
Although data entry
can be performed on a data grid, Microsoft Windows provides more
professional objects to create new records. Because the Microsoft .NET
Framework is so huge as a library and so powerful, there are various
techniques you can use to perform data entry.
|
Practical
Learning: Performing Data Entry
|
|
- Open SQL Query Analyzer
- To create a new database, type the following
IF EXISTS (SELECT *
FROM master..sysdatabases
WHERE name = N'Familia')
DROP DATABASE Familia
GO
CREATE DATABASE Familia
GO
|
- Press F5 to execute the statement
- To create a new table in the above database, delete the above code and
type the following:
USE Familia
GO
IF EXISTS(SELECT name
FROM sysobjects
WHERE name = N'Genders'
AND type = 'Genders')
DROP TABLE Genders
GO
CREATE TABLE Genders (
GenderID int NOT NULL PRIMARY KEY Identity(1, 1),
Gender Varchar(20) NOT NULL)
GO
INSERT INTO Genders (Gender) VALUES ('Unknown');
INSERT INTO Genders (Gender) VALUES ('Female');
INSERT INTO Genders (Gender) VALUES ('Male');
|
- Press F5 to execute the statement
- To create a new table in the above database, delete the above code and
type the following:
IF EXISTS(SELECT name
FROM sysobjects
WHERE name = N'Persons'
AND type = 'Persons')
DROP TABLE Persons
GO
CREATE TABLE Persons (
PersonID int Primary Key NOT NULL Identity(1, 1),
FirstName Varchar(20) NULL,
LastName Varchar(20) NOT NULL,
GenderID int DEFAULT 1 REFERENCES Genders(GenderID),
Notes Text)
GO
INSERT INTO Persons (FirstName, LastName, GenderID, Notes)
VALUES('Massimo', 'Leandro', 2, 'One of the most hard working employees');
INSERT INTO Persons (FirstName, LastName, GenderID, Notes)
VALUES('Christina', 'Cassine', 1, 'Wonderful employees, always on time');
INSERT INTO Persons (FirstName, LastName, GenderID, Notes)
VALUES('Helene', 'Knowles', 1, 'Has been in the company for a while');
INSERT INTO Persons (FirstName, LastName, GenderID, Notes)
VALUES('Alan', 'Holmes', 3, '');
GO
|
- Press F5 to execute the statement
- Open Visual C++ .NET and create a new Windows Forms Application named DataEntry2
- From Server Explorer, open the server that holds the above database then
expand the Familia database followed by the Tables node
- Drag the Persons table and drop it on the form
- In the same way, drag Genders and drop it
on the form
- Under the form, right-click sqlDataAdapter1 or sqlDataAdapter2 and click Generate Dataset...
- While the New radio button is selected, change the name of the dataset to dsPersons
- Select both check boxes to add both tables to the data set:
- Click OK
- Using the controls on the Toolbox, design the form as follows:
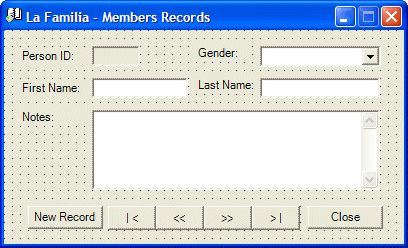 |
Control |
Text |
Name |
Additional Properties |
Label |
Person ID: |
|
|
TextBox |
|
txtPersonID |
TextAlign: Right
ReadOnly: True |
Label |
First Name: |
|
|
TextBox |
|
txtFirstName |
|
Label |
Last Name: |
|
|
TextBox |
|
txtLastName |
|
Label |
Gender: |
|
|
ComboBox |
|
cboGenderID |
DropDownStyle: DropDownList |
Label |
Notes: |
|
|
TextBox |
|
txtNotes |
Multiline: True
ScrollBars: Vertical |
Button |
New Record |
btnNewRecord |
|
Button |
| < |
btnFirst |
|
Button |
<< |
btnPrevious |
|
Button |
>> |
btnNext |
|
Button |
> | |
btnLast |
|
Button |
Close |
btnClose |
|
|
- Using the DataBindings and other fields of the Properties window, bind the
controls as follows:
Control Name |
DataBindings |
Other |
Type |
Value |
txtPersonID |
Text |
dsPersons1 - Persons.PersonID |
|
txtFirstName |
Text |
dsPersons1 - Persons.FirstName |
|
txtLastName |
Text |
dsPersons1 - Persons.LastName |
|
cboGenderID |
SelectedValue |
dsPersons1 - Persons.GenderID |
DataSource: dsPersons1.Genders
DisplayMember: Gender
ValueMember: GenderID |
chkIsMarried |
Checked |
dsPersons1 - Persons.IsMarried |
|
txtNotes |
Text |
dsPersons1 - Persons.Notes |
|
- Double-click an empty area of the form and change the Load event as
follows:
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
this->sqlDataAdapter1->Fill(this->dsPersons1);
this->sqlDataAdapter2->Fill(this->dsPersons1);
}
|
- Press Ctrl + F5 to test the application
- After viewing the first record, close the form
- To allow the user to navigate through records, double-click the | <,
<<, >>, and > | buttons
- Implement their events as
follows:
private: System::Void btnFirst_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1, S"Persons")->Position = 0;
}
private: System::Void btnPrevious_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position =
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position - 1;
}
private: System::Void btnNext_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position =
this->BindingContext->get_Item(dsPersons1,
"Persons")->Position + 1;
}
private: System::Void btnLast_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position =
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Count - 1;
}
|
- Press Ctrl + F5 to test the application and navigate through records
back and forth
- Close the form
- To allow the user to create a new record, double-click the New
Record button
- On the form, double the Close button
- Implement both events as follows:
#pragma once
namespace DataEntry2
{
using namespace System;
using namespace System::ComponentModel;
using namespace System::Collections;
using namespace System::Windows::Forms;
using namespace System::Data;
using namespace System::Drawing;
/// <summary>
/// Summary for Form1
///
/// WARNING: If you change the name of this class, you will need to change the
/// 'Resource File Name' property for the managed resource compiler tool
/// associated with all .resx files this class depends on. Otherwise,
/// the designers will not be able to interact properly with localized
/// resources associated with this form.
