 |
Introduction to Collections |
|
Fundamentals of Collections
Introduction
A collection is a group of values or objects that are treated as a unit. The objects must be either of the same type or compatible. The values or objects should be easily added to the collection, located in the collection, or removed from the collection.
To support collections, the Visual Basic language provides a class named Collection.
Starting a Collection
To start a collection, declare a variable of type Collection. Of course, you must initialize it using the New operator. Here is an example that starts a collection:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Dim employees As New Collection
</script>
<title>Exercise</title>
</head>
<body>
<h3>Exercise</h3>
<%
Dim numbers As Collection
numbers = New Collection
%>
</body>
</html>
Introduction to Using a Collection
There are various ways you can use a collection and we will go through them in this and the rest of our lessons. The primary way to use a collection is by a webcontrol that is specially made for them. To support collections, the webcontrols of ASP.NET have a member named DataSource that is a string-type. Therefore, the primary technique to use a collection is to assign its variable to this member of a webcontrol. This can be done as follows:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim colSocialSciences As New Collection
lbxSocialSciences.DataSource = steps
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSocialSciences" runat="server">
<asp:ListBox id="lbxSocialSciences" runat="server"></asp:ListBox>
</form>
</body>
</html>
Binding the Data to a Webcontrol
After specifying the source of the values of a webcontrol, you must apply the values to the control. To support this, the classes of the webcontrols that support collections are equipped with a method named DataBind. You can simply call it on the variable of the webcontrol. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim colSocialSciences As New Collection
lbxSocialSciences.DataSource = steps
lbxSocialSciences.DataBind()
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSocialSciences" runat="server">
<asp:ListBox id="lbxSocialSciences" runat="server"></asp:ListBox>
</form>
</body>
</html>
Primary Operations on a Collection
Adding a Value to a Collection
The most fundamental operation performed on a collection is to add a value to it. To support this, the Collection class is equipped with a method named Add. It takes one argument as the value to add. You can call this method every time you need to add a value. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim colSocialSciences As New Collection
colSocialSciences.Add("Sociology")
colSocialSciences.Add("Psychology")
colSocialSciences.Add("Philosoply")
colSocialSciences.Add("Linguistics")
colSocialSciences.Add("Anthropology")
colSocialSciences.Add("History")
colSocialSciences.Add("Geography")
colSocialSciences.Add("Demography")
colSocialSciences.Add("Ethnicity")
colSocialSciences.Add("Archaeology")
colSocialSciences.Add("Political Science")
colSocialSciences.Add("Law")
colSocialSciences.Add("Education")
colSocialSciences.Add("Criminology")
colSocialSciences.Add("Economy")
lbxSocialSciences.DataSource = colSocialSciences
lbxSocialSciences.DataBind()
End Sub
</script>
<title>Social Science Studies</title>
</head>
<body>
<h3>Social Science Studies</h3>
<form id="frmSocialSciences" runat="server">
<asp:ListBox id="lbxSocialSciences" Height="200px" runat="server"></asp:ListBox>
</form>
</body>
</html>
This would produce:
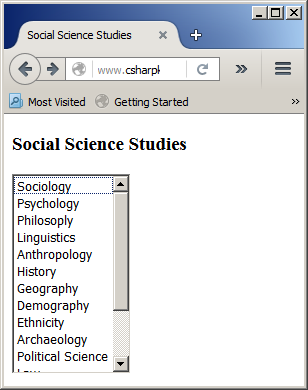
For Each Item of a Collection
To let you access each item of a collection, the Visual
Basic language provides the For Each...Next statement. The formula to use it is:
For Each element [ As data-type ] In collection
statements
options
Next [ element ]
You can first declare a variable that uses the same type as
the items of the collection. This variable will be used as the element.
If you first declare the variable, then omit the As data-type expression.
