 |
Introduction to Databases: Operations on an Array |
|
|
Because an array is primarily a series of objects or
values, there are various pieces of information you would get interested to get
from it. Typical operations include:
|
- Adding elements to the array
- Re-arranging the list or the order of elements in the array.
- Finding out whether the array contains a certain element
- If the array contains a certain element, what index that element has
Although you can write your own routines to perform these
operations, the Array class provides most of the methods you would need to get
the necessary information.
Practical
Learning: Introducing Operations on Arrays
|
|
- To create a new form, on the main menu, click Project -> Add New
Item...
- Click Windows
Form
- Set the name to CustomerTransactions and press Enter
- Design the form as follows:
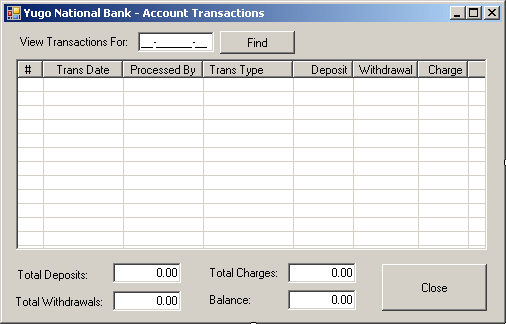 |
Control |
Text |
Name |
Other Properties |
Label |
 |
View Transactions For: |
|
|
MaskedTextBox |
 |
|
txtAccountNumber |
Mask: 00-000000-00 |
Button |
 |
Find |
btnFind |
|
ListView |
 |
|
lvwTransactions |
FullRowSelect: True
GridLines: True
View: Details |
Columns |
(Name) |
Text |
TextAlign |
Width |
colIndex |
# |
|
25 |
colTransactionDate |
Trans Date |
Center |
80 |
colProcessedBy |
Processed By |
Center |
80 |
colTransactionType |
Trans Type |
|
90 |
colDeposit |
Deposit |
Right |
|
colWithdrawal |
Withdrawal |
Right |
65 |
colChargeAmount |
Charge |
Right |
50 |
|
Label |
 |
Total Deposits: |
|
|
TextBox |
 |
|
txtTotalDeposits |
Text: 0.00
TextAlign: Right |
Label |
 |
Total Charges: |
|
|
TextBox |
 |
|
txtTotalCharges |
Text: 0.00
TextAlign: Right |
Label |
 |
Total Withdrawals: |
|
|
TextBox |
 |
|
txtTotalWithdrawals |
Text: 0.00
TextAlign: Right |
Label |
 |
Balance: |
|
|
TextBox |
 |
|
txtBalance |
Text: 0.00
TextAlign: Right |
Button |
 |
Close |
btnClose |
|
|
- Display the first form (Form1) and complete its design as follows:
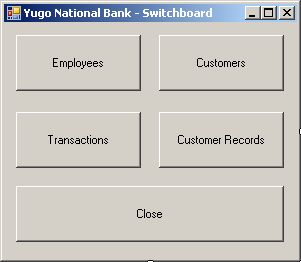 |
Button |
Text |
Name |
 |
Employees |
btnEmployees |
 |
Customers |
btnCustomers |
 |
Transactions |
btnTransactions |
 |
Customer Records |
btnCustomerRecords |
 |
Close |
btnClose |
|
- Double-click the Customer Records button
- In the top section of the file, include the CustomerTransactions.h header
file:
#pragma once
#include "Employee.h"
#include "Employees.h"
#include "AccountsRecords.h"
#include "Customer.h"
#include "Customers.h"
#include "CustomersTransactions.h"
#include "CustomerTransactions.h"
namespace YugoNationalBank1 {
|
- Implement the btnCustomerRecords_Click event as
follows:
System::Void btnCustomerRecords_Click(System::Object^ sender,
System::EventArgs^ e)
{
CustomerTransactions ^ CustRecords = gcnew CustomerTransactions;
CustRecords->ShowDialog();
}
|
- Return to the form (Form1) and double-click the Close button
- Implement its event as follows:
System::Void btnClose_Click(System::Object^ sender, System::EventArgs^ e)
{
Close();
}
|
- Execute the application to test it
- Close the forms and return to your programming environment
Adding an Element to an Array |
|
We have seen that, using the [] operator, you can add a new
element to an array. Still, to support this operation, the Array class is
equipped with the SetValue() method that is overloaded with various
versions. Here is an example that adds an element to the third position of the
array:
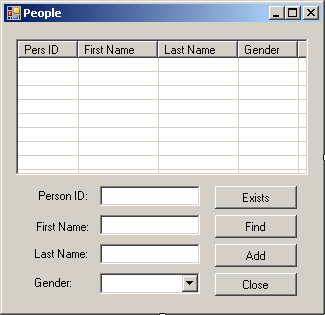
File: Exercise.h
#pragma once
#include "Person.h"
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
namespace Exercise1 {
/// <summary>
/// Summary for Exercise
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public ref class Exercise : public System::Windows::Forms::Form
{
array<CPerson ^> ^ People;
array<CPerson ^> ^ ArrayInitializer();
void ShowPeople(array<CPerson ^> ^ People);
public:
Exercise(void)
{
InitializeComponent();
//
//TODO: Add the constructor code here
//
}
protected:
/// <summary>
/// Clean up any resources being used.
