of
primitive types of values. Here is an example:
#pragma once
#include <windows.h>
#using <System.dll>
#using <System.Drawing.dll>
#using <System.Windows.Forms.dll>
using namespace System;
using namespace System::Drawing;
using namespace System::Windows::Forms;
public ref class CExercise : public Form
{
private:
ListBox ^ lbxNumbers;
void FormLoader(Object ^ sender, EventArgs ^ e);
void InitializeComponents();
public:
CExercise();
};
CExercise::CExercise()
{
InitializeComponents();
}
void CExercise::InitializeComponents()
{
lbxNumbers = gcnew ListBox();
lbxNumbers->Location = Point(10, 10);
Controls->Add(lbxNumbers);
Text = L"Numbers";
StartPosition = FormStartPosition::CenterScreen;
Load += gcnew EventHandler(this, &CExercise::FormLoader);
}
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
double Numbers[] = { 12.44, 525.38, 6.28, 2448.32, 632.04 };
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise());
return 0;
}
Once the array has been created, you can access each one of
its elements using the [] operator. Here is an example:
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
double Numbers[] = { 12.44, 525.38, 6.28, 2448.32, 632.04 };
for (int i = 0; i < 5; i++)
lbxNumbers->Items->Add(Numbers[i]);
}
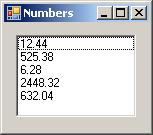
An array can also be made of elements that are each a
composite type. That is, each element can be of a class type. Of course, you
must have a class first. You can use one of the many built-in classes of the
.NET Framework or you can create your own class. Here is an example:
Person.h |
#pragma once
using namespace System;
public ref class CPerson
{
public:
int PersonID;
String ^ FirstName;
String ^ LastName;
String ^ Gender;
public:
CPerson(void);
CPerson(int ID, String ^ fn, String ^ ln, String ^ gdr);
};
|
Person.cpp |
#include "StdAfx.h"
#include "Person.h"
CPerson::CPerson(void)
{
}
CPerson::CPerson(int ID, String ^ fn, String ^ ln, String ^ gdr)
{
PersonID = ID;
FirstName = fn;
LastName = ln;
Gender = gdr;
}
|
After creating the class, you can then use it as the type of
the array. Here is an example:
#pragma once
#include "Person.h"
namespace Arrays
{
. . . No Change
#pragma region Windows Form Designer generated code
. . . No Change
#pragma endregion
private: System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(8);
People[0] = gcnew CPerson(72947, L"Paulette",
L"Cranston", L"Female");
People[1] = gcnew CPerson(70854, L"Harry",
L"Kumar", L"Male");
People[2] = gcnew CPerson(27947, L"Jules",
L"Davidson", L"Male");
People[3] = gcnew CPerson(62835, L"Leslie",
L"Harrington", L"Unknown");
People[4] = gcnew CPerson(92958, L"Ernest",
L"Colson", L"Male");
People[5] = gcnew CPerson(91749, L"Patricia",
L"Katts", L"Female");
People[6] = gcnew CPerson(29749, L"Patrice",
L"Abanda", L"Unknown");
People[7] = gcnew CPerson(24739, L"Frank",
L"Thomasson", L"Male");
for(int i = 0; i < 8; i++)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(People[i]->PersonID->ToString());
lviPerson->SubItems->Add(People[i]->FirstName);
lviPerson->SubItems->Add(People[i]->LastName);
lviPerson->SubItems->Add(People[i]->Gender);
lvwPeople->Items->Add(lviPerson);
}
}
};
}

To assist you with creating or managing arrays, the .NET
Framework provides the Array class. Every array you create is derived
from this class. As a result, all arrays of your program share many
characteristics and they get their foundation from the Array class, which
include its properties and methods. Once you declare an array variable, it
automatically has access to the members of the Array class. For example, instead
of counting the number of elements in an array, you can access the Length
property of the array variable. Here is an example:
System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(8);
People[0] = gcnew CPerson(72947, L"Paulette",
L"Cranston", L"Female");
People[1] = gcnew CPerson(70854, L"Harry",
L"Kumar", L"Male");
People[2] = gcnew CPerson(27947, L"Jules",
L"Davidson", L"Male");
