This exercise uses a panel, a button, and three
scroll bars combined to create an application.
Prerequisites:
Dialog Box
Group Boxes
Radio Buttons
Text Boxes
Panel
Button
Scroll Bars
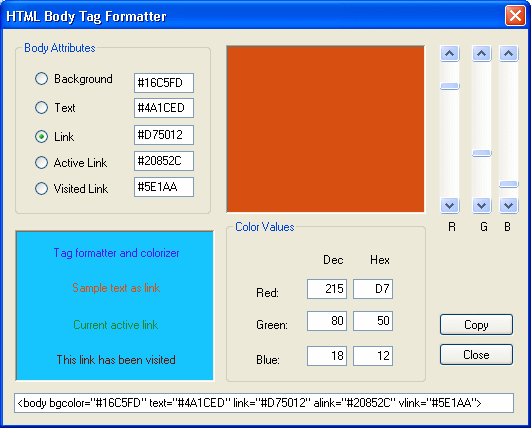
Practical
Learning: Introducing Scroll Bar Controls
|
|
- Start a new Windows Forms Application named BodyTag1
- Set form's FormBorderStyle property to FixedDialog
- Set its MaximizeBox and its MinimizeBox properties to
False each
- Design the form as follows:
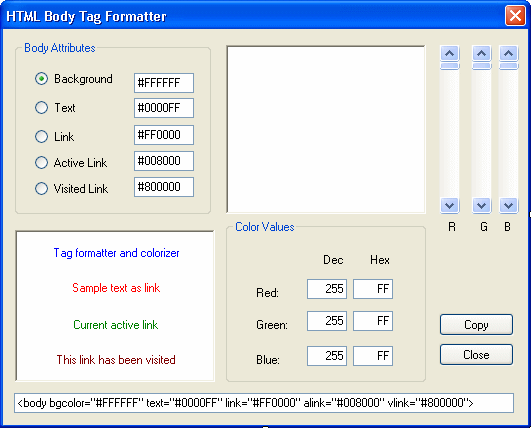 |
Control |
Name |
Text |
Other Properties |
GroupBox |
 |
|
Body Attributes |
|
RadioButton |
 |
rdoBackground |
Background |
Checked: True |
TextBox |
 |
txtBackground |
#FFFFFF |
|
RadioButton |
 |
rdoText |
Text |
|
TextBox |
 |
txtText |
#0000FF |
|
RadioButton |
 |
rdoLink |
Link |
|
TextBox |
 |
txtLink |
#FF0000 |
|
RadioButton |
 |
rdoActiveLink |
Active Link |
|
TextBox |
 |
txtActiveLink |
#008000 |
|
RadioButton |
 |
rdoVisitedLink |
Visited Link |
|
TextBox |
 |
txtVisitedLink |
#800000 |
|
Panel |
 |
pnlPreview |
|
BackColor: White
BorderStyle: Fixed3D |
VScrollBar |
 |
scrRed |
|
LargeChange: 1
Maximum: 255
Value: 0 |
VScrollBar |
 |
scrGreen |
|
LargeChange: 1
Maximum: 255
Value: 0 |
VScrollBar |
 |
scrBlue |
|
LargeChange: 1
Maximum: 255
Value: 0 |
Label |
 |
|
R |
|
Label |
 |
|
G |
|
Label |
 |
|
B |
|
Panel |
 |
pnlBody |
|
BackColor: White
BorderStyle: Fixed3D |
TextBox |
 |
txtTextPreview |
Body tag formatter and colorizer |
BorderStyle: None
ForeColor: Blue
TextAlign: Center |
TextBox |
 |
txtLinkPreview |
Sample text as link |
BorderStyle: None
ForeColor: Red
TextAlign: Center |
TextBox |
 |
txtALinkPreview |
Current active link |
BorderStyle: None
ForeColor: Green
TextAlign: Center |
TextBox |
 |
txtVLinkPreview |
This link has been visited |
BorderStyle: None
ForeColor: Maroon
TextAlign: Center |
GroupBox |
 |
|
Color Values |
|
Label |
 |
|
Red: |
|
TextBox |
 |
txtRed |
255 |
|
Label |
 |
|
Green: |
|
TextBox |
 |
txtGreen |
255 |
|
Label |
 |
|
Blue: |
|
TextBox |
 |
txtBlue |
255 |
|
Button |
 |
btnClose |
Close |
|
Button |
 |
btnCopy |
Copy |
|
TextBox |
 |
txtResult |
<body bgcolor="#FFFFFF" text="#0000FF" link="#FF0000" alink="#008000" vlink="#800000"> |
|
- Right-click the form and click View Code. Declare the following private
String pointers:
#pragma once
namespace BodyTag1 {
. . .