/// </summary>
~Exercise()
{
if (components)
{
delete components;
}
}
. . . No Change
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container ^components;
#pragma region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
{
. . . No Change
}
#pragma endregion
private: System::Void Exercise_Load(System::Object^ sender, System::EventArgs^ e)
{
array<CPerson ^> ^ persons = ArrayInitializer();
ShowPeople(persons);
}
private: System::Void btnAdd_Click(System::Object^ sender, System::EventArgs^ e)
{
CPerson ^ pers = gcnew CPerson;
pers->PersonID = int::Parse(txtPersonID->Text);
pers->FirstName = txtFirstName->Text;
pers->LastName = txtLastName->Text;
if (cbxGenders->Text == L"Male")
pers->Gender = L"Male";
else if (cbxGenders->Text == L"Female")
pers->Gender = L"Female";
else
pers->Gender = L"Unknown";
People->SetValue(pers, pers->PersonID);
ShowPeople(People);
}
};
}
File: Exercise.cpp
#include <windows.h>
#include "Exercise.h"
namespace Exercise1
{
array<CPerson ^> ^ Exercise::ArrayInitializer()
{
People = gcnew array<CPerson ^>(8);
for(int i = 0; i < 8; i++)
{
People[i] = gcnew CPerson;
People[i]->PersonID = 0;
People[i]->FirstName = L"";
People[i]->LastName = L"";
People[i]->Gender = L"Unknown";
}
return People;
}
void Exercise::ShowPeople(array<CPerson ^> ^ People)
{
lvwPeople->Items->Clear();
for each(CPerson ^ pers in People)
{
ListViewItem ^ lviPerson =
gcnew ListViewItem(pers->PersonID->ToString());
lviPerson->SubItems->Add(pers->FirstName);
lviPerson->SubItems->Add(pers->LastName);
lviPerson->SubItems->Add(pers->Gender);
lvwPeople->Items->Add(lviPerson);
}
}
}
int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
Application::Run(gcnew CExercise1::Exercise);
return 0;
}
When the Array::SetValue() method is called, it
replaces the element at the indicated position.
Getting an Element From an Array |
|
The reverse of adding an item to an array is to retrieve
one. You can do this with the [] operator. To support this operation, the Array
class is equipped with the GetValue() method that comes in various
versions. Here is an example of calling it:
System::Void lvwPeople_ItemSelectionChanged(System::Object^ sender,
System::Windows::Forms::ListViewItemSelectionChangedEventArgs^ e)
{
CPerson ^ pers = static_cast<CPerson ^>(People->GetValue(e->ItemIndex));
txtPersonID->Text = pers->PersonID->ToString();
txtFirstName->Text = pers->FirstName;
txtLastName->Text = pers->LastName;
cbxGenders->Text = pers->Gender->ToString();
}
When calling this method, make sure you provide a valid
index, if you do not, you would get an IndexOutOfRangeException
exception.
|
|