People[3] = gcnew CPerson(62835, L"Leslie",
L"Harrington", L"Unknown");
People[4] = gcnew CPerson(92958, L"Ernest",
L"Colson", L"Male");
People[5] = gcnew CPerson(91749, L"Patricia",
L"Katts", L"Female");
People[6] = gcnew CPerson(29749, L"Patrice",
L"Abanda", L"Unknown");
People[7] = gcnew CPerson(24739, L"Frank",
L"Thomasson", L"Male");
for(int i = 0; i < People->Length; i++)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(People[i]->PersonID->ToString());
lviPerson->SubItems->Add(People[i]->FirstName);
lviPerson->SubItems->Add(People[i]->LastName);
lviPerson->SubItems->Add(People[i]->Gender);
lvwPeople->Items->Add(lviPerson);
}
}
When you declare an array
variable, you must specify the number of elements that the array will contain. Here is an example:
System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(4);
}
After declaring the variable, before using the array, you
must initialize it. Otherwise you would receive an error. When initializing the
array, you can only initialize it with the number of elements you had specified.
To illustrate this, consider the following program:
System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(4);
People[0] = gcnew CPerson(72947, L"Paulette",
L"Cranston", L"Female");
People[1] = gcnew CPerson(70854, L"Harry",
L"Kumar", L"Male");
People[2] = gcnew CPerson(27947, L"Jules",
L"Davidson", L"Male");
People[3] = gcnew CPerson(62835, L"Leslie",
L"Harrington", L"Unknown");
People[4] = gcnew CPerson(92958, L"Ernest",
L"Colson", L"Male");
People[5] = gcnew CPerson(91749, L"Patricia",
L"Katts", L"Female");
People[6] = gcnew CPerson(29749, L"Patrice",
L"Abanda", L"Unknown");
People[7] = gcnew CPerson(24739, L"Frank",
L"Thomasson", L"Male");
for(int i = 0; i < People->Length; i++)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(People[i]->PersonID->ToString());
lviPerson->SubItems->Add(People[i]->FirstName);
lviPerson->SubItems->Add(People[i]->LastName);
lviPerson->SubItems->Add(People[i]->Gender);
lvwPeople->Items->Add(lviPerson);
}
}
Notice that the variable is declared to hold only 4 elements
but the user tries to access a 5th one. This would produce an IndexOutOfRangeExcception
exception:
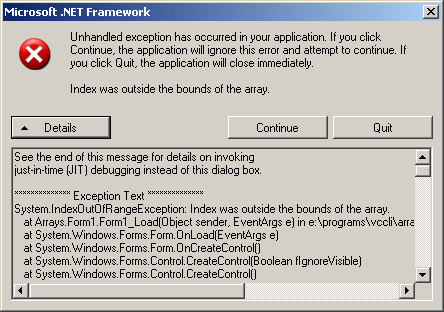
One of the most valuable features of the Array class
is that it allows an array to be "resizable". That is, if you find out
that an array has a size smaller than the number of elements you want to add to
it, you can increase its capacity. To support this, the Array class is equipped
with the static Resize() method. Its syntax is:
public:
generic<typename T>
static void Resize(array<T>^% array, int newSize);
As you can see, this is a generic method that takes two
arguments. The first argument is the name of the array variable that you want to
resize. It must be passed by reference. The second argument is the new size you
want the array to have. Here is an example of calling this method:
System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(4);
People[0] = gcnew CPerson(72947, L"Paulette",
L"Cranston", L"Female");
People[1] = gcnew CPerson(70854, L"Harry",
L"Kumar", L"Male");
People[2] = gcnew CPerson(27947, L"Jules",
L"Davidson", L"Male");
People[3] = gcnew CPerson(62835, L"Leslie",
L"Harrington", L"Unknown");
Array::Resize(People, 8);
People[4] = gcnew CPerson(92958, L"Ernest",
L"Colson", L"Male");
People[5] = gcnew CPerson(91749, L"Patricia",
L"Katts", L"Female");
People[6] = gcnew CPerson(29749, L"Patrice",
L"Abanda", L"Unknown");
People[7] = gcnew CPerson(24739, L"Frank",
L"Thomasson", L"Male");
for(int i = 0; i < People->Length; i++)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(People[i]->PersonID->ToString());
lviPerson->SubItems->Add(People[i]->FirstName);
lviPerson->SubItems->Add(People[i]->LastName);
lviPerson->SubItems->Add(People[i]->Gender);
lvwPeople->Items->Add(lviPerson);
}
}
The advantage of this approach is that you can access the
array from any member of the same class or even from another file of the same
program.