This is followed by the In keyword and the collection you will use. The
statement ends with the Next keyword. In the statements section,
specify the element. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim classification As String
Dim classifications As New Collection
classifications.Add("True Positive")
classifications.Add("False Positive")
classifications.Add("True Negative")
classifications.Add("False Negative")
For Each classification in classifications
Response.Write(classification & "<br>")
Next
%>
</body>
</html>
This would produce:
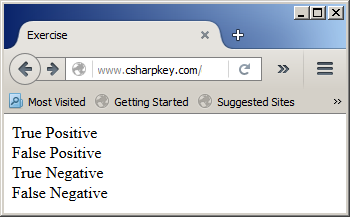
As an option, you can add the element after the Next keyword. Here
is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim classification As String
Dim classifications As New Collection
classifications.Add("True Positive")
classifications.Add("False Positive")
classifications.Add("True Negative")
classifications.Add("False Negative")
For Each classification in classifications
Response.Write(classification & "<br>")
Next classification
%>
</body>
</html>
You don't have to first declare a variable for the item.
After the For Each expression, you can specify the name of the variable
followed by As and its data type. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head>
<title>Exercise</title>
</head>
<body>
<%
Dim classifications As New Collection
classifications.Add("True Positive")
classifications.Add("False Positive")
classifications.Add("True Negative")
classifications.Add("False Negative")
For Each classification As String in classifications
Response.Write(classification & "<br>")
Next classification
%>
</body>
</html>
Primary Characteristics of a Collection
The Number of Items in a Collection
To let you get the number of items in a collection, the Collection class is equipped with a member named Count. Here is an example of accessing this member:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim steps As New Collection
steps.Add("Planning")
steps.Add("Analysis")
steps.Add("Design")
steps.Add("Implementation")
steps.Add("Maintenance")
lbxSDLC.DataSource = steps
lbxSDLC.DataBind()
lblCounter.Text = "Number of Steps: " & CStr(steps.Count)
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSDLC" runat="server">
<asp:ListBox id="lbxSDLC" Height="95px" runat="server"></asp:ListBox>
<br><br>
<asp:Label id="lblCounter" runat="server"></asp:Label>
</form>
</body>
</html>
This would produce:
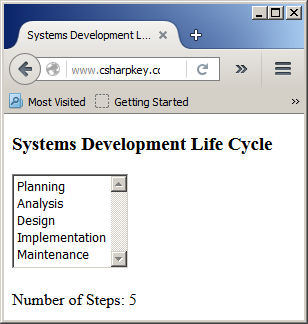
The Index/Key of an Item in the Collection
Every time you add a new item to the collection, the new item occupies a specific position. By default, the first added item occupies a position of 1, the second is at position 2, and so on. The position is also referred to as the index. You can locate an item based on its index.
Every time you add a new item to the collection as we have done so far, the
item receives a default index. The first added item receives an index of 1, the second receives an index of 2, and so on. To
let you access an item, the Collection class has a member named Item.
Based on this, to access an item, type the name of the collection followed by .Item(). In the parentheses, type the index of the item you want to access. Here are examples:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim steps As New Collection
steps.Add("Planning")
steps.Add("Analysis")
steps.Add("Design")
steps.Add("Implementation")
steps.Add("Maintenance")
lbxSDLC.DataSource = steps
lbxSDLC.DataBind()
lblCounter.Text = "Number of Steps: " & CStr(steps.Count)
lblStep1.Text = "Step 1 = " & steps.Item(1)
lblStep2.Text = "Step 2 = " & steps.Item(2)
lblStep3.Text = "Step 3 = " & steps.Item(3)
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSDLC" runat="server">
<asp:ListBox id="lbxSDLC" Height="95px" runat="server"></asp:ListBox>
<br><br>
<asp:Label id="lblCounter" runat="server"></asp:Label>
<br><br>
<asp:Label id="lblStep1" runat="server"></asp:Label>
<br>
<asp:Label id="lblStep2" runat="server"></asp:Label>
<br>
<asp:Label id="lblStep3" runat="server"></asp:Label>
</form>
</body>
</html>
This would produce:

An alternative is to omit .Item. Here are examples:
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim steps As New Collection
steps.Add("Planning")
steps.Add("Analysis")
steps.Add("Design")
steps.Add("Implementation")
steps.Add("Maintenance")
lbxSDLC.DataSource = steps
lbxSDLC.DataBind()
lblCounter.Text = "Number of Steps: " & CStr(steps.Count)
lblStep1.Text = "Step 1 = " & steps(1)
lblStep2.Text = "Step 2 = " & steps(2)
lblStep3.Text = "Step 3 = " & steps(3)
End Sub
</script>
You can use this characteristic to get an item from a
collection.