public ref class Form1 : public System::Windows::Forms::Form
{
public:
Form1(void)
{
InitializeComponent();
//
//TODO: Add the constructor code here
//
}
protected:
/// <summary>
/// Clean up any resources being used.
/// </summary>
~Form1()
{
if (components)
{
delete components;
}
}
. . .
private:
/// <summary>
/// Required designer variable.
/// </summary>
System::ComponentModel::Container ^components;
typedef String ^ string;
string HexBG, HexText, HexLink, HexALink, HexVLink;
. . .
|
- In Class View, expand BodyTag1 and expand the other BodyTag1.
Right-click Form1 -> Add -> Add Function...
- Set the method options as follows:
Return Type: void
Function Name: ApplyColor
Access: private
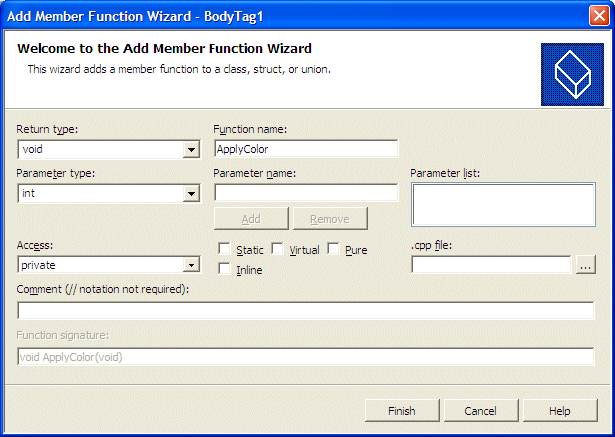
- Click Finish and implement the method as follows:
// This method is shared by the different controls
// when they need to update the application
void ApplyColor(void)
{
// Retrieve the current hexadecimal colors from their Edit controls
HexBG = txtBackground->Text;
HexText = txtText->Text;
HexLink = txtLink->Text;
HexALink = txtActiveLink->Text;
HexVLink = txtVisitedLink->Text;
int iRed = 255 - scrRed->Value;
int iGreen = 255 - scrGreen->Value;
int iBlue = 255 - scrBlue->Value;
// Get the integral position of each ScrollBar control
// and convert it to String
string strRed = Convert::ToString(iRed);
string strGreen = Convert::ToString(iGreen);
string strBlue = Convert::ToString(iBlue);
// Display the position of each ScrollBar
// in its corresponding Edit control
txtDecRed->Text = strRed;
txtDecGreen->Text = strGreen;
txtDecBlue->Text = strBlue;
// Change the color of the Preview panel
// according to the values of the ScrollBar positions
pnlPreview->BackColor =
Drawing::Color::FromArgb(iRed, iGreen, iBlue);
string strHexRed = iRed.ToString(L"X");
string strHexGreen = iGreen.ToString(L"X");
string strHexBlue = iBlue.ToString(L"X");
txtHexRed->Text = strHexRed;
txtHexGreen->Text = strHexGreen;
txtHexBlue->Text = strHexBlue;
if( iRed < 10 )
strHexRed = String::Concat(L"0", iRed.ToString(L"X"));
if( iGreen < 10 )
strHexGreen = String::Concat(L"0", iGreen.ToString(L"X"));
if( iBlue < 10 )
strHexBlue = String::Concat(L"0", iBlue.ToString(L"X"));
// Get the position of each ScrollBar control
// Create a hexadecimal color starting with #
// And display the color in the appropriate Edit control
if( rdoBackground->Checked == true )
{
string BG = L"#";
BG = BG->Concat(BG, strHexRed);
BG = BG->Concat(BG, strHexGreen);
BG = BG->Concat(BG, strHexBlue);
txtBackground->Text = BG;
pnlBody->BackColor = pnlPreview->BackColor;
txtTextPreview->BackColor = pnlPreview->BackColor;
txtLinkPreview->BackColor = pnlPreview->BackColor;
txtALinkPreview->BackColor = pnlPreview->BackColor;
txtVLinkPreview->BackColor = pnlPreview->BackColor;
HexBG = txtBackground->Text;
}
else if( rdoText->Checked == true )
{
string Txt = L"#";
Txt = Txt->Concat(Txt, strHexRed);
Txt = Txt->Concat(Txt, strHexGreen);
Txt = Txt->Concat(Txt, strHexBlue);
txtText->Text = Txt;