As done previously, you can create an array in a method. A
disadvantage of this approach is that the array can be accessed from only the
method (or event) in which it is created. As an alternative, you can declare an
array as a member of a class. Here is an example:
#pragma once
#include <windows.h>
#include "Person.h"
#using <System.dll>
#using <System.Drawing.dll>
#using <System.Windows.Forms.dll>
using namespace System;
using namespace System::Drawing;
using namespace System::Windows::Forms;
public ref class CExercise : public Form
{
private:
array<CPerson ^> ^ People;
void FormLoader(Object ^ sender, EventArgs ^ e);
void InitializeComponents();
public:
CExercise();
};
CExercise::CExercise()
{
InitializeComponents();
}
void CExercise::InitializeComponents()
{
Text = L"People";
StartPosition = FormStartPosition::CenterScreen;
Load += gcnew EventHandler(this, &CExercise::FormLoader);
}
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise());
return 0;
}
The advantage of this approach is that you can access the
array from any member of the same class or even from another file of the same
program. After declaring the variable, you can initialize it. You can do this in
a constructor or in an event that would be fired before any other event that
would use the array. This type of initialization is usually done in a Load
event of a form. After initializing the array, you can then access in another
method or another event of the form. Here is an example:
#pragma once
#include <windows.h>
#include "Person.h"
#using <System.dll>
#using <System.Drawing.dll>
#using <System.Windows.Forms.dll>
using namespace System;
using namespace System::Drawing;
using namespace System::Windows::Forms;
public ref class CExercise : public Form
{
private:
array<CPerson ^> ^ People;
ListView ^ lvwPeople;
ColumnHeader ^ colIndex;
ColumnHeader ^ colFirstName;
ColumnHeader ^ colLastName;
ColumnHeader ^ colGender;
void FormLoader(Object ^ sender, EventArgs ^ e);
void InitializeComponents();
public:
CExercise();
};
CExercise::CExercise()
{
InitializeComponents();
}
void CExercise::InitializeComponents()
{
Text = L"People";
lvwPeople = gcnew ListView;
lvwPeople->Location = Point(12, 12);
lvwPeople->Width = 290;
lvwPeople->Height = 135;
lvwPeople->View = View::Details;
lvwPeople->FullRowSelect = true;
lvwPeople->GridLines = true;
colIndex = gcnew ColumnHeader();
colIndex->Text = L"Pers ID";
colIndex->Width = 50;
lvwPeople->Columns->Add(colIndex);
colFirstName = gcnew ColumnHeader;
colFirstName->Text = L"First Name";
colFirstName->Width = 80;
lvwPeople->Columns->Add(colFirstName);
colLastName = gcnew ColumnHeader;
colLastName->Text = L"Last Name";
colLastName->Width = 80;
lvwPeople->Columns->Add(colLastName);
colGender = gcnew ColumnHeader;
colGender->Text = L"Gender";
colGender->TextAlign = HorizontalAlignment::Center;
colGender->Width = 70;
lvwPeople->Columns->Add(colGender);
Controls->Add(lvwPeople);
StartPosition = FormStartPosition::CenterScreen;
Width = 320;
Height = 185;
Load += gcnew EventHandler(this, &CExercise::FormLoader);
}
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
People = gcnew array<CPerson ^>(8);