/// </summary>
public __gc class Form1 : public System::Windows::Forms::Form
{
public:
Form1(void)
{
InitializeComponent();
}
protected:
void Dispose(Boolean disposing)
{
if (disposing && components)
{
components->Dispose();
}
__super::Dispose(disposing);
}
private: System::Data::SqlClient::SqlConnection * sqlConnection1;
private: System::Data::SqlClient::SqlCommand * sqlSelectCommand2;
private: System::Data::SqlClient::SqlCommand * sqlInsertCommand2;
private: System::Data::SqlClient::SqlCommand * sqlUpdateCommand2;
private: System::Data::SqlClient::SqlCommand * sqlDeleteCommand2;
private: System::Data::SqlClient::SqlDataAdapter * sqlDataAdapter2;
private: DataEntry2::dsPersons * dsPersons1;
private: System::Windows::Forms::Label * label1;
private: System::Windows::Forms::Label * label2;
private: System::Windows::Forms::Label * label3;
private: System::Windows::Forms::Label * label4;
private: System::Windows::Forms::Label * label5;
private: System::Windows::Forms::TextBox * btnPersonID;
private: System::Windows::Forms::TextBox * btnFirstName;
private: System::Windows::Forms::TextBox * btnLastName;
private: System::Windows::Forms::ComboBox * cboGenderID;
private: System::Windows::Forms::TextBox * txtNotes;
private: System::Windows::Forms::Button * btnFirst;
private: System::Windows::Forms::Button * btnPrevious;
private: System::Windows::Forms::Button * btnLast;
private: System::Windows::Forms::Button * btnNext;
private: System::Windows::Forms::Button * btnClose;
private: System::Windows::Forms::Button * btnNewRecord;
private: System::Data::SqlClient::SqlCommand * sqlSelectCommand1;
private: System::Data::SqlClient::SqlCommand * sqlInsertCommand1;
private: System::Data::SqlClient::SqlCommand * sqlUpdateCommand1;
private: System::Data::SqlClient::SqlCommand * sqlDeleteCommand1;
private: System::Data::SqlClient::SqlDataAdapter * sqlDataAdapter1;
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container * components;
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
void InitializeComponent(void)
{
. . .
}
private: System::Void Form1_Load(System::Object * sender, System::EventArgs * e)
{
this->sqlDataAdapter1->Fill(this->dsPersons1);
this->sqlDataAdapter2->Fill(this->dsPersons1);
}
private: System::Void btnFirst_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1, S"Persons")->Position = 0;
// If the user changes anything in any field and moves to another record,
// update the data set (this is how it is done eveywhere, including MS SQL Server,
// MS Access, Paradox, etc
this->sqlDataAdapter1->Update(this->dsPersons1);
}
private: System::Void btnPrevious_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position = this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position - 1;
this->sqlDataAdapter1->Update(this->dsPersons1);
}
private: System::Void btnNext_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position =
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position + 1;
this->sqlDataAdapter1->Update(this->dsPersons1);
}
private: System::Void btnLast_Click(System::Object * sender, System::EventArgs * e)
{
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Position =
this->BindingContext->get_Item(dsPersons1,
S"Persons")->Count - 1;
this->sqlDataAdapter1->Update(this->dsPersons1);
}
private: System::Void btnNewRecord_Click(System::Object * sender, System::EventArgs * e)
{
// We will use the same button to create a new record and update it
// Find out what is the current state of the button
if( this->btnNewRecord->Text->Equals(S"New Record") )
{
// Since the user clicked the New Record button, prepare to create a new record
// We are using exception handling in case something goes wrong
try {
this->BindingContext->get_Item(this->dsPersons1, S"Persons")->EndCurrentEdit();
this->BindingContext->get_Item(this->dsPersons1, S"Persons")->AddNew();
// Since the fields are now empty, give focus to the first control that can be edited
this->cboGenderID->Focus();
// Since the user is currently creating a new record, change the caption of the button
this->btnNewRecord->Text = S"Update";
}// Did something go wrong?
catch(System::Exception* eEdit)
{
MessageBox::Show(eEdit->Message);
}
}
else // Since the user is ready with a new record, acknowledge it
{
// Update the whole data set
this->sqlDataAdapter1->Update(this->dsPersons1);
// Behave as if we were moving to the last record
btnLast_Click(sender, e);
// Since the new record has been added, change the caption of the New Record button
this->btnNewRecord->Text = S"New Record";
}
}
private: System::Void btnClose_Click(System::Object * sender, System::EventArgs * e)
{
Close();
}
};
}
|
- Execute the application and click the New Record button
- Enter a new record and click Update
- Close the form
|
|