Alternatively, every time you add a new item, you can provide an index of your choice. That index is referred to as a key. To specify the key of a new item, pass a second argument to the Collection.Add() method and give the desired value. The key can be any type
but it must be unique among the other keys of the collection. Here are examples:
<%
Dim steps As New Collection
steps.Add("Planning", "P")
steps.Add("Analysis", "A")
steps.Add("Design", "D")
steps.Add("Implementation", "I")
steps.Add("Maintenance", "M")
%>
You can use the key of an item to locate its value. To do this, type the name of the collection followed by parentheses. In the parentheses, type the key of the item you want to access. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim steps As New Collection
steps.Add("Planning", "P")
steps.Add("Analysis", "A")
steps.Add("Design", "D")
steps.Add("Implementation", "I")
steps.Add("Maintenance", "M")
lbxSDLC.DataSource = steps
lbxSDLC.DataBind()
lblStep.Text = "Step D: " & steps("D")
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSDLC" runat="server">
<asp:ListBox id="lbxSDLC" Height="95px" runat="server"></asp:ListBox>
<br><br>
<asp:Label id="lblStep" runat="server"></asp:Label>
</form>
</body>
</html>
This would produce:
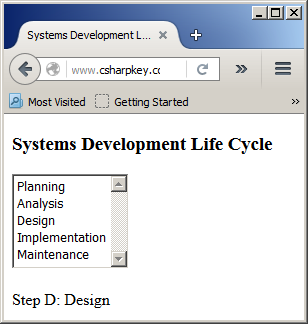
Checking Whether the Collection Contains a Certain Item
To let you find out whether a collection contains a ceretain item, the Collection class is equipped with a method named Contains. To use it, type the name of the collection followed by .Contains(). In the parentheses, enter the key of the item. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim steps As New Collection
steps.Add("Planning", "P")
steps.Add("Analysis", "A")
steps.Add("Design", "D")
steps.Add("Implementation", "I")
steps.Add("Maintenance", "M")
lbxSDLC.DataSource = steps
lbxSDLC.DataBind()
If steps.Contains("A") Then
lblContains1.Text = "Phase A, which is the second, is used to break the project in small logical sections that can each be examined, evaluated, and prepared to appropriately assign it to a competent design."
Else
lblContains1.Text = "There is no Phase A in the systems development life cycle."
End If
If steps.Contains("H") Then
lblContains2.Text = "The SDLC contains Phase H"
Else
lblContains2.Text = "There is no Phase H in the systems development life cycle."
End If
End Sub
</script>
<title>Systems Development Life Cycle</title>
</head>
<body>
<h3>Systems Development Life Cycle</h3>
<form id="frmSDLC" runat="server">
<asp:ListBox id="lbxSDLC" Height="95px" runat="server"></asp:ListBox>
<br><br>
<asp:Label id="lblContains1" runat="server"></asp:Label>
<br><br>
<asp:Label id="lblContains2" runat="server"></asp:Label>
</form>
</body>
</html>
This would produce:
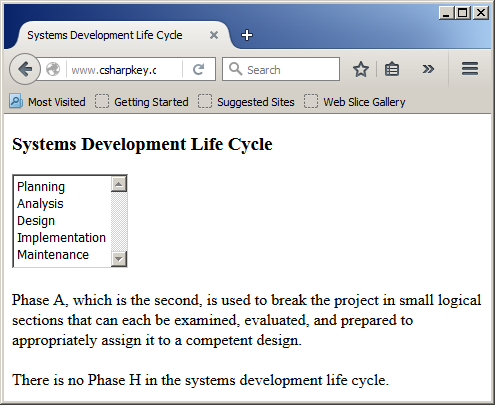
Removing Items From a Collection
To let you remove an item from a collection, the Collection class is equipped with a method named Remove. It is used as in collection-name.Remove(argument). If you want to remove an item based on its index, pass that index to the parentheses of the method. If you want to remove an item based on its key, pass that key in the parentheses of the method.