txtTextPreview->ForeColor =
Drawing::Color::FromArgb(iRed, iGreen, iBlue);
HexText = txtText->Text;
}
else if( rdoLink->Checked == true )
{
string TL = L"#";
TL = TL->Concat(TL, strHexRed);
TL = TL->Concat(TL, strHexGreen);
TL = TL->Concat(TL, strHexBlue);
txtLink->Text = TL;
txtLinkPreview->ForeColor =
Drawing::Color::FromArgb(iRed, iGreen, iBlue);
HexLink = txtLink->Text;
}
else if( rdoActiveLink->Checked == true )
{
string AL = L"#";
AL = AL->Concat(AL, strHexRed);
AL = AL->Concat(AL, strHexGreen);
AL = AL->Concat(AL, strHexBlue);
txtActiveLink->Text = AL;
txtALinkPreview->ForeColor =
Drawing::Color::FromArgb(iRed, iGreen, iBlue);
HexALink = txtActiveLink->Text;
}
else if( rdoVisitedLink->Checked == true )
{
string VL = L"#";
VL = VL->Concat(VL, strHexRed);
VL = VL->Concat(VL, strHexGreen);
VL = VL->Concat(VL, strHexBlue);
txtVisitedLink->Text = VL;
txtVLinkPreview->ForeColor =
Drawing::Color::FromArgb(iRed, iGreen, iBlue);
HexVLink = txtVisitedLink->Text;
}
// Update the contents of the bottom Edit control
string BD = L"<body bgcolor=\"";
BD = BD->Concat(BD, HexBG);
BD = BD->Concat(BD, L"\" text=\"");
BD = BD->Concat(BD, HexText);
BD = BD->Concat(BD, L"\" link=\"");
BD = BD->Concat(BD, HexLink);
BD = BD->Concat(BD, L"\" alink=\"");
BD = BD->Concat(BD, HexALink);
BD = BD->Concat(BD, L"\" vlink=\"");
BD = BD->Concat(BD, HexVLink);
BD = BD->Concat(BD, L"\">");
txtResult->Text = BD;
// throw(gcnew System::NotImplementedException);
}
|
- Double-click each scroll bar and implement them as
follows:
System::Void scrRed_Scroll(System::Object^ sender,
System::Windows::Forms::ScrollEventArgs^ e)
{
ApplyColor();
}
System::Void scrGreen_Scroll(System::Object^ sender,
System::Windows::Forms::ScrollEventArgs^ e)
{
ApplyColor();
}
System::Void scrBlue_Scroll(System::Object^ sender,
System::Windows::Forms::ScrollEventArgs^ e)
{
ApplyColor();
}
|
- Execute the application to test the form
- Close the form and return to your programming environment
- When the user clicks a radio button from the Body Attributes group box,
we need to display its color on the Preview panel. When a particular button
is clicked, we will
retrieve the color of its font from the Body text box, translate that color
into red, green, and blue values, and then use those values to automatically
update the scroll bars and the edit boxes. While we are at it, we also need
to update the corresponding text box in the Body Attributes group box. Since
this functionality will be used by all radio buttons in the group, we will
use a global function to which we can pass two variables.
When the user clicks a particular radio button, that button is represented
by a text box in the lower-left Body section. We need to get the color of
that edit box and pass it to our function. Since the clicked radio button
has a corresponding text box in the Body Attributes group box, we need to
change/update that value with the hexadecimal value of the first argument.
Therefore, we will pass a String argument to our function.