People[0] = gcnew CPerson;
People[0]->PersonID = 72947; People[0]->Gender = L"Female";
People[0]->FirstName = L"Paulette"; People[0]->LastName = L"Cranston";
People[1] = gcnew CPerson;
People[1]->PersonID = 70854; People[1]->Gender = L"Male";
People[1]->FirstName = L"Harry"; People[1]->LastName = L"Kumar";
People[2] = gcnew CPerson;
People[2]->PersonID = 27947; People[2]->Gender = L"Male";
People[2]->FirstName = L"Jules"; People[2]->LastName = L"Davidson";
People[3] = gcnew CPerson;
People[3]->PersonID = 62835; People[3]->Gender = L"Unknown";
People[3]->FirstName = L"Leslie"; People[3]->LastName = L"Harrington";
People[4] = gcnew CPerson;
People[4]->PersonID = 92958; People[4]->Gender = L"Male";
People[4]->FirstName = L"Ernest"; People[4]->LastName = L"Colson";
People[5] = gcnew CPerson;
People[5]->PersonID = 91749; People[5]->Gender = L"Female";
People[5]->FirstName = L"Patricia"; People[5]->LastName = L"Katts";
People[6] = gcnew CPerson;
People[6]->PersonID = 29749; People[6]->Gender = L"Unknown";
People[6]->FirstName = L"Patrice"; People[6]->LastName = L"Abanda";
People[7] = gcnew CPerson;
People[7]->PersonID = 24739; People[7]->Gender = L"Male";
People[7]->FirstName = L"Frank"; People[7]->LastName = L"Thomasson";
for each( CPerson ^ pers in People)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(pers->PersonID->ToString());
lviPerson->SubItems->Add(pers->FirstName);
lviPerson->SubItems->Add(pers->LastName);
String ^ gdr = (String ^)pers->Gender;
lviPerson->SubItems->Add(gdr);
lvwPeople->Items->Add(lviPerson);
}
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise());
return 0;
}
An array can be passed as argument to a method. Here is an
example:
void ArrayInitializer(array<CPerson ^> ^ People)
{
}
In the method, you can use the array as you see fit. For
example you can assign values to the elements of the array. When calling a
method that is passed an array, you can pass the argument by reference so it
would come back with new values. Here are examples:
#pragma once
#include <windows.h>
#include "Person.h"
#using <System.dll>
#using <System.Drawing.dll>
#using <System.Windows.Forms.dll>
using namespace System;
using namespace System::Drawing;
using namespace System::Windows::Forms;
public ref class CExercise : public Form
{
private:
ListView ^ lvwPeople;
ColumnHeader ^ colIndex;
ColumnHeader ^ colFirstName;
ColumnHeader ^ colLastName;
ColumnHeader ^ colGender;
void CExercise::ArrayInitializer(array<CPerson ^> ^ People);
void CExercise::ShowPeople(array<CPerson ^> ^ People);
void FormLoader(Object ^ sender, EventArgs ^ e);
void InitializeComponents();
public:
CExercise();
};
CExercise::CExercise()
{
InitializeComponents();
}
void CExercise::InitializeComponents()
{
Text = L"People";
lvwPeople = gcnew ListView;
lvwPeople->Location = Point(12, 12);
lvwPeople->Width = 290;
lvwPeople->Height = 135;
lvwPeople->View = View::Details;
lvwPeople->FullRowSelect = true;
lvwPeople->GridLines = true;
colIndex = gcnew ColumnHeader();
colIndex->Text = L"Pers ID";
colIndex->Width = 50;
lvwPeople->Columns->Add(colIndex);
colFirstName = gcnew ColumnHeader;
colFirstName->Text = L"First Name";