Clearing a Collection
To let you delete all items from a collection, the Collection class is equipped with a method named Clear. Here are examples:
Collections and Classes
Creating a Collection of Objects
You can create a collection of objects, in which case the members are based on a class. You start the variable normally. One way to add an object is to first define it (we will learn other techniques) through a variable/object, then pass that object as the argument of the Collection.Add(). Here are examples:
<script Language="VB" runat="server">
Public Class Employee
Public EmployeeNumber As String
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim staff As Employee
Dim employees As New Collection
Dim employeeRecord As New Employee
staff = New Employee
staff.EmployeeNumber = "953-084"
staff.FirstName = "Anthony"
staff.LastName = "Walters"
staff.HourlySalary = 18.52
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "204-815"
staff.FirstName = "Jeannine"
staff.LastName = "Rocks"
staff.HourlySalary = 22.74
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "728-411"
staff.FirstName = "Aaron"
staff.LastName = "Gibson"
staff.HourlySalary = 35.08
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "528-492"
staff.FirstName = "Stephen"
staff.LastName = "Brothers"
staff.HourlySalary = 24.93
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "303-415"
staff.FirstName = "Laura"
staff.LastName = "Edom"
staff.HourlySalary = 15.75
employees.Add(staff)
End Sub
</script>
Operations on a Collection of Objects
The characteristics and actions we reviewed for a collection of regular values also apply to a list of objects. For example, to know the number of objects in a collection, you can just get the value of its Count. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script Language="VB" runat="server">
Public Class Employee
Public EmployeeNumber As String
Public FirstName As String
Public LastName As String
Public HourlySalary As Double
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim staff As Employee
Dim employees As New Collection
Dim employeeRecord As New Employee
staff = New Employee
staff.EmployeeNumber = "953-084"
staff.FirstName = "Anthony"
staff.LastName = "Walters"
staff.HourlySalary = 18.52
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "204-815"
staff.FirstName = "Jeannine"
staff.LastName = "Rocks"
staff.HourlySalary = 22.74
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "728-411"
staff.FirstName = "Aaron"
staff.LastName = "Gibson"
staff.HourlySalary = 35.08
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "528-492"
staff.FirstName = "Stephen"
staff.LastName = "Brothers"
staff.HourlySalary = 24.93
employees.Add(staff)
staff = New Employee
staff.EmployeeNumber = "303-415"
staff.FirstName = "Laura"
staff.LastName = "Edom"
staff.HourlySalary = 15.75
employees.Add(staff)
lblCounter.Text = "Number of Employees: " & CStr(employees.Count)
End Sub
</script>
<title>Exercise</title>
</head>
<body>
<h3>Employees Records</h3>
<form id="frmEmployees" runat="server">
<asp:Label id="lblCounter" runat="server"></asp:Label>
</form>
</body>
</html>
This would produce:
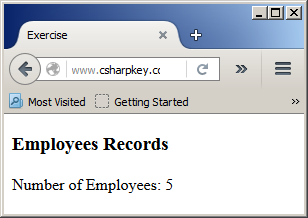
As mentioned previously, if you add objects to a collection by passing only one argument to the Collection.Add() method, each new object receives an incrementing 1-based index. You can use such an index to locate an object. Here is an example:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script runat="server">
Public Class EarthLayer
Public Layer As String
Public Thickness As String
Public Composition As String
Public MassRatio As String
End Class
Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim el As EarthLayer
Dim layers As New Collection
Dim layerChosen As New EarthLayer
el = New EarthLayer
el.Layer = "Crust"
el.Thickness = "3-25 Miles"