In Code Editor, just after the closing curly bracket of the scrBlue_Scroll
method, create the following method:
void ClickOption(Drawing::Color Clr, string Result)
{
// These variables will hold the red, green, and blue
// values of the passed color
int Red, Green, Blue;
// Colorize the Preview panel with the passed color
pnlPreview->BackColor = Clr;
// Get the red value of the color of the Preview panel
Red = pnlPreview->BackColor.R;
// Get the green value of the color of the Preview panel
Green = pnlPreview->BackColor.G;
// Get the blue value of the color of the Preview panel
Blue = pnlPreview->BackColor.B;
// Now that we have the red, green, and blue values of the color,
// Update the scroll bars with the new values
scrRed->Value = 255 - Red;
scrGreen->Value = 255 - Green;
scrBlue->Value = 255 - Blue;
// Update the red, green, and blue values
// of the Numeric Values group box
txtDecRed->Text = Red.ToString();
txtDecGreen->Text = Green.ToString();
txtDecBlue->Text = Blue.ToString();
txtHexRed->Text = Red.ToString(L"X");
txtHexGreen->Text = Green.ToString(L"X");
txtHexBlue->Text = Blue.ToString(L"X");
// Update the string that was passed using
// the retrieved red, green, and blue values
Result = Result->Concat(Result, L"#");
Result = Result->Concat(Result, Red.ToString(L"X"));
Result = Result->Concat(Result, Green.ToString(L"X"));
Result = Result->Concat(Result, Blue.ToString(L"X"));
}
|
- In the form, double-click each radio button and implement it as follows:
System::Void rdoBackground_CheckedChanged(System::Object^ sender,
System::EventArgs^ e)
{
// If the user clicks Background radio button
// set color of the panel to that of the radio button
pnlPreview->BackColor = pnlBody->BackColor;
// Call the ClickOption() method to calculate
// the hexadecimal value of the color
ClickOption(pnlBody->BackColor, txtBackground->Text);
HexBG = txtBackground->Text;
}
System::Void rdoText_CheckedChanged(System::Object^ sender,
System::EventArgs^ e)
{
pnlPreview->BackColor = txtTextPreview->ForeColor;
ClickOption(txtTextPreview->ForeColor, txtText->Text);
HexText = txtText->Text;
}
System::Void rdoLink_CheckedChanged(System::Object^ sender,
System::EventArgs^ e)
{
pnlPreview->BackColor = txtLinkPreview->ForeColor;
ClickOption(txtLinkPreview->ForeColor, txtLink->Text);
HexLink = txtLink->Text;
}
System::Void rdoActiveLink_CheckedChanged(System::Object^ sender,
System::EventArgs^ e)
{
pnlPreview->BackColor = txtALinkPreview->ForeColor;
ClickOption(txtALinkPreview->ForeColor, txtActiveLink->Text);
HexALink = txtActiveLink->Text;
}
System::Void rdoVisitedLink_CheckedChanged(System::Object^ sender,
System::EventArgs^ e)
{
pnlPreview->BackColor = txtVLinkPreview->ForeColor;
ClickOption(txtVLinkPreview->ForeColor, txtVisitedLink->Text);
HexVLink = txtVisitedLink->Text;
}
|
- Double-click the Copy button and implement it as follows:
private: System::Void btnCopy_Click(System::Object^ sender,
System::EventArgs^ e)
{
this->txtResult->SelectAll();
this->txtResult->Copy();
}
|
- Double-click the Close button and implement it as follows:
System::Void btnClose_Click(System::Object^ sender, System::EventArgs^ e)
{
Close();
}
|
- Execute the application
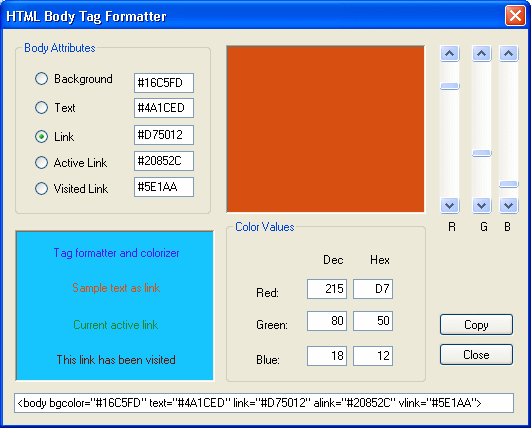
- Close the form
|
|