colFirstName->Width = 80;
lvwPeople->Columns->Add(colFirstName);
colLastName = gcnew ColumnHeader;
colLastName->Text = L"Last Name";
colLastName->Width = 80;
lvwPeople->Columns->Add(colLastName);
colGender = gcnew ColumnHeader;
colGender->Text = L"Gender";
colGender->TextAlign = HorizontalAlignment::Center;
colGender->Width = 70;
lvwPeople->Columns->Add(colGender);
Controls->Add(lvwPeople);
StartPosition = FormStartPosition::CenterScreen;
Width = 320;
Height = 185;
Load += gcnew EventHandler(this, &CExercise::FormLoader);
}
void CExercise::ArrayInitializer(array<CPerson ^> ^ People)
{
People[0] = gcnew CPerson;
People[0]->PersonID = 72947; People[0]->Gender = L"Female";
People[0]->FirstName = L"Paulette"; People[0]->LastName = L"Cranston";
People[1] = gcnew CPerson;
People[1]->PersonID = 70854; People[1]->Gender = L"Male";
People[1]->FirstName = L"Harry"; People[1]->LastName = L"Kumar";
People[2] = gcnew CPerson;
People[2]->PersonID = 27947; People[2]->Gender = L"Male";
People[2]->FirstName = L"Jules"; People[2]->LastName = L"Davidson";
People[3] = gcnew CPerson;
People[3]->PersonID = 62835; People[3]->Gender = L"Unknown";
People[3]->FirstName = L"Leslie"; People[3]->LastName = L"Harrington";
People[4] = gcnew CPerson;
People[4]->PersonID = 92958; People[4]->Gender = L"Male";
People[4]->FirstName = L"Ernest"; People[4]->LastName = L"Colson";
People[5] = gcnew CPerson;
People[5]->PersonID = 91749; People[5]->Gender = L"Female";
People[5]->FirstName = L"Patricia"; People[5]->LastName = L"Katts";
People[6] = gcnew CPerson;
People[6]->PersonID = 29749; People[6]->Gender = L"Unknown";
People[6]->FirstName = L"Patrice"; People[6]->LastName = L"Abanda";
People[7] = gcnew CPerson;
People[7]->PersonID = 24739; People[7]->Gender = L"Male";
People[7]->FirstName = L"Frank"; People[7]->LastName = L"Thomasson";
}
void CExercise::ShowPeople(array<CPerson ^> ^ People)
{
for each( CPerson ^ pers in People)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(pers->PersonID->ToString());
lviPerson->SubItems->Add(pers->FirstName);
lviPerson->SubItems->Add(pers->LastName);
String ^ gdr = (String ^)pers->Gender;
lviPerson->SubItems->Add(gdr);
lvwPeople->Items->Add(lviPerson);
}
}
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(8);
ArrayInitializer(People);
ShowPeople(People);
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise());
return 0;
}
A method can be created to return an array. When creating
the method, on the left side of the name of the method, type the name of the type
of value the method would return, including the square brackets. In the method,
create and initialize an array. Before exiting the method, you must return the
array. Here is an example:
#pragma once
#include <windows.h>
#include "Person.h"
#using <System.dll>
#using <System.Drawing.dll>
#using <System.Windows.Forms.dll>
using namespace System;
using namespace System::Drawing;
using namespace System::Windows::Forms;
public ref class CExercise : public Form
{
private:
ListView ^ lvwPeople;
ColumnHeader ^ colIndex;
ColumnHeader ^ colFirstName;
ColumnHeader ^ colLastName;
ColumnHeader ^ colGender;
array<CPerson ^> ^ CExercise::ArrayInitializer();