el.Composition = "Calcium (Ca), Sodium(Na)"
el.MassRatio = "0.0473%"
layers.Add(el)
el = New EarthLayer
el.Layer = "Mantle"
el.Thickness = "1800 Miles"
el.Composition = "Iron (Fe), Magnesium (Mg), Aluminium (Al), Silicon (Si), Oxygen(O)"
el.MassRatio = "67.3%"
layers.Add(el)
el = New EarthLayer
el.Layer = "Outer Core"
el.Thickness = "1400 Miles"
el.Composition = "Iron (Fe), Sulfur (S), Oxygen (O)"
el.MassRatio = "30.8%"
layers.Add(el)
el = New EarthLayer
el.Layer = "Inner Core"
el.Thickness = "800 Miles"
el.Composition = "Iron (Fe)"
el.MassRatio = "1.7"
layers.Add(el)
layerChosen = layers(2)
lblLayer.Text = layerChosen.Layer
lblThickness.Text = layerChosen.Thickness
lblComposition.Text = layerChosen.Composition
lblMassRatio.Text = layerChosen.MassRatio
End Sub
</script>
<title>Earth Layers</title>
</head>
<body>
<div align="center">
<h3>Earth Layers</h3>
<form id="frmLayers" runat="server">
<table border=0>
<tr>
<td><b>Layer:</b></td>
<td><asp:Label id="lblLayer" runat="server"></asp:Label></td>
</tr>
<tr>
<td><b>Thickness:</b></td>
<td><asp:Label id="lblThickness" runat="server" /></td>
</tr>
<tr>
<td><b>Composition:</b></td>
<td><asp:Label id="lblComposition" runat="server" /></td>
</tr>
<tr>
<td><b>Mass Ratio:</b></td>
<td><asp:Label id="lblMassRatio" runat="server"></asp:Label></td>
</tr>
</table>
</form>
</body>
</html>
This would produce:
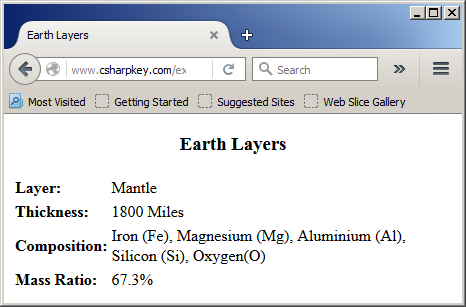
Otherwise, you can create your own keys to objects you are adding to a collection. You can use such a key to locate an object. From there, you can access the members of the class. Here is an example of finding an object in a collection based on its key:
<%@ Page Language="VB" %>
<!DOCTYPE html>
<html>
<head runat="server">
<script Language="VB" runat="server">
Public Class USState
Public State As String
Public AreaSqrKm As Integer
Public AreaSqrMi As Integer
Public AdmissionToUnionYear As Integer
Public AdmissionToUnionOrder As Integer
Public Capital As String
End Class
Sub btnSelectClick(ByVal sender As Object, ByVal e As EventArgs)
Dim uss As USState
Dim Code As String
Dim states As New Collection
Dim stateSelected As New USState
uss = New USState
uss.State = "Alabama"
uss.AreaSqrKm = 135775
uss.AreaSqrMi = 52423
uss.AdmissionToUnionYear = 1819
uss.AdmissionToUnionOrder = 22
uss.Capital = "Montgomery"
states.Add(uss, "AL")
uss = New USState
uss.State = "Alaska"
uss.AreaSqrKm = 1700139
uss.AreaSqrMi = 656424
uss.AdmissionToUnionYear = 1959
uss.AdmissionToUnionOrder = 49
uss.Capital = "Juneau"
states.Add(uss, "AK")
uss = New USState
uss.State = "Arizona"
uss.AreaSqrKm = 295276
uss.AreaSqrMi = 114006
uss.AdmissionToUnionYear = 1912
uss.AdmissionToUnionOrder = 48
uss.Capital = "Phoenix"
states.Add(uss, "AZ")
uss = New USState
uss.State = "Arkansas"
uss.AreaSqrKm = 137742
uss.AreaSqrMi = 53182
uss.AdmissionToUnionYear = 1836
uss.AdmissionToUnionOrder = 25
uss.Capital = "Little Rock"
states.Add(uss, "AR")
uss = New USState
uss.State = "California"
uss.AreaSqrKm = 424002
uss.AreaSqrMi = 163707
uss.AdmissionToUnionYear = 1850
uss.AdmissionToUnionOrder = 31
uss.Capital = "Sacramento"
states.Add(uss, "CA")
uss = New USState
uss.State = "Colorado"
uss.AreaSqrKm = 269620
uss.AreaSqrMi = 104100
uss.AdmissionToUnionYear = 1876
uss.AdmissionToUnionOrder = 38
uss.Capital = "Denver"
states.Add(uss, "CO")
code = txtStateCode.Text
If states.Contains(code) Then
stateSelected = states(code)
lblState.Text = stateSelected.State
lblAreaSqrKm.Text = FormatNumber(stateSelected.AreaSqrKm, 0)
lblAreaSqrMi.Text = FormatNumber(stateSelected.AreaSqrMi, 0)
lblAdmissionToUnionYear.Text = CStr(stateSelected.AdmissionToUnionYear)
lblAdmissionToUnionOrder.Text = CStr(stateSelected.AdmissionToUnionOrder)
lblCapital.Text = stateSelected.Capital
Else
lblMessage.Visible = True
lblMessage.Text = "There is no state with that code."
lblState.Text = ""
lblAreaSqrKm.Text = ""
lblAreaSqrMi.Text = ""
lblAdmissionToUnionYear.Text = ""
lblAdmissionToUnionOrder.Text = ""
lblCapital.Text = ""
End If
End Sub
</script>
<title>States Statistics</title>
</head>
<body>
<div align="center">
<h3>States Statistics</h3>
<form id="frmStates" runat="server">
<table>
<tr>
<td><b>Enter State Code:</b>
<asp:TextBox id="txtStateCode" Width="40px" runat="server" />
<asp:Button id="btnFind" text="Find"
OnClick="btnSelectClick"
runat="server"></asp:Button> </td>
<td> </td>
</tr>
<tr>
<td>State</td>
<td><asp:Label id="lblState" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Area (Sqr-Km):</td>
<td><asp:Label id="lblAreaSqrKm" runat="server" /></td>
</tr>
<tr>
<td>Area (Sqr-Miles):</td>
<td><asp:Label id="lblAreaSqrMi" runat="server" /></td>
</tr>
<tr>
<td>Year of Admission to the Union</td>
<td><asp:Label id="lblAdmissionToUnionYear" runat="server"></asp:Label></td>
</tr>
<tr>
<td>Order of Admission to the Union:</td>
<td><asp:Label id="lblAdmissionToUnionOrder" runat="server" /></td>
</tr>
<tr>
<td>Capital:</td>
<td><asp:Label id="lblCapital" runat="server" /></td>
</tr>
</table>
<asp:Label id="lblMessage" Visible="False" runat="server" />
</form>
</body>
</html>
Here is an example of using the webpage:
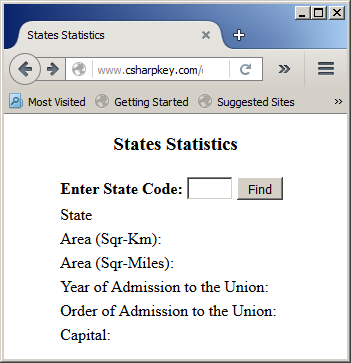
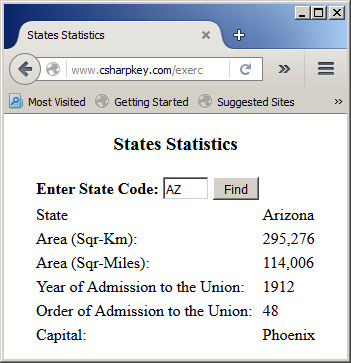
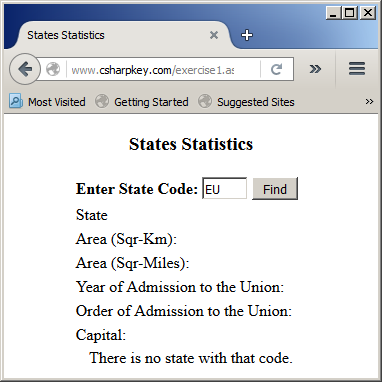
The other operations use the same approach: Look for, or locate an object based on its index or key. Once you have the object, you can access the members of the class and use them as you see fit.
|