void CExercise::ShowPeople(array<CPerson ^> ^ People);
void FormLoader(Object ^ sender, EventArgs ^ e);
void InitializeComponents();
public:
CExercise();
};
CExercise::CExercise()
{
InitializeComponents();
}
void CExercise::InitializeComponents()
{
Text = L"People";
lvwPeople = gcnew ListView;
lvwPeople->Location = Point(12, 12);
lvwPeople->Width = 290;
lvwPeople->Height = 135;
lvwPeople->View = View::Details;
lvwPeople->FullRowSelect = true;
lvwPeople->GridLines = true;
colIndex = gcnew ColumnHeader();
colIndex->Text = L"Pers ID";
colIndex->Width = 50;
lvwPeople->Columns->Add(colIndex);
colFirstName = gcnew ColumnHeader;
colFirstName->Text = L"First Name";
colFirstName->Width = 80;
lvwPeople->Columns->Add(colFirstName);
colLastName = gcnew ColumnHeader;
colLastName->Text = L"Last Name";
colLastName->Width = 80;
lvwPeople->Columns->Add(colLastName);
colGender = gcnew ColumnHeader;
colGender->Text = L"Gender";
colGender->TextAlign = HorizontalAlignment::Center;
colGender->Width = 70;
lvwPeople->Columns->Add(colGender);
Controls->Add(lvwPeople);
StartPosition = FormStartPosition::CenterScreen;
Width = 320;
Height = 185;
Load += gcnew EventHandler(this, &CExercise::FormLoader);
}
array<CPerson ^> ^ CExercise::ArrayInitializer()
{
array<CPerson ^> ^ People = gcnew array<CPerson ^>(8);
People[0] = gcnew CPerson;
People[0]->PersonID = 72947; People[0]->Gender = L"Female";
People[0]->FirstName = L"Paulette"; People[0]->LastName = L"Cranston";
People[1] = gcnew CPerson;
People[1]->PersonID = 70854; People[1]->Gender = L"Male";
People[1]->FirstName = L"Harry"; People[1]->LastName = L"Kumar";
People[2] = gcnew CPerson;
People[2]->PersonID = 27947; People[2]->Gender = L"Male";
People[2]->FirstName = L"Jules"; People[2]->LastName = L"Davidson";
People[3] = gcnew CPerson;
People[3]->PersonID = 62835; People[3]->Gender = L"Unknown";
People[3]->FirstName = L"Leslie"; People[3]->LastName = L"Harrington";
People[4] = gcnew CPerson;
People[4]->PersonID = 92958; People[4]->Gender = L"Male";
People[4]->FirstName = L"Ernest"; People[4]->LastName = L"Colson";
People[5] = gcnew CPerson;
People[5]->PersonID = 91749; People[5]->Gender = L"Female";
People[5]->FirstName = L"Patricia"; People[5]->LastName = L"Katts";
People[6] = gcnew CPerson;
People[6]->PersonID = 29749; People[6]->Gender = L"Unknown";
People[6]->FirstName = L"Patrice"; People[6]->LastName = L"Abanda";
People[7] = gcnew CPerson;
People[7]->PersonID = 24739; People[7]->Gender = L"Male";
People[7]->FirstName = L"Frank"; People[7]->LastName = L"Thomasson";
return People;
}
void CExercise::ShowPeople(array<CPerson ^> ^ People)
{
for each( CPerson ^ pers in People)
{
ListViewItem ^ lviPerson = gcnew ListViewItem(pers->PersonID->ToString());
lviPerson->SubItems->Add(pers->FirstName);
lviPerson->SubItems->Add(pers->LastName);
String ^ gdr = (String ^)pers->Gender;
lviPerson->SubItems->Add(gdr);
lvwPeople->Items->Add(lviPerson);
}
}
void CExercise::FormLoader(Object ^ sender, EventArgs ^ e)
{
array<CPerson ^> ^ People = ArrayInitializer();
ShowPeople(People);
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise());
return 0;
}
|
|